On this article, we’re going to see the way to Cut up a File right into a Listing in Python.
After we need every line of the file to be listed at consecutive positions the place every line turns into a component within the file, the splitlines() or rstrip() methodology is used to separate a file into a listing. Let’s see a number of examples to see the way it’s accomplished.
The file is opened utilizing the open() methodology the place the primary argument is the file path and the second argument is a string(mode) which might be ‘r’ ,’w’ and so on.. which specifies if information is to be learn from the file or written into the file. Right here as we’re studying the file mode is ‘r’. the learn() methodology reads the information from the file which is saved within the variable file_data. splitlines() methodology splits the information into strains and returns a listing object. After printing out the checklist, the file is closed utilizing the shut() methodology.
Create a textual content file with the title “examplefile.txt” as proven within the beneath picture which is used as an enter.
Python3
|
Output:
['This is line 1,', 'This is line 2,', 'This is line 3,']
Instance 2: Utilizing the rstrip()
On this instance as an alternative of utilizing the splitlines() methodology rstrip() methodology is used. rstrip() methodology removes trailing characters. the trailing character given on this instance is ‘n’ which is the newline. for loop and strip() strategies are used to separate the file into a listing of strains. The file is closed on the finish.
Python3
|
Output:
[['This is line 1,'], ['This is line 2,'], ['This is line 3,']]
Instance 3: Utilizing break up()
We are able to use a for loop to iterate by means of the contents of the information file after opening it with Python’s ‘with’ assertion. After studying the information, the break up() methodology is used to separate the textual content into phrases. The break up() methodology by default separates textual content utilizing whitespace.
Python3
|
Output:
['This', 'is', 'line', '1,'] ['This', 'is', 'line', '2,'] ['This', 'is', 'line', '3,']
Instance 4: Splitting a textual content file with a generator
A generator in Python is a particular trick that can be utilized to generate an array. A generator, like a operate, returns an array one merchandise at a time. The yield key phrase is utilized by turbines. When Python encounters a yield assertion, it saves the operate’s state till the generator known as once more later. The yield key phrase ensures that the state of our whereas loop is saved between iterations. When coping with giant recordsdata, this may be helpful
Python3
|
Output:
['This', 'is', 'line', '1,'] ['This', 'is', 'line', '2,'] ['This', 'is', 'line', '3,']
Instance 5: Utilizing checklist comprehension
Python checklist comprehension is a good looking solution to work with lists. checklist comprehensions are highly effective and so they have shorter syntax. Moreover, checklist comprehension statements are typically simpler to learn.
To learn the textual content recordsdata in earlier examples, we had to make use of a for loop. Utilizing checklist comprehension, we will change ours for loop with a single line of code.
After acquiring the information by means of checklist comprehension, the break up() is used to separate the strains and append them to a brand new checklist. let’s see an instance to grasp.
Python3
|
Output:
['This is line 1,', 'This is line 2,', 'This is line 3,'] ['This', 'is', 'line', '1,'] ['This', 'is', 'line', '2,'] ['This', 'is', 'line', '3,']
Instance 6: Splitting a single textual content file into a number of textual content recordsdata
If we’ve got a big file and viewing all the information in a single file is troublesome, we will break up the information into a number of recordsdata. let’s see an instance the place we break up file information into two recordsdata.
Python checklist slicing can be utilized to separate a listing. To start, we learn the file with the readlines() methodology. The file’s first-half/higher half is then copied to a brand new file known as first half.txt. Inside this for loop, we’ll use checklist slicing to put in writing the primary half of the principle file to a brand new file.
A second loop is used to put in writing the opposite a part of the information right into a second file. The second half of the information is contained within the second half.txt. To carry out the slice, we have to use the len() methodology to find out the rely of strains in the principle file Lastly, the int() methodology is used to transform the division consequence to an integer worth
Python3
|
Output:
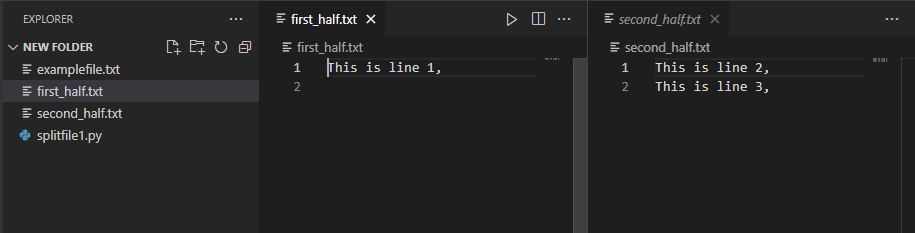