Textual content spotlight is a characteristic that may be detected on a number of websites and has a number of makes use of. For instance, browsers spotlight components discovered with the search perform, and textual content editors and instruments like Notion or Grammarly spotlight spelling and grammar errors.
Textual content spotlight can improve consumer expertise, however including this performance to an internet site or software will be tough. Luckily, that is about to get loads simpler due to the CSS Customized Spotlight API, which permits us to simply add fashion to a textual content vary. As of this writing, this characteristic remains to be in Working Draft, so it’s topic to vary.
On this article, I’ll present an summary of the API’s options and issues to contemplate concerning habits and accessibility. I’ll additionally share a number of examples as an instance using the CSS Customized Spotlight API in varied use circumstances.
Soar forward:
Understanding the fundamentals
Utilizing the CSS Customized Spotlight API requires three steps: creating the types within the stylesheet, setting a spotlight vary, after which making use of these types to the vary.
Earlier than diving into these steps, I need to reiterate that the CSS Customized Spotlight API remains to be a Working Draft, that means it’s nonetheless topic to adjustments. As well as, this characteristic doesn’t at present work on Firefox, so that you shouldn’t use it for manufacturing proper now if Firefox is critical in your venture.
With that mentioned, let’s begin with step one.
Creating the spotlight types
This CSS Customized Spotlight API requires us to make use of the ::spotlight()
pseudo-element to set the types we want. The W3C Working Draft defines this pseudo-element as “the portion of a doc that’s being contained or partially contained in all of the ranges of the registered customized spotlight”.
We’ll want so as to add a reputation for the customized spotlight we’re creating contained in the curly brackets, so a CSS declaration for a customized spotlight ought to look one thing like this:
::spotlight(sample-highlight) { background-color: rebeccapurple; shade: whitesmoke; }
You could be questioning if there’s a restriction within the fashion guidelines that you could apply for this spotlight, The reply is sure! The CSS guidelines we will use are fairly restricted. To be extra exact, we will solely use the next guidelines, as they’re relevant to the ::choice
pseudo-element:
background-color
caret-color
shade
cursor
fill
stroke
stroke-width
text-decoration
These guidelines had been chosen as a result of they are often effectively utilized by the browser with out recreating the location’s structure or inserting new DOM components (i.e., we might recreate this performance by wrapping the weather with an inline HTML tag like a span
).
To match the efficiency distinction between the CSS Customized Spotlight API and the standard textual content formatting methodology of inserting spans, see this efficiency comparability demo by Fernando Fiori.
Setting the vary
After creating a mode, the following step is setting the vary with CSS. First, we’ll outline our textual content vary with the constructor Vary()
; this can permit us to pick a fraction of a doc. This fragment can include nodes or components of textual content nodes.
The type of components the Vary()
constructor can choose is vital as a result of which means to get the textual content fragment we need to use, we’ll want to make use of features that return a kind of forms of components.
Let’s take into account the next textual content instance:
<p>This instance has <em>cursive</em> and <sturdy>daring</sturdy> textual content</p>
Now, let’s create the fashion:
:root::spotlight(instance) { background-color: rebeccapurple; shade: whitesmoke; }
If we attempt to create a spread with the p
component, every node or textual content fragment might be a brand new vary.
We will use the vary.setStart
and vary.setEnd
strategies to pick the a part of the textual content that we need to spotlight.
For this pattern textual content, every new component might be a brand new vary, so we’ll have the next:

Suppose we need to spotlight the textual content “cursive and robust” within the above sentence. On this case, the setStart
methodology needs to be 1
. You would possibly assume the setEnd
methodology needs to be 3
, however this isn’t precisely appropriate!
If we set the beginning of the vary at 1
, the API begins to pick it from the very starting of the component, and if we set the ending vary to 3
, the choice will finish initially of the third component. So, to pick all of the textual content contained in the sturdy
component, the attribute vary.setEnd
for this instance needs to be 4
.
The setStart
and setEnd
strategies obtain two arguments: the DOM component we need to use and the place. Right here’s the way it will look in our code:
const paragraph = doc.querySelector("p") const exampleRange = new Vary(); exampleRange.setStart(paragraph, 1); exampleRange.setEnd(paragraph, 4);
Making use of the spotlight
Now that we’ve got our desired vary, it’s time to spotlight it! For this, we have to create a brand new Spotlight()
constructor that can obtain our vary as an attribute:
const spotlight = new Spotlight(exampleRange)
Subsequent, we have to name the CSS.spotlight.set
methodology. It receives two attributes: the spotlight
class we created in our stylesheet and the brand new spotlight
that we beforehand created. That is how it will look in our code:
CSS.highlights.set("instance", spotlight);
However, what if you have to clear the highlights you created?
Simply name the CSS.highlights.clear()
methodology and the highlights might be cleared with no drawback.
Creating and highlighting extra advanced ranges
The method we demonstrated earlier for making a spotlight vary works nicely after we need to choose a full phrase, however what occurs when we have to choose simply a part of a phrase? Let’s return to our instance, and this time let’s attempt to spotlight solely the letters “previous tex”.
First, let’s create our fashion for this spotlight:
:root::spotlight(partial) { background-color: goldenrod; shade: black; }
OK, now how can we keep away from deciding on the complete fragment?
My method to this difficulty was to look carefully at how this component (on this case, the entire p
component) is made. First, I examined it in additional element with the console utilizing console.dir()
to verify the record of properties. Then I regarded on the childNodes
property and this was the outcome:
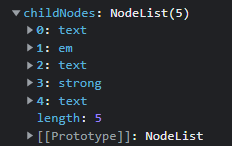
The textual content we need to spotlight, “previous tex”, is contained in the sturdy
component. To begin our vary with this textual content, we might use paragraph.childNodes[3]
, however that would choose the entire component. So, how can we choose simply part of the component?
As I discussed beforehand, Vary
will choose both a component node or a textual content node. In our case, we’ll have to verify inside this component for one thing that returns a textual content node.
Extra nice articles from LogRocket:
Let’s return to our console.dir()
as a result of it has a property that we’ll have the ability to use: firstChild
:
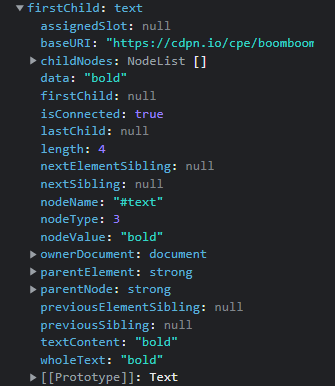
So now we’ve got paragraph.childNodes[3]
. firstChild
will return our string as a Textual content
prototype.
To set our beginning place, we have to verify the place the “o” in “previous” is positioned. On this instance, it’s the second character, so if we divide it as an array, the “o” can be place 1
. So after we set the setStart
methodology for our vary it is going to be like this:
partialRange.setStart(paragraph.childNodes[3].firstChild, 1)
Nice! Now, let’s set the top of this vary.
The final characters that we need to spotlight, “tex”, are positioned within the final component of our childNodes
. As you’ll discover within the childNodes
property’s record above, the final component has the sort textual content
, so accessing the firstChild
’s property isn’t obligatory.
Subsequent, we have to verify the place to finish our vary; we’ll use the setEnd
methodology, like so:
partialRange.setEnd(paragraph.childNodes[4], 4)
Now, all we’ve got to do is create the spotlight:
const partialHighlight = new Spotlight(partialRange); CSS.highlights.set("partial", partialHighlight)
Right here’s how the spotlight would look:
See the Pen
CSS customized spotlight demo by Cristian Diaz (@ItsCrisDiaz)
on CodePen.
I’m exhibiting each examples in the identical CodePen for a cause — it’s vital to contemplate how the browser will prioritize what to render when a number of types are utilized to the identical string.
For instance, the string “previous” in “daring” has each types. Which fashion might be displayed will rely on the place we resolve to name the CSS.highlights.set
methodology.
On this case, I referred to as the tactic that provides the instance
fashion first, and the tactic that provides the partial
fashion second. As a result of that is JavaScript, the final methodology that was declared will prevail. So, on this case, the “previous” string could have the partial
fashion utilized.
Lastly, as you would possibly suppose, the Vary()
methodology will be fairly exhausting to completely perceive. This methodology will probably begin to fall brief when you get thinking about scale. We’ll have a look at some examples later on this article that use different strategies for extra advanced use circumstances. However first, we have to take into account an vital consideration: accessibility.
Dealing with accessibility limitations
The CSS Customized Spotlight API may be very versatile, however what occurs when you have to expose the highlighting to assistive applied sciences like display readers? This API covers this, however proper now, it’s restricted to only some use circumstances.
Let’s begin with the fundamentals. Once you create a spotlight with the Spotlight()
constructor, there may be an attribute referred to as kind
. This attribute offers semantic that means to a spotlight and defines how it is going to be uncovered to display readers and different assistive applied sciences!
The categories we will use are pretty restricted to those three:
spotlight
: assigned by defaultspelling-error
: used to spotlight misspelled content materialgrammar-error
: used to spotlight grammatically incorrect content material
If the highlighting you utilize has one in every of these varieties, you undoubtedly ought to add the sort. To take action, simply change the attribute like so:
// Making a spotlight with a brand new vary const spotlight = new Spotlight(vary) // Altering the attribute kind of this vary spotlight.kind = "spelling-error"
Why solely these three varieties? In line with the W3C specification, it’s as a result of these are presumed to be the commonest use circumstances for this API:
This preliminary set of varieties was chosen as a result of they’re anticipated to be standard use circumstances for Spotlight API and there may be some current assist for expressing their semantics in platform accessibility APIs as we speak.
The specification additionally mentions that “UAs (Person Brokers) ought to make customized highlights out there to assistive applied sciences” and proper now it largely does.
Right here once more, I need to emphasize that the CSS Customized Spotlight API remains to be a piece in progress. I made some assessments with NVDA and Chrome and located that it’ll determine spelling or grammar errors earlier than the textual content is highlighted, however is not going to expose these errors when the textual content kind is spotlight
.
Why is that this vital? Nicely, if this spotlight isn’t there for merely visible functions however as an alternative must be uncovered to assistive applied sciences, you would possibly want to contemplate an alternative choice for this job. One answer I believe works nicely is the one ProgrammingTT demonstrates on this video the place the highlighted content material is wrapped within the <mark>
component.
As of this writing, solely two forms of spotlight are uncovered to assistive applied sciences, however the chance so as to add extra spotlight varieties is open, as W3C mentions:
Accessibility APIs at present don’t have any method to categorical the particular semantics of different anticipated Spotlight API use circumstances. Extra varieties might later be added to
HighlightType
as accessibility APIs achieve assist for expressing extra standard use circumstances of Spotlight API.
Now, with that mentioned, let’s try some use circumstances for this API!
CSS customized spotlight API examples
To get a greater understanding of what this API can do, let’s check out some implementations of this API made by Microsoft Edge’s group, in addition to an instance that I constructed. These use circumstances may even allow us to see alternative ways of dealing with textual content ranges.
Choose and spotlight internet textual content
Let’s begin with an instance from the Microsoft Edge video “Spotlight textual content on the internet with the CSS Customized Highlighting API.” On this venture, you’ll be able to choose textual content, spotlight it to retailer it in an inventory, after which verify it later. The saved textual content might be highlighted utilizing the CSS Customized Spotlight API.
For this instance, Patrick Brosset makes use of the doc.getSelection()
methodology to get create a spread with the next choice:
const choice = doc.getSelection(); const vary = choice.getRangeAt(0);
Since any methodology that returns a textual content fragment or a node fragment might be legitimate, doc.getSelection()
does the trick. Subsequent, Brosset begins including ranges with the Spotlight
methodology after which highlights them as we demonstrated beforehand.
Since there’s no reside demo of this video, I made a decision to recreate the performance of highlighting choice ranges. That is similar to our earlier instance however with one key distinction: this time there may be the chance that we’ll want to spotlight a number of ranges. For this, let’s first create some world variables:
let SELECTION_TEXTS = [] let selectionHighlighter = new Spotlight()
The primary variable is an array that can retailer every of the ranges we need to spotlight, and the second creates an occasion of the Spotlight()
class.
Why are we dealing with this globally? As a result of as an alternative of utilizing the syntax we used earlier than, we’ll use a way of this class so as to add our ranges.
Now, it’s time to create our perform to spotlight these textual content ranges:
const textHighlight = () => { const choice = doc.getSelection(); const vary = choice.getRangeAt(0) SELECTION_TEXTS.push(vary) SELECTION_TEXTS.forEach((choice) => { selectionHighlighter.add(choice) }) CSS.highlights.set("instance", selectionHighlighter) };
This can be a bit totally different from what we’ve got seen beforehand. Earlier than including something to our selectionHighlighter
, we’ve got to first add the brand new vary to our array. Then, we have to iterate it with the forEach
methodology to start out including the choice vary to the selectionHighlighter
with the Spotlight. add()
methodology. Subsequent, we simply have to name the CSS.highlights.set()
methodology as we’ve got executed earlier than.
However, what occurs if we have to clear the highlights? For this, we will use the next perform:
const clearHighlight = () => { selectionHighlighter.clear() SELECTION_TEXTS = [] CSS.highlights.clear() }
Much like the Spotlight.add()
, we will use Spotlight.clear()
to take away all highlighted components in our world variable. Then, we simply have to clear the worldwide variable we used for our array after which use the CSS.highlights.clear()
methodology as we beforehand demonstrated.
To see how this works, try this demo:
See the Pen
Choice textual content spotlight by Cristian Diaz (@ItsCrisDiaz)
on CodePen.
I selected this instance as a result of I wished to point out how we will use different methods to pick the specified textual content vary. On this case, doc.getSelection()
works, however what occurs when you have to create one thing on a much bigger scale? That is the place our second use case is available in!
Search in web page element
This subsequent demo, for a search in-page element, was additionally made by the Microsoft Edge group. It demonstrates find out how to create textual content nodes on a much bigger scale with using a TreeWalker
to get all nodes in a DOM component (on this case, the fundamental
component). As for the CSS Customized Spotlight API, we’ll want all textual content nodes, so we’ll use the NodeFilter.SHOW_TEXT
occasion.
Subsequent, TreeWalker
checks each node through the use of the TreeWalker.nextNode()
methodology and shops them within the allTextNodes
variable which supplies the next outcome:

This course of offers us an array of textual content components; we will verify every of them by including its title because the enter
. Then, it’s only a matter of including the ranges of the textual content nodes, discovering these outcomes, after which highlighting them as we‘ve executed earlier than with the CSS.highlights.set()
methodology. This methodology illustrates how we will use a TreeWalker
to assist us verify textual content nodes reliably on a much bigger scale.
This demo would significantly profit from extra semantic that means to the highlights for assistive applied sciences. Bear in mind, this API solely has three varieties, and highlighting a search outcome isn’t one in every of them! So W3C, should you’re seeing this, together with one of these semantic that means for search outcomes will surely turn out to be useful!
Now, let’s check out an instance that I constructed; it successfully makes use of the out there semantic that means!
Order this phrase app
My venture thought was to create an app the place the consumer receives a scrambled phrase after which wants to supply the accurately ordered phrase as the reply. The consumer will obtain visible suggestions through spotlight relying on whether or not the phrases throughout the submission are in the best order. I selected this instance as a result of it may reap the benefits of a spotlight semantic kind (on this case grammar-error
) to enhance the app’s accessibility!
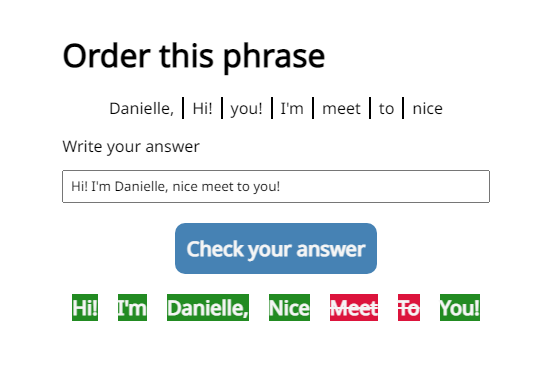
First, we have to create our spotlight courses! If we rely solely on background shade to spotlight the best and incorrect solutions, the outcomes can be tough to tell apart for customers with shade blindness. As a substitute, I made a decision to make use of strikeout (or line-through
) for incorrect solutions:
:root::spotlight(wrong-highlight) { background-color: crimson; shade: whitesmoke; text-decoration-line: line-through; text-decoration-thickness: 2px; } :root::spotlight(right-highlight) { background-color: forestgreen; shade: whitesmoke; }
As I discussed earlier than, the important thing to creating the semantic that means is including the property kind
to the specified spotlight class. On this case, I solely need to emphasize these phrases that seem within the incorrect order as a result of these create a grammar error, so that is what I did after I created the Spotlight()
cases globally. Since there might be a number of ranges to spotlight, I’ll should declare empty arrays, so I made a decision so as to add these globally as nicely:
let RIGHT_RANGES = []; let WRONG_RANGES = []; const rightHighlight = new Spotlight(); const wrongHighlight = new Spotlight(); wrongHighlight.kind = "grammar-error";
After a number of validations to verify if the added phrases coincide with those within the reply, it’s time to start out including our ranges! I made a decision to pick the reply’s container (on this case, the ol
) after which verify every of its kids. As every interior li
consists of solely this component, the vary begins at 0
and ends at 1
.
With that in thoughts, right here is the code I used:
const answerListNodes = ANSWER_LIST.kids; // Selects the vary of every <li> to start out the highlighting course of reply.forEach((phrase, index) => { const wordRange = new Vary(); wordRange.setStart(answerListNodes[index], 0); wordRange.setEnd(answerListNodes[index], 1); if (phrase === RIGHT_ORDER[index]) { // If the reply is correct RIGHT_RANGES.push(wordRange); } else { // If the reply is incorrect WRONG_RANGES.push(wordRange); } });
Subsequent, we will use the RIGHT_RANGES
and WRONG_RANGES
arrays so as to add the chosen ranges to our Spotlight()
cases after which use the CSS.highlights.set()
methodology to spotlight them on the display:
// Highlights the solutions in the best place RIGHT_RANGES.forEach((component) => { rightHighlight.add(component); }); CSS.highlights.set("right-highlight", rightHighlight); // Highlights the solutions in the best place WRONG_RANGES.forEach((component) => { wrongHighlight.add(component); }); CSS.highlights.set("wrong-highlight", wrongHighlight);
If you wish to see the way it works, try this CodePen:
See the Pen
Order phrase with customized spotlight by Cristian Diaz (@ItsCrisDiaz)
on CodePen.
Once more, I used this method as a result of the CSS customized spotlight API supplies a semantic kind that works fairly nicely for this instance.
Last ideas
The CSS Customized Spotlight API is a brand new characteristic that provides some fascinating options for some points we face referring to usability and accessibility. It additionally gives superior efficiency in comparison with different choices. This API generally is a bit tough when you think about find out how to choose a spread, however as soon as you work that out, the software opens up a complete new set of prospects!
This API remains to be in course of and at present lacks world assist, however I believe it has nice potential. I’m hopeful that sooner or later this API will broaden its browser assist and also will assist extra semantic varieties that can be utilized for assistive applied sciences.
Is your frontend hogging your customers’ CPU?
As internet frontends get more and more advanced, resource-greedy options demand increasingly more from the browser. If you happen to’re all in favour of monitoring and monitoring client-side CPU utilization, reminiscence utilization, and extra for your entire customers in manufacturing, attempt LogRocket.https://logrocket.com/signup/
LogRocket is sort of a DVR for internet and cellular apps, recording the whole lot that occurs in your internet app or web site. As a substitute of guessing why issues occur, you’ll be able to combination and report on key frontend efficiency metrics, replay consumer classes together with software state, log community requests, and mechanically floor all errors.
Modernize the way you debug internet and cellular apps — Begin monitoring without spending a dime.