Angular is a frontend JavaScript framework developed by Google for constructing scalable enterprise-grade net purposes. A few of these purposes can get fairly massive, affecting the load time of your software.
To cut back load time and enhance the general expertise of your customers, you should use a method often known as lazy loading. This native Angular characteristic means that you can load solely the required bits of the net app first, then load different modules as wanted.
On this article, you’ll find out about lazy loading and the way it may also help velocity up your net app.
What’s Lazy Loading?
Lazy loading refers back to the strategy of loading webpage parts solely when they’re required. Its counterpart is keen loading, when every thing hundreds — or tries to load — instantly. Fetching all pictures, movies, CSS, and JavaScript code eagerly would possibly imply lengthy load instances — unhealthy information for customers.
Lazy loading is usually used for pictures and movies on websites that host loads of content material. As an alternative of loading all media without delay, which might use loads of bandwidth and lavatory down web page views, these parts are loaded when their location on the web page is about to scroll into view.
Angular is a single-page software framework that depends on JavaScript for a lot of its performance. Your app’s assortment of JavaScript can simply turn out to be massive because the app grows, and this comes with a corresponding enhance in knowledge use and cargo time. To hurry issues up, you should use lazy loading to first fetch required modules and defer the loading of different modules till they’re wanted.
Advantages of Lazy Loading in Angular
Lazy loading provides advantages that can make your website extra user-friendly. These embody:
- Sooner load time: JavaScript incorporates directions for displaying your web page and loading its knowledge. Due to this, it’s a render-blocking useful resource. This implies the browser has to attend to load the entire JavaScript earlier than rendering your web page. When lazy loading in Angular, the JavaScript is cut up into chunks which can be loaded individually. The preliminary chunk incorporates solely logic that’s wanted for the primary module of the web page. It’s loaded eagerly, then the remaining modules are loaded lazily. By lowering the scale of the preliminary chunk, you’ll make the location load and render sooner.
- Much less knowledge utilization: By splitting the information into chunks and loading as wanted, you would possibly use much less bandwidth.
- Conserved browser assets: For the reason that browser hundreds solely the chunks which can be wanted, it doesn’t waste reminiscence and CPU attempting to interpret and render code that isn’t required.
Implementing Lazy Loading in Angular
To comply with together with this tutorial, you’ll need the next:
- NodeJS put in
- Fundamental data of Angular
Step Up Your Mission
You’ll use the Angular CLI to create your challenge. You’ll be able to set up the CLI utilizing npm by working the command:
npm set up -g @angular/cli
After that, create a challenge named Lazy Loading Demo like this:
ng new lazy-loading-demo --routing
That command creates a brand new Angular challenge, full with routing. You’ll be working solely within the src/app
folder, which incorporates the code on your app. This folder incorporates your fundamental routing file, app-routing.module.ts
. The construction of the folder ought to appear to be this:
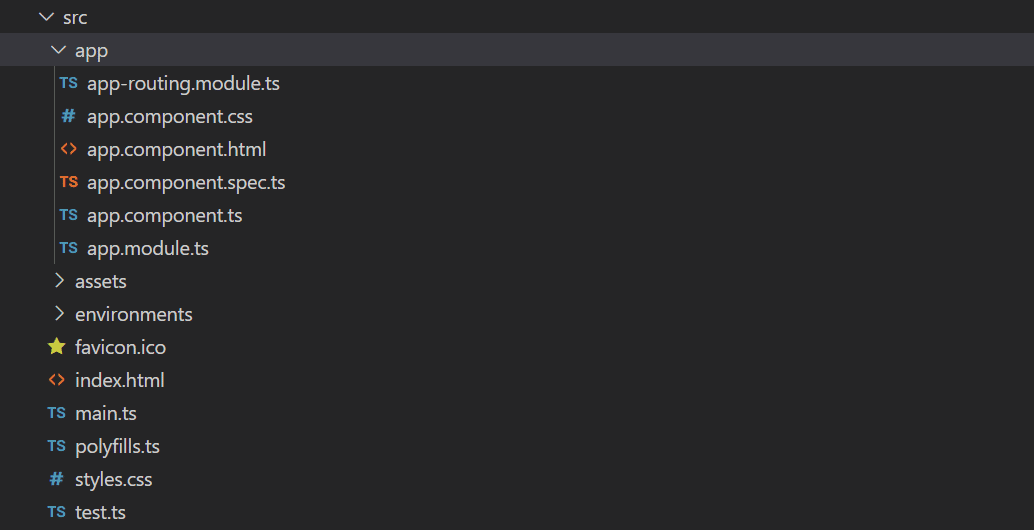
Create a Characteristic Module with Routes
Subsequent, you’ll create a characteristic module that can load lazily. To create this module, run this command:
ng generate module weblog --route weblog --module app.module
This command creates a module named BlogModule
, together with routing. Should you open src
/app/app-routing.module.ts
, you will note it now seems to be like this:
import { NgModule } from '@angular/core';
import { RouterModule, Routes } from '@angular/router';
const routes: Routes = [ { path: 'blog', loadChildren: () => import('./blog/blog.module').then(m => m.BlogModule) }];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
The half that’s vital for lazy loading is the third line:
const routes: Routes = [ { path: 'blog', loadChildren: () => import('./blog/blog.module').then(m => m.BlogModule) }];
That line defines the routes. The route for the weblog makes use of the loadChildren
argument as a substitute of element
. The loadChildren
argument tells Angular to lazy load the route — to dynamically import the module solely when the route is visited, after which return it to the router. The module defines its personal little one routes, similar to weblog/**
, in its routing.module.ts
file. The weblog module you generated seems to be like this:
import { NgModule } from '@angular/core';
import { RouterModule, Routes } from '@angular/router';
import { BlogComponent } from './weblog.element';
const routes: Routes = [{ path: '', component: BlogComponent }];
@NgModule({
imports: [RouterModule.forChild(routes)],
exports: [RouterModule]
})
export class BlogRoutingModule { }
You’ll notice that this routing file incorporates a single route, ''
. This resolves for /weblog
and factors to the BlogComponent. You’ll be able to add extra elements and outline these routes on this file.
For instance, if you happen to needed so as to add a element that may pull particulars a couple of explicit weblog publish, you might create the element with this command:
ng generate element weblog/element
That generates the element for the weblog element and provides it to the weblog module. So as to add a route for it, you may merely add it to your routes array:
const routes: Routes = [{ path: '', component: BlogComponent },
{path:"/:title",component: DetailComponent}];
This provides a route that resolves for weblog/:title
(for instance, weblog/angular-tutorial
). This array of routes is lazy-loaded and never included within the preliminary bundle.
Confirm Lazy Loading
You’ll be able to simply test that lazy loading is working by working ng serve
and observing the output. On the backside of your output, it is best to get one thing like this:
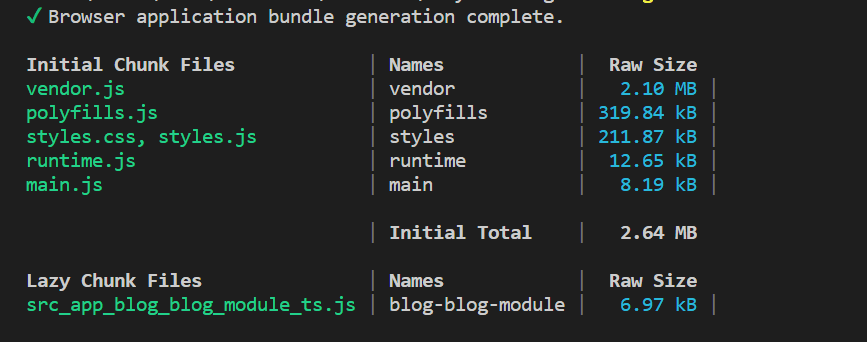
ng serve
.The output above is split into two components: Preliminary Chunk Information
are the information loaded when the web page first hundreds. Lazy Chunk Information
are lazy-loaded. The weblog module is listed on this instance.
Checking for Lazy Loading By means of Browser Community Logs
One other method to verify lazy loading is through the use of the Community tab in your browser’s Developer Instruments panel. (On Home windows, that’s F12 in Chrome and Microsoft Edge, and Ctrl–Shift–I in Firefox. On a Mac, that’s Command–Possibility–I in Chrome, Firefox and Safari.)
Choose the JS
filter to view solely JavaScript information loaded over the community. After the preliminary load of the app, it is best to get one thing like this:
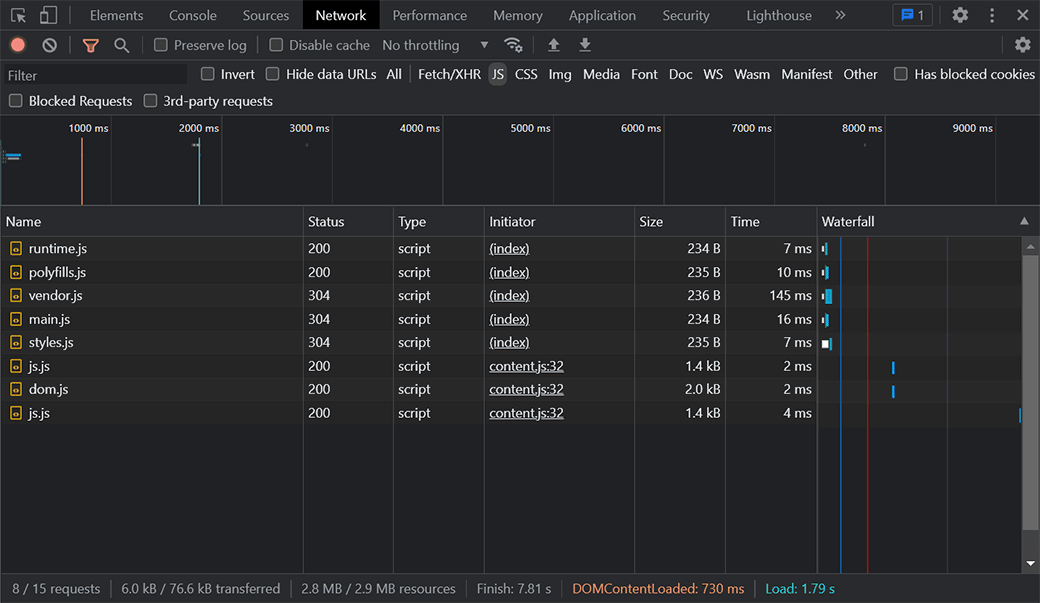
While you navigate to /weblog
, you’ll discover a brand new chunk, src_app_blog_blog_module_ts.js
, is loaded. This implies your module was requested solely whenever you navigated to that route, and it’s being lazily loaded. The community log ought to look one thing like this:
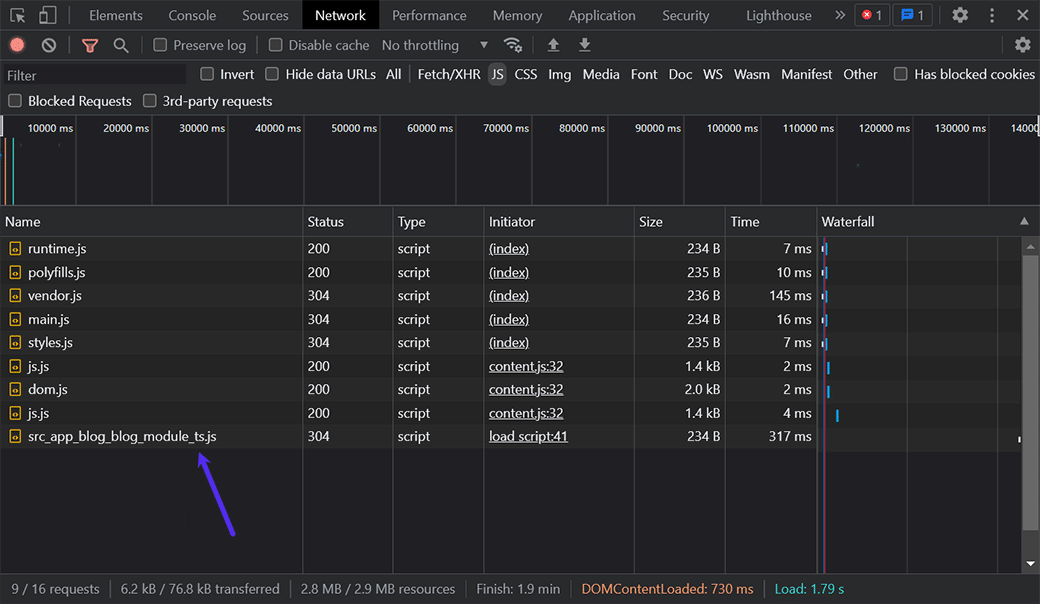
Lazy Loading vs Keen Loading
For comparability, let’s additionally create an eagerly loaded module and see the way it impacts the file dimension and cargo time. To display this, you’ll create a module for authentication. Such a module would possibly have to be loaded eagerly, as authentication is one thing you would possibly require all customers to do.
Generate an AuthModule by working this command within the CLI:
ng generate module auth --routing --module app.module
That generates the module and a routing file. It additionally provides the module to the app.module.ts
file. Nonetheless, not like the command we used to generate a module final time, this one doesn’t add a lazy-loaded route. It makes use of the --routing
parameter as a substitute of --route <identify>
. That provides the authentication module to the imports
array in app.module.ts
:
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
AppRoutingModule,
AuthModule //added auth module
],
suppliers: [],
bootstrap: [AppComponent]
})
Including AuthModule to your AppModule imports array means the authentication module is added to the preliminary chunk information and can be included with the primary JavaScript bundle. To confirm this, you may run ng serve
once more and observe the output:
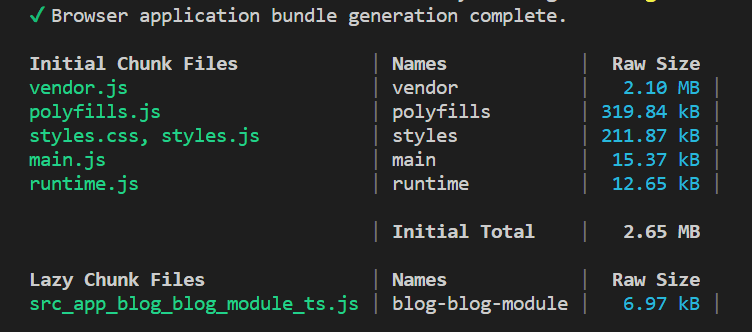
ng serve
command after authentication module is added.As you may see, the authentication module will not be included as a part of the lazy chunk information. Moreover, the scale of the preliminary bundle has elevated. The fundamental.js
file virtually doubled in dimension, growing from 8 KB to fifteen KB. On this instance, the rise is small, because the elements don’t comprise a lot code. However, as you fill the elements with logic, this file dimension will enhance, making a powerful case for lazy loading.
Abstract
You’ve discovered methods to use lazy loading in Angular to fetch modules solely when they’re required. Lazy loading is a good approach to enhance load instances, cut back knowledge utilization, and higher make the most of your frontend and backend assets.
Lazy loading, together with expertise like content material distribution networks and minifying JavaScript, will enhance each your web site’s efficiency and your customers’ satisfaction.
Should you’re growing a WordPress website and wish to actually crank up the velocity, examine Kinsta Edge Caching to see some spectacular numbers.
Get all of your purposes, databases and WordPress websites on-line and below one roof. Our feature-packed, high-performance cloud platform contains:
- Simple setup and administration within the MyKinsta dashboard
- 24/7 knowledgeable assist
- One of the best Google Cloud Platform {hardware} and community, powered by Kubernetes for optimum scalability
- An enterprise-level Cloudflare integration for velocity and safety
- International viewers attain with as much as 35 knowledge facilities and 275+ PoPs worldwide
Take a look at it your self with $20 off your first month of Utility Internet hosting or Database Internet hosting. Discover our plans or speak to gross sales to seek out your finest match.