What’s Queue?
A queue is a linear information construction that’s open at each ends and the operations are carried out in First In First Out (FIFO) order.
We outline a queue to be a listing during which all additions to the listing are made at one finish, and all deletions from the listing are made on the different finish. Â The component which is first pushed into the order, the operation is first carried out on that.
FIFO Precept of Queue:
- A Queue is sort of a line ready to buy tickets, the place the primary individual in line is the primary individual served. (i.e. First come first serve).
- Place of the entry in a queue able to be served, that’s, the primary entry that can be faraway from the queue, is named the entrance of the queue(typically, head of the queue), equally, the place of the final entry within the queue, that’s, the one most not too long ago added, is named the rear (or the tail) of the queue. See the beneath determine.
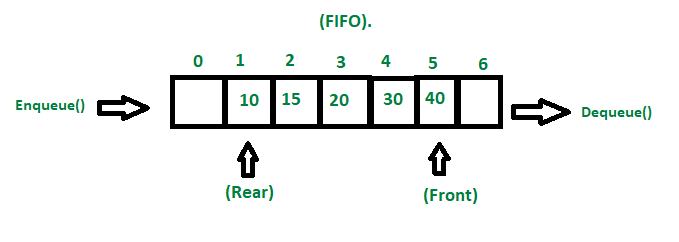
FIFO property of queue
Traits of Queue:
- Queue can deal with a number of information.
- We are able to entry each ends.
- They’re quick and versatile.Â
Queue Illustration:
Array Illustration:
Like stacks, Queues can be represented in an array: On this illustration, the Queue is applied utilizing the array. Variables used on this case are
- Queue: the identify of the array storing queue components.
- Entrance: the index the place the primary component is saved within the array representing the queue.
- Rear: the index the place the final component is saved in an array representing the queue.
Array illustration of queue:
C++
|
Linked Listing Illustration:
A queue can be represented in Linked-lists, Pointers, and Constructions.
Linked-list illustration of Queue:
C++
|
Varieties of Queue:
There are several types of queue:
Enter Restricted Queue
It is a easy queue. In such a queue, the enter could be taken from just one finish however deletion could be finished from any of the ends.
Output Restricted Queue
That is additionally a easy queue. In such a queue, the enter could be taken from each ends however deletion could be finished from just one finish.
Round Queue
It is a particular sort of queue the place the final place is related again to the primary place. Right here additionally the operations are carried out in FIFO order.
Double-Ended Queue (Deque)
In a double-ended queue the insertion and deletion operations, each could be carried out from each ends.
Precedence Queue
A precedence queue is a particular queue the place the weather are accessed based mostly on the precedence assigned to them.
To study extra about several types of queues, learn the article on “Varieties of Queues“.
Fundamental Operations of Queue:
There are few supporting operations (auxiliary operations):
entrance():Â
This operation returns the component on the entrance finish with out eradicating it.
C++
|
rear():Â
This operation returns the component on the rear finish with out eradicating it.
C++
|
isEmpty():Â
This operation returns a boolean worth that signifies whether or not the queue is empty or not.
C++
|
isFull():Â
This operation returns a boolean worth that signifies whether or not the queue is full or not.
C++
|
Enqueue():Â
Provides (or shops) a component to the top of the queue.
The next steps ought to be taken to enqueue (insert) information right into a queue:
- Step 1: Verify if the queue is full.
- Step 2: If the queue is full, return overflow error and exit.
- Step 3: If the queue shouldn’t be full, increment the rear pointer to level to the subsequent empty area.
- Step 4: Add the information component to the queue location, the place the rear is pointing.
- Step 5: return success.
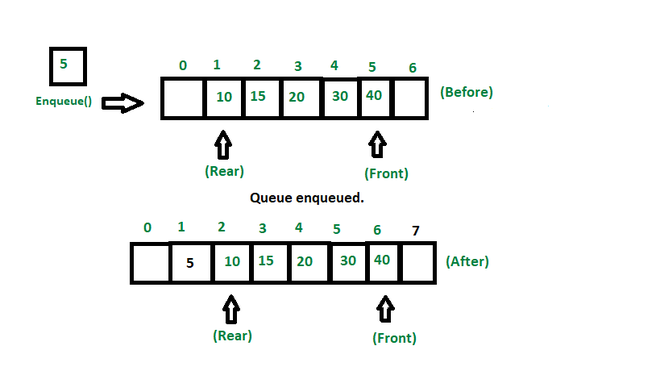
Enqueue illustration
Implementation of Enqueue:
C++
|
Dequeue():Â
Removes (or entry) the primary component from the queue.
The next steps are taken to carry out the dequeue operation:
- Step 1: Verify if the queue is empty.
- Step 2: If the queue is empty, return the underflow error and exit.
- Step 3: If the queue shouldn’t be empty, entry the information the place the entrance is pointing.
- Step 4: Increment the entrance pointer to level to the subsequent accessible information component.
- Step 5: The Return success.
Â
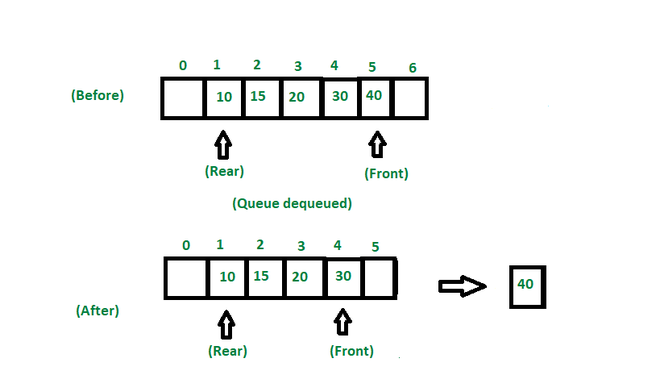
Dequeue operation
Implementation of dequeue:
C++
|
Implementation of queue:
Beneath is an implementation of all of the operations utilized in a queue.
C++
|
10 enqueued to queue 20 enqueued to queue 30 enqueued to queue 40 enqueued to queue 10 dequeued from queue Entrance merchandise is 20 Rear merchandise is 40
Time complexity: All of the operations have O(1) time complexity.
Auxiliary House: O(N)Â
Functions of Queue:
Software of queue is frequent. In a pc system, there could also be queues of duties ready for the printer, for entry to disk storage, and even in a time-sharing system, to be used of the CPU. Inside a single program, there could also be a number of requests to be saved in a queue, or one activity might create different duties, which have to be finished in flip by maintaining them in a queue.
- It has a single useful resource and a number of shoppers.
- It synchronizes between gradual and quick units.
- In a community, a queue is utilized in units resembling a router/change and mail queue.
- Variations: dequeue, precedence queue and double-ended precedence queue.
FAQs (Incessantly requested questions):
1. What information construction can be utilized to implement a precedence queue?
Precedence queues could be applied utilizing a wide range of information constructions, together with linked lists, arrays, binary search timber, and heaps. Precedence queues are greatest applied utilizing the heap information construction.
2. Queues are used for what objective?
Along with making your information persistent, queues scale back errors that happen when totally different components of your system are down.
3. In information constructions, what’s a double-ended queue?
In a double-ended queue, components could be inserted and eliminated at each ends.
4. What is healthier, a stack or a queue?
If you would like issues to return out within the order you set them in, use a queue. Stacks are helpful once you wish to reorder issues after placing them in.Â
Associated articles: