Find out how to incorporate decentralized storage into your workflow
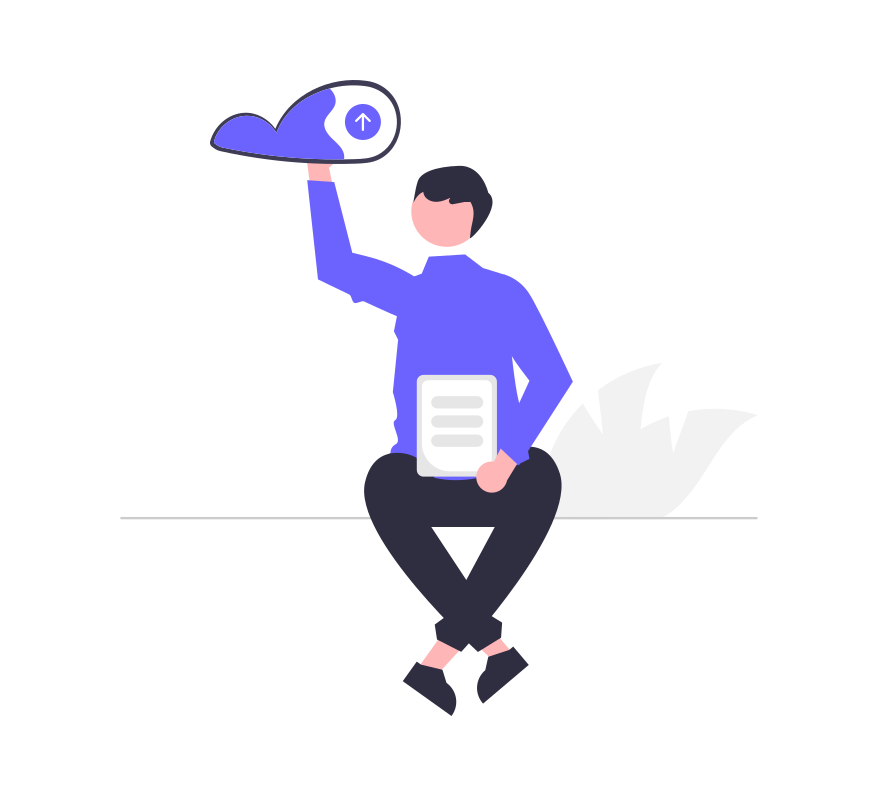
Rising applied sciences, such because the InterPlanetary FileSystem (IPFS), can contribute to an ecosystem that’s extra verifiable and open. Since IPFS depends on content material identifiers (CIDs) that are a hash of the content material, you may be assured that the returned information is appropriate. As well as, IPFS is an open and public community. So anyone can entry content material on the community if they’ve the right CID.
One undertaking I’m significantly enthusiastic about is web3.storage, which is a free service that reduces the friction of utilizing decentralized storage.

On this put up, I’ll …
- Present an introduction to web3.storage and methods to set it up
- Present examples of fundamental CRUD operations with IPFS in Python
- Describe my present resolution to create a decentralized information repository
Our intention at this time is to offer a user-friendly expertise that massively reduces the burden for onboarding new use circumstances into the web3 ecosystem at this time — whereas offering an improve path for the long run. — Web3.Storage
Web3.Storage permits customers and builders to make use of decentralized storage offered by IPFS and the Filecoin community. Something uploaded is duplicated throughout geographically distributed storage suppliers, making certain the resiliency of the community. The service additionally handles the work of pinning your content material throughout a number of servers.
Be conscious of what you add, since anyone can entry the content material on the community. Nevertheless, you may encrypt content material earlier than importing. My rule of thumb is, solely add content material you might be snug with being completely public.
The everlasting half is necessary. Not like location-based addressing (e.g. URLs), content-based addressing (e.g. CIDs) makes it difficult to take away information from the community as soon as it has been uploaded since it may be pinned on a number of servers.
Your quota is initially capped at 1TiB however may be elevated free of charge by submitting a request. The overhead price is at the moment sponsored by Protocol Labs, this may seemingly swap to some type of crypto-native fee mannequin within the close to future (e.g. staking Filecoin to extend storage limits).
The spine that holds all the pieces collectively is IPFS, a hypermedia protocol designed to make the online extra resilient by addressing information by its content material as an alternative of by its location. To do that, IPFS makes use of CIDs as an alternative of URLs — which level to the server the information is hosted on.
There’s much more to web3.storage and I encourage you to discover the docs in case you are additionally excited by this undertaking — particularly in case you are a developer.
Establishing web3.storage
Go to https://web3.storage to create an account
See the documentation for detailed directions.
Create API Token
An API token is critical to make use of web3.storage from the command line
- Log into your web3.storage account
- Click on on Account on the prime after which Create API token
- Enter a descriptive title to your token and click on Create
- You possibly can click on Copy to repeat your new API token to your clipboard.
Don’t share your API key with anyone, it’s particular to your account. You must also make an observation of the Token subject someplace and securely retailer it.
Set up the w3 Command-Line Interface
The w3
command-line interface (CLI) is node-based instrument to make use of web3.storage from the terminal
On a Mac, you may simply set up node
by way of homebrew, that is additionally set up the node bundle supervisor (npm
).
brew set up node
Use npm
to put in the w3
command-line interface.
npm set up -g @web3-storage/w3
Run the next command to attach w3
to web3.storage.
w3 token
You can be prompted for the API token you beforehand created.
The next shows details about every of the obtainable instructions.
w3 --help
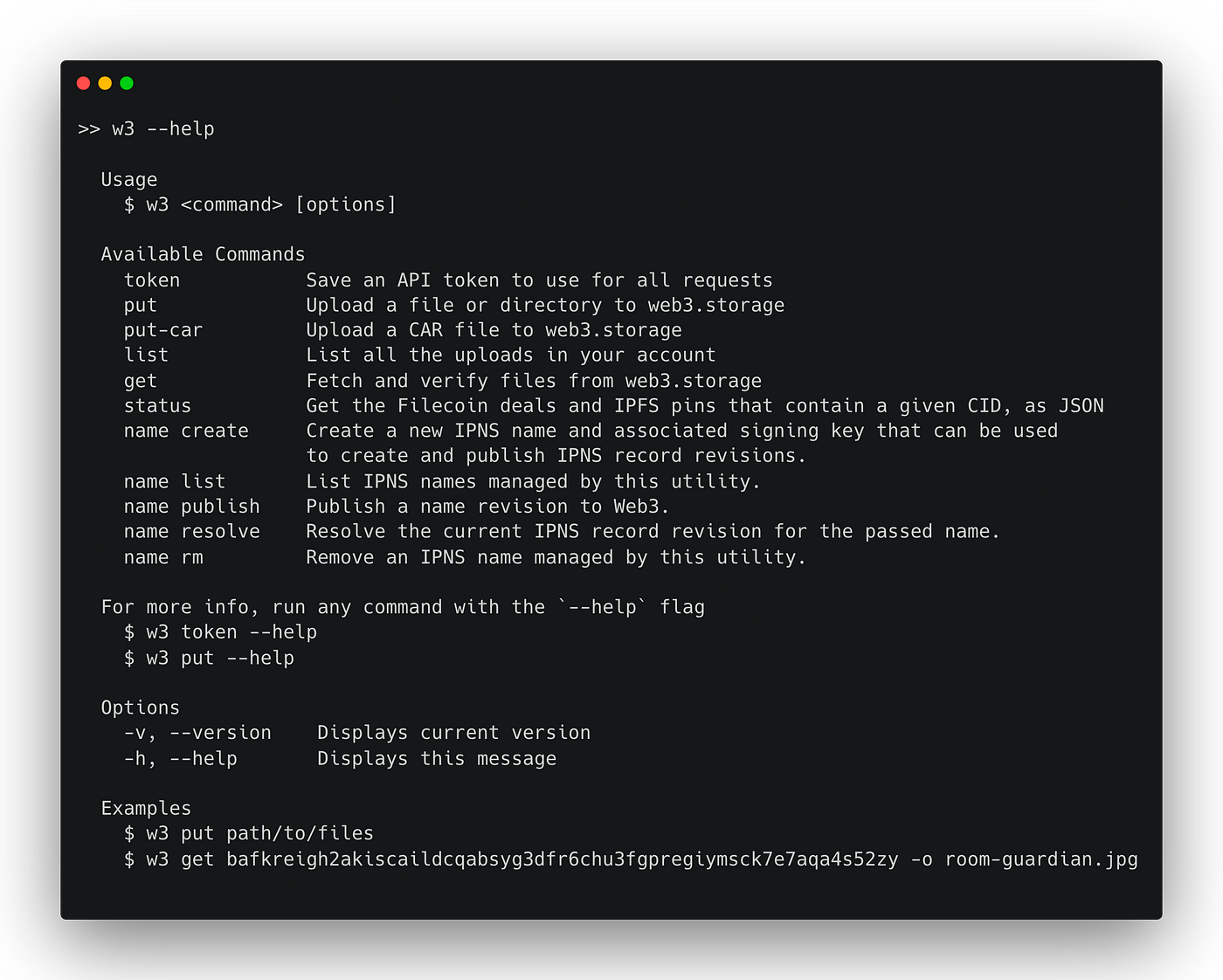
Add and Obtain Instructions
w3 put /path/to/file
(that is how we add content material to web3.storage)w3 get CID
(that is how we obtain content material from a selected CID)
Listing your recordsdata on web3.storage
w3 record
Instance utilizing put
First, create a textual content file with a message.
echo "Hi there web3.storage" > hi there.txt
Let’s now use the put
command to push the file to IPFS.
w3 put hi there.txt --name hi there
hi there
would be the title that seems in web3.storage, usew3 record
to confirm
The CID and a public gateway hyperlink are output.

In the event you adopted the steps above precisely, then your CID must be equivalent to mine. The CID is a hash that uniquely identifies the content material.
Use the hyperlink beneath to view the message via a public gateway.
https://dweb.hyperlink/ipfs/bafybeifzoby6p5khannw2wmdn3sbi3626zxatkzjhvqvp26bg7uo6gxxc4
Observe: the hyperlink might be totally different in case your message is totally different
Sooner or later, web3.storage will hopefully be S3 compliant, which means we are able to entry information saved there much like how we entry information in S3 buckets.
For now, we are able to use HTTP requests to learn the information into Python. Nevertheless, libraries like pandas
permit us to instantly learn CSV recordsdata from a gateway URL. Additionally, ipfsspec
permits us to learn zarr
information shops from IPFS with xarray
.
I’ll display studying every of those within the following sections
Studying JSON
Right here is an instance of studying a .json
file saved on IPFS.
Studying CSV recordsdata
When you have a CSV file, you may learn it instantly right into a pandas
DataFrame.
The Zarr format is new storage format which makes giant datasets simply accessible to distributed computing, making it an enchancment over the generally used NetCDF — a format for storing multidimensional information.
In the event you beginning to swap to zarr format, I encourage you try Pangeo’s “Information to making ready cloud-optimized information”.
NetCDF recordsdata are nonetheless quite common and xarray’s capabilities make it straightforward to transform these NetCDF recordsdata to zarr information shops.
import xarray as xr
ds = xr.open_dataset('/path/to/file.nc')
ds.to_zarr("./file.nc", consolidated=True)
consolidated=True
creates a “hidden” dotfile together with the information retailer that makes studying from zarr sooner with xarray
. In a easy check, I discovered studying consolidated information shops to be 5 instances sooner than unconsolidated.
zarr (consolidated): 635 ms
zarr (unconsolidated): 3.19 s
If you need to check out the code above, I uploaded NOAA Optimum Interpolation SST V2 dataset in consolidated and unconsoldiated zarr format to IPFS. This dataset offers weekly technique of ocean sea floor temperature (SST) from 1990 to the current with a 1 diploma spatial decision.
The gateway URL for this information is proven beneath
https://dweb.hyperlink/ipfs/bafybeicocwmfbct57lt62klus2adkoq5rlpb6dfpjt7en66vo6s2lf3qmq
Add Zarr information shops to IPFS
When importing zarr
recordsdata to IPFS you must be certain that to add the “hidden” dotfiles. With w3
this entails including the --hidden
flag:
w3 put ./* --hidden --name filename.zarr
Learn Zarr information shops from IPFS
With a view to learn zarr information shops from IPFS with xarray
you will want the ipfsspec
bundle (in addition to xarray
and zarr
)
conda set up -c conda-forge xarray zarr
pip set up ipfsspec
ipfsspec
ensures xarray
can interpret the IPFS protocol.
Discover within the instance beneath I’m utilizing the IPFS protocol as an alternative of the gateway URL with HTTPS. Nevertheless, behind the scenes the code is definitely studying from a gateway.
IPFS can be utilized as an information repository. Nevertheless, you must hyperlink the CID to one thing that’s human-readable to ensure that this to be a viable resolution. I’m not conscious of any greatest practices surrounding CID administration. Nevertheless, taking some cues from greatest practices for NFT information, my present method is to retailer CIDs and their related file title as a key:worth
pair in JSON after which use a NoSQL database, comparable to MongoDB, for queries.
After you add the content material to web3.storage you add a brand new report to the file that identifies what the dataset is and the CID of the content material. That is the minimal quantity of data wanted.
Right here is an instance of CIDs saved as a JSON array.
The pymongo
bundle makes it straightforward to work with MongoDB in Python.
Different method
An alternate method I’ve been contemplating, however haven’t applied but, is profiting from the w3 record
output.
This shows the CID and title you provided whenever you uploaded the content material.
The concept is to jot down an awk
script to generate a JSON file from the output.
Some downsides or pitfalls to this embody:
- Dealing with CIDs that time to directories as an alternative of pure recordsdata
- Ignoring CIDs which might be irrelevant to your database
The most important hurdle I can see is coping with directories. For that purpose alone I’m sticking with manually updating my database, particularly since it’s small and straightforward to handle — for now not less than.