Cell software builders typically have to show webpages inside their purposes. The only strategy to present a webpage is to open the actual webpage within the person’s default net browser, however this has some apparent drawbacks.
For instance, when the person opens a URL in a browser through your software, the actual motion immediately switches the present software context and launches the browser software, so it’s not user-friendly — and the browser UI just isn’t customizable, both. Platform-specific webview elements additionally let builders render net content material, however webviews sometimes don’t share looking states with the opposite net browsers and don’t embrace the most recent net APIs.
The Chromium open-source browser solved this URL navigation downside by providing the Customized Tabs function in 2015. Now, many main Android browsers implement the Customized Tabs protocol.
What’s the Customized Tabs function?
The Customized Tabs function lets cellular app builders launch a webpage in a customizable browser occasion that shares the identical cookie jar and permissions mannequin with the unique browser software. The flutter_custom_tabs
bundle presents a cross-platform answer for implementing Chrome Customized Tabs on Android and a Safari View Controller-based, Customized Tabs-like function on iOS.
On this submit, we’ll talk about each function that flutter_custom_tabs
presents on the Android platform through the next sections:
flutter_custom_tabs
options
The flutter_custom_tabs
bundle lets builders launch a URL on Chrome Customized Tabs on Android and Safari View Controller on iOS. The bundle contains many spectacular options, which we’ll assessment on this part.
Versatile, full-featured, and minimal API
This bundle presents a easy API operate just like the url_launcher bundle to make use of Customized Tabs with a really versatile configuration object that covers nearly all native Android Customized Tabs options. You possibly can simply configure the browser toolbar, exercise launcher animations, and a few browser options through the library configuration object.
Cross-platform assist
Chrome Customized Tabs is a function launched for the Android platform, however this bundle presents the same function on the iOS platform through the native Safari View Controller API. flutter_custom_tabs
even works with the Flutter net platform by opening a brand new browser tab as a web-based Customized Tabs different through the url_launch_package.
Error dealing with
Chrome Customized Tabs requires the Chrome browser, one other Customized Tabs-supported browser, or not less than a browser app to launch a webpage. When there is no such thing as a browser current within the system, this library can not accomplish its job. You possibly can deal with such errors simply with Dart try-catch blocks.
Flutter Chrome Customized Tabs tutorial
Now we all know the flutter_custom_tabs
bundle’s highlighted options. Let’s set up it right into a Flutter challenge and take a look at all supported options.
Organising the flutter_custom_tabs
bundle
You possibly can both take a look at the upcoming code examples with a brand new Flutter challenge or use the code examples straight in an current challenge.
When you plan to create a brand new challenge, create one with the next command:
flutter create customtabs cd customtabs
Now we are able to add flutter_custom_tabs
to the dependencies checklist of the pubspec.yaml
file and hyperlink the exterior bundle by operating the next command:
flutter pub get flutter_custom_tabs
Ensure that your dependencies checklist contains the newly linked bundle:
dependencies: flutter: sdk: flutter flutter_custom_tabs: ^1.0.4 # --- Different dependencies ---
Subsequent, run the challenge with the flutter run
command to put in the newly linked bundle and run the app.
You will note the default Flutter app when you run flutter run
if the plugin set up was profitable. You possibly can go away the appliance operating and take a look at the upcoming code snippets, due to Flutter’s scorching reloading function.
Launching a URL with the fundamental syntax
Let’s launch a URL with Chrome Customized Tabs with no further configuration to get began with this bundle. Add the next code to the lib/predominant.dart
file.
import 'bundle:flutter/materials.dart'; import 'bundle:flutter_custom_tabs/flutter_custom_tabs.dart'; void predominant() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({Key? key}) : tremendous(key: key); @override Widget construct(BuildContext context) { return MaterialApp( title: 'Customized Tabs Demo', theme: ThemeData( primarySwatch: Colours.blue ), house: House() ); } } class House extends StatelessWidget { const House({Key? key}) : tremendous(key: key); @override Widget construct(BuildContext context) { return Scaffold( appBar: AppBar( title: const Textual content('Customized Tabs Demo'), ), physique: Heart( little one: TextButton( little one: const Textual content('Open GitHub', fashion: TextStyle(fontSize: 20)), onPressed: () => _launchURL(), ), ), ); } void _launchURL() async { attempt { launch('https://github.com'); } catch(e) { debugPrint(e.toString()); } } }
Right here, we used the launch
async operate from the flutter_custom_tabs
bundle to open a Chrome occasion through the Customized Tabs function. We used a typical Dart try-catch error-handling strategy to detect Customized Tabs initialization points.
When you run the above code and click on on the Materials textual content button (ours reads Open GitHub), the app will open the GitHub web site in Customized Tabs, as proven beneath.
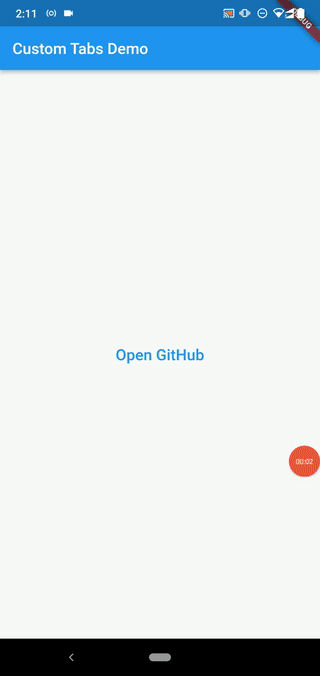
As proven within the above preview, I received the GitHub dashboard with out signing in, since I already signed in to the GitHub web site through Chrome — you will note all Customized-Tabs-launched web sites the identical as you see them in your Chrome browser due to the shared browser storage mannequin (i.e., cookies, settings, and many others.). You possibly can return to your app rapidly with a single faucet on the left aspect shut button, or you may press the again button a number of occasions, relying in your looking depth.
Customizing the toolbar through Android Intent
Regardless that Customized Tabs use the identical put in Chrome browser, it lets builders customise the first UI parts, such because the toolbar. The Customized Tabs API passes these configuration particulars to Chrome through the well-known Android Intent object. The launch
operate accepts an elective configuration object for Customized Tabs customization.
For instance, we are able to change the toolbar background shade with the toolbarColor
property. Replace your _launchURL
operate supply with the next code snippet:
void _launchURL() async { attempt { launch('https://github.com', customTabsOption: CustomTabsOption( toolbarColor: Colours.blue ) ); } catch(e) { debugPrint(e.toString()); } }
Now you’ll get a blue-color toolbar for the Chrome occasion, as proven within the following preview:
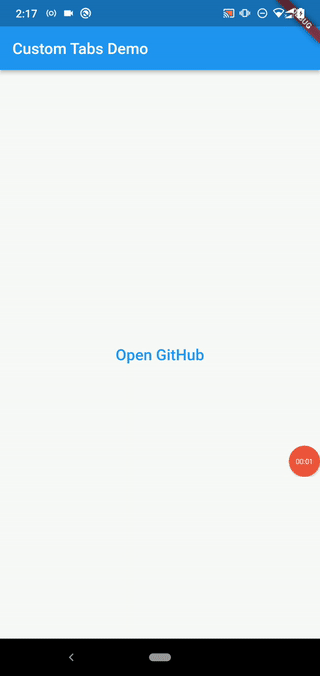
Right here, we hard-coded the Colours.blue
worth for the toolbar shade, however you may all the time apply your app theme’s major shade from the context
reference with the Theme.of()
operate name, as proven beneath:
void _launchURL(BuildContext context) async { attempt { launch('https://github.com', customTabsOption: CustomTabsOption( toolbarColor: Theme.of(context).primaryColor, ) ); } catch(e) { debugPrint(e.toString()); } }
Then, you additionally have to move the context
reference from the button press callback:
onPressed: () => _launchURL(context)
Right here is the whole supply code for utilizing your theme’s major shade for Customized Tabs:
import 'bundle:flutter/materials.dart'; import 'bundle:flutter_custom_tabs/flutter_custom_tabs.dart'; void predominant() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({Key? key}) : tremendous(key: key); @override Widget construct(BuildContext context) { return MaterialApp( title: 'Customized Tabs Demo', theme: ThemeData( primarySwatch: Colours.teal ), house: House() ); } } class House extends StatelessWidget { const House({Key? key}) : tremendous(key: key); @override Widget construct(BuildContext context) { return Scaffold( appBar: AppBar( title: const Textual content('Customized Tabs Demo'), ), physique: Heart( little one: TextButton( little one: const Textual content('Open GitHub', fashion: TextStyle(fontSize: 20)), onPressed: () => _launchURL(context), ), ), ); } void _launchURL(BuildContext context) async { attempt { launch('https://github.com', customTabsOption: CustomTabsOption( toolbarColor: Theme.of(context).primaryColor, ) ); } catch(e) { debugPrint(e.toString()); } } }
When you run the above code, you will note the teal major theme shade we selected within the Customized Tab toolbar, as proven within the following preview:
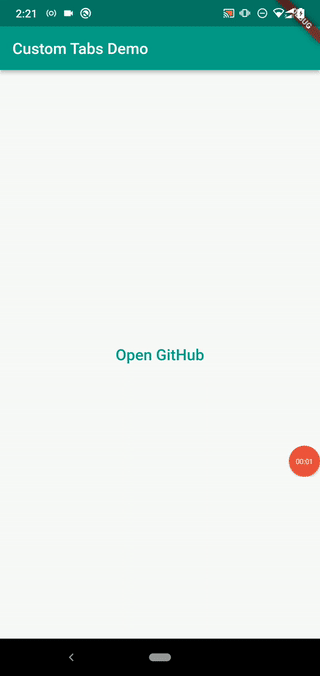
The showPageTitle
property helps you to management the visibility of the webpage title on the toolbar. The next configuration object asks Customized Tabs to indicate the webpage title:
customTabsOption: CustomTabsOption( // --- Different choices --- // ... showPageTitle: true, )
Now we are able to see the webpage title as follows:
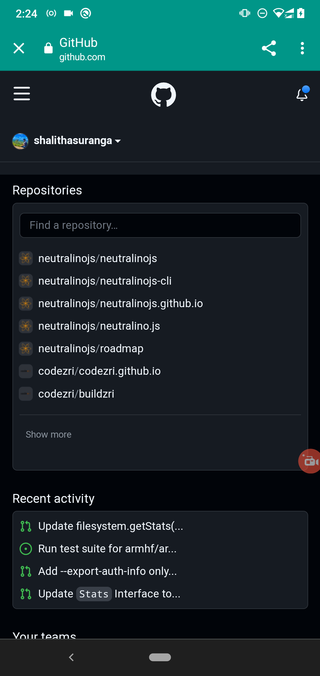
This bundle presents two extra configuration properties for toolbar-related customizations. The enableDefaultShare
and enableUrlBarHiding
properties assist present or conceal the sharing menu merchandise and present/conceal the toolbar whereas scrolling, respectively.
Customizing Android exercise animations
The Android platform sometimes performs a system animation throughout each exercise launch. Chrome Customized Tabs additionally inherits the identical system animation by default. The flutter_custom_tabs bundle presents a really versatile strategy to customise the exercise animation of the Chrome Customized Tab through the CustomTabsSystemAnimation
and CustomTabsAnimation
lessons.
This bundle presents two inbuilt exercise animations: slideIn
and fade
. You possibly can activate the slideIn
pre-built animation with the next code snippet:
customTabsOption: CustomTabsOption( // --- Different choices --- // .... animation: CustomTabsSystemAnimation.slideIn(), )
Now you will note the slideIn
animation as proven within the following preview:
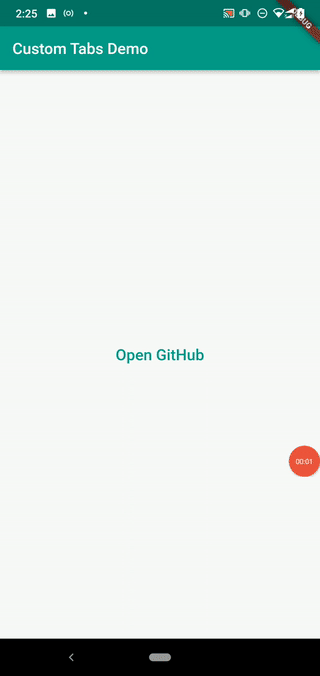
We are able to use the fade
animation by including the animation: CustomTabsSystemAnimation.fade()
configuration.
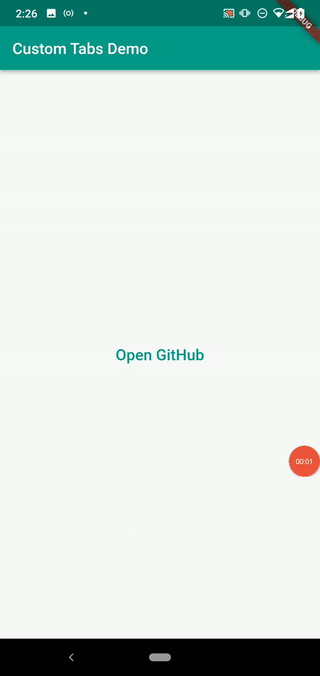
This bundle is so versatile — it helps you to use any Android framework core animation as a substitute of the pre-built library animations. For instance, you may construct a customized animation through the use of the next configuration object:
import 'bundle:flutter_custom_tabs_platform_interface/flutter_custom_tabs_platform_interface.dart'; // Import CustomTabsAnimation customTabsOption: CustomTabsOption( // --- Different choices --- // ... animation: const CustomTabsAnimation( startEnter: 'android:anim/screen_rotate_minus_90_enter', startExit: 'android:anim/fade_out', endEnter: 'android:anim/screen_rotate_minus_90_enter', endExit: 'slide_down/fade_out', ), )
Now you will note a unique rotating transition animation than the earlier pre-built library animations:
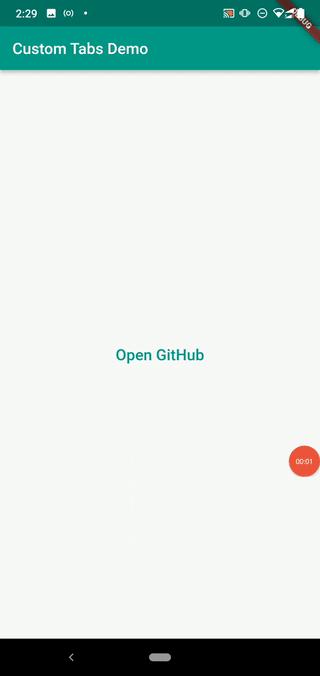
You possibly can present Android framework core animation identifiers for the CustomTabsAnimation
constructor, and browse all Android framework core animations on supply.
Every animation constructor parameter has the next definition:
startEnter
: Enter animation for Customized TabsstartExit
: Exit animation for the appliance exercise that launched Customized TabsendEnter
: Enter animation for the appliance that launched Customized TabsendExit
: Exit animation for Customized Tabs
Attempt to make your personal transition animations from Android framework core animations! Simply ensure to implement your animations with out negatively or drastically affecting the person expertise.
Superior Customized Tabs configurations
The above are frequent options that each Flutter developer must configure Chrome Customized Tabs. The flutter_custom_tabs
bundle additionally helps some superior configurations for particular necessities. For instance, you may prioritize fallback browsers if Chrome just isn’t current on the person’s system with the extraCustomTabs
array.
The next configuration prioritizes Mozilla Firefox after which Microsoft Edge if Chrome just isn’t current on the person’s system by referring Android bundle names:
customTabsOption: CustomTabsOption( extraCustomTabs: const <String>[ 'org.mozilla.firefox', 'com.microsoft.emmx' ], )
Additionally, this bundle helps you to allow and disable Google Play Prompt Apps in Customized Tabs through the enableInstantApps
boolean configuration property. For instance, the next configuration prompts the Prompt Apps function on Customized Tabs:
>customTabsOption: CustomTabsOption( enableInstantApps: true )
This bundle additionally helps you to ship some header values with the HTTP request, as proven beneath:
customTabsOption: CustomTabsOption( // --- Different choices --- // ... headers: { 'content-type': 'textual content/plain' } )
Notice that these header values must be CORS safelisted request headers — in any other case, it’s worthwhile to configure Google Digital Asset Hyperlinks together with your area identify to ship arbitrary request headers.
iOS and net assist configuration
The flutter_custom_>tabs bundle helps Android, iOS, and net platforms. On Android, it makes use of Chrome or different fallback browsers through the underlying CustomTabsLauncher platform-specific Kotlin library.
On iOS, it makes use of the system-included SFSafariViewController class straight through Flutter technique channel APIs for opening a personalized Safari occasion. On the net platform, it opens a brand new browser tab through the url_launcher_web bundle.
The online model of flutter_custom_tabs just isn’t customizable, since we are able to’t change the browser UI with client-side JavaScript. However, we are able to customise the Safari occasion on iOS in a considerably related style to Customized Tabs. Take a look at the next configuration object:
>customTabsOption: CustomTabsOption( // --- Different choices --- // ... safariVCOption: SafariViewControllerOption( preferredBarTintColor: Colours.blue, preferredControlTintColor: Colours.white, barCollapsingEnabled: true, entersReaderIfAvailable: true, dismissButtonStyle: SafariViewControllerDismissButtonStyle.shut, ), )
The above configuration units the next Safari UI customizations:
- Use the blue shade for the toolbar background with the
preferredBarTintColor
property - Use the white shade for the toolbar parts with the
preferredControlTintColor
property - Auto-collapse the toolbar when the person scrolls with the
barCollapsingEnabled
property - Activate the reader mode routinely with the
entersReaderIfAvailable
property - Use the Shut button to return to the appliance with the
dismissButtonStyle
property
Customized Tabs vs. Default browser vs. Webview
Customized Tabs just isn’t the one strategy to render net content material on Flutter purposes — we’ve got Flutter packages to open the default browser and implement in-app webviews, too. Let’s talk about the professionals and cons of launching the default browser and utilizing webview by evaluating every choice with the Customized Tabs answer.
Level of comparability | Customized Tabs | Default browser | Webview |
---|---|---|---|
Initialization | Affords the quickest initialization time with native Android and Chrome Customized Tabs efficiency optimizations | Webpage initialization is gradual as a result of the default net browser doesn’t use particular initialization efficiency optimizations as Customized Tabs | Webpage initialization could be boosted with varied efficiency tweaks, however webview elements don’t sometimes assist full net APIs and browser options as net browsers do |
Customizability | In a position to customise the UI (Toolbar and menu) in accordance with the Flutter software theme | Not in a position to customise the UI, because the URL launching course of is managed by the Android system — not explicitly by the developer | Extremely customizable if the webview resides in an exercise created by the developer |
UX/Navigating between browser and app | Inbuilt, user-friendly navigation assist between the appliance and Customized Tab | The default browser could seem as a very totally different app in comparison with the Flutter app person expertise, and the person sometimes wants to seek out methods to shut the browser app to return to your app once more | Builders can supply the identical person expertise as the opposite native components of the app with varied UI and inside webview options as a result of they will customise the webview as they want |
Cookie and permissions sharing | Shares the cookie jar and permission mannequin, so customers can see the very same web site state on each Chrome and Customized Tabs situations | Use the identical cookie jar and permission mannequin as a result of URLs are launched in the identical default browser within the system | Neither the cookie jar nor the permission mannequin is shared amongst browsers and webview elements. The person could must check in twice into webpages from the browser and webview, not like with Customized Tabs |
Availability | Out there for Flutter builders through flutter_custom_tabs and flutter_web_browser plugins | Out there for Flutter builders through the url_launcher plugin | Out there for Flutter builders through the url_launcher plugin (very restricted customization) and webview_flutter plugin (versatile customization) |
Let’s summarize the above comparability desk in accordance with your software improvement wants.
When you solely have to show a webpage, think about using Customized Tabs slightly than launching the default browser for a greater person expertise. If it’s worthwhile to current your personal net content material with native-web, communication-bridge-like superior customizations, the webview-oriented strategy provides you full flexibility and assist.
Suppose twice earlier than utilizing the default browser choice — it does change the appliance context, affecting the person expertise.
Greatest practices for implementing Customized Tabs
Growth finest practices all the time assist us to construct high-quality software program methods that customers recognize and should suggest to different customers. As you have got already skilled, implementing Customized Tabs may be very simple with the flutter_custom_tabs bundle. Regardless that the implementation just isn’t advanced, we’d like to consider finest practices for attaining higher software high quality.
Think about the next finest practices if you are working with Customized Tabs in Flutter.
- Attempt to supply the identical feel and appear in Customized Tabs as you do within the software — together with animations and colours much like your software screens
- At all times take a look at fallback choices: Chrome and another well-liked browsers assist Customized Tabs, however in some instances, customers could not use Chrome or a browser that helps Customized Tabs — or the person could not have an internet browser software. Due to this fact, test whether or not your software will use the default browser or a webview implementation in such situations
- Don’t redefine the identical
CustomTabsOption
object in lots of locations by following the DRY programming precept — implement a shared operate for launching all Customized Tabs, as we created the_launchURL
operate earlier than - Add cross-platform assist and take a look at on actual gadgets. Make certain to configure flutter_custom_tabs for iOS through the use of the
safariVCOption
configuration property
Check out the next full configuration for the ultimate bullet level:
void _launchURL(BuildContext context) async { closing theme = Theme.of(context); attempt { launch('https://github.com', // Android Customized Tabs config customTabsOption: CustomTabsOption( showPageTitle: true, toolbarColor: theme.primaryColor, animation: CustomTabsSystemAnimation.fade(), // fallback choices extraCustomTabs: const <String>[ 'org.mozilla.firefox', 'com.microsoft.emmx', ], headers: { 'content-type': 'textual content/plain' } ), // iOS Safari View Controller config safariVCOption: SafariViewControllerOption( preferredBarTintColor: theme.primaryColor, preferredControlTintColor: Colours.white, barCollapsingEnabled: true, entersReaderIfAvailable: false, dismissButtonStyle: SafariViewControllerDismissButtonStyle.shut, ), ); } catch(e) { // Deal with the URL launching failure right here debugPrint(e.toString()); } }
You possibly can obtain a whole pattern Flutter Customized Tabs challenge supply code from my GitHub repository.
Conclusion
On this tutorial, we’ve mentioned nearly all of the options that the flutter_custom_tabs supplies. This bundle supplies a easy operate to combine Customized Tabs in Flutter purposes. Regardless that it presents a minimal Dart operate for working with Customized Tabs, it helps you to make nearly all customizations you want utilizing Chrome Customized tabs in Flutter.
Nonetheless, flutter_custom_tabs has a number of points that must be mounted. For instance, some configuration choices like enableDefaultShare
don’t work as anticipated. Additionally, there is no such thing as a choice for including extra motion/menu gadgets to a selected Customized Tab, though the native Android API helps customized motion/menu gadgets in Customized Tabs. I additionally observed that the Customized Tab closing animation didn’t work correctly. As a result of these points, Flutter builders began supporting some different plugins, like flutter_web_browser.
However, total, the flutter_custom_tabs bundle continues to be the most effective Flutter Customized Tabs plugin on the market, and it presents a versatile and fully-featured interface for builders. It even supplies you Customized Tabs-like implementations for each Flutter net and iOS. This bundle has a excessive recognition rating on the Dart packages registry and has a well-structured codebase — therefore, we are able to use flutter_custom_tabs
in manufacturing apps with none challenge.
Package deal maintainers will add the lacking options and repair current points quickly to assist your complete Flutter developer group.
LogRocket: Full visibility into your net apps
LogRocket is a frontend software monitoring answer that allows you to replay issues as in the event that they occurred in your personal browser. As an alternative of guessing why errors occur, or asking customers for screenshots and log dumps, LogRocket helps you to replay the session to rapidly perceive what went flawed. It really works completely with any app, no matter framework, and has plugins to log extra context from Redux, Vuex, and @ngrx/retailer.
Along with logging Redux actions and state, LogRocket data console logs, JavaScript errors, stacktraces, community requests/responses with headers + our bodies, browser metadata, and customized logs. It additionally devices the DOM to report the HTML and CSS on the web page, recreating pixel-perfect movies of even essentially the most advanced single-page apps.