A search element serves as a consumer’s entry to an utility to simply lookup and entry information inside an app. Customers expertise the search element as an interface to speak with an utility.
It allows them to search out, kind, and filter varied elements of an utility’s content material by an enter subject the place they will specify search key phrases or/and a filter choice to filter search outcomes.
There are completely different approaches to including search performance to your net utility, reminiscent of creating the search element from scratch or utilizing search instruments to avoid wasting time and enhance accuracy, and productiveness.
On this weblog submit, you’ll be taught step-by-step learn how to implement a typo-friendly search element in your React app utilizing a easy API.
This text may also examine two serps (Algolia and Typesense) and take a look at their benefits over one another to provide you a base data on which ones is a greater possibility for search performance relying in your utility wants.
Prerequisites
This text assumes you might be aware of the next:
- React
- Utility Programming Interface (API)
- Primary CSS
Desk of Contents
What are the issues to think about when constructing a typo-friendly element in React?
Having a typo-friendly search element will present your customers with a greater consumer expertise whereas navigating by your utility. When making a search element, it’s important to notice what options it’s best to contemplate to ship an environment friendly search element and an general satisfying consumer expertise.
Autocomplete characteristic
This characteristic routinely gives predictions to finish a phrase as quickly because the consumer begins to kind the phrase. It saves time because it helps customers to shortly full their desired search question.
Autocorrect characteristic
This characteristic gives recommendations to appropriate typos, incorrect spellings, and capitalization. This relieves a consumer from needing to make sure if phrases are appropriate to the letter as they search by your utility.
Recommend associated outcomes characteristic
This characteristic gives customers with an inventory of steered phrases associated to the time period they’re looking out.
Demo application: Typosearch
This demo tutorial will stroll by learn how to construct a easy search utility utilizing ReactJS, and likewise learn how to add typo-friendly options reminiscent of correction recommendations and next-word prediction.
The applying consists of three main parts: the SearchBar.js
element, the Suggestion.js
element, and the general App.js
element that wraps all different parts.
If you kind in a key phrase, it does two issues:
- It tries to foretell the doubtless subsequent phrase, for instance, the phrase “separate” is probably succeeded by “from”
- It suggests some phrases whenever you occur to mistype a phrase. For instance, whenever you kind “poople”, it suggests “folks” as one of many steered choices to repair the typo.
These options might be carried out utilizing the free Datamuse API, which has 100,000 day by day API name limits.
Set up
To get began, clone the challenge from GitHub.
Set up dependencies by working the next command into your terminal:
npm set up
Your folder construction ought to appear like this:
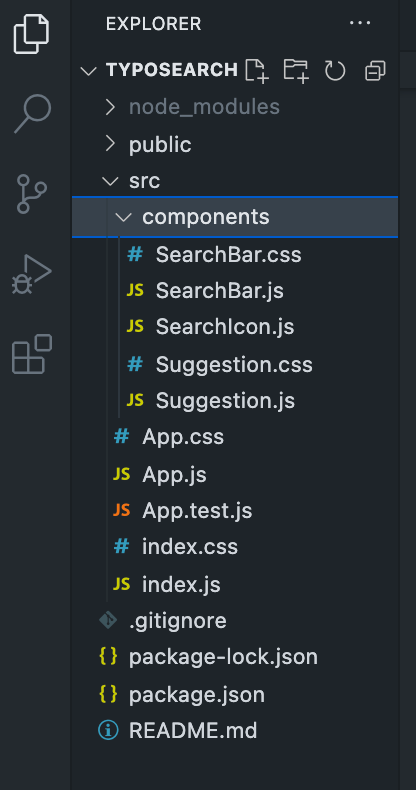
Run the next command to spin up a neighborhood server for previewing the applying:
npm run begin
The parts
There are three parts that make up this challenge and they’re:
- SearchBar Element: It is a easy search subject the place you kind in your search key phrase
import React from 'react'; import './SearchBar.css'; import SearchIcon from './SearchIcon'; perform SearchBar({ handleSearch, nextWord, searchValue, selectSug }) { const handleKeyDown = (e) => { if (e.key === 'Tab') selectSug(false, nextWord); } return ( <> { searchValue &&Hit the Tab key to just accept suggestion
}
{ searchValue && nextWord && {nextWord} } alert(searchValue)}>
</> ) } export default SearchBar;
- Suggestion Element: this element mainly accommodates three-word recommendations which can be meant for correcting your typo entered into the SearchBar element
import React from 'react'; import './Suggestion.css'; perform Suggestion({ correctionSugs, searchValue, selectSug }) { return ( <> { searchValue && correctionSugs.size > 1 && <div className="suggestion"> <h4 className="suggestion__header">Did you imply?</h4> { correctionSugs.map(merchandise => { return <p className="suggestion__sug" key={merchandise} onClick={() => selectSug(merchandise)}>{merchandise}</p> }) } </div> } </> ) } export default Suggestion;
- App: That is the house element that encapsulates different parts. It performs computations and passes props to parts the place crucial
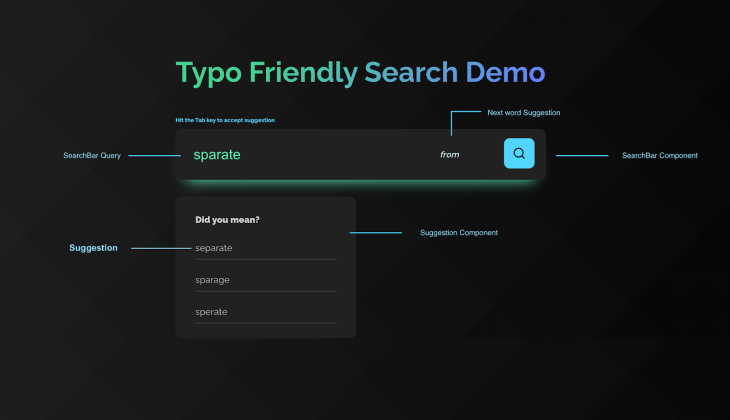
The way it works
If you kind into the enter subject, the applying makes two API calls. The primary name is for predicting the commonest subsequent phrase to your search key phrase. When this information is returned from the API, it’s saved in a state variable nextWordSug
and handed as a prop to the SearchBar.js
element the place it’s rendered. You’ll be able to hit the Tab button to routinely append the following phrase suggestion to your search key phrase.
attempt { const res = await fetch(`https://api.datamuse.com/phrases?lc=${phrase}`); const information = await res.json(); if (information.size > 1) setNextWordSug(information[0].phrase); } catch (err) { alert('Error occured whereas getting susggestions!'); }
The opposite is for suggestion corrections for a mistyped phrase. When the info is returned from the API, the primary three recommendations are extracted, saved within the correctionSugs
state variable, and handed as a prop to the Suggestion.js
element the place it’s rendered. You’ll be able to click on on any of the recommendations to just accept the suggestion — this replaces your typo with the accepted suggestion.
attempt { const res = await fetch(`https://api.datamuse.com/phrases?sp=${phrase}`); const information = await res.json(); let _data = []; for (let i = 0; i < 3; i++) { if (information.size >= 3) { if (information[0].phrase !== phrase.toLowerCase()) _data.push(information[i].phrase); } setCorrectionSugs(_data); } } catch (err) { alert('Error occured whereas getting susggestions!'); console.log(err); }
And that’s all there may be to it! You’ll be able to discuss with the supply code for in-depth evaluate.
Algolia vs. Typesense
Though you’ll be able to construct a search element in your React utility utilizing the strategy above, you too can leverage search instruments to shortly spin up a search element in your React utility.
This part of the article compares two environment friendly search instruments — Algolia and Typesense, that you may simply combine into your React utility to enhance the search expertise of your finish customers.
In contrast to making a customized search element your self, these search instruments are simpler to make use of, intuitive, and supply a number of out-of-the-box functionalities reminiscent of autocomplete and autocorrect, so that you just don’t should manually code these options your self.
What’s Algolia?
Algolia is a search engine providing that gives you with a set of constructing blocks for creating a quick, intuitive, and an general wonderful search expertise in your customers. It provides you auto-correct and full search functionalities that assist your customers discover solutions quicker.
It provides a developer-friendly API shopper, intuitive UX instruments and several other integrations that can assist you shortly create a search element in your utility. It gives a dashboard for builders to handle their search analytics and permits you additionally combine your personal analytics software to see how search.
World language assist
Algolia is language-agnostic, and it has assist for symbol-based languages together with Chinese language, Japanese, Arabic, and many others, with out requiring any extra work. Because of this it helps each left-to-right (LTR) and right-to-left (RTL) scripts.
Typo-tolerance
Typo-tolerance is a characteristic that tolerates customers’ misspellings and gives them with the merchandise they seek for. Algolia provides this by matching phrases which can be closest in spelling to the searched phrase.
What’s Typesense?
Typesense is a typo-tolerant search engine that’s constructed utilizing cutting-edge search algorithms, optimized with immediate search-as-you-type experiences.
It enhances the search expertise with autocomplete, filters, question recommendations, and options. In contrast to Algolia, Typesense is open-source, which means you’ll be able to host it your self, however they do supply a cloud-based resolution — Typesense Cloud.
No Runtime Dependencies
Typesense is a single binary that requires no runtime dependencies. This implies that you may run Typsense regionally or in manufacturing with a single command.
Easy to setup
Typesense is straightforward to arrange, handle, combine with, function, and scale.
Key variations and similarities
- Algolia provides personalization & server-based search analytics. Typesense however allows you to create your search on the client-side and ship search metrics to your most popular net analytics software
- Typesense is absolutely open supply, which means you’ll be able to both host it your self (at no cost) or use its managed SaaS providing — Typesense Cloud. Algolia is a proprietary closed supply
- Each Typesense and Algolia give you a dashboard which you should utilize to entry your information and index configuration, and likewise monitor your search analytics
- Typesense provides immediate search-as-you-type experiences for information units that may slot in RAM, as much as 24 TB, whereas Algolia provides immediate search-as-you-type experiences for datasets as much as 128 GB in dimension
- They each supply options like autocomplete, autocorrect, typo-tolerance, faceting, and extra
Conclusion
Search is a vital characteristic that’s helpful in nearly each trendy utility, particularly in eCommerce purposes, documentation, databases, and chat purposes.
Making a typo-friendly element in React will be fairly a troublesome activity, particularly if you need to construct the functionalities your self. Nonetheless, leveraging search engine providers like Algolia and Typesense can relieve you of the effort.
Selecting a greater search engine in your React app relies on your use case. Algolia is a good selection if value shouldn’t be an issue, Typesense however provides an open-source model that may be self-hosted at no cost.
Each Algolia and Typesense are categorized as search as a service instruments, with a number of out-of-the-box functionalities to enhance the general consumer expertise.
Full visibility into manufacturing React apps
Debugging React purposes will be troublesome, particularly when customers expertise points which can be laborious to breed. If you happen to’re excited about monitoring and monitoring Redux state, routinely surfacing JavaScript errors, and monitoring gradual community requests and element load time, attempt LogRocket.
LogRocket is sort of a DVR for net and cellular apps, recording actually the whole lot that occurs in your React app. As an alternative of guessing why issues occur, you’ll be able to combination and report on what state your utility was in when a difficulty occurred. LogRocket additionally displays your app’s efficiency, reporting with metrics like shopper CPU load, shopper reminiscence utilization, and extra.
The LogRocket Redux middleware package deal provides an additional layer of visibility into your consumer classes. LogRocket logs all actions and state out of your Redux shops.
Modernize the way you debug your React apps — begin monitoring at no cost.