The time period “Memoization” comes from the Latin phrase “memorandum” (to recollect), which is often shortened to “memo” in American English, and which implies “to rework the outcomes of a perform into one thing to recollect.”.
In computing, memoization is used to hurry up laptop applications by eliminating the repetitive computation of outcomes, and by avoiding repeated calls to features that course of the identical enter.
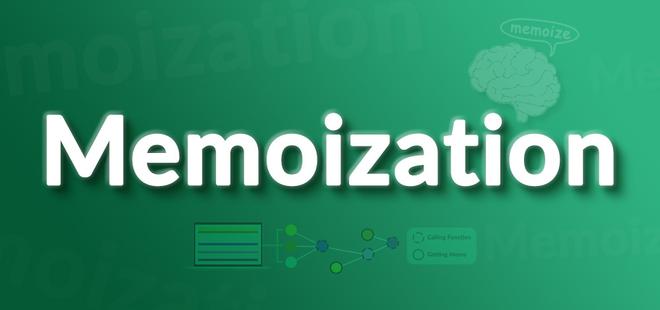
What’s memoization
Why is Memoization used?
Memoization is a particular type of caching that’s utilized in dynamic programming. The aim of caching is to enhance the efficiency of our applications and hold knowledge accessible that can be utilized later. It mainly shops the beforehand calculated results of the subproblem and makes use of the saved outcome for a similar subproblem. This removes the additional effort to calculate repeatedly for a similar downside. And we already know that if the identical downside happens repeatedly, then that downside is recursive in nature.
The place to make use of Memoization?
We will use the memoization approach the place the use of the previously-calculated outcomes comes into the image. This sort of downside is usually used within the context of recursion, particularly with issues that contain overlapping subproblems.
Let’s take an instance the place the identical subproblem repeats repeatedly.
Instance to point out the place to make use of memoization:
Allow us to attempt to discover the factorial of a quantity.
Under is a recursive methodology for locating the factorial of a quantity:
int factorial(unsigned int n)
{
if (n == 0)
return 1;
return n * factorial(n – 1);
}
What occurs if we use this recursive methodology?
When you write the whole code for the above snippet, you’ll discover that there might be 2 strategies within the code:
1. factorial(n) 2. foremost()
Now if we’ve a number of queries to search out the factorial, corresponding to discovering factorial of two, 3, 9, and 5, then we might want to name the factorial() methodology 4 occasions:
factorial(2) factorial(3) factorial(9) factorial(5)
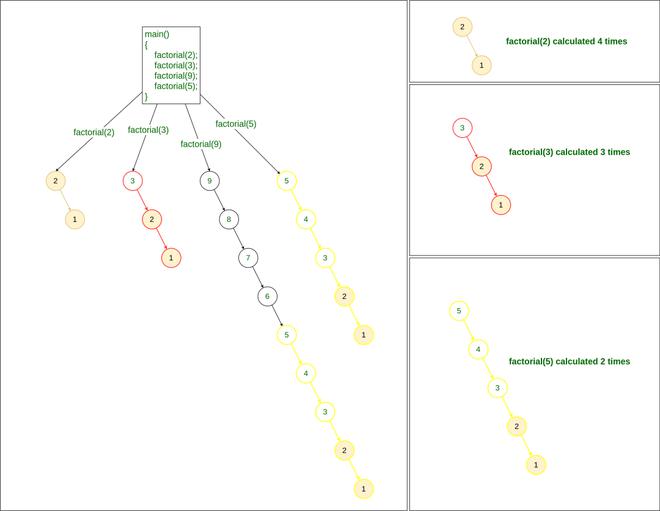
Recursive methodology to search out Factorial
So it’s secure to say that for locating factorial of numbers Okay numbers, the time complexity wanted might be O(N*Okay)
- O(N) to search out the factorial of a selected quantity, and
- O(Okay) to name the factorial() methodology Okay totally different occasions.
How Memorization can assist with such issues?
If we discover within the above downside, whereas calculation factorial of 9:
- We’re calculating the factorial of two
- We’re additionally calculating the factorial of three,
- and We’re calculating the factorial of 5 as effectively
Subsequently if we retailer the results of every particular person factorial on the first time of calculation, we will simply return the factorial of any required quantity in simply O(1) time. This course of is named Memoization.
Resolution utilizing Memoization (How does memoization work?):
If we discover the factorial of 9 first and retailer the outcomes of particular person sub-problems, we will simply print the factorial of every enter in O(1).
Subsequently the time complexity to search out factorial numbers utilizing memorization might be O(N)
- O(N) to search out the factorial of the most important enter
- O(1) to print the factorial of every enter.
Varieties of Memoization
The Implementation of memoization relies upon upon the altering parameters which might be accountable for fixing the issue. There are numerous dimensions of caching which might be utilized in memoization approach, Under are a few of them:
- 1D Memoization: The recursive perform that has just one argument whose worth was not fixed after each perform name.
- 2D Memoization: The recursive perform that has solely two arguments whose worth was not fixed after each perform name.
- 3D Memoization: The recursive perform that has solely three arguments whose values weren’t fixed after each perform name.
How Memoization approach is utilized in Dynamic Programming?
Dynamic programming helps to effectively resolve issues which have overlapping subproblems and optimum substructure properties. The concept behind dynamic programming is to interrupt the issue into smaller sub-problems and save the outcome for future use, thus eliminating the necessity to compute the outcome repeatedly.
There are two approaches to formulate a dynamic programming resolution:
- High-Down Method: This strategy follows the memoization approach. It consists of recursion and caching. In computation, recursion represents the method of calling features repeatedly, whereas cache refers back to the strategy of storing intermediate outcomes.
- Backside-Up Method: This strategy makes use of the tabulation approach to implement the dynamic programming resolution. It addresses the identical issues as earlier than, however with out recursion. On this strategy, iteration replaces recursion. Therefore, there isn’t a stack overflow error or overhead of recursive procedures.
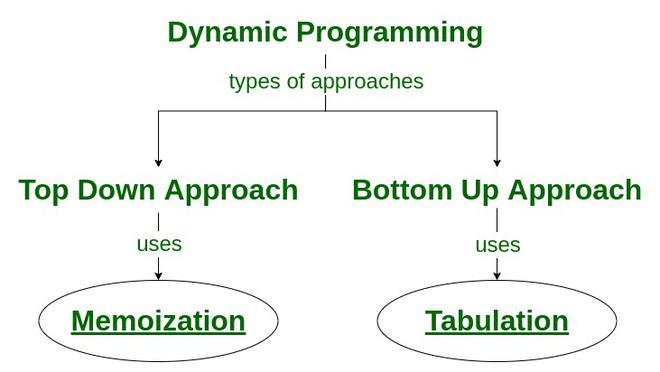
How Memoization approach is utilized in Dynamic Programming
How Memoization is totally different from Tabulation?
For extra particulars please refer: Tabulation vs. Memoization
Coding Follow Issues on Memoization
Query | Article | Follow | Video |
---|---|---|---|
Rely methods to achieve the n’th stair | View | Remedy | Watch |
Phrase Break Drawback | DP-32 | View | Remedy | Watch |
Program for Fibonacci numbers | View | Remedy | Watch |
nth Catalan Quantity | View | Remedy | Watch |
Gold Mine Drawback | View | Remedy | Watch |
Subset Sum Drawback | View | Remedy | Watch |
Reducing a Rod | View | Remedy | Watch |
Min Value Path | View | Remedy | Watch |
Minimal variety of jumps to achieve finish | View | Remedy | Watch |
Longest Palindromic Substring | Set 1 | View | Remedy | Watch |
Longest Repeating Subsequence | View | Remedy | Watch |
Rely methods to achieve the nth stair utilizing step 1, 2 or 3 | View | Remedy | Watch |
Rely of various methods to specific N because the sum of 1, 3 and 4 | View | Remedy | Watch |
Rely variety of methods to cowl a distance | View | Remedy | Watch |
Rely of arrays having consecutive component with totally different values | View | Remedy | Watch |
Largest Sum Contiguous Subarray | View | Remedy | Watch |
Smallest sum contiguous subarray | View | Remedy | Watch |
Distinctive paths in a Grid with Obstacles | View | Remedy | Watch |
Other ways to sum n utilizing numbers larger than or equal to m | View | Remedy | Watch |
Ceaselessly requested questions (FAQs) about Memoization
1: Is memoization higher than DP?
Memoization is the top-down strategy to fixing an issue with dynamic programming. It’s known as memoization as a result of we’ll create a memo for the values returned from fixing every downside.
2: Is memoization the identical as caching?
Memoization is definitely a particular kind of caching. The time period caching can usually discuss with any storing approach (like HTTP caching) for future use, however memoizing refers extra particularly to caching perform that returns the worth.
3: Why memoization is top-down?
The highest-Down strategy breaks the big downside into a number of subproblems. if the subproblem is solved already then reuse the reply. In any other case, Remedy the subproblem and retailer the lead to some reminiscence.
4: Does memoization use recursion?
Memoization follows top-down strategy to fixing the issue. It consists of recursion and caching. In computation, recursion represents the method of calling features repeatedly, whereas cache refers back to the strategy of storing intermediate outcomes.
5: Ought to I take advantage of tabulation or memoization?
For issues requiring all subproblems to be solved, tabulation sometimes outperforms memoization by a relentless issue. It is because the tabulation has no overhead of recursion which reduces the time for resolving the recursion name stack from the stack reminiscence.
Every time a subproblem must be solved for the unique downside to be solved, memoization is preferable since a subproblem is solved lazily, i.e. solely the computations which might be required are carried out.
6: The place is memoization used?
Memoization is an optimization approach used to hurry up laptop applications by caching the outcomes of pricy perform calls and returning them when the identical inputs are encountered once more.
7: Why is it known as memoization?
The time period “memoization” comes from the Latin phrase “memorandum” (“to recollect”), which is often shortened to “memo” in American English, and which implies “to rework the outcomes of a perform into one thing to recollect.”.
8: How does memoization cut back time complexity?
Fixing the identical downside repeatedly takes time and will increase the run-time complexity of the general program. This downside might be resolved by sustaining some cache or reminiscence the place we’ll retailer the already calculated results of the issue for some explicit enter. In order that if we don’t need to recalculate the identical downside, we will merely use the outcome that’s saved within the reminiscence and cut back the time complexity.
9: What’s the distinction between memoization and caching?
Memoization is definitely a particular kind of caching that entails caching the return worth of a perform based mostly on enter. Caching is a extra basic time period. For instance, HTTP caching is caching however it isn’t memoization.
10: Why tabulation is quicker than memoization?
Tabulation is often quicker than memoization, as a result of it’s iterative and fixing subproblems requires no overhead of recursive calls.
Conclusion
Memoization is a programming idea and might be utilized to any programming language. Its absolute objective is to optimize this system. Often, this downside is seen when applications carry out heavy computations. This system cache all of the earlier outcome that’s computed so that it’s going to not must recalculate for a similar downside.
Associated Articles: