When including a function to file audio in your app, it’s often a good suggestion to indicate some kind of visible illustration to the person that audio is being recorded. For instance, you may add a timer to the UI that informs the person how lengthy the audio has been recording. However, if you happen to actually wish to give your customers a clear visible expertise, waveforms could possibly be a recreation changer.
On this article, we’ll learn to create easy waveforms if you’re both recording audio or enjoying an audio file in Flutter. Let’s get began!
Leap forward:
What are audio waveforms, and why do we’d like them?
Put merely, audio waveforms are graphs that signify how loud completely different elements of the audio are. The x-axis represents time, and the y-axis represents amplitude. So, the upper the waves, the louder the sound is. Equally, decrease waves or flatter waves signify softer areas within the audio.
Waveforms let the person know in the event that they’re talking softly or loudly in order that they’ll regulate their quantity accordingly. For instance, a person could possibly be attempting to talk softly, nevertheless it could possibly be too tender for the microphone to choose up. By trying on the graph, they might simply determine to elevate their quantity for the microphone.
Waveforms additionally turn out to be useful if you wish to play or stream an audio file. For instance, when medical doctors take heed to the sound relayed by a stethoscope, it might solely make the expertise higher to see a graph on the display displaying the sound patterns.
On this tutorial, we’ll construct a function like within the photographs beneath for a Flutter app:
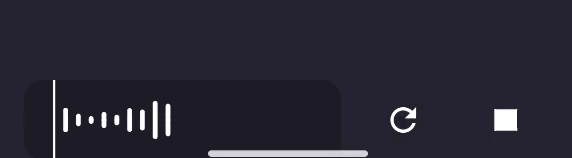
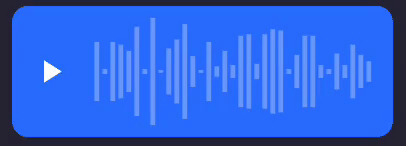
Organising our Flutter app
We’ll begin by including the required dependencies and permissions, together with the Audio Waveforms bundle:
flutter pub add audio_waveforms
Import the bundle in our predominant.dart
file:
import 'bundle:audio_waveforms/audio_waveforms.dart';
Now, add the permission to file to the Android manifest:
<uses-permission android:title="android.permission.RECORD_AUDIO" />
Creating the waveforms
The AudioWaveforms
widget will create our waveforms. However, earlier than we are able to proceed, we have to create a recorderController
that will likely be handed to our AudioWaveforms
widget. Let’s declare a RecordController
in our state with the next code:
late ultimate RecorderController recorderController;
Then, to initialize the controller, enter the instructions beneath:
void _initialiseController() { recorderController = RecorderController() ..androidEncoder = AndroidEncoder.aac ..androidOutputFormat = AndroidOutputFormat.mpeg4 ..iosEncoder = IosEncoder.kAudioFormatMPEG4AAC ..sampleRate = 16000; }
We are able to change the pattern fee and encoders in line with our wants. We’ll name this methodology in our initState
:
void initState() { tremendous.initState(); _initialiseController(); }
Now, utilizing the controller to file audio and show a waveform is so simple as including the AudioWaveform
widget to our widget tree as follows:
AudioWaveforms( measurement: Measurement(MediaQuery.of(context).measurement.width, 200.0), recorderController: recorderController, ), void _startRecording() async { await recorderController.file(path); // replace state right here to, for eample, change the button's state }
To begin the recording, we’ll name the strategy above on the press of a button:
IconButton( icon: Icon(Icons.mic), tooltip: 'Begin recording', onPressed: _startRecording )
We’ll cease the recording with the next code:
ultimate path = await recorderController.cease();
Stopping the recorder will return the trail of the file the place the recording is saved.
Customizing the waveforms
We already noticed that we’ve got management over the pattern fee and the encoding in RecordController
.AudioWaveforms
additionally permits us to vary the model of the waves, together with facets like measurement
, waveStyle
, colour
, padding
, and margin
:
AudioWaveforms( enableGesture: true, measurement: Measurement(MediaQuery.of(context).measurement.width / 2, 50), recorderCAudioWaveforms( enableGesture: true, measurement: Measurement(MediaQuery.of(context).measurement.width / 2, 50), recorderController: recorderController, waveStyle: const WaveStyle( waveColor: Colours.white, extendWaveform: true, showMiddleLine: false, ), ornament: BoxDecoration( borderRadius: BorderRadius.round(12.0), colour: const Shade(0xFF1E1B26), ), padding: const EdgeInsets.solely(left: 18), margin: const EdgeInsets.symmetric(horizontal: 15), )
We are able to additionally apply colour gradients to the waves:
waveStyle: WaveStyle( gradient: ui.Gradient.linear( const Offset(70, 50), Offset(MediaQuery.of(context).measurement.width / 2, 0), [Colors.red, Colors.green], ), )
Taking part in audio
Now, we’ll learn to play audio recordsdata and generate waveforms for them. The principle variations right here from the earlier instance for recording the audio are:
- We’ll create a
PlayerController
as an alternative of aRecordController
- We’ll use
AudioFileWaveforms
as an alternative ofAudioWaveforms
First, we’ll run the identical code as earlier than, changing RecordController
with PlayerController
:
late ultimate PlayerController playerController;
Nonetheless, this time, we’re making the next alteration:
void _initialiseController() { playerController = PlayerController(); }
Go this playerController
to the AudioFileWaveforms
widget in your widget tree:
AudioFileWaveforms( measurement: Measurement(MediaQuery.of(context).measurement.width, 100.0), playerController: playerController, )
We have to present the trail of the audio file to the controller. You’ll be able to seize the trail of the file any method you want. We’ll use path_provider
for this job. Add path_provider
to your pubspec.yaml
, then on the high of your predominant.dart
, add the code beneath:
import 'bundle:path_provider/path_provider.dart';
You can retailer the trail in a variable known as path
:
String? path; late Listing listing; void initState() { tremendous.initState(); _initialiseController(); listing = await getApplicationDocumentsDirectory(); path = "${listing.path}/test_audio.aac"; playerController.preparePlayer(path); }
Discover that we additionally name the preparePlayer
methodology on our controller by offering the trail to our audio file. We are able to begin and cease the participant the identical method we did with recordController
:
await playerController.startPlayer(); await playerController.stopPlayer(); void _playandPause() async { playerController.playerState == PlayerState.enjoying ? await playerController.pausePlayer() : await playerController.startPlayer(finishMode: FinishMode.loop); }
Now, we are able to name the _playandPause
methodology on a button click on. We additionally present a finishMode
to the startPlayer
methodology to loop the audio and the waveform when it ends. You can additionally add different choices to pause the waveform or cease it with FinishMode.pause
and FinishMode.cease
, respectively.
We are able to additionally add the flexibility to hunt the audio utilizing gestures on the waveform:
AudioFileWaveforms( enableSeekGesture: true, )
We’ll model our waveforms as follows:
AudioFileWaveforms( measurement: Measurement(MediaQuery.of(context).measurement.width / 2, 70), playerController: playerController, density: 1.5, playerWaveStyle: const PlayerWaveStyle( scaleFactor: 0.8, fixedWaveColor: Colours.white30, liveWaveColor: Colours.white, waveCap: StrokeCap.butt, ), )
Discover that we’re utilizing PlayerWaveStyle
to offer the kinds as an alternative of WaveStyle
, which is best fitted to recorders.
Disposing the controllers
Earlier than ending up, it’s necessary to eliminate the controllers that we used to file and play audio. Within the dispose
methodology, we’ll add the next code:
@override void dispose() { tremendous.dispose(); recorderController.dispose(); playerController.dispose(); }
Conclusion
We simply noticed how straightforward it’s to create an audio participant and show waveforms in Flutter utilizing the Audio Waveforms bundle. Waveforms are a dynamic visible illustration of the amount of sound; they supply suggestions to the person of how effectively the microphone is capturing their spoken phrases in order that they’ll enhance or lower their quantity accordingly.
We discovered find out how to add customizations to our waveforms in order that they go well with our visible preferences, begin and cease the recording, and eventually, eliminate the controllers for recording and enjoying audio. I hope you loved this tutorial; remember to go away a remark when you have any questions.
LogRocket: Full visibility into your net and cellular apps
LogRocket is a frontend software monitoring resolution that allows you to replay issues as in the event that they occurred in your individual browser. As a substitute of guessing why errors occur, or asking customers for screenshots and log dumps, LogRocket allows you to replay the session to rapidly perceive what went incorrect. It really works completely with any app, no matter framework, and has plugins to log further context from Redux, Vuex, and @ngrx/retailer.
Along with logging Redux actions and state, LogRocket information console logs, JavaScript errors, stacktraces, community requests/responses with headers + our bodies, browser metadata, and customized logs. It additionally devices the DOM to file the HTML and CSS on the web page, recreating pixel-perfect movies of even probably the most complicated single-page and cellular apps.