As any developer can attest, code isn’t prepared for manufacturing after the primary draft. One key a part of the event course of is debugging — eradicating or altering all elements of your code that don’t work.
The Xdebug extension for PHP is a well-liked method to root out and destroy all the bugs in your code.
One of many nice facets of Xdebug is how versatile it’s. No matter your most popular framework or improvement setting, you’ll be capable to discover a model of Xdebug that slots into your workflow. From there, getting a deal with on the device received’t take lengthy.
This tutorial will take a look at Xdebug in depth, together with the set up course of, integrating it into your setup, and basic utilization.
First, let’s provide you with extra context on what Xdebug is and what it does.
Introducing Xdebug
Xdebug is likely one of the hottest extensions to debug your PHP code. You’ll set up it from inside your chosen setting, and it acts as a “step debugger.”

In brief, this lets you work in your code line by line so you possibly can step by and take a look at how the code acts and interacts inside your program, in addition to examine its output. From there, you can also make modifications as you see match.
Xdebug can do far more, although:
- You may analyze the efficiency of your code utilizing a set of metrics and visualizations.
- Once you run PHP unit checks, you possibly can see which suites of code you run and execute.
- Xdebug consists of “tracing” capabilities, which is able to write each perform name to disk. This can embrace arguments, variable assignments, and return values.
- Xdebug additionally makes enhancements to the usual PHP error reporting. We’ll cowl extra on this later.
Given the function set, there are many methods to make use of Xdebug (and any related debugger) inside your workflow. We’ll cowl these within the subsequent part.
Why You’d Need To Use Xdebug
Many builders received’t have a devoted debugging workflow that makes use of third-party instruments and extensions. It’s because PHP consists of its personal rudimentary error logging. You’ll use instructions resembling error_log
, var_dump
, and print to see the outcomes of variables and performance calls.
For instance, there are many snippets you possibly can repurpose for WordPress improvement — Stack Overflow is rife with them:
perform log_me($message) {
if ( WP_DEBUG === true ) {
if ( is_array($message) || is_object($message) ) {
error_log( print_r($message, true) );
} else {
error_log( $message );
}
}
}
Nevertheless, there are some vital drawbacks to this strategy:
- You first should make sure you allow error logs for the platform you’re working with. On this case, you’ll wish to allow
WP_DEBUG
(extra on this shortly). - This instance of “dump” debugging affords much less scope for investigation than step debugging. Right here, you possibly can solely output no matter you outline.
The latter level requires a lot handbook effort, particularly in case your day job isn’t as a sysadmin. For instance, if you wish to debug a code block, you would possibly add your snippet based mostly on a variable you outline. Nevertheless, it won’t be the supply of the issue and even point out what’s occurring.
As a substitute, a device resembling Xdebug can work its magic to offer larger scope:
- You may “break” your code at numerous factors throughout the execution to see what is going on in real-time.
- There are myriad metrics, visualizations, branches, and extra that will help you confirm what your code is doing and the way it responds.
- Generally, you possibly can even change values on the fly throughout the debugging course of. This affords immense worth, even for suites of code that work effectively. You may basically perform handbook unit checks at any level.
- Since you use breakpoints to mark up areas to debug, you don’t have to work with snippets inside your code. This retains your code cleaner and reduces the variety of future points.
Total, utilizing a device resembling Xdebug is a proactive determination slightly than a reactive one. You should utilize step debugging as a part of the core improvement course of, very similar to implementing unit checks as a part of test-driven improvement (TDD).
How To Flip On PHP Error Logging
Whilst you might debug your code with out a particular error, it’s typically good to know if a problem happens with out having Xdebug open. This provides you a start line for exploration. It’s not strictly mandatory, however generally is a useful a part of your chain.
To report each error that arises, you’ll want so as to add a line to the highest of the related PHP file:
error_reporting(E_ALL);
It is a catch-all command, and you may obtain the identical utilizing the ini_set
perform:
ini_set('error_reporting', E_ALL);
This allows you to change settings inside your php.ini file on a project-by-project foundation. Whilst you might go into this file and make a handbook change, it’s typically a greater thought to work with ini_set
to alter the precise parameter:
ini_set('display_errors', '1');
After you have lively error reporting set to your liking, you possibly can start working with Xdebug.
How To Use Xdebug
Over the subsequent few sections, we’ll present you how one can use Xdebug, together with the steps you’ll have to set issues up. Whereas we will’t cowl each device side, this quick-start information will get you going quick.
First, although, you might want to set up Xdebug. Let’s learn how to do it.
1. Set up Xdebug for Your Working System (OS)
As a result of Xdebug is adaptable to any variety of setups, the precise course of for each shall be barely totally different. On the OS degree, there are a number of variations:
- Home windows: It is a considerably sophisticated setup course of that includes utilizing an current PHP file and an set up wizard, then downloading the correct model on your system.
- Linux: The strategy right here is arguably probably the most simple: You should utilize a bundle supervisor to put in Xdebug, or the PHP Extension Group Library (PECL).
- Mac: This methodology can be easy: As soon as you put in PECL, you possibly can run
pecl set up xdebug
from a Terminal occasion. You’ll additionally have to have XCode command line instruments and PHP put in in your system.
Nevertheless, most customers received’t wish to persist with a system-level occasion of Xdebug. As a substitute, you’ll wish to combine it into your personal improvement setting.
2. Combine Xdebug Into Your Growth Atmosphere
As soon as you put in Xdebug on your OS, it’s best to join it to your setting.
There are such a lot of supported methods and instruments right here that we will’t go into all of them. Afterward, we’ll give you directions for each DevKinsta and PhpStorm. Even so, there are many different in style environments to select from. Under are a few of our high suggestions.
Various Vagrant Vagrants (VVV)
VVV is likely one of the named environments on the Make WordPress web site:
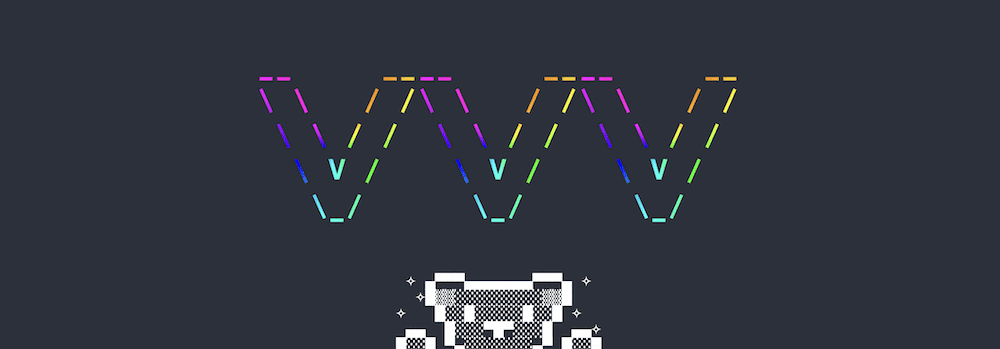
The excellent news is that VVV already features a model of Xdebug, however you might want to activate it. You are able to do this utilizing Safe Shell (SSH) inside a Terminal window:
vagrant ssh -c "switch_php_debugmod xdebug"
There’s slightly little bit of a efficiency hit, although, and also you’ll want to show this feature again on in case you provision your websites.
Laravel Valet
For some customers, Laravel’s Valet represents a near-perfect net improvement setting. Even higher, you possibly can combine Xdebug with it.
To do that, you’ll have to create a configuration file for the debugger. You will discover your personal path utilizing php --ini
on the command line, which is able to return a number of totally different file paths:
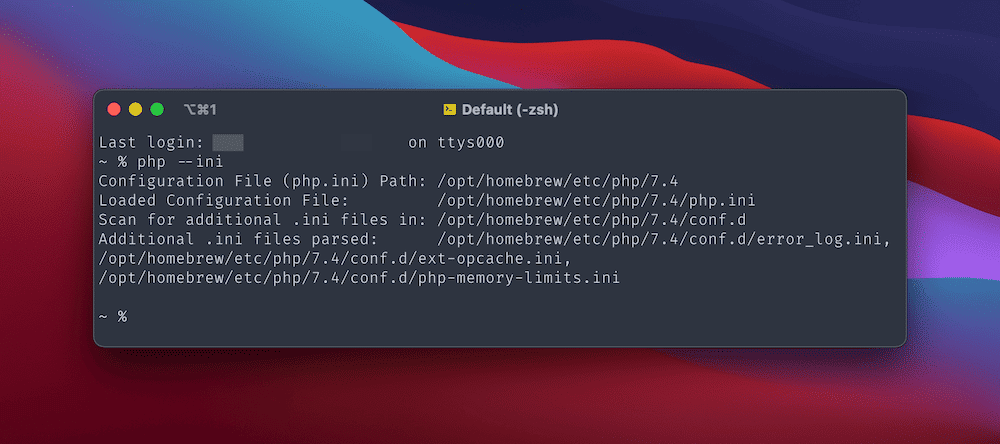
Subsequent, create a brand new xdebug.ini file on the path for added .ini information. In our instance, it’s at /choose/homebrew/and many others/php/7.4/conf.d.
When you open this new file, additionally open the trail to the Loaded Configuration File (your essential php.ini file). With each open, add the next to the underside:
- php.ini:
zend_extension="xdebug.so"
- xdebug.ini:
xdebug.mode=debug
When you’ve saved your modifications, run valet restart
from the Terminal, then add phpinfo(); exit;
to certainly one of your website’s information. You’ll wish to test whether or not this works by a fast web page load throughout the browser.
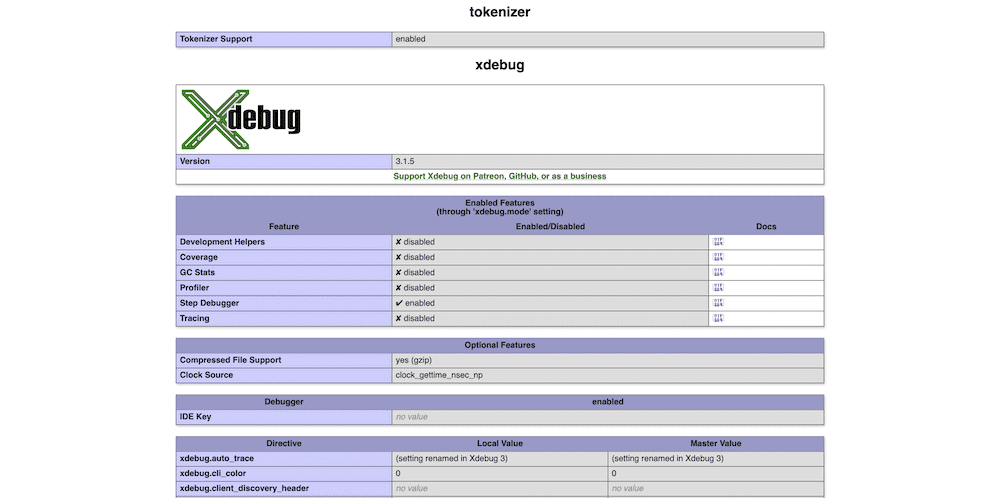
Word that you could be have to restart PHP utilizing sudo brew providers restart php
in addition to test that your system set up of Xdebug is appropriate utilizing php --info | grep xdebug
. You’ll discover the Xdebug-specific traces throughout the output:
From right here, you possibly can look to include Xdebug into your coding editor of alternative.
XAMPP
Very like Valet, there are a number of elements to the method for XAMPP. Nevertheless, Home windows and macOS variations have two totally different processes.
Start by putting in XAMPP, then run a fast test to see if the php_xdebug.dll file (Home windows) or xdebug.so file (macOS) exists in your system:
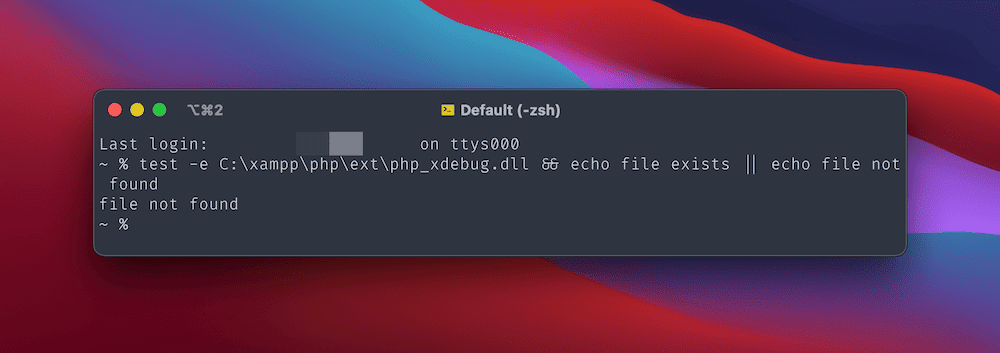
If the file exists, you possibly can transfer on to the configuration. In any other case, you’ll first have to obtain both the correct binary for Home windows — a 64-bit file on your most popular PHP model — or set up a number of extra dependencies in case you’re on a Mac.
For Home windows, rename the DLL file php_xdebug.dll, then transfer it to the xamppphpext file path. Subsequent, open the xamppphpphp.ini file in your most popular code editor and add the next:
output_buffering = Off
On the [XDebug]
part, add the subsequent three traces:
zend_extension=xdebug
xdebug.mode=debug
xdebug.start_with_request=set off
When you save your modifications, restart Apache and check for Xdebug.
For Mac, you’ll wish to make sure you set up the Xcode command line instruments utilizing xcode-select --install
at a Terminal occasion. After that, there are three packages you’ll wish to set up utilizing Homebrew:
brew set up autoconf automake libtool
In some instances, you’ll additionally have to reinstall XAMPP to get each the core program and the “Developer Recordsdata.” It’s best to be capable to re-install solely these information, however you’ll wish to perform a backup of your current setup first.
Subsequent, navigate to the obtain for the Xdebug supply folder in your system and unpack the TGZ file. Inside a Terminal window, navigate to that listing and run the next:
phpize
pecl set up xdebug
Word that you could be want to make use of sudo
right here too. From right here, you possibly can edit the XAMPP php.ini file. For many macOS installations, you’ll discover it at /Functions/XAMPP/xamppfiles/and many others/php.ini. Inside this listing, you’ll additionally discover the trail to your xdebug.so file — be aware this down and use it rather than the file path placeholder for this snippet:
[xdebug]
zend_extension=/path/to/xdebug.so
xdebug.mode=develop,degug
xdebug.start_with_request=sure
To check whether or not this works, create a brand new xdebug_info.php file inside the principle htdocs XAMPP listing. Inside, add the next:
<?php
xdebug_info();
…then refresh Apache and check Xdebug within the browser.
Utilizing PhpStorm With Xdebug
As soon as you put in Xdebug by the OS and your improvement setting, you’ll additionally have to view the debugger itself. You’ll do that by your chosen code editor or built-in improvement setting (IDE). As along with your setting, there are such a lot of to select from, and each may need a special strategy.
That stated, many builders choose to make use of JetBrains’ PhpStorm. In reality, PhpStorm affords “WordPress-aware help” — and it’s a well-liked alternative for a lot of different causes, too.
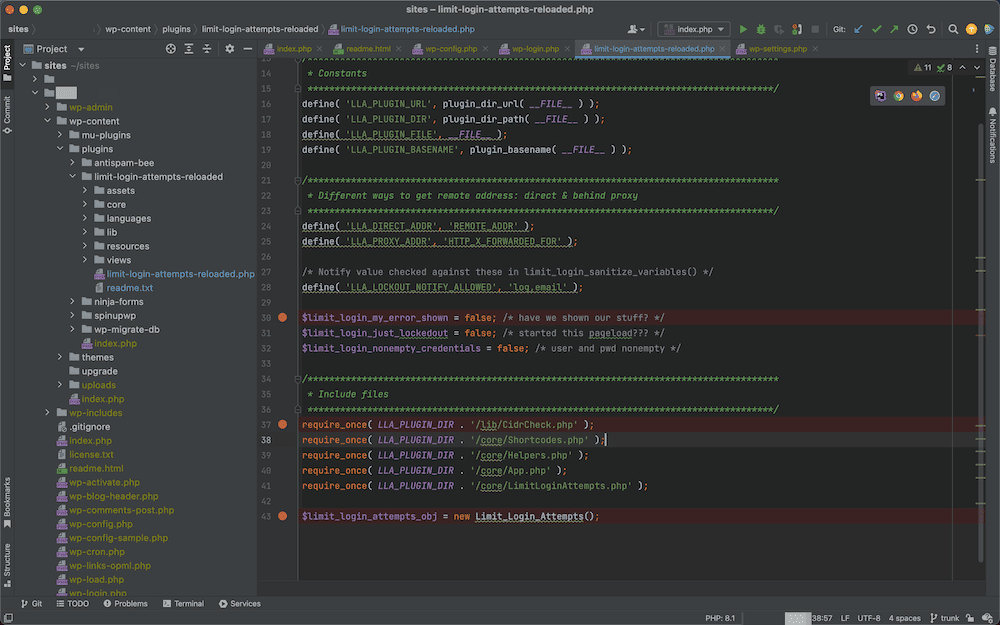
The JetBrains web site consists of full directions on connecting Xdebug and PhpStorm, however we’ll evaluate them right here.
First, navigate to the Languages & Frameworks > PHP web page throughout the Preferences pane. Right here, open up the Extra Gadgets kebab menu subsequent to the CLI Interpreter dropdown subject:
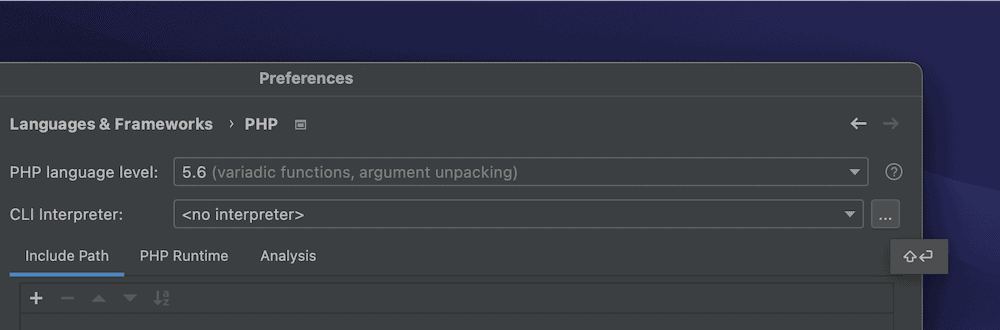
This can present some additional particulars about your PHP model and interpreter. If you happen to click on the Extra gadgets ellipsis subsequent to the Configuration file choice, you’ll see full paths on your php.ini file:
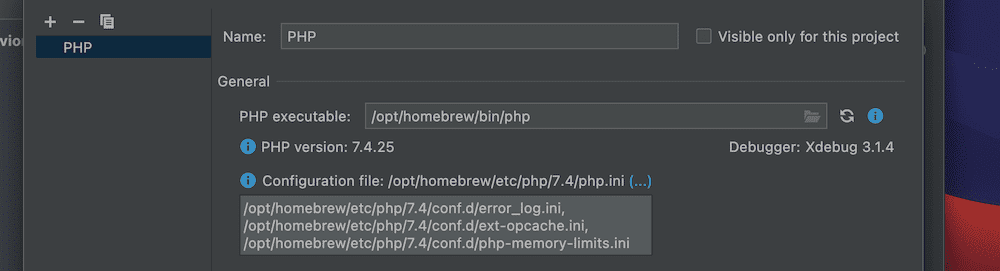
You’ll be working with this PHP file subsequent to proceed the setup course of.
Working Throughout the php.ini File
The primary activity right here is to edit out any traces that affect how Xdebug will work with PhpStorm.
Throughout the php.ini file, search for the next traces and both take away them or remark them out:
zend_extension=<path_to_zend_debugger>
zend_extension=<path_to_zend_optimizer>
These traces received’t be current in all instances, so don’t be alarmed in case you aren’t seeing them.
Subsequent, add the next to the file:
[xdebug]
zend_extension="xdebug.so"
xdebug.mode=debug
xdebug.client_host=127.0.0.1
xdebug.client_port="<the port (9003 by default) to which Xdebug connects>"
There are some things to notice about this suite of code:
- It’s possible you’ll have already got an
[xdebug]
part, through which case you possibly can omit the primary designation. - The
zend_extension
entry may have you so as to add the complete path of xdebug.so to attach. - Whereas it would seem like a placeholder, the
xdebug.client_port
parameter is the way you’ll set it inside your code.
When you add these, save and shut the file, then check the PHP model from the command line (utilizing php --version
):
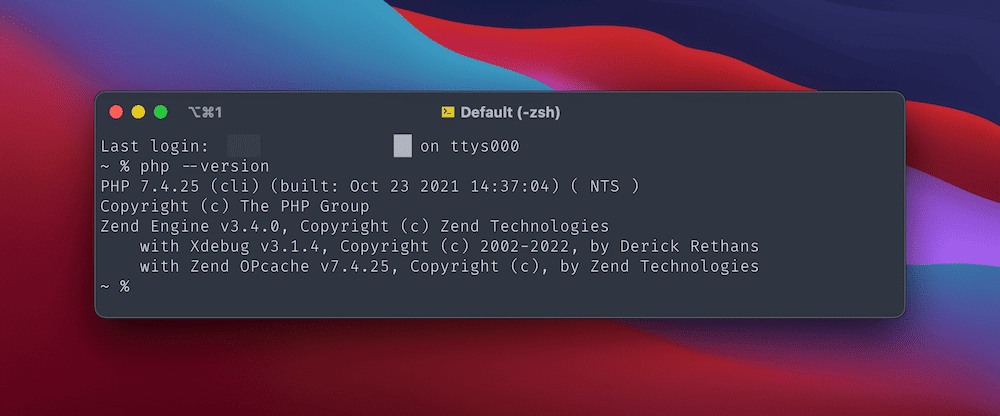
If in case you have a working model of Xdebug, it’ll present as one of many PHP extensions. You can even add phpinfo();
to a brand new file and check this within the browser.
That is nearly all you might want to do to have Xdebug work as your default debugger with PhpStorm. The ultimate step earlier than utilizing it’s putting in a browser helper extension.
Putting in a Browser Helper Extension
The ultimate key connection you’ll have to make is between your browser and PhpStorm, completed by activating step debugging on the server. Whilst you might do that from the command line utilizing particular GET
or POST
values, it’s extra simple to make use of an extension.
We suggest using the devoted Xdebug Helper extension. You may set up it in your browser of alternative:
If you wish to discover different extensions, the JetBrains web site affords a number of extra choices for the preferred browsers.
When you’ve put in your chosen browser extension, you shouldn’t have to regulate any additional configuration settings. From right here, you possibly can start to make use of Xdebug with PhpStorm.
Utilizing Xdebug
Whereas we’ll use PhpStorm right here, you’ll see the same format and interface between totally different IDEs — although there may also be some apparent variations.
There are a number of ideas that mix to type the entire debugging expertise:
- Breakpoints: These are the factors the place Xdebug will cease to allow you to examine the output. You’re in a position to set as many of those as you’d like.
- Listening for connections: You may toggle this on and off, although most builders will all the time go away it on.
- The debugging display screen: Nearly all of your time shall be spent throughout the debugging interface — it’s the place you’ll work with the assorted traces of code, variables, and parameters.
Step one is to activate listening — you received’t be capable to debug something with out it. To do that, click on on the Run > Begin Listening for PHP Debug Connections choice within the toolbar:
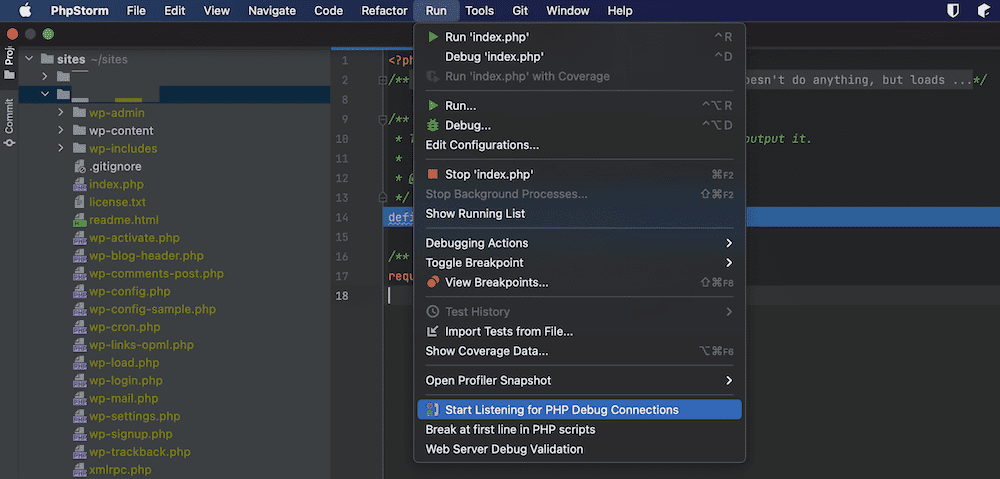
In its place, you possibly can click on on the “phone” icon inside PhpStorm’s toolbar:
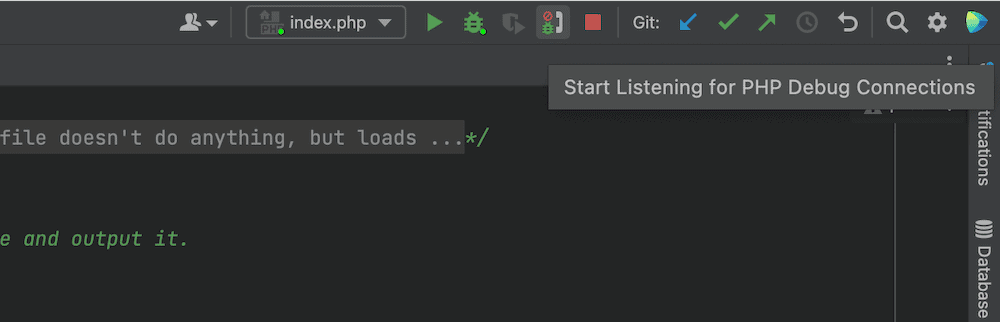
Both of those choices will begin the listening for connections.
From right here, you possibly can start to set breakpoints throughout the code editor’s gutters. A pink dot signifies a breakpoint, which you’ll click on to activate:
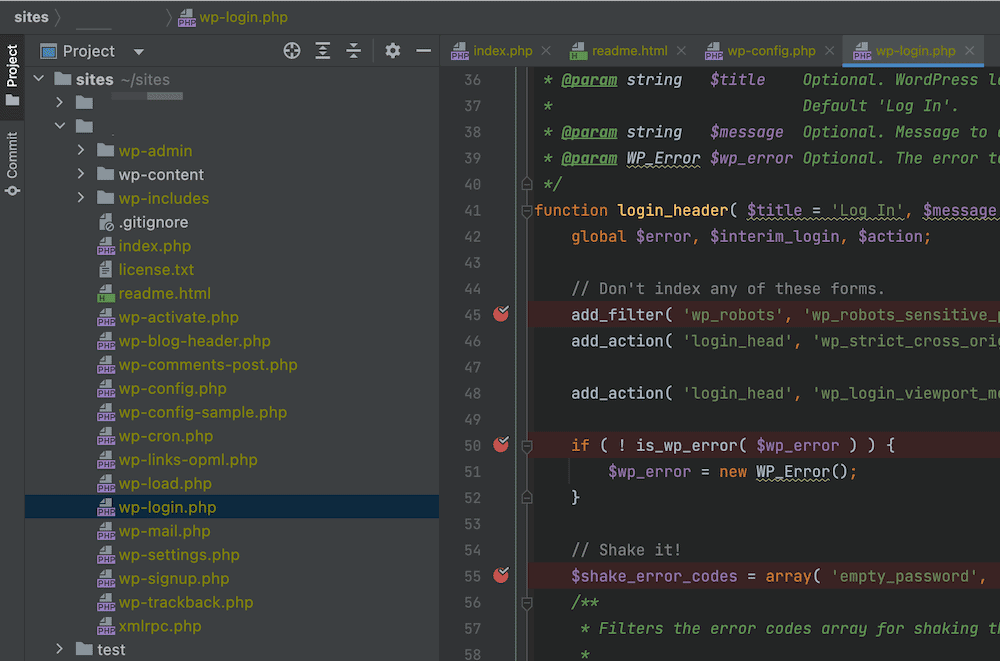
Once you wish to debug your code, probably the most simple method is to start listening, set breakpoints, then head to the precise web page in your browser. Find the icon on your extension throughout the browser, then click on on it and choose the “Debug” choice:
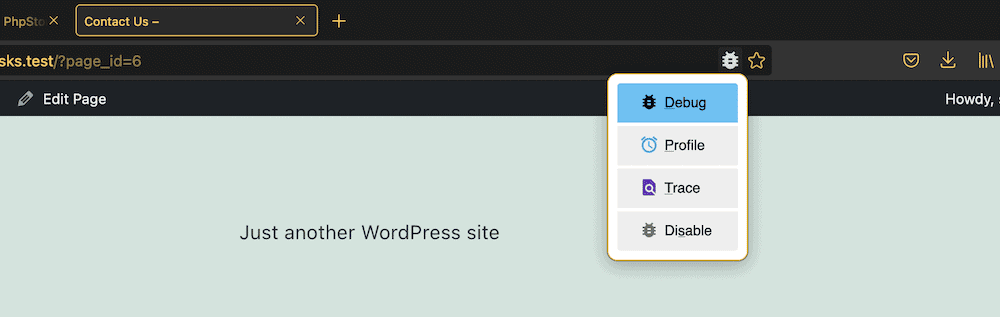
This can open the debugger in PhpStorm and ship both the great or unhealthy information:
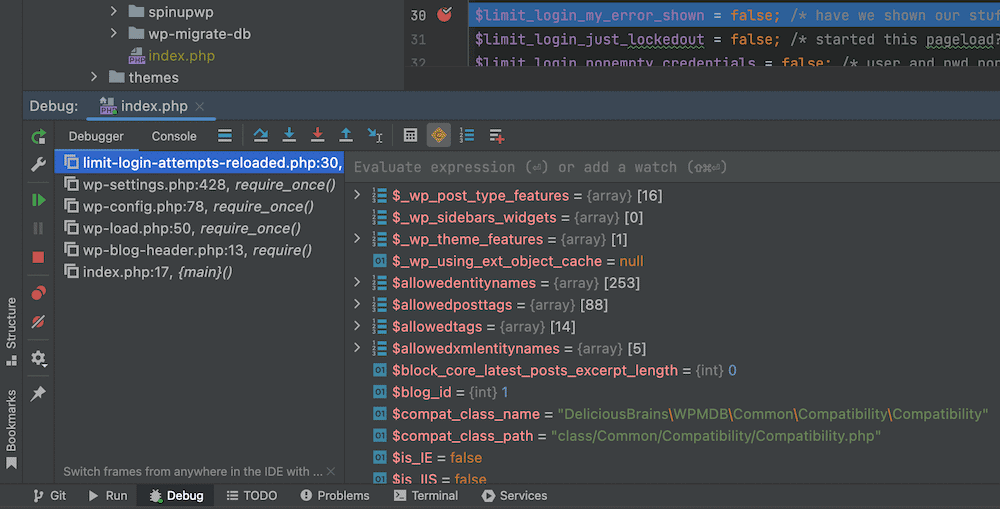
If you happen to right-click on the assorted values, attributes, parameters, and variables, you’ll be capable to entry an extra context menu. This provides you loads of additional scope to check and debug your code:
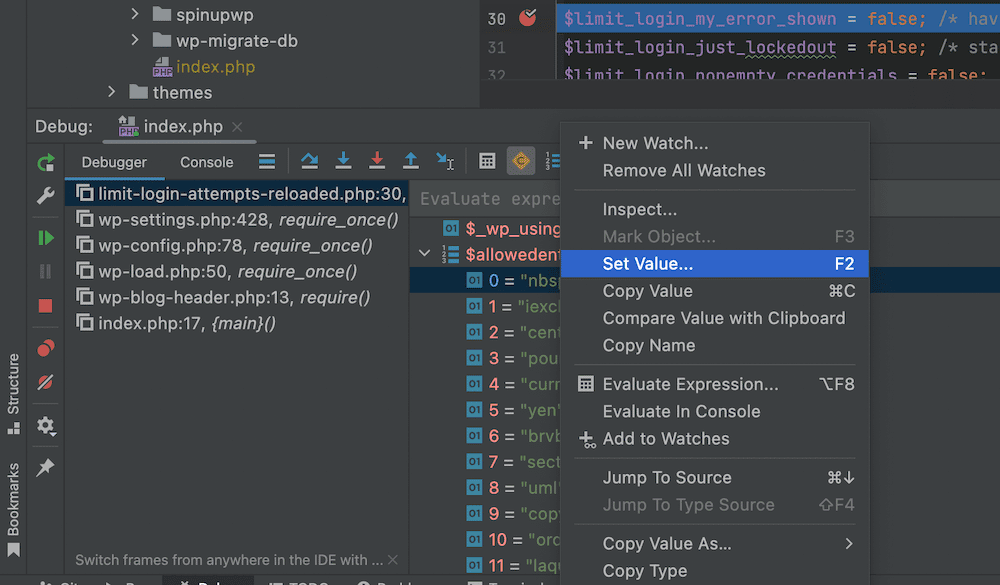
For instance, you would set totally different values for variables alongside the trail. This may be a deliberate try to interrupt your code and see what occurs, or it may very well be a method to check out code that already wants a repair. Both method, this offers you a implausible methodology for debugging your code with out having to change it first.
How Kinsta Helps You to Debug Your WordPress Web site
WordPress comes with its personal set of debugging choices by WP_DEBUG
and different instruments, resembling Question Monitor. These allow a mode through which you’ll begin to see beforehand hidden error messages throughout your website and dashboard. From there, you possibly can start to determine what the issue is.
You can even save these error messages utilizing WP_DEBUG_LOG
, which supplies you a method to doc the problems along with your website. We cowl how one can set this up in one other article on the weblog. It is a breeze to arrange by your MyKinsta dashboard (and the Websites > Instruments display screen):
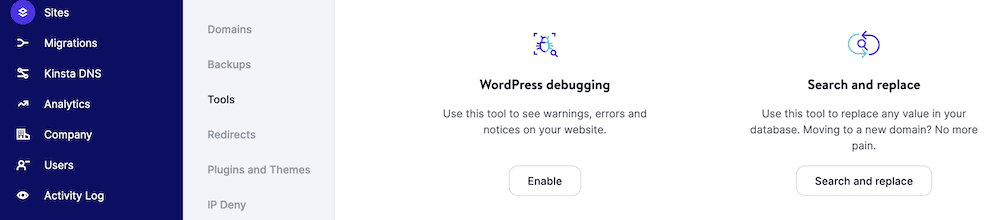
If you happen to pair this with the free DevKinsta native setting device, you’ll even have a one-click method to allow and disable WP_DEBUG
for every website you spin up:
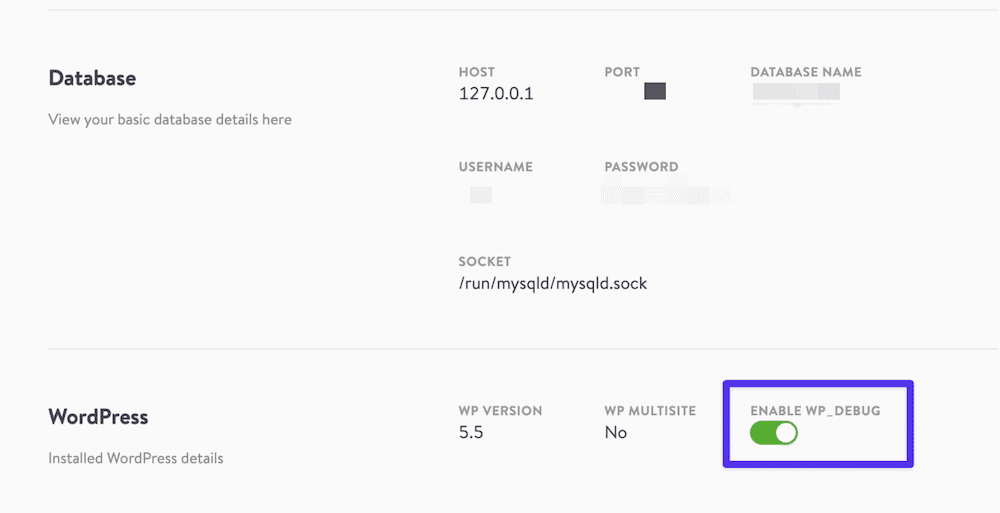
This implies you possibly can catch errors in your website throughout improvement, and ensure they don’t make it by to your dwell website. These modes are additionally simple to show off — important for each website and person safety.
All Kinsta plans additionally include the built-in Kinsta APM device, which is our custom-designed efficiency monitoring device for WordPress websites.
Command Cheat Sheet
Earlier than we wrap up this put up, we should always point out shortcuts.
Like many different software program items, there are numerous methods to navigate round Xdebug (and PhpStorm) utilizing the keyboard alone. In reality, you would even use the command line to debug PHP scripts.
As soon as Xdebug is up and working, you need to use the next instructions to get round:
Command | Shortcut |
---|---|
Particular the port to pay attention on (resembling [9003] ) |
-p [value] |
Units a breakpoint on the required line for the file path given. | breakpoint_set -t line file:///<path> -n <line> |
Runs your script till the tip, or the subsequent breakpoint | run |
Steps into the subsequent executable line | step_into |
Lists variables and values within the present scope | context_get |
Shows the worth of the required property | property_get -n <property> |
Whereas your particular code editor may have its personal devoted shortcuts, the main focus right here is on PhpStorm. Check out this desk of keyboard shortcuts for utilizing Xdebug with PhpStorm:
Command | Home windows | macOS |
---|---|---|
Discover Motion | Ctrl + Shift + A | Shift + Cmd + A |
Open the Debugger | Shift + F9 | Ctrl + D |
Toggle Breakpoint | Management + F8 | Cmd + F8 |
Step Into | F7 | F7 |
Step Over | F8 | F8 |
View Breakpoints | Ctrl + Shift + F8 | Shift + Cmd + F8 |
Resume the Program | F9 | F9 |
Consider the Present Expression | Alt + F8 | Choice + F8 |
Fortunately, there isn’t lots to memorize right here. You should open the debugger, set breakpoints per line, pay attention for connections, and run your scripts.
Nevertheless, in case you want a shortcut for a selected activity, you need to use the PhpStorm Discover Motion command:
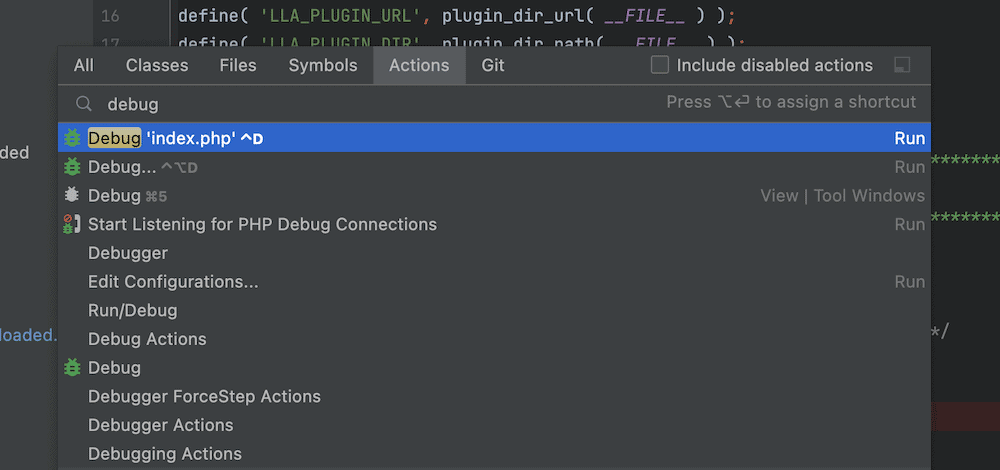
As soon as you start to kind on this house, you’ll be proven a dynamic listing of instructions and associated shortcuts. You can even discover a PDF model of all keyboard shortcuts by the Assist > Keyboard Shortcuts PDF menu.
In order for you extra of a real-time take a look at shortcuts as you’re employed with the mouse, JetBrains supplies the Key Promoter X plugin:
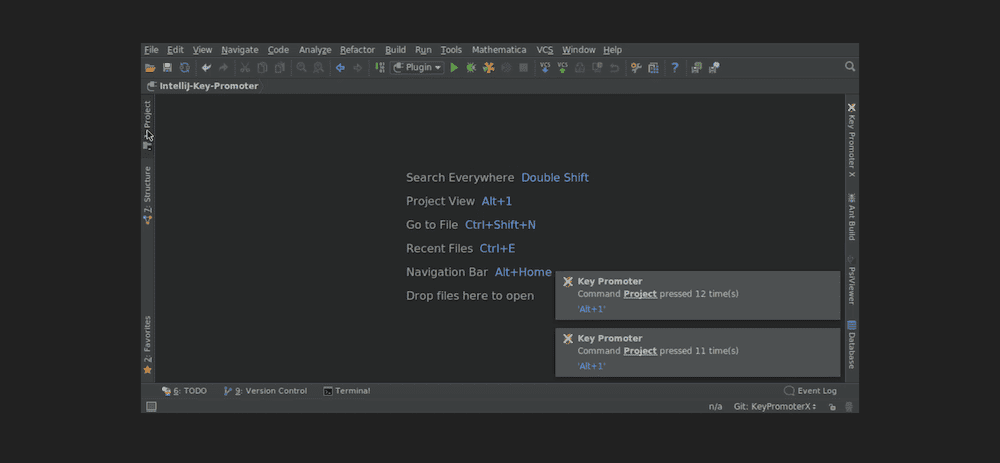
This helpful device will show notifications of your newest carried out motion, together with its associated keyboard shortcut. When you be taught and use the shortcuts, you possibly can part this plugin out and restore that precious actual property to your display screen.
Abstract
The apply of debugging has come a great distance from its humble beginnings; it now encompasses a a lot wider scope than its progenitors might have presumably imagined. To hold out a radical job in terms of fixing your PHP code, you’ll want to make use of a reliable device. There are various excellent extensions and instruments to select from, however Xdebug is an debatable frontrunner.
As we’ve seen, Xdebug can adapt to even probably the most eclectic of tastes in code editors, and it’s particularly nice when paired with PhpStorm. Nevertheless, no matter your setup, there’ll typically be a model of Xdebug to fit your wants. On the entire, it’s a robust, versatile, and intuitive device to make use of.
Do you suppose Xdebug deserves its excessive reward, or is there one other debugging device that you just want? Tell us within the feedback part under!
Save time, prices and maximize website efficiency with:
- Instantaneous assist from WordPress internet hosting specialists, 24/7.
- Cloudflare Enterprise integration.
- International viewers attain with 35 information facilities worldwide.
- Optimization with our built-in Software Efficiency Monitoring.
All of that and far more, in a single plan with no long-term contracts, assisted migrations, and a 30-day-money-back-guarantee. Try our plans or speak to gross sales to seek out the plan that’s best for you.