Have you ever ever wished to make use of a shiny new function in a brand new launch of JavaScript, however couldn’t due to browser compatibility? Perhaps your group needs to check out new ES2015 options, however can’t as a result of a variety of browsers don’t assist it but?
If both of the above conditions resonates with you, odds are you’ve most likely used a transpiler to generate a model of your code that labored on most browser variations. This might assist you to use newer options whereas nonetheless supporting a variety of browser variations.
Nevertheless, when you solely need to use a small variety of options, a transpiler could also be overkill — a few of them add vital overhead that will increase the bundle dimension. In such instances, a polyfill would possibly work higher.
On this article, we’ll focus on how one can use polyfills in your React apps, masking the next matters:
What’s a polyfill?
A polyfill permits you to use options that aren’t supported by a browser (or a particular browser model) by including a fallback that mimics the specified habits utilizing supported APIs. You should utilize a polyfill while you need to use a JavaScript function like String.padEnd
, which isn’t supported by older browsers.
By together with a fallback for browsers that don’t assist it but, you should utilize such new options in growth whereas nonetheless transport code that’s assured to run on all browsers.
Most main browsers are up to date recurrently to incorporate assist for brand spanking new JavaScript and CSS options, however lots of people hardly replace their browsers, which is the place the necessity for transpilers and polyfills is available in.
In a perfect world, everybody would replace their browsers instantly to assist new options as they’re shipped, and we wouldn’t must transpile our code. Till that day comes, nonetheless, we have to assist older variations of browsers.
Utilizing a JavaScript polyfill in React: A sensible instance
Let’s implement a quite simple function utilizing String.padEnd
in a React app.
String.padEnd
was launched together with String.padStart
in ECMAScript 2019 and is at the moment supported by the most recent model of each main browser (we don’t speak about Web Explorer anymore).
Nevertheless, we’ll add a polyfill to allow it to run on these older variations with out assist. First, we’ll write our polyfill after which check out utilizing a devoted polyfill library to deal with it for us. By implementing each approaches, we should always perceive how polyfills work a little bit higher.
The function to be carried out
Let’s envision a consumer who says, “I need to have the ability to view every day of the week together with an emoji. The weekdays ought to be displayed in a tabular format.”
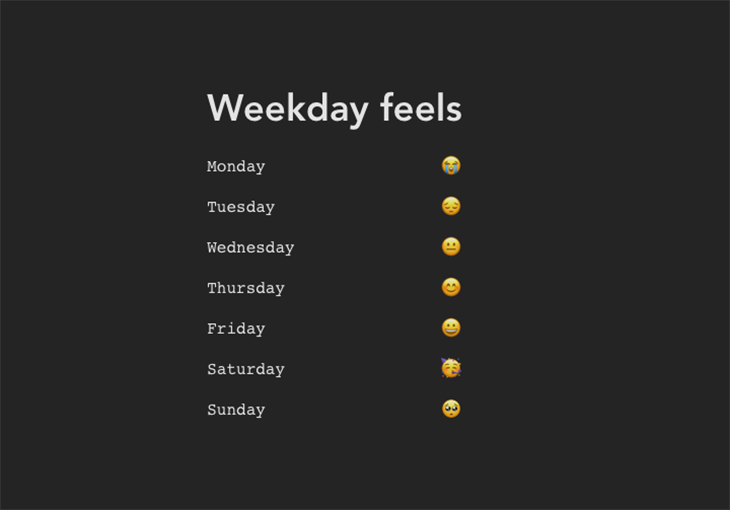
This can be a quite simple function the place we make the most of the padEnd
perform to generate equal areas between the weekday and its corresponding emoji to simulate a desk view. It’s a foolish function, however hopefully ought to be ample for studying how polyfills work.
Challenge setup
To get began, clone this repository containing a starter React and Vite template.
Open up your terminal and run the next command to get a ready-made template.
git clone https://github.com/ovieokeh/react-polyfill-tutorial.git
Navigate into the newly created folder.
cd react-polyfill-tutorial
Set up the required dependencies and begin the appliance.
Extra nice articles from LogRocket:
npm set up npm run dev
How we’re simulating older browser variations
It’ll be cumbersome to obtain older variations of a browser to simulate not having the String.padEnd
function, and due to this, I’ve included a script within the HTML that deletes padEnd
from the String
prototype. To view this script, open the index.html
file.
It’s best to see the under design:
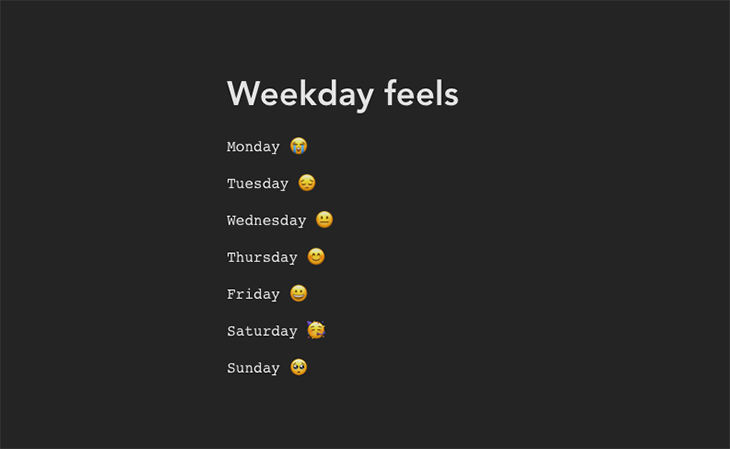
Writing a polyfill from scratch
Now that we have now our undertaking working, let’s implement the tabular design.
Open up the src/App.jsx
file in your most well-liked editor and replace line 29 to pad the day.identify
attribute with 23 areas.
// App.jsx // ... line 28 const dayName = day.identify.padEnd(23); // ... line 30
Now, save your modifications and look at the consequence within the browser. You’ll discover an error in your browser console, as we’re simulating the browser not having the String.padEnd
function.
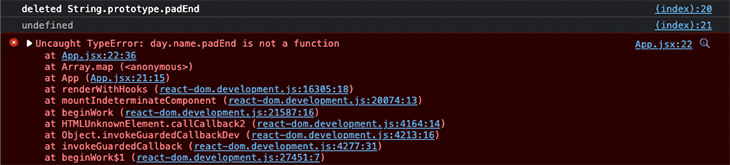
We are able to repair this by writing our polyfill. Discover there’s a remark above the App
element (line 13 of the repo). Let’s add some code there like so:
// ... line 13 if (!String.prototype.padEnd) { console.log("padEnd just isn't supported. Utilizing polyfill."); String.prototype.padEnd = perform padEnd(targetLength, padString) { targetLength = typeof targetLength !== "quantity" || targetLength < 0 ? 0 : targetLength; padString = String(padString || " "); if (this.size > targetLength) return String(this); targetLength = targetLength - this.size; if (targetLength > padString.size) { padString += padString.repeat(targetLength / padString.size); } return String(this) + padString.slice(0, targetLength); }; } // ... line 32
The contents of the polyfill usually are not as vital as the truth that we’re offering a fallback for a function, String.padEnd
that won’t exist in a browser. (I positively advocate you learn by means of it, after all.) The fallback implements the ECMAScript spec for String.padEnd
.
In abstract, we’re including a verify to see if the padEnd
function is supported within the present browser runtime, and if not, present a fallback that implements the habits as outlined within the official ECMAScript specs.
Should you navigate again to the browser, you must see that the error is gone and the design appears to be like like we count on it to look.
Your code at this stage ought to appear to be this:
Utilizing a polyfill library
Now that we’ve written a polyfill by hand, you must hopefully perceive extra about how polyfills work. Nevertheless, as with most issues in programming, there are already libraries that exist for the only objective of offering polyfills.
As an alternative of doing the verify for the existence of a function after which including a polyfill manually, you should utilize a library with a group of polyfills that can do the identical factor for you.
Let’s discover the 2 main methods of utilizing a polyfill library;
- Loading from a CDN
- Loading from an NPM bundle
Loading from a CDN
There are additionally a number of choices obtainable for loading polyfills from a CDN. The most well-liked one is arguably Polyfill.io, so we’ll use it for instance. Nevertheless, the idea ought to nonetheless apply to some other polyfill library with a CDN URL.
Open the index.html
file and embody a <``script``>
tag on the backside of the file.
// ... line 16 <script defer src="https://polyfill.io/v3/polyfill.min.js?options=String.prototype.padEnd|all the time"></script> // ... line 18
We use the defer
attribute on the script tag to forestall it from blocking the React script from working. Learn extra concerning the defer
attribute right here.
We’re together with a polyfill of the String.prototype.padEnd
function and passing the |all the time
flag. It will pressure the CDN to return the polyfill, as solely browsers with out assist for the padEnd
function will get a JavaScript bundle (keep in mind, most main browsers already assist padEnd
so with out the |all the time
flag, the polyfill received’t be returned).
Now, you may return to the App.jsx
file and delete the guide polyfill we added earlier. Refresh the web page and ensure that the app remains to be working positive. Should you examine the console for logs, you’ll discover that we’re nonetheless simulating deleting the native String.prototype.padEnd
perform.
Despite the fact that we used Polyfill.io for this instance, the idea applies to any polyfill library with a CDN model obtainable.
Your code at this stage ought to appear to be this.
Loading from an npm bundle
One other means to make use of a polyfill library is thru npm
. There are packages you may set up from npm
that can assist you to present polyfills for JavaScript options.
The most well-liked by far is core-js
, so we’ll use that for this instance, however I think about most different libraries ought to comply with an analogous conference.
Open your terminal within the undertaking root and set up the core-js
bundle like so:
npm i core-js
With the bundle put in, we will embody the precise polyfill we’d like, which, on this case, is String.prototype.padEnd
as a result of we’re simulating a runtime with out native assist for padEnd
.
Open the App.jsx
app and on the prime of the file, embody the import for the polyfill.
import "core-js/precise/string/pad-end"; // remainder of code
Now we will safely take away the CDN import from the earlier part. Open the index.html
file and delete the script
tag for the polyfill.io
import.
Your code at this stage ought to appear to be this.
Navigate to the browser and ensure that the code nonetheless runs as we count on.
Conclusion
Now we have efficiently polyfilled the String.prototype.padEnd
function in three alternative ways. By now, you must have a very good understanding of polyfills and how one can work with them no matter your undertaking construction.
Polyfills usually are not one thing you often work with straight, because of the myriad construct instruments obtainable that deal with transpiling code mechanically. Nevertheless, it’s all the time good to have an understanding of what these robots are doing behind the scenes. In spite of everything, it’s solely a matter of time earlier than they steal our jobs.
Should you adopted the tutorial throughout, you’re superior .
LogRocket proactively surfaces and diagnoses a very powerful points in your React apps
1000’s of engineering and product groups use LogRocket to scale back the time it takes to know the foundation reason for technical and usefulness points of their React apps. With LogRocket, you’ll spend much less time on back-and-forth conversations with prospects and take away the limitless troubleshooting course of. LogRocket permits you to spend extra time constructing new issues and fewer time fixing bugs.
Proactively repair your React apps — strive LogRocket right this moment.