What, How, and Why.
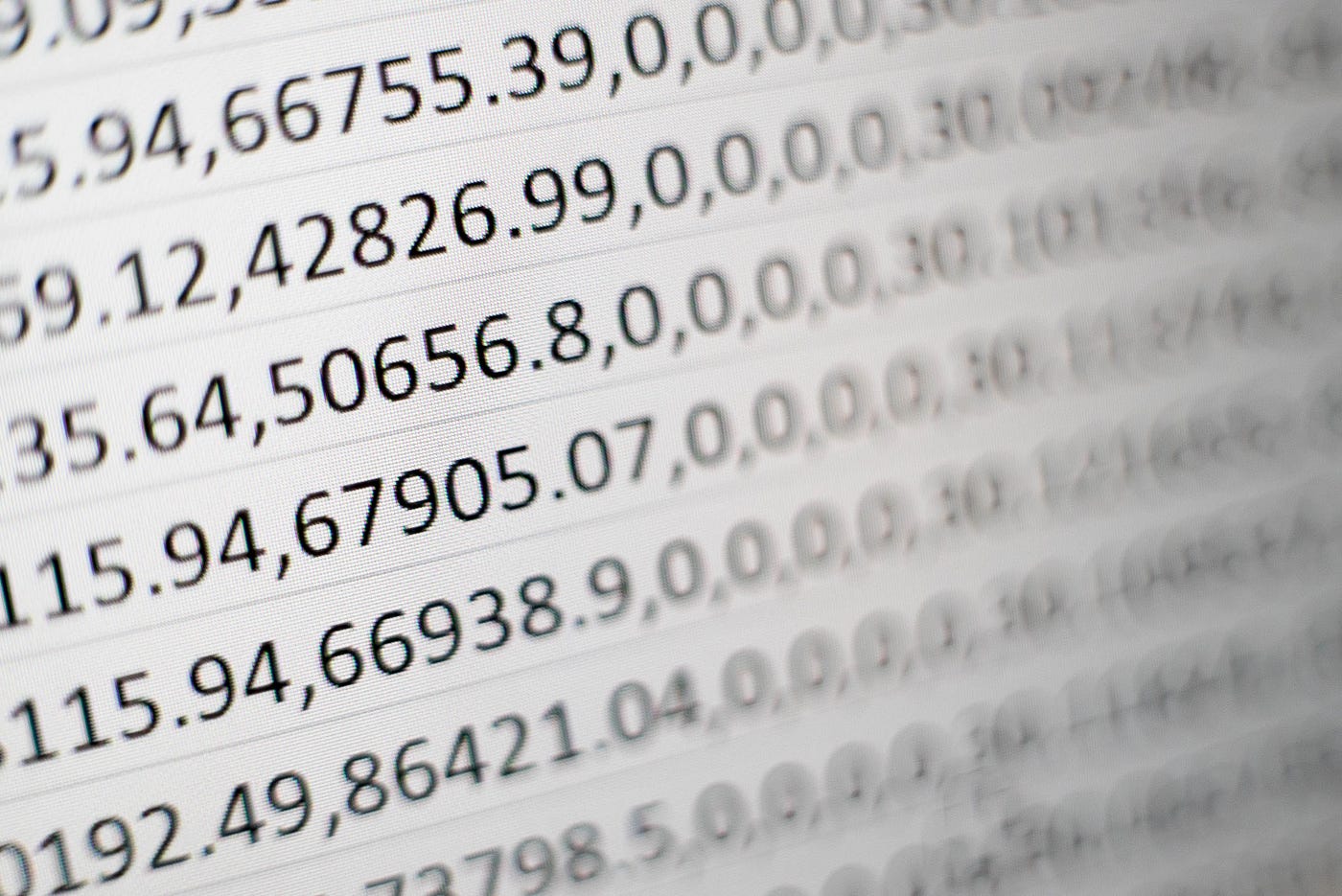
Index:
1.Introduction
2.Indexing an array
3.Slicing array
4.Operations on a array
5.Arithmetic capabilities in Numpy
6.Concatenation of array
Splitting of an array
NumPy is for numbers, Pandas is for knowledge
NumPy is a free and open supply Python library that can provide help to manipulate numbers and apply numerical capabilities. The place Pandas is nice to deal with dataframes, NumPy is nice to use maths.
An array is a grid of values (of the identical sort), listed by a constructive integer. In Numpy, the scale are known as axes (0 and 1 for a 2-dimensional array), and the axes are rank.
The rank of an array is just the variety of axes (or dimensions) it has. A easy listing has rank 1: a2 dimensional array (typically known as a matrix) has rank 2: a 3 dimensional array has rank 3.
Diving an excessive amount of into the ocean of arrays might be harmful and technical. So for this text we’ll restrict ourselves to 1 or two arrays (1 or 2 dimensions) as a result of they’re probably the most frequent in knowledge evaluation.
It’s higher apply to make use of Numpy arrays as an alternative of lists. They’re sooner, devour much less reminiscence, and extra handy.
Indexing an array is just like indexing an inventory. The primary worth is 0, and it is advisable to use sq. bracket to name your knowledge.
my_array = np.prepare(5)print(my_array)
[0,1,2,3,4]print(my_array[0])0
As launched above, an array can have a number of dimensions, the strategy to index the arrays stays the identical. We will retrieve a component utilizing two indices that choose the row and the column.
my_array = np.array([[0,1,2],[3,4,5]])
my_arrayarray([[0,1,2],
[3,4,5]])my_array[0][1]
1
You can even choose a row or a column:
my_array
array([[0,1,2]
,[3,4,5]])my_array[0]
[0 1 2]my_array[:,1]
[1 4]
The slice system is made like this [start:end], the place the beginning is inclusive and the top unique.
my_array = [1,2,3,4,5,6,7,8]
my_array[1,3]
[2, 3]my_array[1:]
[2,3,4,5,6,7,8]my_array[:]
[1,2,3,4,5,6,7,8]
It’s the identical for multidimensional array.
my_array = np.array([[1,2,3,4]
,[5,6,7,8]
,[9,10,11,12]])print(my_array[:,1:3])
[[2,3]
[6,7]
[10,11]]
It’s potential to do operations on an array. We will add them collectively. Please word that the operation received’t work if the scale is totally different:
array_1 = np.prepare(5)
array_2 = np.array([100,101,102,103,104])array_3 = array_1 + array_2
print(array_3)
array([100,102,104,106,108])
You can even multiply the arrays or apply any type of numerical operation, the logic stays the identical.
- Utilizing Numpy with Comparability Expressions
When evaluating array, NumPy applies a Boolean logic.
my_array = [0,1,2,3,4,5]
new_array = my_array > 1
array([False, False, True, True, True, True)my_array[new_array]
array([2,3,4,5])my_array[my_array > 1]
array([2,3,4,5])
On prime of the in-built Python capabilities, NumPy supplies arithmetic capabilities. They comply with the identical array manipulation guidelines seen beforehand:
np.add()
np.subtract()
np.adverse()
np.multiply()
np.divide()
np.floor_divide()
np.energy()
Arrays might be concatenated provided that they’ve the identical form. You’ll be able to add a couple of array without delay.
array_a = np.array([1,2,3])
array_b = np.array([4,5,6])
array_c = np.concatenate([array_a, array_b])
array([1,2,3,4,5,6])
2D arrays are notably attention-grabbing with matrix. By default, concatenation is about on axis=0
. Which can return extra rows however the identical variety of columns. If we would like the arrays to be subsequent to every, we have to set axis=1
.
If the arrays don’t match, like, not the identical numbers of rows and axes on 1, or not the identical numbers of rows however axes on 0, then it is not going to work and return an error message.
It’s potential to separate an array into a number of sub-arrays. Let’s see this as the other of concatenation. If you happen to merely affiliate a quantity to the break up()
operate, NumPy will attempt to be sensible and separate your array into sub-arrays of the identical measurement.
If it’s not potential to create sub-arrays of the identical measurement. One should use the array_split()
operate.
my_array = [1,2,3,4,5,6]
split_array = np.break up(my_array, 2)
print(split_array)
[array(1,2,3)),[array(4,5,6)]my_new_array = [1,2,3,4,5,6,7]
mna = np.split_array(my_new_array)
[array(1,2,3)),[array(4,5,6)], [array(7)]
You’ve received the likelihood to go for a custom-made consequence and break up the 1D array at positions indicated.
my_array = [1,2,3,4,5,6,7]
split_array = np.break up(my_array, [2,4])
print(split_array)
[array([1,2])],array([3,4]),array([5,6,7])]
You can even resolve to separate the arrays vertically or horizontally with the vsplit()
and hsplit()
capabilities
NumPy comes up with a helpful strategy to manipulate knowledge. Higher, sooner, and extra environment friendly than Lists, arrays are a extensively used answer. This introduction confirmed the way it works and a few helpful operations & queries potential.