As soon as, most internet purposes refreshed all the internet web page with consumer actions to speak with internet servers. Later, the AJAX (Asynchronous JavaScript and XML) idea made internet utility content material dynamic with no need to reload webpages by providing a method to talk with internet servers within the background.
AJAX then grew to become a W3C-approved internet commonplace. Because of this, builders can now use AJAX APIs in any commonplace browser to fetch JSON, XML, HTML, or uncooked string content material in internet pages by way of the HTTP protocol.
The React Native framework affords a cross-platform utility improvement answer with an embedded JavaScript engine (Hermes), platform-specific UI aspect wrappers, and React. The React Native framework implements polyfills for AJAX browser APIs with underlying platform-specific networking APIs, so we will use commonplace JavaScript internet APIs for sending AJAX requests in React Native.
On this tutorial, I’ll reveal varied approaches for dealing with AJAX requests in your React Native apps with sensible examples. Additionally, I’ll clarify deal with AJAX errors and community latencies in a user-friendly method.
Leap forward:
Methods to make AJAX requests in React Native
Let’s begin by figuring out the doable methods to make AJAX requests in React Native — then, we will virtually take a look at them with examples. We are able to name these HTTP shopper libraries or APIs since they each assist us to speak with a server utilizing the HTTP protocol.
We are able to use the next inbuilt and library-based methods to make AJAX requests:
The inbuilt Fetch API
In 2015, the Fetch API was launched as a contemporary successor to the well-known legacy XMLHttpRequest API. Fetch affords a promise-based trendy interface for sending AJAX requests with many HTTP configurations, similar to HTTP strategies, headers, payloads, and many others. You don’t want a third-party polyfill to make use of Fetch in React Native since it’s a pre-included characteristic within the React Native framework.
The inbuilt XMLHttpRequest API
XMLHttpRequest (a.okay.a. XHR) was the oldest commonplace API for sending AJAX requests earlier than the Fetch API. The XHR API affords a standard event-based strategy for AJAX programming. React Native affords this API together with the Fetch API for networking necessities. Trendy builders these days don’t attempt to use the XHR API straight, primarily because of Fetch and different developer-friendly AJAX libraries, like Axios.
Axios
Axios is a well-liked open-source HTTP shopper library that works on browser environments (together with IE 11) and Node.js. Most frontend builders these days select Axios attributable to its minimal, promise-based API, computerized request/response transformation, and world configuration assist.
Different AJAX libraries
Whereas Axios is a well-liked HTTP shopper library, the JavaScript library ecosystem has a whole bunch of open-source options. These options act as a wrapper for the XMLHttpRequest
object or Fetch
operate, as Axios does.
The Wretch and SuperAgent libraries are fashionable options to Axios.
AJAX with caching libraries
Most internet purposes carry out CRUD operations by speaking with a RESTful internet API, which use the AJAX idea to speak with their backend internet APIs. Builders usually have to write down further code to enhance the usability of CRUD operations within the frontend.
For instance, we will load regularly used information sooner on the UI by caching information within the frontend, and decrease bandwidth utilization by deduping community calls. TanStack Question (previously React Question) and SWR-like caching libraries assist builders to make AJAX requests effectively and in a user-friendly method by writing much less code.
A React Native AJAX tutorial six methods
We’re going to create a full-stack cell app that lists guide particulars. First, let’s create a easy RESTful API, then we will eat it utilizing the varied inbuilt AJAX APIs and libraries above to grasp make AJAX requests in React Native.
Making a RESTful backend
Create a brand new listing and Node.js undertaking with the next instructions:
mkdir bookstore-backend cd bookstore-backend npm init # --- or --- yarn init
Add the Specific.js library to start out creating the RESTful API:
Extra nice articles from LogRocket:
npm set up specific # --- or --- yarn add specific
Create a brand new file named server.js
, and add the next code:
const specific = require('specific'); const app = specific(); const books = [ { id: 100, title: 'Golang experts', author: 'John Stack', price: 200 }, { id: 101, title: 'C++ for beginners', author: 'John Doe', price: 250 }, { id: 102, title: 'Flutter development', author: 'Steven Doe', price: 350 }, { id: 103, title: 'JavaScript internals', author: 'John Stack', price: 300 } ]; const port = 5000; app.get('/books', (req, res) => { res.json(books); }); app.pay attention(port, () => { console.log(`Bookstore API is working at port ${port}`); });
Right here, we return a hard-coded guide listing by way of the GET /books
endpoint. Begin the RESTful API server with the next command:
node server.js
Check the app backend by navigating to http://localhost:5000/books
out of your browser or making a request with the curl
command. You’ll get the guide listing as a JSON array as follows:
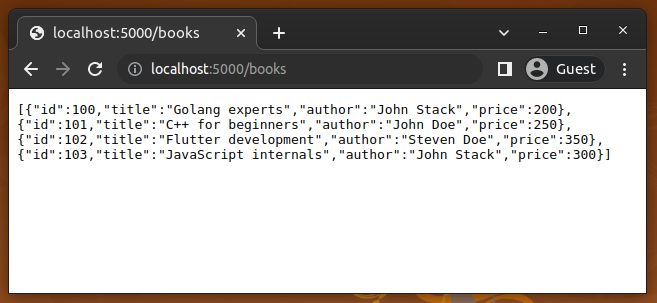
Let’s make AJAX requests to the GET /books
endpoint with the above APIs and libraries by making a easy React Native bookstore app. You may maintain this server occasion working within the background, since we’ll want it quickly after we implement the UI.
Creating the app frontend
Now, we’ll create our app’s React Native frontend, which may have minimal error dealing with options and a local loading indicator.
First, we are going to develop the frontend with a hard-coded guide listing on the client-side, then we are going to modify the app’s supply code to fetch the guide info from the backend by way of varied AJAX APIs and libraries.
Create a brand new React Native undertaking:
npx react-native init Bookstore
The above command will scaffold a brand new undertaking within the Bookstore
listing. Subsequent, run the app on a bodily system or emulator/simulator to ensure that every thing works fantastic:
cd Bookstore npm begin # --- or --- yarn begin npx react-native run-android # --- or --- npx react-native run-ios
Change your App.js
supply file’s content material with the next supply code:
import React, { useState, useEffect } from 'react'; import { SafeAreaView, View, FlatList, StyleSheet, Textual content, ActivityIndicator, } from 'react-native'; const BookItem = ({ title, creator, value }) => ( <View model={types.merchandise}> <View model={types.itemLeft}> <Textual content model={types.title}>{title}</Textual content> <Textual content model={types.creator}>{creator}</Textual content> </View> <View model={types.itemRight}> <Textual content model={types.value}>${value}</Textual content> </View> </View> ); const BookList = () => { const [books, setBooks] = useState([]); const [loading, setLoading] = useState(true); const [error, setError] = useState(false); async operate getBooks() { await new Promise((resolve) => setTimeout(resolve, 2000)); setBooks([{ id: 100, title: 'Golang experts', author: 'John Stack', price: 200 }, { id: 101, title: 'C++ for beginners', author: 'John Doe', price: 250 }]); setLoading(false); } useEffect(() => { getBooks(); }, []); if(error) return <Textual content model={types.errorMsg}>Unable to connect with the server.</Textual content>; if(loading) return <ActivityIndicator measurement="giant"/>; return ( <FlatList information={books} renderItem={({ merchandise }) => <BookItem {...merchandise} />} keyExtractor={merchandise => merchandise.id} /> ); } const App = () => { return ( <SafeAreaView model={types.container}> <BookList/> </SafeAreaView> ); } const types = StyleSheet.create({ container: { flex: 1, marginTop: '10%', }, merchandise: { flex: 1, flexDirection: 'row', flexWrap: 'wrap', alignItems: 'flex-start', backgroundColor: '#eee', padding: 12, marginVertical: 8, marginHorizontal: 16, }, itemLeft: { width: '70%' }, itemRight: { width: '30%', }, title: { fontSize: 22, coloration: '#222', }, creator: { fontSize: 16, coloration: '#333', }, value: { fontSize: 20, coloration: '#333', textAlign: 'proper' }, errorMsg: { coloration: '#ee8888', fontSize: 20, padding: 24, textAlign: 'middle' }, }); export default App;
The above code renders a guide listing utilizing the inbuilt React Native FlatList
part. As talked about earlier than, we aren’t utilizing any AJAX request handlers but, however we simulated a community request with the getBooks
asynchronous operate. We initially carried out the app utilizing a simulated community operation with hard-coded information to simply reveal all AJAX dealing with strategies by modifying the getBooks
operate solely.
The getBooks
operate simulates the community latency with the setTimeout
operate and returns hard-coded information after 2000 milliseconds. The UI renders a loading animation to point the community latency and an error message if we invoke setError(true)
.
I used loading
and error
state variables within the App
part since I solely name the getBooks
operate as soon as. However, if we name getBooks
in lots of locations, we will implement a reusable customized Hook known as useBooks
for higher code high quality. Study extra about creating customized Hooks from this text.
When you run the above code, you will note the next consequence:
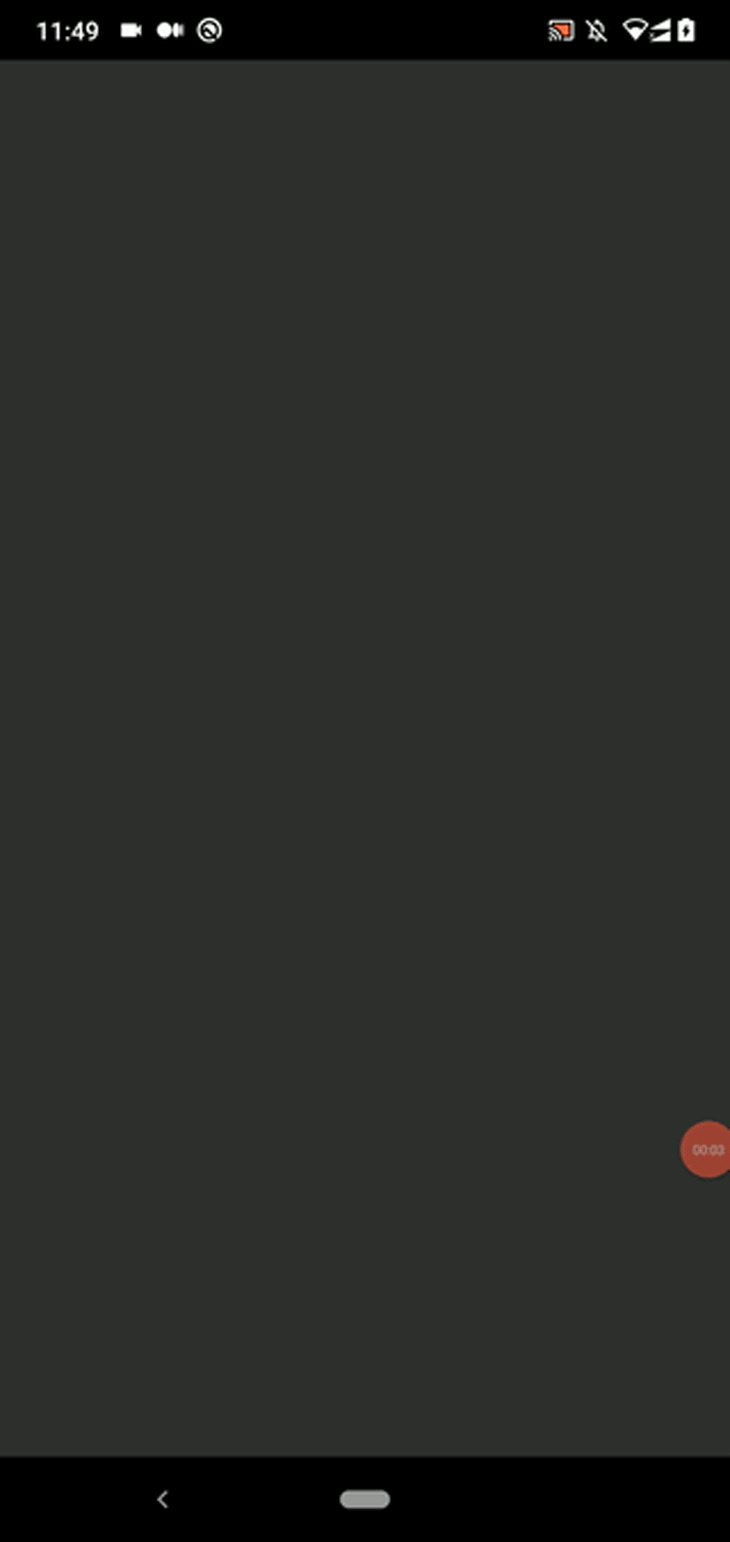
Utilizing the Fetch API
Let’s load books to the React Native bookstore app from our backend internet service with the inbuilt Fetch API. We don’t must import anything, for the reason that fetch
operate resides within the world script scope much like the browser setting.
Use the next code for the present getBooks
operate:
async operate getBooks() { attempt { const books = await fetch('http://<computer_ip>:5000/books') .then((response) => response.json() ); setBooks(books); } catch(err) { setError(true); } lastly { setLoading(false); } }
Be certain to make use of the identical WiFi community for each your pc and cell take a look at system, then enter your pc’s native IP deal with within the above URL the place it says computer_ip
. The awaited fetch
operate doesn’t return JSON straight (it returns a Response
object), so now we have to make use of then
as soon as to get the promise, which then resolves to the obtained JSON content material.
When you run the app, you will note guide particulars from the backend as follows:
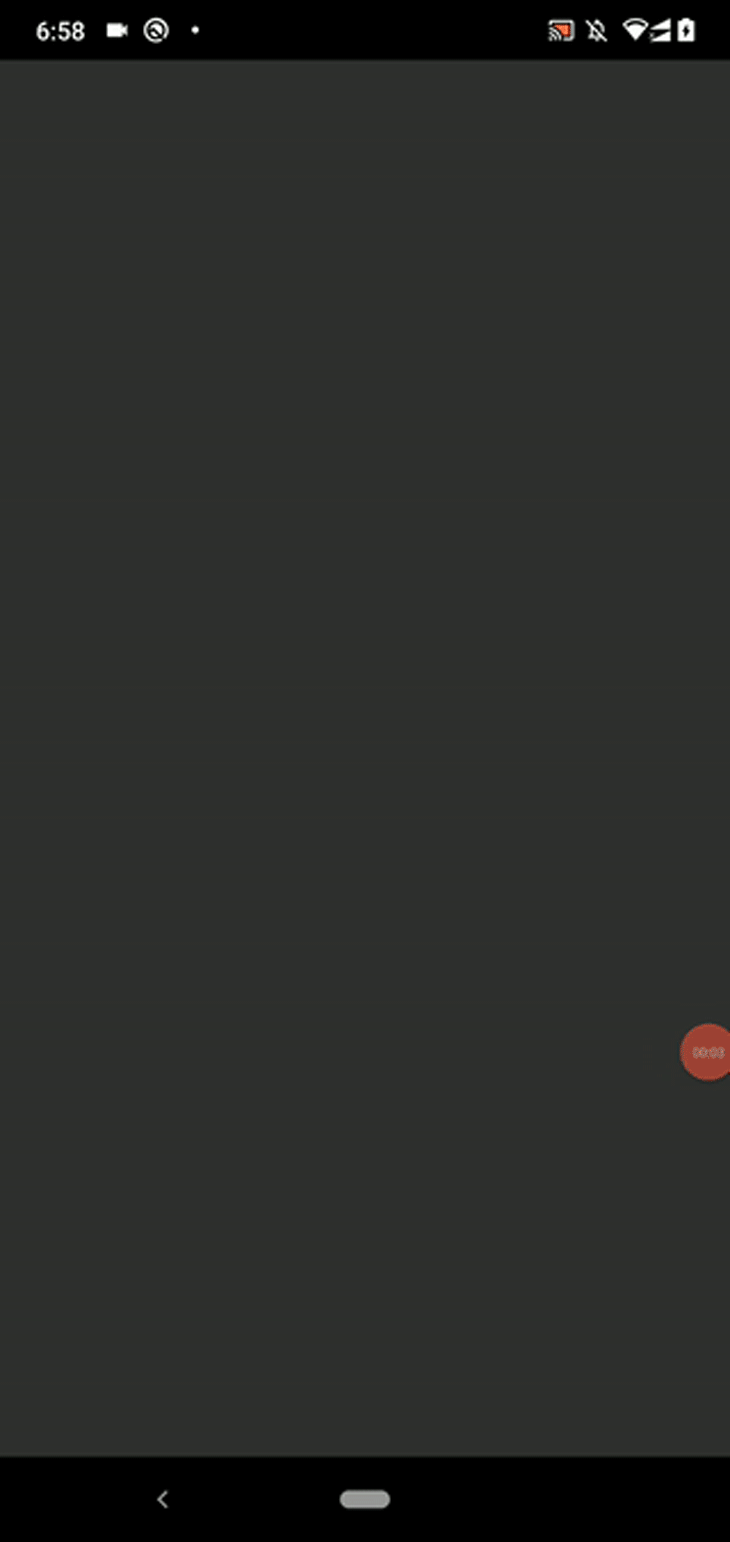
fetch
accepts an choices object that allows you to set the HTTP technique, physique, headers, and many others. Take a look at the next code snippet that performs a POST
request:
const choices = { technique: 'POST', physique: JSON.stringify({inStock: false}) }; await fetch('/books', choices) .then((response) => response.json() );
If you happen to make AJAX requests to a number of endpoints, you need to use the Fetch API like fetch('/books')
, fetch('/books/100')
, and many others. with a world base URL configuration by implementing a world Fetch interceptor.
Earlier, we didn’t get an opportunity to carry out fundamental error dealing with exams as a result of we didn’t invoke the setError(true)
operate name. Now, we will take a look at it by working the frontend with out working the server. You need to get an error message as an alternative of the guide listing, as proven beneath:
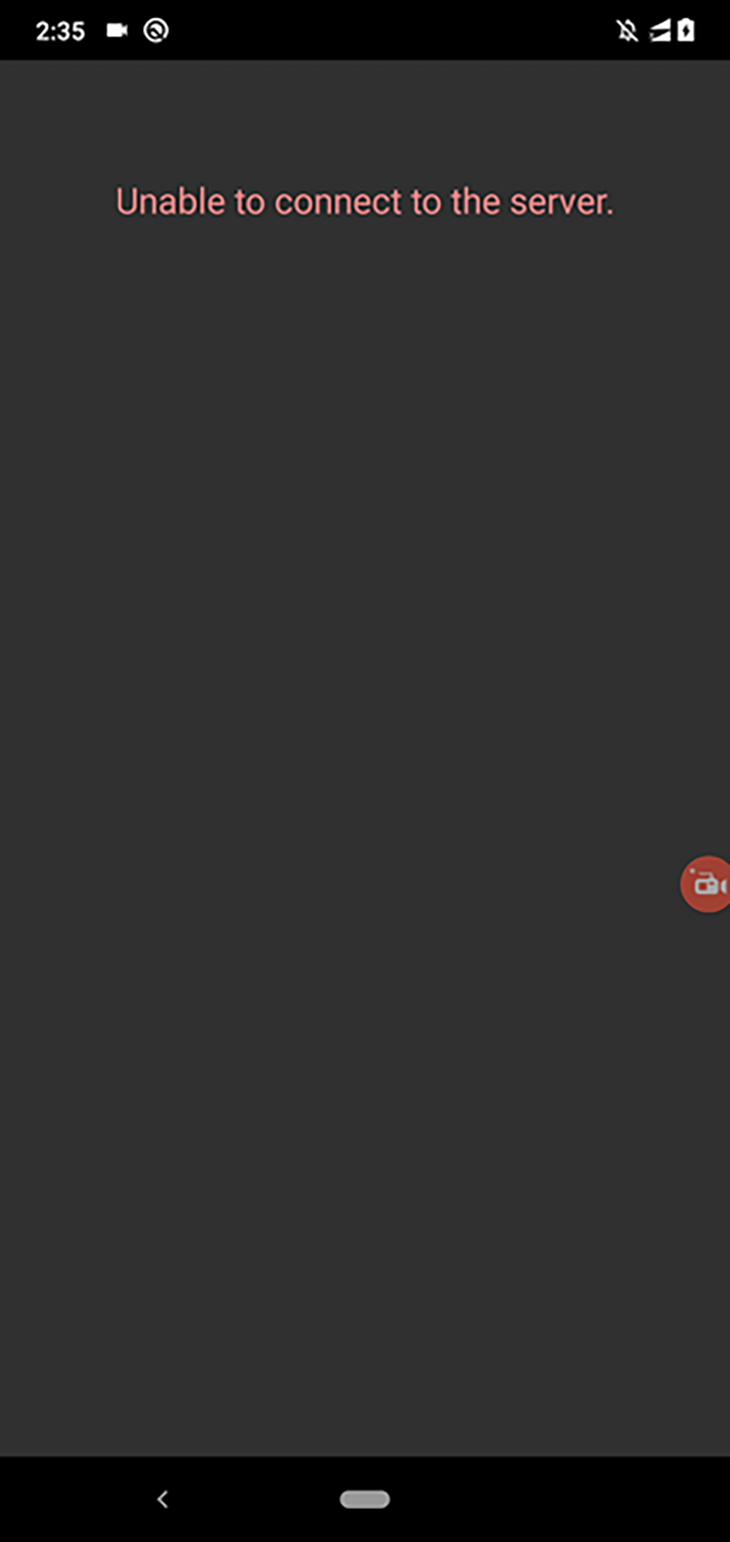
We’ll talk about different fashionable AJAX error dealing with methods in a separate part.
Utilizing Axios
First, set up the Axios package deal:
npm set up axios # --- or --- yarn add axios
Subsequent, import the axios
object, as proven beneath:
import axios from 'axios';
Now, replace the getBooks
operate with the next code to make an AJAX request with Axios:
async operate getBooks() { attempt { const { information: books } = await axios.get('http://<computer-ip>:5000/books'); setBooks(books); } catch(err) { setError(true); } lastly { setLoading(false); } }
Axios transforms information based on the HTTP response content material sort, so we don’t must request JSON explicitly, as we did in our Fetch API instance. Right here, we use JavaScript’s destructuring syntax to assign the information
property worth to the books
variable immediately.
Axios affords us handy features for HTTP strategies. For instance, we will make a POST
request as follows:
await axios.put up('/books/101', {inStock: false});
Just like the GET
response, we will put up JSON content material straight with out utilizing the JSON.stringify
operate, not like with fetch
.
If you happen to make AJAX requests to a number of endpoints, you need to use Axios like axios.get('/books')
, axios.patch('/books/100')
, and many others., with a world base URL configuration by updating your config defaults.
Utilizing various AJAX libraries SuperAgent and Wretch
There are numerous Axios options on the market. Let’s attempt two trending HTTP shopper libraries: SuperAgent and Wretch.
Set up the SuperAgent package deal to get began:
npm set up superagent # --- or --- yarn add superagent
Now, import the superagent
API as follows:
import superagent from 'superagent';
Add the next code to the getBooks
operate to finish the implementation:
async operate getBooks() { attempt { const { physique: books } = await superagent.get('http://<computer-ip>:5000/books'); setBooks(books); } catch(err) { setError(true); } lastly { setLoading(false); } }
SuperAgent affords a promise-based API much like Axios, so we will use the fashionable await
key phrase, as proven above. It affords a plugin system to increase the performance of the library. For instance, we will use the superagent-use
plugin to outline a world configuration and superagent-prefix
to outline a base URL for all requests.
Wretch is a wrapper round fetch
with a extra readable API than Fetch. It eliminates the extreme callback within the inbuilt fetch
operate whereas requesting JSON information. Let’s use Wretch in our app!
First, set up the Wretch package deal:
npm set up wretch # --- or --- yarn add wretch
Subsequent, use the wretch
operate within the getBooks
operate, as proven beneath:
async operate getBooks() { attempt { const books = await wretch('http://<computer-ip>:5000/books').get().json(); setBooks(books); } catch(err) { setError(true); } lastly { setLoading(false); } }
As you may see, wretch
tries to supply a extra minimal API than fetch
with a extra developer-friendly syntax. The json
operate gives a promise that resolves to the JSON content material — we will use await
in a extra intuitive method than inbuilt fetch
.
Wretch affords you pre-built trendy middlewares for request deduplication, caching, retrying, and delaying requests. Dealing with POST
requests can be straightforward as Axios:
await wretch('/books/101').put up({inStock: false}).json();
Utilizing TanStack Question
All of the above methods provide good options for dealing with AJAX requests in React Native — we will use them to attach app frontends with RESTful backends by way of the HTTP protocol. However a typical trendy React Native app goes past fundamental AJAX dealing with with caching, request deduplication, computerized retries, and varied usability enhancements. Some AJAX libraries like Wretch provide trendy middlewares for a few of these necessities, however utilizing a devoted caching library brings you a lot pre-built usability and efficiency enhancements for dealing with AJAX information.
You may combine a caching library with a most popular AJAX library for usability and efficiency enhancements. Let’s use TanStack Question together with Axios for higher AJAX dealing with.
Just remember to’ve already put in the Axios library into your undertaking. Subsequent, set up the TanStack Question library, as proven beneath:
npm set up @tanstack/react-query # --- or --- yarn add @tanstack/react-query
Now, substitute your App.js
supply code with the next code:
import React, { useState } from 'react'; import { SafeAreaView, View, FlatList, StyleSheet, Textual content, ActivityIndicator, } from 'react-native'; import { useQuery, useQueryClient, QueryClient, QueryClientProvider, } from '@tanstack/react-query'; import axios from 'axios'; const BookItem = ({ title, creator, value }) => ( <View model={types.merchandise}> <View model={types.itemLeft}> <Textual content model={types.title}>{title}</Textual content> <Textual content model={types.creator}>{creator}</Textual content> </View> <View model={types.itemRight}> <Textual content model={types.value}>${value}</Textual content> </View> </View> ); const BookList = () => { const [books, setBooks] = useState([]); const [loading, setLoading] = useState(true); const queryClient = useQueryClient(); const question = useQuery(['books'], getBooks); async operate getBooks() { const response = await axios.get('http://<computer-ip>:5000/books'); return response.information; } if(question.isLoading) return <ActivityIndicator measurement="giant"/>; if(question.isError) return <Textual content model={types.errorMsg}>Unable to connect with the server.</Textual content>; return ( <FlatList information={question.information} renderItem={({ merchandise }) => <BookItem {...merchandise} />} keyExtractor={merchandise => merchandise.id} /> ); } const App = () => { const queryClient = new QueryClient(); return ( <QueryClientProvider shopper={queryClient}> <SafeAreaView model={types.container}> <BookList/> </SafeAreaView> </QueryClientProvider> ); } const types = StyleSheet.create({ container: { flex: 1, marginTop: '10%', }, merchandise: { flex: 1, flexDirection: 'row', flexWrap: 'wrap', alignItems: 'flex-start', backgroundColor: '#eee', padding: 12, marginVertical: 8, marginHorizontal: 16, }, itemLeft: { width: '70%' }, itemRight: { width: '30%', }, title: { fontSize: 22, coloration: '#222', }, creator: { fontSize: 16, coloration: '#333', }, value: { fontSize: 20, coloration: '#333', textAlign: 'proper' }, errorMsg: { coloration: '#ee8888', fontSize: 20, padding: 24, textAlign: 'middle' } }); export default App;
As you may see, TanStack Question affords you information loading and error statuses, so that you don’t must outline a number of state variables or customized Hooks to implement the loading state and error messages. Right here, we present the loading animation based on the isLoading
property and an error message based on the isError
property.
TanStack Question implements question deduplication by default, so when you use the identical request twice, your app will solely make one HTTP request. Confirm it by utilizing two BookList
part situations, as follows:
<SafeAreaView model={types.container}> <BookList/> <BookList/> </SafeAreaView>
You could find the variety of invocations of the GET /books
endpoint by utilizing a breakpoint or a easy console.log
, as follows:
app.get('/books', operate (req, res) { console.log('Check'); res.json(books); });
Research extra about caching libraries and discover a most popular one in your wants with this text.
Dealing with AJAX errors
AJAX requests can fail attributable to community points, server-side breakdowns, and internet area configuration issues. If we don’t deal with such errors within the frontend, customers could face non-user-friendly app behaviors. Due to this fact, it’s at all times good to implement error dealing with methods in AJAX-oriented React Native apps for enhanced user-friendliness.
Builders sometimes use one of many following methods to deal with AJAX errors in a user-friendly method:
- Present an error message for the consumer by explaining the failure motive, as we did within the earlier examples
- Retry the AJAX request routinely a number of occasions, and present an error message if it nonetheless fails
- Provide customers a method to retry the failed request manually with a button
- Implement computerized retry, a developer-friendly error message, and a guide retry motion
- Retry when the consumer connects to the web after being offline through the AJAX requests’ transport
TanStack Question retries failed requests a number of occasions till it receives information from the info supplier operate. Fetch customers can use the fetch-retry
package deal, and Axios customers can use the axios-retry
package deal to implement computerized retries for AJAX requests.
For instance, take a look at the next code snippet that makes use of axios-retry.
import axiosRetry from 'axios-retry'; axiosRetry(axios, { retryCondition: () => true, retries: 3, retryDelay: () => 1000 });
Right here, we set three retry makes an attempt with a 1000 millisecond delay in between every retry try. Add the above code to the bookstore app, begin the app with out working the backend, let the loading animation spin a second, after which begin the RESTful server. You will note the guide listing because of computerized retries:
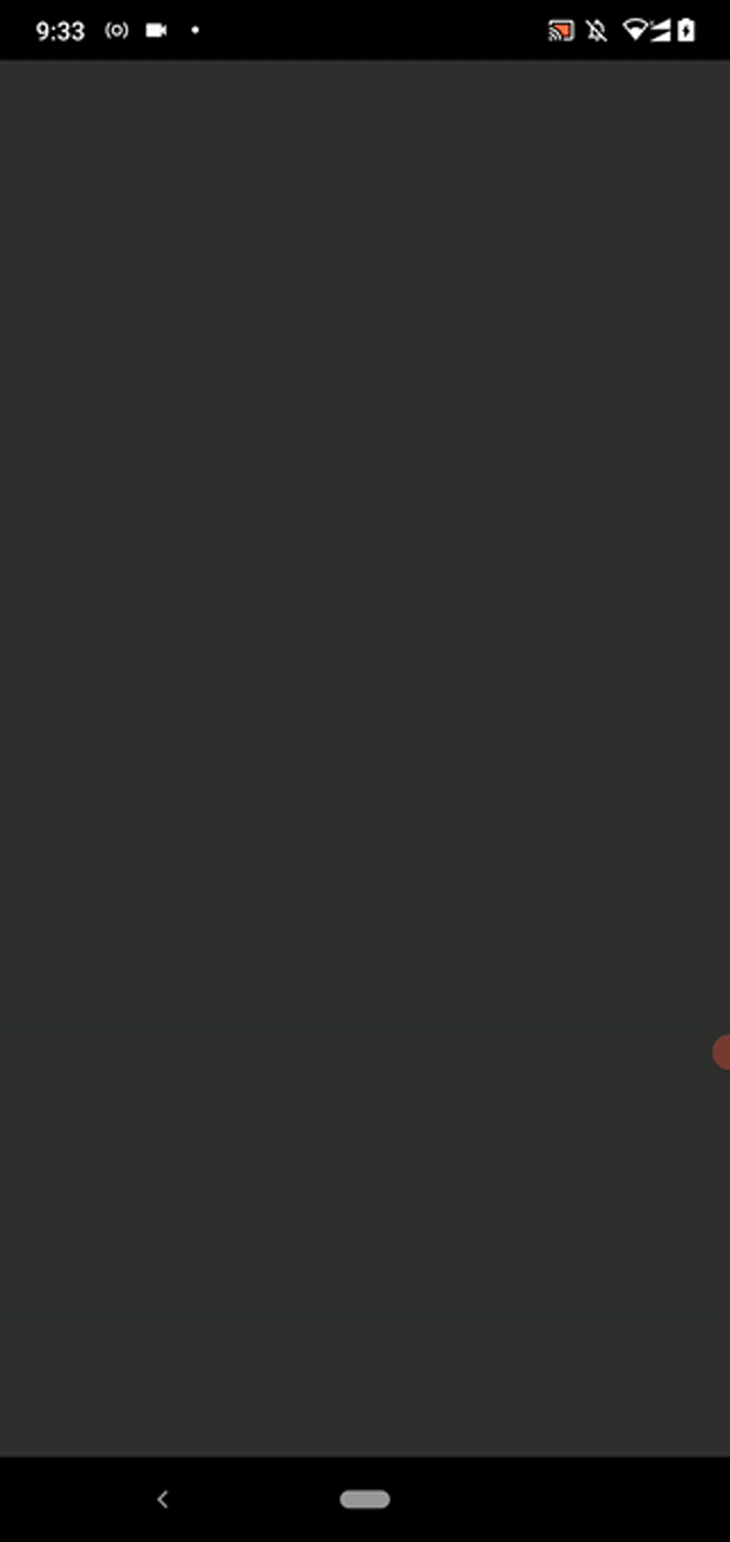
If we don’t begin the RESTful server for 3 seconds, you will note the error message as a result of the axios-retry library gained’t make greater than three retries with the above setup:
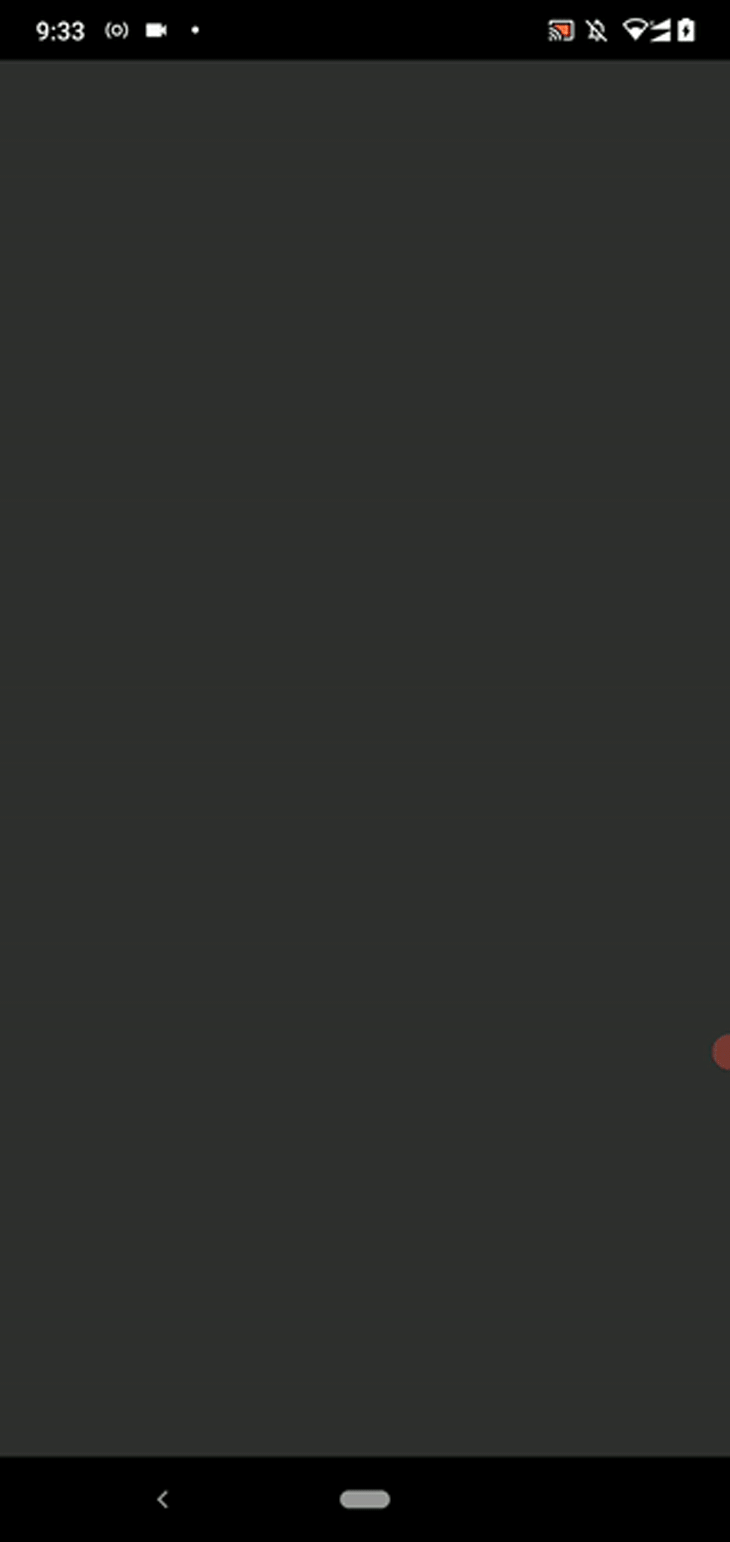
We are able to additionally use the exponential backoff retry idea to implement retries by contemplating system and server-side useful resource utilization. Select an excellent AJAX error dealing with technique like computerized retries for higher utility usability.
You can even add a guide retry button with the next code:
import React, { useState, useEffect } from 'react'; import { Button, SafeAreaView, View, FlatList, StyleSheet, Textual content, ActivityIndicator, } from 'react-native'; import axios from 'axios'; import axiosRetry from 'axios-retry'; axiosRetry(axios, { retryCondition: () => true, retries: 3, retryDelay: () => 1000 }); const BookItem = ({ title, creator, value }) => ( <View model={types.merchandise}> <View model={types.itemLeft}> <Textual content model={types.title}>{title}</Textual content> <Textual content model={types.creator}>{creator}</Textual content> </View> <View model={types.itemRight}> <Textual content model={types.value}>${value}</Textual content> </View> </View> ); const BookList = () => { const [books, setBooks] = useState([]); const [loading, setLoading] = useState(true); const [error, setError] = useState(false); async operate getBooks() { attempt { setLoading(true); setError(false); const { information: books } = await axios.get('http://<computer-ip>:5000/books'); setBooks(books); } catch(err) { setError(true); } lastly { setLoading(false); } } useEffect(() => { getBooks(); }, []); if(error) return ( <View> <Textual content model={types.errorMsg}>Unable to connect with the server.</Textual content> <View model={types.btnWrapper}> <Button onPress={() => getBooks()} title="Retry"></Button> </View> </View> ); if(loading) return <ActivityIndicator measurement="giant"/>; return ( <FlatList information={books} renderItem={({ merchandise }) => <BookItem {...merchandise} />} keyExtractor={merchandise => merchandise.id} /> ); } const App = () => { return ( <SafeAreaView model={types.container}> <BookList/> </SafeAreaView> ); } const types = StyleSheet.create({ container: { flex: 1, marginTop: '10%', }, merchandise: { flex: 1, flexDirection: 'row', flexWrap: 'wrap', alignItems: 'flex-start', backgroundColor: '#eee', padding: 12, marginVertical: 8, marginHorizontal: 16, }, itemLeft: { width: '70%' }, itemRight: { width: '30%', }, title: { fontSize: 22, coloration: '#222', }, creator: { fontSize: 16, coloration: '#333', }, value: { fontSize: 20, coloration: '#333', textAlign: 'proper' }, errorMsg: { coloration: '#ee8888', fontSize: 20, padding: 24, textAlign: 'middle' }, btnWrapper: { paddingLeft: '15%', paddingRight: '15%', paddingTop: '15%' }, }); export default App;
The above code produces a retry button after an AJAX request failure. If the net server works once more if you click on the Retry button, the guide listing will work as typical, as proven within the preview beneath:
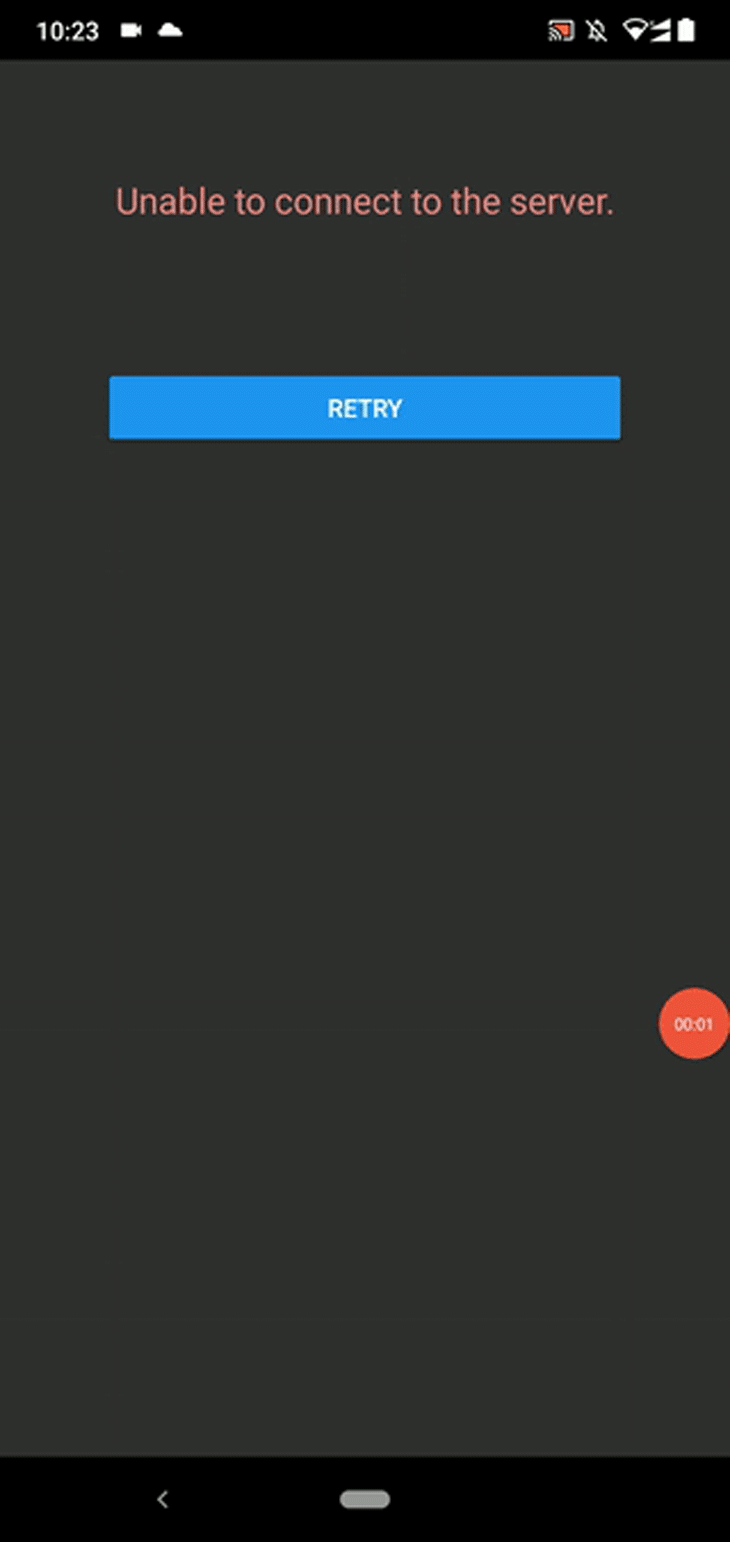
Fetch vs. Axios vs. Different HTTP purchasers vs. TanStack Question
Now, now we have despatched AJAX requests to a RESTful internet API by way of inbuilt APIs and varied third-party libraries. Each strategy is competitively good, however which one do you propose to make use of in your subsequent React Native app?
Earlier than you determine, it’s price noting that TanStack Question isn’t one more HTTP shopper — it’s a caching library that you need to use together with any HTTP shopper library for syncing your app frontend and internet providers.
Select one based on the next comparability elements:
Comparability issue | Fetch | Axios | Different purchasers (SuperAgent, Wretch, and many others.) | TanStack Question |
---|---|---|---|---|
API sort | Promise-based minimal | Promise-based minimal | Promise-based minimal/detailed, legacy event-based, or callback-based | Detailed OOP, callback-based |
International configuration assist | Not supported as an inbuilt characteristic, but it surely’s doable by making a wrapper/interceptor | Supported, often called config defaults | Most libraries assist setting world configurations by making a customized library occasion | Supported, supplied by way of the QueryClient class |
Underlying React Native JavaScript API utilized in Hermes | Fetch | XMLHttpRequest | Fetch, XMLHttpRequest | Is dependent upon the HTTP shopper |
Usability and efficiency enhancements: caching, deduping requests, and many others. | Builders should implement them themselves or use a group library | Builders should implement them themselves or use a group library | Builders should implement them themselves or use a group library. Wretch affords official extensions for deduping requests, retrying, delaying requests, and caching | Caching, cache manipulation (for constructing realtime UIs), deduping requests, and computerized retries by default. Builders can simply implement re-fetch information on focus, community standing change information refetching, and many others. |
Extending AJAX request/response dealing with logic | Doable with wrappers/interceptors | Doable with request/response interceptors | Most libraries include inbuilt plugin methods. i.e., Wretch middlewares, SuperAgent plugins, and many others. | The accountability of sending AJAX requests doesn’t belong to this library because it expects information by way of a promise. Prolong your AJAX library as an alternative. |
Conclusion
We studied varied methods to ship AJAX requests in React Native on this tutorial. We in contrast every strategy primarily based on a number of essential comparability elements. Up to now, internet apps fetched XML paperwork and HTML segments with AJAX to make internet pages dynamic. Internet and React Native cell apps sometimes use RESTful JSON-based internet APIs by way of AJAX.
It’s doable to make use of any most popular doc format like YAML, XML, and many others., as a communication message format for internet providers. However, these days, JSON is the trade default for transporting information with AJAX. React Native builders may use WebSockets to transcend AJAX’s request-response mannequin.
Many builders favor AJAX due to the readable and developer-friendly RESTful mannequin, inbuilt APIs and library availability, and easiness in HTTP-oriented improvement. Select a way to ship AJAX requests from the above strategies and deal with errors in a user-friendly and hardware-resources-friendly method.
LogRocket: Immediately recreate points in your React Native apps.
LogRocket is a React Native monitoring answer that helps you reproduce points immediately, prioritize bugs, and perceive efficiency in your React Native apps.
LogRocket additionally helps you enhance conversion charges and product utilization by exhibiting you precisely how customers are interacting along with your app. LogRocket’s product analytics options floor the the reason why customers do not full a selected circulation or do not undertake a brand new characteristic.
Begin proactively monitoring your React Native apps — attempt LogRocket without spending a dime.