On this article, we will likely be understanding the right way to write GET and POST requests to GRAPHQL APIs utilizing the Python request module. Coping with GraphQL API is a bit totally different in comparison with the easy REST APIs. Now we have to parse in a question that includes parsing the GraphQL queries within the request physique.
What are Requests in Python
Requests is a python package deal/library which helps in working with HTTP requests utilizing Python. We will very conveniently parse the physique, headers, and different parameters to a request utilizing strategies offered by the requests library like, GET, PUT, POST, DELETE, and so forth. Utilizing the requests module we will likely be parsing the queries to a GraphQL API.Â
What’s GraphQL API In Python
GraphQL API is a question language for fetching information from a database. It has a distinct method than conventional REST APIs. Utilizing GraphQL API, we will fetch the one required data, this avoids the drawbacks of beneath fetching and over fetching in comparison with the REST APIs. One of these API permits us to fetch very fine-tuned information in an outlined schema in order that the appliance can eat it, solely the info which is required will likely be fetched utilizing the GraphQl queries to the API.
Establishing requests in Python
For this text, we will likely be utilizing a public open API known as the Fruits API, utilizing the talked about directions, we will find the question explorer and mess around with the queries. It’s also possible to attempt your personal API or another open GraphQL API to your understanding.Â
Step 1:
To arrange requests, we have to set up requests in your native growth atmosphere. You’ll be able to both use a digital atmosphere or set up the package deal globally(not advisable). So, we’ll first arrange the digital atmosphere and set up the requests package deal in that atmosphere.
pip set up virtualenv
pip set up requests
Step 2:Â
It will set up the digital atmosphere package deal in python globally, so we will use it to create digital environments on our system.Â
virtualenv venv
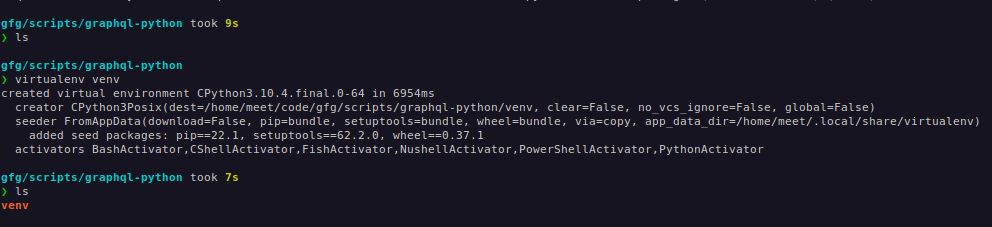
Â
The above command will create a digital atmosphere within the present listing with the title venv you possibly can title no matter you want.Â
Now, we additionally have to activate the atmosphere, so if any packages are put in within the session they’re saved or put in on this digital atmosphere and never globally. To do the activation of the digital atmosphere, we have to run the activate file situated within the venv folder.
This command is totally different for Linux/macOS and Home windows:
For Home windows: venvScriptsactivate
For Linux/macOS: supply venv/bin/activate
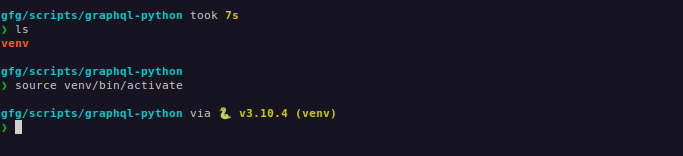
Â
Now, we will now create scripts and make the most of this library for our purpose which is to ship get/publish requests to a GraphQL API.
GET methodology utilizing Python requests
We will ship a GET request to the given GraphQL API with the get methodology offered within the requests library which we imported. Â Right here as a result of design of the API we’ve got to make use of the POST methodology to fetch the outcomes however it might rely on the API, we are going to dive into that later.
So, we’ve got to get the question for fetching the small print of the Fruit on this case. We will use the given schema like fruit adopted by the id of it and parse within the attributes which we need to fetch, On this case, we’re fetching the scientific title and tree_name however that may be another attributes or all attributes from the given set of schema.
Now we have to parse the question to the JSON physique, to try this we are going to name the request.publish methodology which can publish the question and return us the info. Right here, the publish methodology takes just a few arguments just like the URL to fetch and the JSON physique to parse. We are going to parse the URL which we outlined earlier as the primary argument after which observe it by the JSON which will likely be a JSON object with a key question and can cross the question physique in it. This will likely be saved in a variable for later use.
Python3
|
Output:
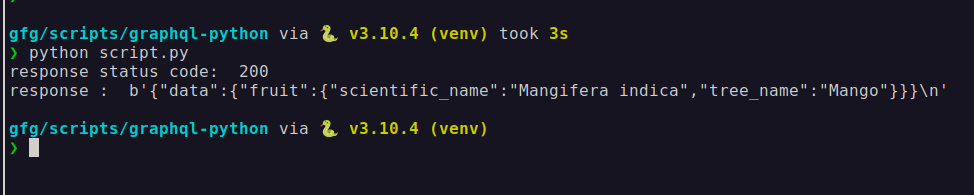
Â
Right here, we will see that the question has returned the info with the required attributes which might be scietific_name and tree_name which have been parsed within the question. That is GraphQL that’s the reason we will get the precise information that’s required of us. We will entry the status_cide from the response object and the returned content material utilizing the content material attribute.
Now, we additionally want to have a look at different GraphQL API which behaves a bit in another way than the present API which was about Fruits. The API is Star Wars API which we are going to see can fetch the outcomes with a GET request. The next API could be accessed from the hyperlink.
Python3
|
Output:
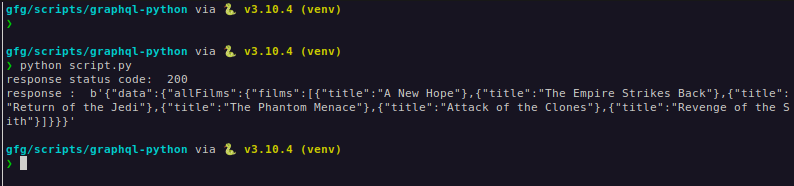
Â
Right here we’ve got used the GET methodology for fetching the info. The question is totally different in comparison with the earlier instance for the reason that API can be totally different. The parameters parsed have remained the identical format however the important thing adjustments are simply the values of variables like URL and the physique question. This question will even return the specified final result if we use a POST methodology as follows:
response = requests.publish(url=url, json={"question": physique})
So, each API which makes use of GraphQL generally is a bit totally different when it comes to its utilization, so we’ve got to get round a bit to seek out which strategies are suited nicely right here.
POST methodology utilizing Python requests
We will additionally publish information to a GraphQl API utilizing the requests library. We merely need to observe the schema and parse the info appropriately. We’re utilizing the Fruit API once more to publish the info to it. So, we’ve got a distinct sort of schema, as an alternative, we’ve got a mutation to write down as an alternative of a question. We observe up the documentation to the fitting to get the schema.
We will now write up the schema and parse the attributes which we need to return after the mutation has been saved i.e. the POST request has been made, we are going to parse the required information.
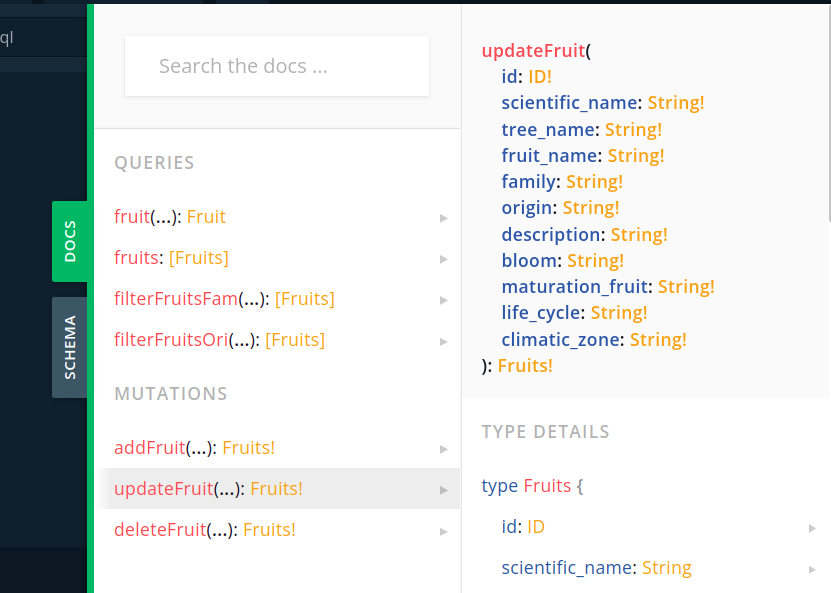
Â
Right here, we’re making a mutation with the attribute title and the worth which we need to assign in order the key-value map. After the mutation, we question the attributes which we wish, right here we’re querying the attributes like id, scientific_name, tree_name, fruit_name, and origin. However these could be another attributes or all attributes relying on the requirement.Â
Python3
|
Output:
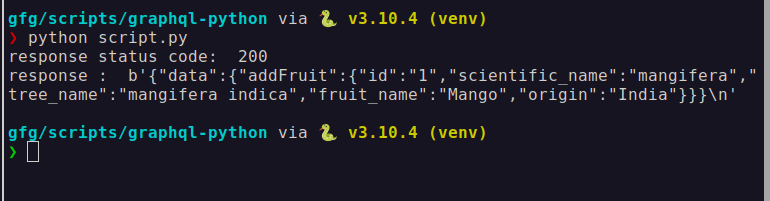
Â
The POST methodology is used within the above script for posting the info so the requests.publish methodology as beforehand stated takes in a few arguments like URL and JSON, it additionally takes extra arguments however is optionally available and depending on the request we’re making.Â
UPDATE methodology utilizing Python requests
Just like POST requests we will use the Replace/PUT methodology to replace particular or all fields within the schema. Right here as nicely we have to create a mutation schema as beforehand. We are going to make the key-value maps for the attribute title and assign worth to it. Thereafter we will point out the attributes we want to fetch from the question after the replace has been profitable.Â
Now we have used the publish methodology once more to replace the attributes outlined earlier, we’ve got adopted the schema within the documentation.
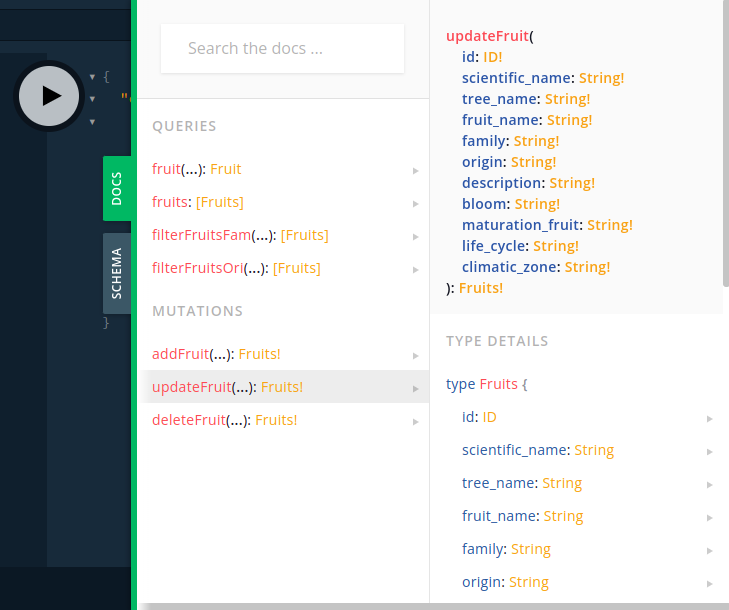
Â
Python3
|
Output:

Â
Right here, we’ve got up to date the fruit attributes, utilizing the updateFruit mutation, we’ve got assigned the values and parsed the mutation. For the particular design of the API, you may also attempt the requests.replace methodology to replace the document if the publish methodology doesn’t work.
DELETE methodology utilizing Python requests
We will even use the delete choice to delete a document from the database with the GraphQl API utilizing requests. We are going to once more have to make use of the publish methodology to delete the document. For additional operations, you may also want the requests.delete methodology to delete the document utilizing the GraphQL API. Now we have used the Delete Fruit mutation which takes within the parameter which is the id of the fruit additional we can also fetch the specified attributes however we are going to return the values as null since they’re deleted.
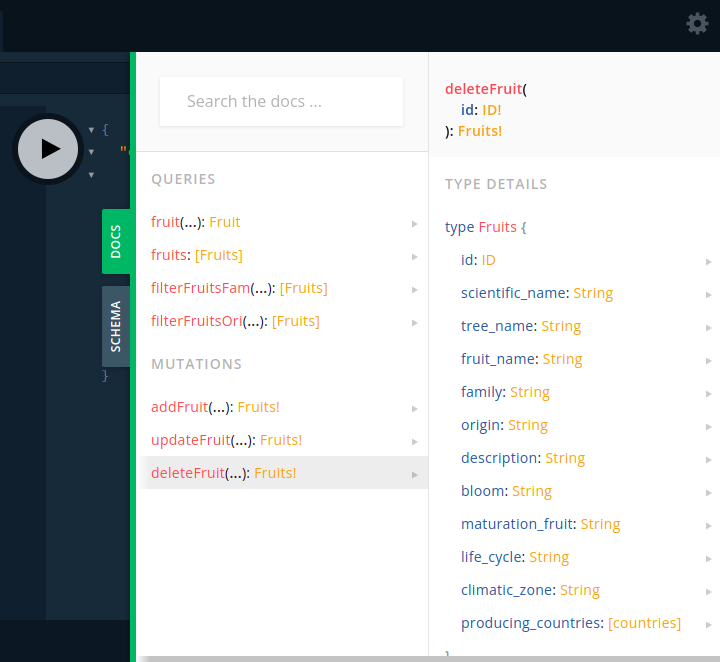
Â
Python3
|
Output:
So, that is how we delete the article from the database utilizing the GrpahQL API. The parameters handed to the publish methodology operate just like the URL of the API and the physique which can comprise the mutation question.Â

Â