Plenty of developers assume that working with blockchain technologies requires programming languages created specifically for the blockchain, like Solidity, which helps you to create good contracts that run on Ethereum. However you’ll be able to bridge the hole between Web2 and Web3 utilizing languages you’re already comfy with.
On this submit, we’ll present builders the best way to construct Web3 purposes utilizing JavaScript. We’ll embody a tutorial that lets you construct the muse of any Web3 software you wish to construct.
For these of you acquainted with net purposes and their improvement, you most likely create apps with a entrance finish/again finish division. You may additionally have a database on the again finish. Your entrance finish—the shopper—often runs in a browser or as an app, so the applied sciences concerned embody HTML, JavaScript, and numerous frameworks and libraries to help these (amongst others). Your again finish lives on a distant server and offers knowledge to the shopper.
In a Web3 workflow, you continue to have a entrance finish/again finish division, besides that the again finish is the blockchain. As long as you’ve got libraries and APIs that may talk with the blockchain, you’ll be able to create apps that use an online or cellular entrance finish. For builders who function in a primarily Web2 world, this makes it very simple to construct Web3 apps utilizing Web2 expertise.
The one catch is that as a result of responses from the blockchain can take variable quantities of time, it’s greatest to request and obtain from blockchains utilizing asynchronous strategies. As we’ll see within the tutorial under, we have now a most well-liked methodology to try this in net interfaces: Guarantees.
The XRP Ledger (XRPL) is a decentralized, open-source blockchain designed to facilitate quick, low-cost worldwide funds. As an alternative of counting on advanced good contract logic, the XRPL is programmable within the sense that core builders can create native protocols. For builders with Web2 expertise, that is significantly advantageous as a result of the XRPL permits them to leverage a strong and environment friendly blockchain utilizing acquainted programming languages similar to JavaScript, Java, and Python. This implies they will construct and work together with the XRPL by means of accessible APIs while not having to study new, advanced applied sciences, making the transition to blockchain improvement easy and intuitive.
A vibrant group of builders, validators, and corporations, together with the XRPL Foundation, XRPL Labs, Ripple, and XRPL Commons, actively contribute to and develop enhancements for the XRPL. Protocols are purposefully designed and carried out as native options of the XRPL, with many providing help for actions like funds, buying and selling, escrow, and tokenization. So as to add a brand new one, an modification course of should happen whereby validators debate and check all assumptions, and solely vote it in as a dwell characteristic when they’re satisfied it would work as meant and never negatively affect the XRPL. Any new options or modifications to the blockchain require settlement from at the least 80% of validators. This ensures that the XRPL stays safe and dependable whereas constantly evolving, providing builders a secure and progressive platform.
The XRPL is powered by its native token XRP, which was purpose-built for funds and stands out as one of many few cryptocurrencies not categorized as a safety within the US, backed by regulatory readability in a number of different international locations.
The XRP Ledger’s decentralized nature is underscored by its community of over 600 nodes that course of transactions and keep the ledger. Its distinctive Proof-of-Affiliation (PoA) consensus protocol, operated by various entities together with universities, exchanges, and companies, enhances safety and belief within the community.
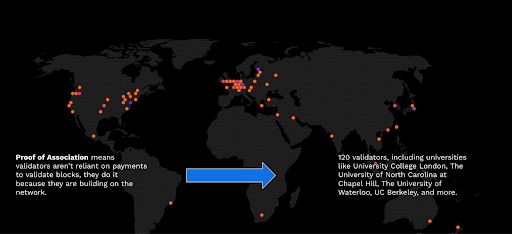
One of many standout options for builders is the XRPL’s native decentralized exchange (DEX) performance. The DEX contains a twin method to buying and selling by supporting each conventional order books and automatic market making. This built-in system permits customers to put a commerce, and the ledger robotically selects the tactic that gives the most effective worth for execution. The DEX additionally gives on-chain liquidity for quite a few foreign money pairs and contains superior options similar to auto-bridging and pathfinding, which optimize buying and selling effectivity. This built-in trade allows seamless asset transfers and liquidity entry, simplifying advanced monetary operations.
Furthermore, the XRPL is quick and sustainable, processing transactions each three to 5 seconds at fractions of a cent. This eco-friendly and cost-effective method helps mass market adoption, making it a gorgeous alternative for builders specializing in sustainability and scalability.
Since its inception in 2012, the XRPL has processed over 2.8 billion transactions, shifting over $1 trillion in worth with out experiencing any safety breaches or failures. This monitor file of reliability and efficiency offers a reliable platform for builders to check and build a Web3 business.
Lastly, the XRPL permits builders to entry full blockchain performance utilizing widespread programming languages. This eliminates the necessity to study and handle advanced good contracts, enabling Web2 builders to seamlessly transition to blockchain improvement and begin constructing purposes on the XRPL.
The best solution to present you the way this all works is to allow you to attempt it for your self. Under we’ve included a just-start for purposes you’ll be able to run by means of your browser. This reveals you the best way to:
- Import the xrpl.js library into a brand new undertaking.
- Connect with the ledger.
- Get an account.
- Request knowledge.
- Pay attention for occasions.
If you are studying and creating new purposes, you need to use the Testnet servers. These servers work the identical as our livenet ledger, however they don’t use actual XRP or foreign money. This tutorial makes use of Testnet—which is what most builders use when getting began—however when you develop one thing that you just’re able to set dwell, you’ll be able to change to the livenet servers simply.
This tutorial guides you thru the fundamentals of constructing an XRPL-connected software in JavaScript or TypeScript utilizing the xrpl.js shopper library. The scripts and config recordsdata used on this information can be found within the GitHub Repository. If utilizing Node.js, we advocate model 14, although variations 12 and 16 are additionally often examined.
The way you load xrpl.js into your undertaking relies on your improvement atmosphere:
For net browsers, add a `<script>` tag to your HTML:
<script src="https://unpkg.com/xrpl/construct/xrpl-latest-min.js"></script>
You’ll be able to load the library from a CDN, as within the above instance, or obtain a launch and host it by yourself web site. This hundreds the module into the highest degree as `xrpl`.
Fore Node.js, add the library utilizing npm. This updates your `bundle.json` file or creates a brand new one if it did not exist already:
npm set up xrpl
Then import it:
const xrpl = require("xrpl")
Earlier than you may make queries and submit transactions, it’s essential to hook up with the XRPL. To do that with xrpl.js, create an occasion of the `Consumer` class and use the `join()` methodology.
Tip: Many community capabilities in xrpl.js use Promises to return values asynchronously. The code samples right here use the async/await pattern to attend for the precise results of the Guarantees.
// In browsers, use a <script> tag. In Node.js, uncomment the next line:
// const xrpl = require('xrpl')
// Wrap code in an async perform so we will use await
async perform foremost() {
// Outline the community shopper
const shopper = new xrpl.Consumer("wss://s.altnet.rippletest.web:51233")
await shopper.join()
// ... customized code goes right here
// Disconnect when achieved (If you happen to omit this, Node.js will not finish the method)
await shopper.disconnect()
}
foremost()
The earlier part reveals you the way to connect with the Testnet, which is likely one of the obtainable parallel networks. Whenever you’re prepared to maneuver to manufacturing, you may want to connect with the XRP Ledger Mainnet. You are able to do that in two methods:
const MY_SERVER = "ws://localhost:6006/"
const shopper = new xrpl.Consumer(MY_SERVER)
await shopper.join()
See the instance core server config file for extra details about default values.
const PUBLIC_SERVER = "wss://xrplcluster.com/"
const shopper = new xrpl.Consumer(PUBLIC_SERVER)
await shopper.join()
The xrpl.js library has a `Pockets` class for dealing with the keys and handle of an XRPL account. On Testnet, you’ll be able to fund a brand new account like this:
// Create a pockets and fund it with the Testnet faucet:
const fund_result = await shopper.fundWallet()
const test_wallet = fund_result.pockets
console.log(fund_result)
If you happen to solely wish to generate keys, create a brand new `Pockets` occasion like this:
const test_wallet = xrpl.Pockets.generate()
Or, if you have already got a seed encoded in base58, you may make a `Pockets` occasion from it like this:
const test_wallet = xrpl.Pockets.fromSeed("sn3nxiW7v8KXzPzAqzyHXbSSKNuN9") // Take a look at secret; do not use for actual
Use the `Consumer` occasion’s `request()` methodology to entry the XRPL’s WebSocket API::
// Get information from the ledger in regards to the handle we simply funded
const response = await shopper.request({
"command": "account_info",
"account": test_wallet.handle,
"ledger_index": "validated"
})
console.log(response)
You’ll be able to arrange handlers for numerous varieties of occasions in xrpl.js, similar to at any time when the XRP Ledger’s consensus process produces a brand new ledger version. To do this, first name the subscribe method to get the kind of occasions you need, then connect an occasion handler utilizing the `on(eventType, callback)` methodology of the shopper.
// Take heed to ledger shut occasions
shopper.request({
"command": "subscribe",
"streams": ["ledger"]
})
shopper.on("ledgerClosed", async (ledger) => {
console.log(`Ledger #${ledger.ledger_index} validated with ${ledger.txn_count} transactions!`)
})
You’ll be able to take a better have a look at a curated list of among the initiatives and purposes that leverage JavaScript. Considered one of these purposes is GemWallet, a non-custodial crypto pockets that opens the gateway to the XRPL whereas embracing the realm of decentralized finance and NFTs. GemWallet bridges know-how, safety, and consumer expertise to allow extra seamless engagement with crypto belongings and DeFi protocols. GemWallet is privacy-focused; it does not monitor any personally identifiable info, pockets addresses, or asset balances.
“Because the founding father of GemWallet.app, which is the most important net pockets on the XRPL, my journey into improvement began with a powerful basis in Web2 expertise, significantly in JavaScript and TypeScript. Transitioning these expertise to the Web3 house was each difficult and rewarding. One of many largest hurdles was understanding the nuances of blockchain know-how and the way it integrates with present net applied sciences underneath the hood. Nevertheless, the wealth of sources and group help obtainable made the training curve manageable. Constructing GemWallet.app has been an unbelievable expertise, and I discovered that having a stable grasp of JavaScript was invaluable in making a seamless consumer expertise whereas leveraging the distinctive capabilities of blockchain know-how.” —Florian Bouron, GemWallet Founder
Crossmark is one other instance: a browser extension pockets constructed for interacting with the XRP Ledger. Crossmark provides to an already various community of wallets and offers tooling for builders constructing decentralized purposes on sidechains. The pockets will present a simple solution to change between customized networks and can assist bridge communities and cross-chain options. The builders behind Crossmark have been longtime supporters of the XRPL, citing its velocity, effectivity, and decentralization. Crossmark is constructed on the XRPL for these distinctive traits. Crossmark will present a novel utility providing refined monetary operations, demanding safe and fast interactions.
XRPL Learning Portal is an interactive device that simplifies the training expertise for constructing on the XRPL. The XRPL Studying Portal gives a simple and interactive method for builders to get began of their Web3 journey. Whether or not you wish to begin by getting acquainted with the fundamentals of crypto and blockchain or leap proper into studying the best way to code on the XRPL, you’ll be able to create your studying path with this new platform.
As a part of the XRPL Studying Portal’s dedication to empowering builders to transition seamlessly from Web2 to Web3, we’re excited to spotlight a newly added course, “Blockchain for Business.” This complete course is designed to bridge your present Web2 information with sensible purposes on the XRPL, enabling you to construct sturdy blockchain options for your corporation.
- Intro to Blockchain: Perceive the essential rules and potential affect of blockchain know-how on companies. This module will lay the muse to your blockchain journey, making advanced ideas accessible and related.
- Introduction to DeFi and the XRPL DEX: Start your exploration of decentralized finance (DeFi) and XRPL’s personal decentralized trade. Find out how XRPL’s DEX operates and the way it can profit your corporation with environment friendly, clear monetary transactions.
- Exchanging Tokens on the DEX: Grasp the XRPL DEX’s superior options like pathfinding and auto-bridging to safe the most effective charges for token exchanges and trades. This phase ensures you’ll be able to leverage XRPL’s know-how for optimum transaction outcomes.
- Web3 Ethics: Discover the core rules of constructing moral crypto initiatives, specializing in transparency, fairness, accessibility, and balancing freedom with accountability. Be taught to create initiatives that aren’t solely revolutionary but in addition ethically sound.
- What are Stablecoins?: Perceive one among crypto’s strongest instruments: stablecoins. Find out how they work and the way they are often utilized by companies, merchants, and on a regular basis individuals for stability and effectivity in monetary operations.
This course is a superb alternative for Web2 builders to increase their talent set, leveraging acquainted programming languages and instruments to construct on the XRPL. By integrating your present information with blockchain know-how, you’ll be able to drive innovation and create worth within the rising Web3 panorama.
Able to discover how one can construct Web3 apps utilizing the languages you already know? Enroll within the XRPL Studying Portal’s “Blockchain for Business” course at the moment. Go to the XRPL Learning Portal to get began and comply with @RippleXDev on Twitter for the most recent updates and insights.