WordPress has develop into essentially the most used content material administration system (CMS) due in no small half to its software programming interface (API). The WordPress REST API permits WordPress to “discuss” with different functions written in numerous languages — together with Python.
Python is an extensible programming language with various makes use of and a human-readable syntax, making it a strong device for remotely managing WordPress content material.
Listed here are some WordPress REST API use circumstances in your apps and the way you should utilize Python to assist them:
- Use predefined templates to allow your app to show uncooked knowledge into formatted posts with explanations rapidly.
- Construct a back-office software on Django and Python that shows limited-time presents to your clients each time an object-specific low cost or gross sales occasion happens.
- Combine Python scripts to run inside your WordPress website
This tutorial will show you how to create a easy Python console software that communicates with and executes operations on the WordPress REST API. The whole undertaking code can also be out there.
Putting in and Configuring WordPress
First, let’s set up and run a WordPress web site domestically in your improvement machine. This is a superb approach to begin with WordPress because you don’t should create an account or purchase a website identify for hosting.
Earlier than putting in WordPress domestically, some elements are required to run in your pc, together with the Apache net server, a neighborhood database, and the PHP language through which WordPress is written.
Luckily, we are able to use DevKinsta, a free native WordPress improvement suite out there for all main OSes (you don’t should be a Kinsta buyer to make use of it).
DevKinsta is offered for Home windows, Mac, and Linux, and installs WordPress plus all of its dependencies in your native machine.
Earlier than putting in DevKinsta, you have to have Docker operating domestically, so obtain and set up the Docker Engine in the event you haven’t but.
After putting in Docker Desktop, you may mechanically obtain the package deal that matches your OS.
Whenever you run the DevKinsta installer, Docker begins initializing instantly:
Subsequent, select New WordPress website from the Create new Website menu:
Now the DevKinsta installer requires you to create the credentials for the WordPress admin account:
As soon as put in, DevKinsta is a standalone software. Now you may entry each the WordPress website (by way of the Open Website button) and the WordPress admin dashboard (WP Admin button).
Subsequent, it’s worthwhile to allow SSL and HTTPS in your web site. This improves the safety of your web site by way of an SSL certificates.
Now go to the DevKinsta app and click on the Open website button. A brand new browser tab will present the house web page of your WordPress website:
That is your WordPress weblog, the place you can begin writing. However to allow Python to entry and use the WordPress REST API, we should first configure the WordPress Admin.
Now click on the WP Admin button on the DevKinsta app, then present your person and password to entry the WordPress Dashboard:
When you’re logged in, you’ll see WordPress Dashboard:
WordPress makes use of cookie authentication as its customary technique. However if you wish to management it utilizing the REST API, you have to authenticate with a method that grants entry to the WordPress REST API.
For this, you’ll use Utility Passwords. These are 24-character lengthy strings that WordPress generates and associates with a person profile that has permission to handle your web site.
To make use of Utility Passwords, click on the Plugin menu on the Dashboard, then seek for the plugin with the identical identify. Then set up and activate the Utility Passwords Plugin:
To start creating your software password, begin by increasing the Customers menu and clicking All Customers:
Now, click on Edit beneath your admin person identify:
Scroll down the Edit Person web page and discover the Utility Passwords part. Right here, present a reputation for the Utility Password, which you’ll use later to authenticate your Python app requests and devour the REST API:
Click on Add New Utility Password so WordPress can generate a random 24-character password for you:
Subsequent, copy this password and put it aside in a secure location to make use of later. Keep in mind, you gained’t have the ability to retrieve this password when you shut this web page.
Lastly, you have to configure permalinks. WordPress means that you can create a customized URL construction in your permalinks and archives. Let’s change it so {that a} WordPress put up titled, e.g., “Your First WordPress Web site” may be accessed by way of the intuitive URL https://your-website.native:port/your-first-wordpress-website/. This strategy brings a number of advantages, together with improved usability and aesthetics.
To configure permalinks, broaden the Settings part and click on the Permalinks menu. Right here, change the Frequent Settings to Put up identify:
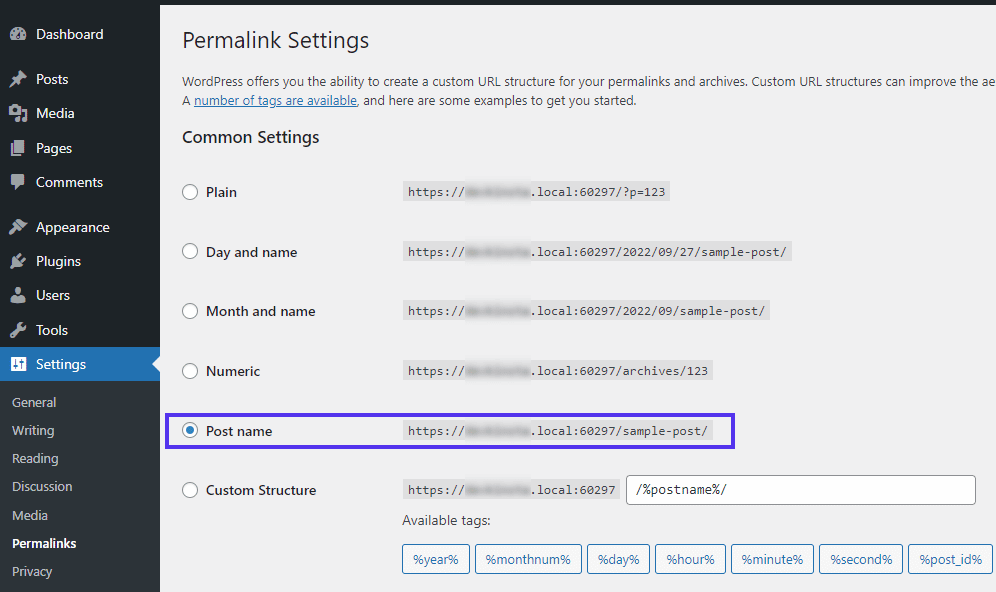
Setting the permalink construction utilizing the Put up identify construction can also be crucial as a result of it is going to permit us to retrieve posts later in our Python code utilizing the JSON format. In any other case, a JSON decoding error will likely be thrown.
How To Management WordPress From Python
WordPress is written in PHP, however it has a REST API that allows different programming languages, websites, and apps to devour its content material. Exposing the WordPress content material in REST structure makes it out there in JSON format. Subsequently, different companies can combine with WordPress and carry out create, learn, replace and delete (CRUD) operations with out requiring a neighborhood WordPress set up.
Subsequent, you’ll construct a easy Python app to see how you should utilize the WordPress REST API to create, retrieve, replace, and delete posts.
Create a brand new listing in your new easy Python undertaking and identify it one thing like PythonWordPress
:
../PythonWordPress
Now, you’ll create a digital setting in your undertaking, permitting it to keep up an impartial set of put in Python packages, isolating them out of your system directories and avoiding model conflicts. Create a digital setting by executing the venv
command:
python3 -m venv .venv
Now, run a command to activate the .venv digital setting. This command varies by OS:
- Home windows:
.venvScriptsactivate
- Mac/Linux:
.venv/bin/activate
Subsequent, retailer the configuration associated to your WordPress account. To separate the app configuration out of your Python code, create a .env file in your undertaking listing, and add these setting variables to the file:
WEBSITE_URL="<>"
API_USERNAME="<>"
API_PASSWORD="<>"
Luckily, studying the information above from a Python app is straightforward. You possibly can set up the Python-dotenv package deal so your software can learn configuration from the .env file:
pip set up python-dotenv
Then, set up aiohttp, an asynchronous HTTP shopper/server for Python:
pip set up aiohttp
Now add a file named app.py with the next code:
import asyncio
menu_options = {
1: 'Checklist Posts',
2: 'Retrieve a Put up'
}
def print_menu():
for key in menu_options.keys():
print (key, '--', menu_options[key] )
async def predominant():
whereas(True):
print_menu()
possibility = input_number('Enter your alternative: ')
#Examine what alternative was entered and act accordingly
if possibility == 1:
print('Itemizing posts...')
elif possibility == 2:
print('Retrieving a put up...')
else:
print('Invalid possibility. Please enter a quantity between 1 and 5.')
def input_number(immediate):
whereas True:
strive:
worth = int(enter(immediate))
besides ValueError:
print('Mistaken enter. Please enter a quantity ...')
proceed
if worth < 0:
print("Sorry, your response should not be adverse.")
else:
break
return worth
def input_text(immediate):
whereas True:
textual content = enter(immediate)
if len(textual content) == 0:
print("Textual content is required.")
proceed
else:
break
return textual content
if __name__=='__main__':
asyncio.run(predominant())
The code above shows a console menu and asks you to enter a quantity to decide on an possibility. Subsequent, you’ll broaden this undertaking and implement the code that allows you to record all posts and retrieve a particular put up utilizing its put up id.
Fetching Posts in Code
To work together with WordPress REST API, you have to create a brand new Python file. Create a file named wordpress_api_helper.py with the next content material:
import aiohttp
import base64
import os
import json
from dotenv import load_dotenv
load_dotenv()
person=os.getenv("API_USERNAME")
password=os.getenv("API_PASSWORD")
async def get_all_posts():
async with aiohttp.ClientSession(os.getenv("WEBSITE_URL")) as session:
async with session.get("/wp-json/wp/v2/posts") as response:
print("Standing:", response.standing)
textual content = await response.textual content()
wp_posts = json.hundreds(textual content)
sorted_wp_posts = sorted(wp_posts, key=lambda p: p['id'])
print("=====================================")
for wp_post in sorted_wp_posts:
print("id:", wp_post['id'])
print("title:", wp_post['title']['rendered'])
print("=====================================")
async def get_post(id):
async with aiohttp.ClientSession(os.getenv("WEBSITE_URL")) as session:
async with session.get(f"/wp-json/wp/v2/posts/{id}") as response:
print("Standing:", response.standing)
textual content = await response.textual content()
wp_post = json.hundreds(textual content)
print("=====================================")
print("Put up")
print(" id:", wp_post['id'])
print(" title:", wp_post['title']['rendered'])
print(" content material:", wp_post['content']['rendered'])
print("=====================================")
Discover the usage of the aiohttp library above. Fashionable languages present syntax and instruments that allow asynchronous programming. This will increase the appliance responsiveness by permitting this system to carry out duties alongside operations like net requests, database operations, and disk I/O. Python presents asyncio as a basis for its asynchronous programming framework, and the aiohttp library is constructed on high of asyncio to carry asynchronous entry to HTTP Consumer/Server operations made in Python.
The ClientSession
perform above runs asynchronously and returns a session
object, which our program makes use of to carry out an HTTP GET operation towards the /wp-json/wp/v2/posts
endpoint. The one distinction between a request to retrieve all posts and a request for a particular one is that this final request passes a put up id
parameter within the URL route: /wp-json/wp/v2/posts/{id}
.
Now, open the app.py file and add the import
assertion:
from wordpress_api_helper import get_all_posts, get_post
Subsequent, modify the predominant
perform to name the get_all_posts
and get_post
capabilities:
if possibility == 1:
print('Itemizing posts...')
await get_all_posts()
elif possibility == 2:
print('Retrieving a put up...')
id = input_number('Enter the put up id: ')
await get_post(id)
Then run the app:
python app.py
You’ll then see the appliance menu:
Now strive possibility 1 to view the record of posts that your Python app retrieves, and possibility 2 to pick a put up:
Creating Posts in Code
To create a WordPress put up in Python, start by opening the wordpress_api_helper.py file and add the create_post
perform:
async def create_post(title, content material):
async with aiohttp.ClientSession(os.getenv("WEBSITE_URL")) as session:
async with session.put up(
f"/wp-json/wp/v2/posts?content material={content material}&title={title}&standing=publish"
, auth=aiohttp.BasicAuth(person, password)) as response:
print("Standing:", response.standing)
textual content = await response.textual content()
wp_post = json.hundreds(textual content)
post_id = wp_post['id']
print(f'New put up created with id: {post_id}')
This code calls the put up
perform within the session
object, passing the auth
parameter beside the REST API endpoint URL. The auth
object now comprises the WordPress person and the password you created utilizing Utility Passwords. Now, open the app.py file and add code to import create_post
and the menu:
from wordpress_api_helper import get_all_posts, get_post, create_post
menu_options = {
1: 'Checklist Posts',
2: 'Retrieve a Put up',
3: 'Create a Put up'
}
Then add a 3rd menu possibility:
elif possibility == 3:
print('Making a put up...')
title = input_text('Enter the put up title: ')
content material = input_text('Enter the put up content material: ')
await create_post(title, f"{content material}")
Then, run the app and take a look at possibility 3, passing a title and content material to create a brand new put up in WordPress:
Selecting possibility 1 once more will return the id and the title of the newly added put up:
You too can open your WordPress web site to view the brand new put up:
Updating Posts in Code
Open the wordpress_api_helper.py file and add the update_post
perform:
async def update_post(id, title, content material):
async with aiohttp.ClientSession(os.getenv("WEBSITE_URL")) as session:
async with session.put up(
f"/wp-json/wp/v2/posts/{id}?content material={content material}&title={title}&standing=publish"
, auth=aiohttp.BasicAuth(person, password)) as response:
print("Standing:", response.standing)
textual content = await response.textual content()
wp_post = json.hundreds(textual content)
post_id = wp_post['id']
print(f'New put up created with id: {post_id}')
Then open the app.py file and add code to import update_post
and the menu:
from wordpress_api_helper import get_all_posts, get_post, create_post, update_post
menu_options = {
1: 'Checklist Posts',
2: 'Retrieve a Put up',
3: 'Create a Put up',
4: 'Replace a Put up'
}
Then, add a fourth menu possibility:
elif possibility == 4:
print('Updating a put up...')
id = input_number('Enter the put up id: ')
title = input_text('Enter the put up title: ')
content material = input_text('Enter the put up content material: ')
await update_post(id, title, f"{content material}")
Then run the app and take a look at possibility 4, passing a put up id, title, and content material to replace an present put up.
Selecting possibility 2 and passing the up to date put up id will return the main points of the newly added put up:
Deleting Posts in Code
You possibly can cross the put up id to the REST API to delete a put up.
Open the wordpress_api_helper.py file and add the delete_post
perform:
async def delete_post(id):
async with aiohttp.ClientSession(os.getenv("WEBSITE_URL")) as session:
async with session.delete(
f"/wp-json/wp/v2/posts/{id}"
, auth=aiohttp.BasicAuth(person, password)) as response:
print("Standing:", response.standing)
textual content = await response.textual content()
wp_post = json.hundreds(textual content)
post_id = wp_post['id']
print(f'Put up with id {post_id} deleted efficiently.')
Now open the app.py file and add code to import delete_post
and the menu:
from wordpress_api_helper import get_all_posts, get_post, create_post, update_post, delete_post
menu_options = {
1: 'Checklist Posts',
2: 'Retrieve a Put up',
3: 'Create a Put up',
4: 'Replace a Put up',
5: 'Delete a Put up',
}
Then, add a fifth menu possibility:
elif possibility == 5:
print('Deleting a put up...')
id = input_number('Enter the put up id: ')
await delete_post(id)
Now run the app and take a look at possibility 5, passing an id to delete the prevailing put up in WordPress:
Observe: The deleted put up should still seem in the event you run the Checklist Posts possibility:
To substantiate that you simply’ve deleted the put up, wait just a few seconds and take a look at the Checklist Posts possibility once more. And that’s it!
Abstract
Due to the WordPress REST API and Python’s HTTP shopper libraries, Python apps and WordPress can group up and discuss to one another. The good thing about the REST API is that it means that you can function WordPress remotely from a Python app, the place Python’s highly effective language permits automated content material creation that follows your required construction and frequency.
DevKinsta makes creating and creating a neighborhood WordPress website fast and straightforward. It gives a neighborhood setting for creating WordPress themes and plugins and presents a simplified deployment mannequin courtesy of its Docker-based, self-contained set up mannequin.
What’s your expertise working with Python and WordPress?
When able to broaden on that have, you may learn The Full Information to WordPress REST API Fundamentals to discover different potentialities.
Save time, prices and maximize website efficiency with:
- Instantaneous assist from WordPress internet hosting consultants, 24/7.
- Cloudflare Enterprise integration.
- International viewers attain with 35 knowledge facilities worldwide.
- Optimization with our built-in Utility Efficiency Monitoring.
All of that and rather more, in a single plan with no long-term contracts, assisted migrations, and a 30-day-money-back-guarantee. Try our plans or discuss to gross sales to seek out the plan that’s best for you.