One product ensuing from the work achieved by Interop 2022, the cross-browser initiative to seek out and tackle an important interoperability ache factors on the internet, is the HTML inert
attribute. Created to cater for interoperable conduct as a part of bettering the <dialog>
specification, the inert
property is a boolean worth that causes the browser to disregard all the occasions triggered on the weather it’s utilized to. We’re principally all in favour of its skill to disregard occasions from each customers and assistive applied sciences.
On this article, we’ll discover the inert
attribute, observe a number of the ache factors we confronted earlier than its introduction, and determine whether or not or not we are able to use it to alleviate or utterly overcome these points when programming for the net. We’ll cowl the next:
The total code for the examples lined on this article is obtainable on GitHub. Let’s get began!
How was consumer focus managed earlier than inert
?
It’s good follow to stay in-keeping with the W3C specification of how interactive parts and the remainder of the web page should behave when lively.
Earlier than the creation of <dialog>
and the inert
property, implementing concentrate on parts that required extra consideration, like modals, required builders to restrict focus within interactive areas, take heed to focus change occasions, and drive the main focus again in case it leaked outdoors the bounds of the goal ingredient. This all needed to be achieved when the interactive ingredient was nonetheless lively.
Focus implementation additionally included guaranteeing that interplay with the web page’s parts outdoors of the interactive ingredient was unattainable, trapping the consumer to solely the interactive house. The JavaScript code beneath reveals an instance of implementing the main focus loop inside interactive parts:
// trap-focus.js const FOCUSABLE_SELECTORS_LIST = 'a[href]:not([disabled]), enter:not([disabled]), choose:not([disabled]), textarea:not([disabled]), button:not([disabled]), space[href], [tabindex="0"], [contenteditable], object, iframe', TAB_KEY_CODE = 9 let firstFocusElement, lastFocusElement operate initiateFocusLooping(modal){ let focusableModelElements = modal.querySelectorAll(FOCUSABLE_SELECTORS_LIST) firstFocusElement = focusableModelElements[0] lastFocusElement = focusableModelElements[focusableModelElements.length - 1] firstFocusElement.focus({focusVisible: true}); modal.addEventListener("keydown", throttleFocus, false) } operate endFocusLooping(modal){ modal.removeEventListener("keydown", throttleFocus, false) } operate throttleFocus(el){ if(el.keyCode === TAB_KEY_CODE || el.key === "Tab"){ if(el.shiftKey && doc.activeElement === firstFocusElement) { el.preventDefault(); lastFocusElement.focus(); } else if(!el.shiftKey && doc.activeElement === lastFocusElement) { el.preventDefault(); firstFocusElement.focus() } } }
Within the code above, we first deal with keyboard navigation by attending to know all the focusable parts throughout the interactive element. We then monitor keyboard navigation makes an attempt, restrict them to the locality of the interactive element, and actively implement the anticipated sequential navigation that’s routinely applied inside HTML paperwork.
So we don’t repeat the code unnecessarily, we’ll reference the trap-focus.js
file all through the next examples.
In respect to the interactive house, a developer has to pay attention to all of the attainable actions that would happen inside a web page. For one, you must work on dealing with keyboard navigation by way of Tab and Shift + Tab, forcing the looping of focus throughout the focusable parts throughout the interactive house. Nevertheless, you additionally should take heed to the keyboard EscKeyDown
occasion, which causes the closure of the interactive element:
// Listening to Esc key press and returning focus to ingredient that triggered the interactive ingredient operate listenToEscKeyDown(el) { if(!modal.classList.accommodates("disguise") && (el.key === "Esc" || el.keyCode === 27)){ toggleModal() ELEMENT_THAT_OPENED_INTERACTIVE_ELEMENT.focus() } }
For the remainder of the instance code, we’ll reference the listenToEscKeyDown()
operate above in order to not repeat it.
When contemplating visible interplay for customers with a mouse and touchscreen, we have to place a hard and fast positioned overlay block between the interactive element and the remainder of the web page’s parts, as is the case with modals. Alternately, you would place it on high of the web page parts that shouldn’t be accessed, as is the case with full-screen and side-slide navigation menus, stopping customers from by chance interacting with them.
Within the code beneath, we finalize the modal instance that makes use of capabilities from the trap-focus.js
instance above. We additionally add an overlay container:
<model> /* modal, modal-overlay, and conceal kinds */ </model> <physique> <most important> <h1>Legacy Modal</h1> <div> <p> Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Phasellus vestibulum lorem sed risus. </p> <button class="open-modal">Register</button> </div> </most important> <div id="registration-modal" class="modal-overlay disguise" function="dialog"> <div class="modal"> <h2 aria-label="Registration Modal">Registration</h2> <p>Whats up there, you possibly can't focus outdoors my boundaries! :-)<p> <kind motion=""> <div> <enter sort="textual content" title="title" placeholder="Thunder Storm"> </div> <div> <enter sort="electronic mail" title="electronic mail" placeholder="[email protected]"> </div> <div> <enter sort="password" title="password"> </div> <div> <legend> Pet of alternative: </legend> <label> <enter sort="radio" worth="cat" title="pet"> Cat </label> <label> <enter sort="radio" worth="canine" title="pet"> Canine </label> </div> <div> <label for="phrases"> <enter sort="checkbox"> Conform to <a href="#phrases">phrases</a> </label> </div> <div> <button>Save</button> <button sort="button" class="close-modal">Discard</button> </div> </kind> </div> </div> <script src="https://weblog.logrocket.com/trap-focus.js"></script> <script> let modal = doc.getElementById("registration-modal") let closeModalButton = doc.querySelector(".close-modal") let openModalButton = doc.querySelector(".open-modal") operate toggleModal() { if(modal.classList.accommodates("disguise")){ modal.classList.take away("disguise") initiateFocusLooping(modal) doc.addEventListener("keydown", listenToEscKeyDown, false) } else { modal.classList.add("disguise") endFocusLooping(modal) doc.removeEventListener("keydown", listenToEscKeyDown, false) } } modal.addEventListener("keydown", throttleFocus, false) openModalButton.addEventListener("click on", toggleModal, false) closeModalButton.addEventListener("click on", toggleModal, false) </script> </physique>
The toggleModal()
operate toggles the visibility of the modal by utilizing lessons and implements respective listeners to our modal element.
With the implementation above, we find yourself with the next modal element:
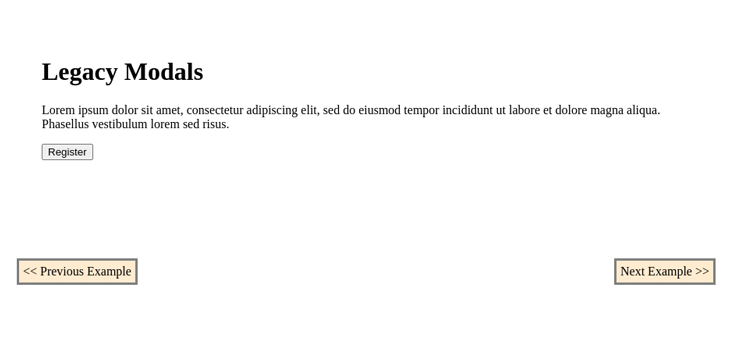
This focus technique grew to become often called focus trapping. As seen within the implementation above, we’ve carried out all of the required steps to put focus in an interactive house for all attainable varieties of navigation till the meant job was completed throughout the house.
Issues with the main focus trapping methodology
The main target trapping methodology confronted quite a few challenges; one main challenge was coping with net accessibility, particularly on cellular units.
The assistive applied sciences on these units, like TalkBack and VoiceOver, present help via double-tap and swipe occasions. On the time of writing, we don’t have an ordinary approach to implement the logic for these occasions as we’d in any other case with key-presses and clicks.
If we check the modal we applied within the earlier instance with TalkBack on cellular, we find yourself with the next:
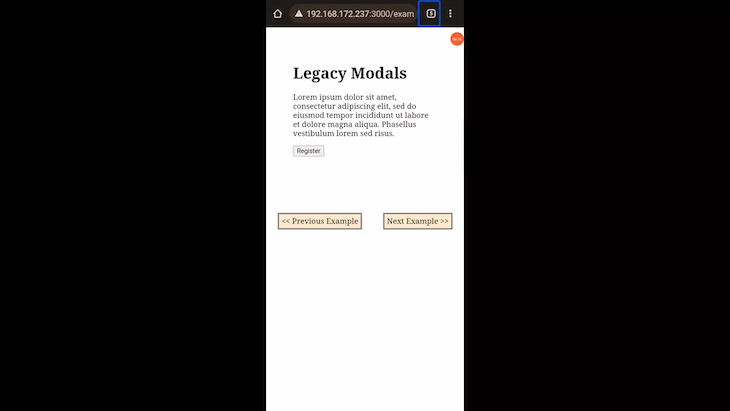
As we are able to observe within the demonstration above, the navigation focus goes past our lure to the underlying parts, which, in follow, will not be presupposed to be accessed whereas the modal is lively.
To make this work, we may disguise all the weather however the interactive ingredient, the modal, from the assistive applied sciences.
We will accomplish that by including the aria-hidden
property and setting it to true
on all parts however the interactive element in focus. Doing so would inform display screen readers that other than our element, the opposite parts throughout the present doc must be off limits:
<!-- ... web page HTML ... --> <script src="https://weblog.logrocket.com/trap-focus.js"></script> <script> let modal = doc.getElementById("registration-modal") let closeModalButton = doc.querySelector(".close-modal") let openModalButton = doc.querySelector(".open-modal") operate toggleAriaHidden(allElements, exception, apply = true){ for(const el of allElements){ if(el.tagName !== "script" && el !== exception) if(apply){ el.setAttribute("aria-hidden", "true") } else { el.removeAttribute("aria-hidden") } } } operate toggleModal() { if(modal.classList.accommodates("disguise")){ modal.classList.take away("disguise") initiateFocusLooping(modal) doc.addEventListener("keydown", listenToEscKeyDown, false) } else { modal.classList.add("disguise") endFocusLooping(modal) doc.removeEventListener("keydown", listenToEscKeyDown, false) } toggleAriaHidden(doc.querySelector("physique").youngsters, modal, false) } modal.addEventListener("keydown", throttleFocus, false) openModalButton.addEventListener("click on", toggleModal, false) closeModalButton.addEventListener("click on", toggleModal, false) </script>
The toggleAriaHidden()
operate within the code above facilitates including aria-hidden
to all the weather that should be inaccessible:
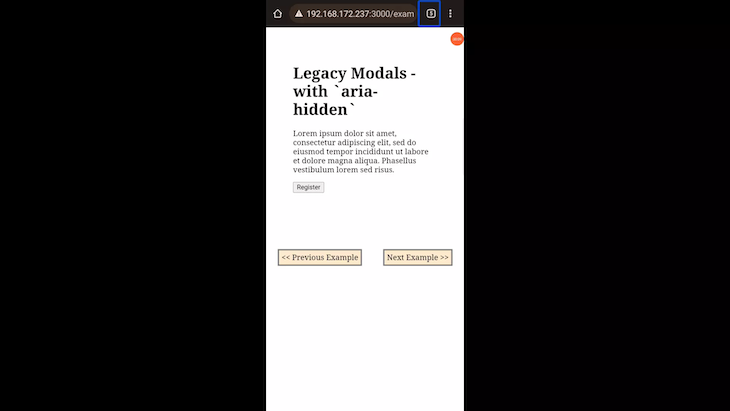
Within the demonstration above, we are able to see that after having utilized aria-hidden
, the main focus navigation doesn’t leak into the underlying parts. Nevertheless, the difficulty with this repair is that within the presence of numerous parts inside a web page, it turns into not solely inefficient, however impractical.
To beat that, we may leverage the aria-hidden
attribute, the place all youngsters parts below the container ingredient the place it’s utilized are rendered inaccessible. Nevertheless, this might require laying out the HTML paperwork in order that parts larger up within the web page’s hierarchy could be made inaccessible with aria-hidden
whereas nonetheless sustaining the traditional conduct inside our pages.
In doing so, our interactive parts can’t be youngsters of the weather that may be made inaccessible utilizing aria-hidden
. Subsequently, we are able to scale back the variety of manipulations which might be utilized to the DOM.
One other challenge we face with focus trapping is within the case of modals. We have to add fastened positioned wrapping containers and overlays between the interactive parts and the remainder of the web page. In any other case, we are able to place these wrapping containers on high of the quickly inaccessible elements of the web page when the interactive parts are in focus.
We want the wrappers to behave as buffers or traps for all mouse occasions, which might in any other case find yourself in areas that must be inaccessible. You’ll be able to see this implementation in follow by observing the .modal-overlay
container within the earlier instance.
How inert
acts as a spotlight guard
The inert
attribute shifts the implementation of concentrate on interactive areas from a lure to extra of a guard like exercise. By making the quickly unneeded areas of a web page inaccessible as an alternative of trapping a consumer to particular areas, it leaves all focus associated actions to the interactive areas themselves.
Its implementation resembles what we see when utilizing the JavaScript alert()
operate. When inert
is added to a component, that a part of the markup turns into frozen or guarded. Attainable interactions like clicks and focus change makes an attempt with each mice and keyboards grow to be unavailable.
Extra nice articles from LogRocket:
A developer’s work is made easy by implementing logic that’s centered on the interactive house reasonably than being aware of the remainder of the web page’s markup and its conduct.
inert
can be synonymous with accessibility, that means, not solely do the weather it’s utilized to grow to be inaccessible to visible interactions, but additionally to assistive applied sciences present in cellular units.
In our instance above, utilizing inert
 would extremely scale back the quantity of code used to implement the modal.
When utilizing the inert
attribute, it’s good follow to implement a visible indication of the inert areas of the display screen as an alternative of the lively ones. We have to implement this as a result of the attribute itself doesn’t deal with it, as we’d anticipate with parts like <dialog>
and its ::backdrop
pseudo-class. It’s vital to make the inert areas apparent in order that net app customers are stored within the know of what’s occurring on a webpage. In any other case, they may be left annoyed.
We will model parts containing the inert
attribute as follows:
[inert]{ filter: grayscale(1); opacity: .2; }
With inert
, the earlier instance would seem like the next:
<model> /* Take away .modal-overlay from kinds, leaving .modal and .disguise lessons */ </model> <physique> <most important> <h1>Changing Legacy Focus Trapping with `inert`</h1> <div> <p> Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Phasellus vestibulum lorem sed risus. </p> <button class="open-modal">Register</button> </div> </most important> <!-- Eliminated modal overlay container --> <div class="modal disguise"> <h2>Registration</h2> <p>Whats up there, you possibly can't focus outdoors my boundaries! :-)<p> <kind motion=""> <div> <enter sort="textual content" title="title" placeholder="Thunder Storm"> </div> <div> <enter sort="electronic mail" title="electronic mail" placeholder="[email protected]"> </div> <div> <enter sort="password" title="password"> </div> <div> <legend> Pet of alternative: </legend> <label> <enter sort="radio" worth="cat" title="pet"> Cat </label> <label> <enter sort="radio" worth="canine" title="pet"> Canine </label> </div> <div> <label for="phrases"> <enter sort="checkbox"> Conform to <a href="#phrases">phrases</a> </label> </div> <div> <button>Save</button> <button sort="button" class="close-modal">Discard</button> </div> </kind> </div> <script src="https://weblog.logrocket.com/trap-focus.js"></script> <script> let modal = doc.querySelector("modal") let closeModalButton = doc.querySelector(".close-modal") let openModalButton = doc.querySelector(".open-modal") let otherPageElements = doc.querySelector("most important") // Take away toggleAriaHidden() operate operate toggleModal() { if(modal.classList.accommodates("disguise")){ modal.classList.take away("disguise") initiateFocusLooping(modal) doc.addEventListener("keydown", listenToEscKeyDown, false) otherPageElements.toggleAttribute("inert") } else { modal.classList.add("disguise") endFocusLooping(modal) doc.removeEventListener("keydown", listenToEscKeyDown, false) otherPageElements.toggleAttribute("inert") } } modal.addEventListener("keydown", throttleFocus, false) openModalButton.addEventListener("click on", toggleModal, false) closeModalButton.addEventListener("click on", toggleModal, false) </script> </physique>
Potential use instances for inert
inert
will be helpful when implementing quite a few interactive house based mostly parts for web sites and net apps. Let’s see a couple of examples of those parts.
Customized affirm bins
We may use inert
to implement customized affirm bins that adapt the schema of apps we construct, getting rid of the default browser particular customized bins.
Any such implementation gives us not solely the facility to model our affirm bins or modals, but additionally the power to put any attainable variety of choices, other than the default two we get with JavaScript’s affirm()
.
Under is an instance of a affirm field utilizing inert
:
<model> /* Customized kinds */ </model> <physique> <most important> <h1 tabindex="0">Easy affirm field with `inert`</h1> <div> <textarea title="" id="" cols="30" rows="10">Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Phasellus vestibulum lorem sed risus.</textarea> </div> <div> <button class="delete-prompt">Delete Remark</button> </div> </most important> <div class="affirm disguise" function="dialog"> <p>Are you positive about deleting this textual content?<p> <button class="keep-text">Proceed writing</button> <button class="delete-text">Sure</button> </div> </physique> <script src="https://weblog.logrocket.com/js/trap-focus.js"></script> <script> let confirmBox = doc.querySelector(".affirm"), agreeBtn = doc.querySelector(".delete-text"), disagreeBtn = doc.querySelector(".keep-text"), deletionConfirmationBtn = doc.querySelector(".delete-prompt"), mainElement = doc.querySelector("most important"), textArea = doc.querySelector("textarea") deletionConfirmationBtn.addEventListener("click on", toggleModal, false) disagreeBtn.addEventListener("click on", toggleModal, false) agreeBtn.addEventListener("click on", operate(){ toggleModal(); textArea.innerText = "" }, false) operate toggleModal() { mainElement.toggleAttribute("inert") if(confirmBox.classList.accommodates("disguise")){ confirmBox.classList.take away("disguise") confirmBox.setAttribute("aria-modal", "true") initiateFocusLooping(confirmBox) doc.addEventListener("keydown", listenToEscKeyDown, false) } else { confirmBox.classList.add("disguise") confirmBox.removeAttribute("aria-modal") endFocusLooping(confirmBox) doc.removeEventListener("keydown", listenToEscKeyDown, false) } } </script>
Under is an indication of our affirm field in motion:
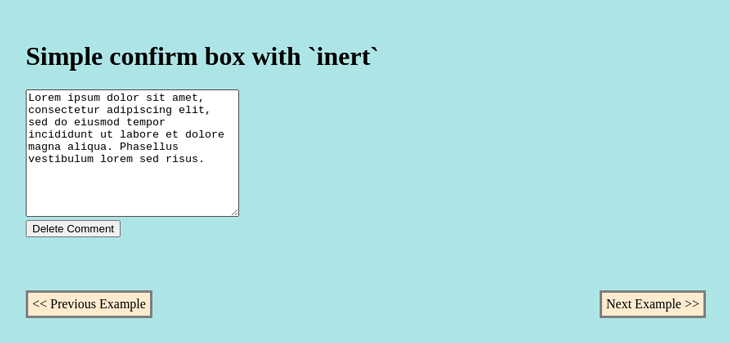
Authentication kinds
With modal parts like prompts, vital notifications, and compulsory authentication kinds, it’s applicable to make use of inert
. With inert
, we are able to simply information a consumer to the interactive ingredient with out worrying about accessibility; inert
can be acknowledged and supported by assistive applied sciences.
One of many standard tendencies in main social media net apps are conditional authentication kinds. With these kinds, a restrict is ready on the quantity of feed that unauthenticated customers can view. After a specific amount of content material has been scrolled via, the unauthenticated guests are prompted to enroll and register whereas being frozen from navigating on the lively web page.
Let’s see how we are able to implement such kinds whereas utilizing inert
:
<model> /* [inert], most important, kind, .feed, .feed-item, most important, .feed-item, .target-item, .authentication-form, .disguise */ </model> <physique> <most important> <div class="feed"> <div class="feed-item"> <p> Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Phasellus vestibulum lorem sed risus. </p> </div> </div> </most important> <div class="authentication-form disguise" function="dialog"> <h2 aria-label="Authentication Type">Tremendous Login</h2> <p>Whats up there, to maintain having fun with our feed, please register! :-)<p> <kind motion=""> <enter sort="textual content" title="electronic mail" placeholder="electronic mail"> <enter sort="password" title="password" placeholder="password"> <div> <button class="close-modal">Signal In</button> </div> </kind> </div> </physique> <script src="https://weblog.logrocket.com/js/trap-focus.js"> </script> <script> let loginModal = doc.querySelector(".authentication-form"), feed = doc.querySelector(".feed"), feedSample = doc.querySelector(".feed-item"), targetElement, feedGuarded = false; // populate feed for(let i = 1; i < 20; i++){ let newFeedNode = feedSample.cloneNode(true) newFeedNode.classList.add(i) if(i === 10) { // make the tenth put up our goal merchandise newFeedNode.classList.add("target-item") targetElement = newFeedNode } feed.appendChild(newFeedNode) } operate monitorFeed(){ if(targetElement.getBoundingClientRect().high <= 100){ guardFeed() } } doc.addEventListener("scroll", monitorFeed, false) operate guardFeed() { if(!feedGuarded){ feed.toggleAttribute("inert") loginModal.classList.take away("disguise") initiateFocusLooping(loginModal) feedGuarded = true // restrict scrolling window.onscroll = operate() doc.documentElement.scrollTop, window.scrollX // cease scroll occasion listening doc.removeEventListener("scroll", monitorFeed, false) } } </script>
Under is the demonstration of the authentication kind in motion.
With slide-out aspect navigation menus, we anticipate customers’ consideration to be on the navigation ingredient, avoiding mouse clicks and keyboard navigation on the web page behind it. In such a situation, we are able to use inert
to information customers.
Let’s see a working instance:
<physique> <enter sort="checkbox" class="nav-hum" id="nav-hum"> <label for="nav-hum" tabindex="0"></label> <nav class="navigation" aria-label="Essential"> <h3>Slide-out Nav</h3> <ul> <li> <a category="side-nav" href="https://weblog.logrocket.com/using-html-inert-property-manage-user-focus/example-1.html">Instance 1</a> </li> <!-- Extra hyperlinks --> <li> <a category="side-nav" href="example-5.html">Instance 7</a> </li> </ul> </nav> <most important class="page-content"> <h1>Slide-out aspect navigation menu</h1> <p tabindex="0"> Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Phasellus vestibulum lorem sed risus. </p> <!-- extra objects --> </most important> </physique> <script> let navBtn = doc.querySelector("#nav-hum") let pageContents = doc.querySelector("most important") navBtn.addEventListener("change", (el) => { pageContents.toggleAttribute("inert") }) </script>
Many of the logic that entails displaying and hiding the slide-out menu on this instance is carried out contained in the CSS stylesheets:
.page-content{ place: absolute; left: 0; high: 0; proper: 0; z-index: 1; margin: 0; show: flex; peak: 100%; flex-direction: column; background-color: white; } .page-content > *{ width: 100%; } nav.navigation{ place: fastened; show: flex; flex-direction: column; justify-content: begin; left: 0; proper: 0; high: 0; backside: 0; z-index: 0; margin: auto; padding: 1rem; padding-top: 1.5rem; background-color: bisque; } .navigation > ul{ list-style: none; } .navigation > ul > li{ margin-top: 1rem; } .nav-hum { place: absolute; clip: rect(0, 0, 0, 0); } label[for="nav-hum"] { place: fastened; high: 1rem; left: 1rem; z-index: 2; width: 30px; peak: 30px; cursor: pointer; background-image: url("information:picture/svg+xml;utf8,<svg xmlns="http://www.w3.org/2000/svg" viewBox='0 0 24 24' width="24" peak="24"><path fill="none" d='M0 0H24V24H0z'/><path d='M21 18v2H3v-2h18zM17.404 3.904L22 8.5l-4.596 4.596-1.414-1.414L19.172 8.5 15.99 5.318l1.414-1.414zM12 11v2H3v-2h9zm0-7v2H3V4h9z'/></svg>"); background-size: comprise; } .nav-hum:checked + label { left: 215px; background-image: url("information:picture/svg+xml;utf8,<svg xmlns="http://www.w3.org/2000/svg" viewBox='0 0 24 24' width="24" peak="24"><path fill="none" d='M0 0H24V24H0z'/><path d='M21 18v2H3v-2h18zM6.596 3.904L8.01 5.318 4.828 8.5l3.182 3.182-1.414 1.414L2 8.5l4.596-4.596zM21 11v2h-9v-2h9zm0-7v2h-9V4h9z'/></svg>"); } .nav-hum:checked ~ .page-content { box-shadow: inset -1px 0 3px 0px rgba(0,0,0,0.5); remodel: translate(200px); overflow: hidden; }
Inserting the 2 collectively, we get the next output:
Browser compatibility
On the time of writing, the HTML inert
characteristic has compatibility assist for all the most recent variations of main browsers, together with cellular and desktop, aside from Firefox. Be happy to take a look at the compatibility chart to study extra.
Utilizing inert
in incompatible browsers
It’s good follow to think about the situations the place new options won’t be supported and work on making their assist as broad as attainable, particularly when working on the internet.
To ensure that our net apps don’t break or malfunction whereas utilizing inert
, as may be the case with incompatible browsers, we should always use it’s polyfill.
Begin by putting in the npm module:
npm set up --save wicg-inert
Import the polyfill into your challenge:
import "wicg-inert"
Add the script to the HTML web page:
<html> ... <script src="https://weblog.logrocket.com/node_modules/wicg-inert/dist/inert.min.js"></script> </physique> </html>
For legacy browsers, you’ll have to incorporate extra polyfills for Map
, Set
, Component.prototype.matches
, and Node.prototype.accommodates
. To keep away from serving up pointless polyfills and stay in step with the W3C polyfill guideline, the inert
polyfill doesn’t bundle all these different polyfills.
Abstract
On this article, we’ve discovered concerning the inert
HTML attribute, exploring how we are able to use it when implementing interactive house parts, like modals, whereas managing consumer focus actions in a extra guided method than trapping.
We’ve seen sensible examples of how one can use inert
and discovered about its assist within the present listing of mainstream browsers. We’ve additionally reviewed some greatest practices whereas utilizing inert
, like including visible indication of inert areas of the HTML web page and including its assist for incompatible browsers.
For extra info relating to the inert
attribute, it’s inception, tips, and progress, you possibly can verify the next references: