Introduction
If there’s one factor Flutter is thought for, it’s creating lovely consumer interfaces. By combining numerous top-level widgets and an acceptable structure, we are able to create a UI that blows the minds of our customers. Flutter takes it even additional by offering widgets that allow us so as to add interactive animations to consumer interfaces, which helps create a novel expertise for our customers.
There are numerous top-level widgets that come collectively to type the idea for the UIs we see and work together with: from columns and rows for layouts, SingleChildScrollView
for vertical and horizontal scrolling, the GestureDetector
for interactivity, the assorted implicit animation widgets, and an entire lot extra. Figuring out the suitable mixture from the ocean of top-level widgets that Flutter offers us can grant us entry to a variety of mixtures and functionalities, and helps obtain our desired UI.
On this article, we’ll be one among such widget: the Chip widget.
We’ll see how it’s used as a element for constructing and enhancing consumer interfaces in Flutter. We could?
The Chip widget is a top-level widget that gives a rounded field with textual content and customized interactions. By means of its properties, we now have entry to a spread of actions that can be utilized to beautify our consumer interfaces and make them interactive.
A preferred use case is for class tiles. Utilizing Chip widgets, we are able to customise the class tile, in addition to simply add and take away classes in our UI easily and simply, enhancing UX inside our cellular apps.
The Chip widget exposes a spread of properties that let you add totally different types and carry out numerous actions.
const Chip( {Key? key, Widget? avatar, required Widget label, TextStyle? labelStyle, EdgeInsetsGeometry? labelPadding, Widget? deleteIcon, VoidCallback? onDeleted, Coloration? deleteIconColor, String? deleteButtonTooltipMessage, BorderSide? facet, OutlinedBorder? form, Clip clipBehavior = Clip.none, FocusNode? focusNode, bool autofocus = false, Coloration? backgroundColor, EdgeInsetsGeometry? padding, VisualDensity? visualDensity, MaterialTapTargetSize? materialTapTargetSize, double? elevation, Coloration? shadowColor, )
Let’s check out a few of these properties:
label
: Takes middle stage within theChip
widget as a result of it’s a required property. Many occasions, it’s used to show aTextual content
widget to offer info on theChip
labelStyle
: Applies styling to thelabel
avatar
: Shows an icon, picture, and so on., earlier than thelabel
propdeleteIcon
: Takes within the icon that’s to be displayed when theonDeleted
property is known asonDeleted
: Known as when the consumer clicks thedeleteIcon
property with a view to delete the chipelevation
: Takes in a double worth that defines the quantity of elevated top of theChip
padding
: Applies padding between the contents of the chip and the skin
Sorts of Chip widgets
InputChip
: Used to characterize a fancy piece of knowledge in a compact typeChoiceChip
: Permits a consumer select from a set of choices offeredFilterChip
: Makes use of tags/phrases to filter content materialActionChip
: Used to characterize an motion associated to the Chip’s main goal, i.e., so as to add a filter
Any of those Chip widgets may be mixed with different widgets in Flutter to create a ravishing, interactive UI. Within the subsequent part, we’ll dive right into a pattern implementation and see how we are able to boost our consumer interfaces utilizing Chip widgets.
Lastly, time to get all the way down to it. We’ll be constructing a pattern software that makes use of the Chip widget to show a listing of classes. Customers will be capable to add and take away classes with out affecting the consumer interface.
Extra nice articles from LogRocket:
First, create a mission by operating the command under.
flutter create chip_project
This creates the codebase, together with the assorted recordsdata wanted to run our software.
Subsequent, take away the default generated code within the essential.dart
file and change it with a stateless widget that returns MaterialApp
. Identify the Stateless
widget MyApp
.
void essential() => runApp(const MyApp()); class MyApp extends StatelessWidget { const MyApp({Key? key}) : tremendous(key: key); @override Widget construct(BuildContext context) { return const MaterialApp( title: 'Materials App', house: Scaffold(), ); } }
Subsequent, create a brand new stateful widget and name it HomeView
. We‘ll use this widget to create a easy consumer interface that exhibits us a method of displaying, including, and eradicating Chip widgets.
class HomeView extends StatefulWidget { const HomeView({Key? key}) : tremendous(key: key); @override State<HomeView> createState() => _HomeViewState(); } class _HomeViewState extends State<HomeView> { @override Widget construct(BuildContext context) { return Container(); } }
Earlier than we proceed, hyperlink the HomeView
widget to the MyApp
widget created within the final step.
.... @override Widget construct(BuildContext context) { return const MaterialApp( title: 'Materials App', house: HomeView(), ); } ....
Now we are able to begin filling out the HomeView
widget and show the Chips on the display screen. With a purpose to dynamically structure the Chips in our consumer interface, we’ll use a Wrap
widget and move the chips to it as youngsters.
What occurs subsequent is that, based mostly on display screen width, the Chips mechanically lay themselves out in the absolute best method, avoiding overflow or clumping of the consumer interface. Virtually, which means remaining Chips go the subsequent line and structure easily if the width of the display screen can’t match all of them on the identical line.
For the aim of this text, we’ll create a static listing of classes that can show utilizing the Chip widgets. This listing can characterize information coming from anyplace, so in your case, it could possibly be a listing of knowledge coming from an API endpoint.
.... Record<String> classes = [ 'Music', 'Arts', 'Culture', 'Education', 'Flowers', ]; ....
Subsequent, we’ll create the 2 features, which we’ll be utilizing so as to add and take away information from the listing. This additionally means including and eradicating Chips, because the Chips render based mostly on the info within the listing, i.e., classes
.
void addCategories(String categoryName) { classes.add(categoryName); setState(() {}); } void removeCategories(String categoryName) { classes.take away(categoryName); setState(() {}); }
Add a Scaffold widget with an Appbar
and padding to make the consumer interface look higher. At this level, that is what the code for our HomeView
Stateful widget seems to be like:
class HomeView extends StatefulWidget { const HomeView({Key? key}) : tremendous(key: key); @override State<HomeView> createState() => _HomeViewState(); } class _HomeViewState extends State<HomeView> { Record<String> classes = [ 'Music', 'Arts', 'Culture', 'Education', 'Flowers', ]; void addCategories(String categoryName) { classes.add(categoryName); setState(() {}); } void removeCategories(String categoryName) { classes.take away(categoryName); setState(() {}); } @override Widget construct(BuildContext context) { return Scaffold( appBar: AppBar( title: const Textual content('Chip Widgets in use'), centerTitle: true, backgroundColor: Colours.black, ), physique: SafeArea( baby: Padding( padding: const EdgeInsets.all(16.0), baby: Heart( baby: Column( mainAxisAlignment: MainAxisAlignment.middle, youngsters: [ // .... ], ), ), ), ), ); } }
We are able to now transfer forward so as to add the Wrap and Chip widgets. We’ll show the Chips based mostly on the info within the listing, which implies that there’ll be a Chip
on the display screen if there’s an merchandise in our classes
listing. To realize this, we map each merchandise within the listing to a Chip widget and show the merchandise title because the label on the Chip.
With a purpose to take away a Chip
from the listing, we’ll move the removeCategories
operate created earlier to the onDeleted
callback that the Chip
widget offers.
If the classes
listing is empty, we show a Textual content
to the consumer informing them of the empty state, i.e., No classes accessible.
.... [ const SizedBox(height: 16), Wrap( spacing: 24, children: categories.map( (category) { return Chip( onDeleted: () { removeCategories(category); }, deleteIcon: const Icon(Icons.remove_circle), label: Text(category), ); }, ).toList(), ), if (categories.isEmpty) const Center( child: Text('No Categories available!'), ), ], ....
Including and eradicating classes
Subsequent, we add a TextField
. The TextField
permits us to manually add a brand new class to the listing of classes, thereby including to the variety of Chip
widgets on the display screen.
Within the onSubmitted
callback for the TextField
, name the addCategories
operate and move within the class from the textual content enter retrieved from the consumer. You may add a little bit of styling to the TextField
widget to make it look higher on the display screen.
.... const SizedBox(top: 64), const Textual content( 'Enter the Class and press enter so as to add it.', type: TextStyle( fontSize: 18, fontWeight: FontWeight.w500, ), ), const SizedBox(top: 16), TextField( onSubmitted: (class) { addCategories(class); }, cursorColor: Colours.black, ornament: InputDecoration( labelText: 'Enter Class Identify', hintText: 'Enter Class Identify', labelStyle: TextStyle(coloration: Colours.black), enabledBorder: OutlineInputBorder( borderRadius: BorderRadius.round(16), ), focusedBorder: OutlineInputBorder( borderRadius: BorderRadius.round(16), ), ), ), ....
With these added, we’re good to go! Let’s take a look at run our software. Run the command under to run the app.
flutter run
Right here is the consequence under:
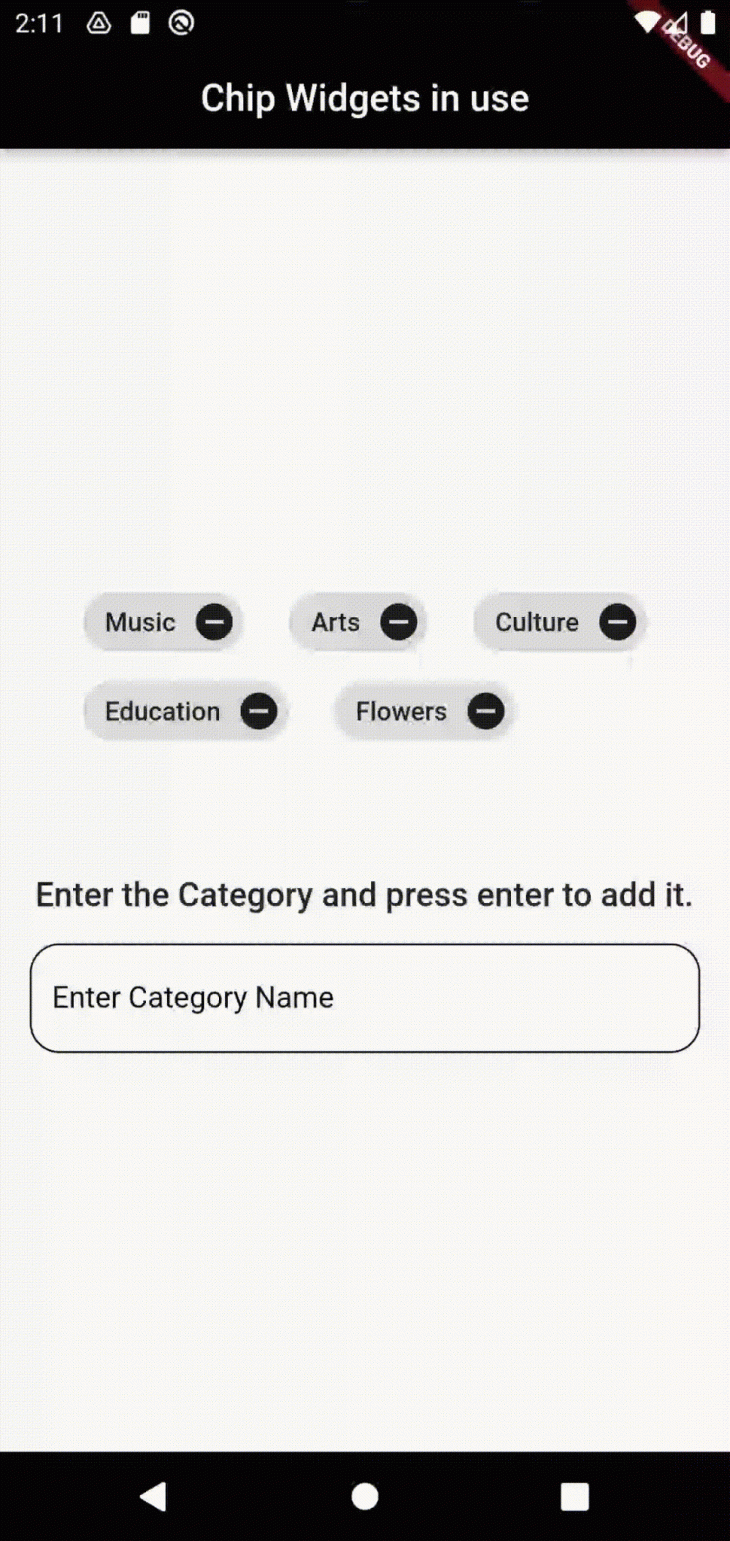
Take a look at the total code for the pattern software right here.
Conclusion
Hurray! You’ve accomplished nicely reaching this part. You’ve discovered what the Chip widget is, the assorted varieties of Chip widgets, the assorted properties it exposes and the way, collectively, we’ve constructed a pattern software that exhibits the Chip widget in use and the way it can enormously support in our improvement. Now you’ll be able to confidently make use of it in your purposes.
You probably have any questions, be happy to achieve out to me by way of any of my social media platforms. I’ll be glad to help and reply any questions. Until I write to you once more, comfortable Fluttering.
LogRocket: Full visibility into your net and cellular apps
LogRocket is a frontend software monitoring answer that allows you to replay issues as in the event that they occurred in your personal browser. As a substitute of guessing why errors occur, or asking customers for screenshots and log dumps, LogRocket allows you to replay the session to rapidly perceive what went improper. It really works completely with any app, no matter framework, and has plugins to log extra context from Redux, Vuex, and @ngrx/retailer.
Along with logging Redux actions and state, LogRocket data console logs, JavaScript errors, stacktraces, community requests/responses with headers + our bodies, browser metadata, and customized logs. It additionally devices the DOM to report the HTML and CSS on the web page, recreating pixel-perfect movies of even essentially the most complicated single-page net and cellular apps.