Alternate options to the Apply perform to enhance the efficiency by 700x
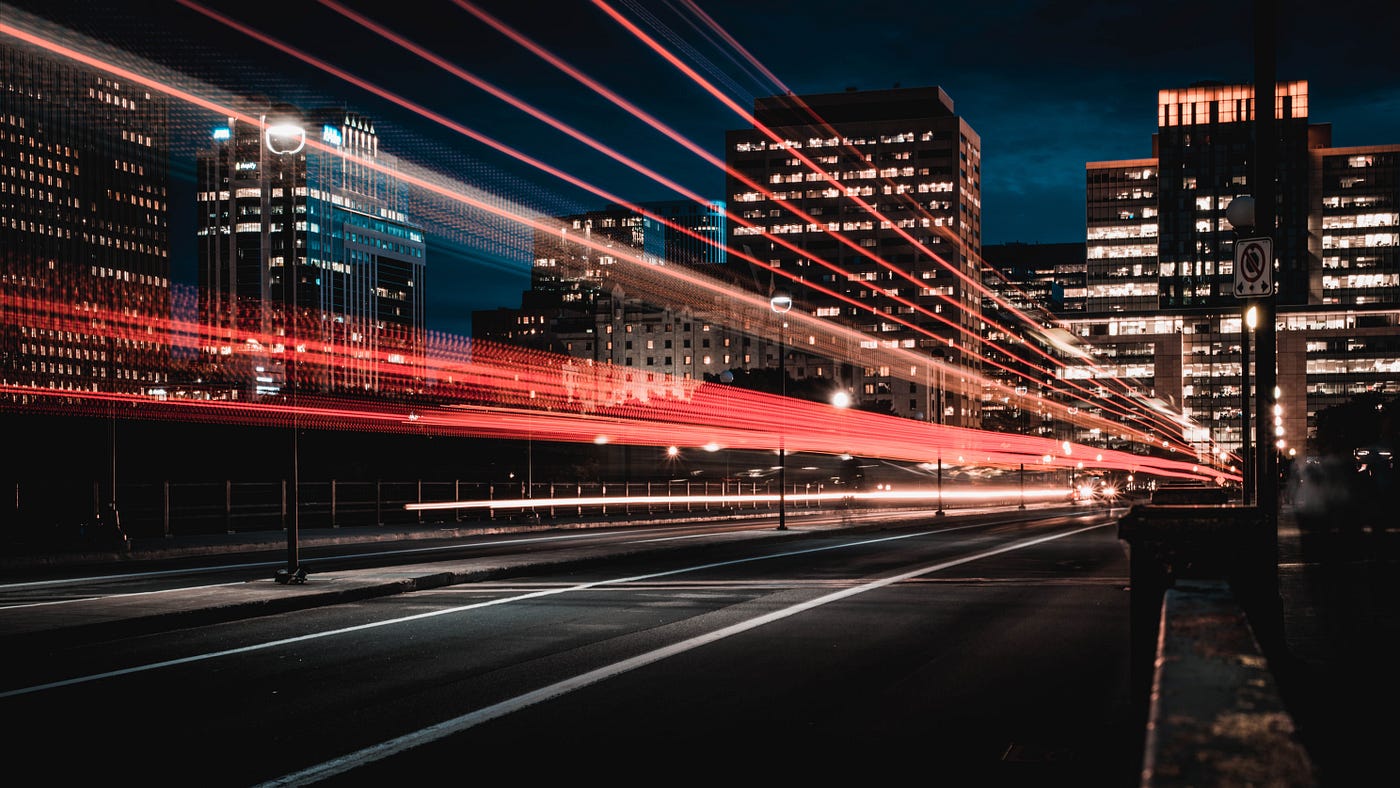
What’s Apply perform — when you don’t know this already!
Apply perform is a one-liner perform that can be utilized to use operations on all the weather of a dataframe or throughout the rows or columns of a dataframe.
Introduction
Apply perform is actively utilized by Knowledge Scientists/Analysts with a purpose to carry out the information manipulation duties however is it actually optimum to make use of when your information has too many rows?
Probably not!
On this weblog, we’ll take a look at the next 3 very helpful alternate options that you need to use rather than the Apply perform particularly you probably have numerous rows:
- Parallelization
- Loops (Sure, it might be quicker!)
- Vectorization
However first, let’s take a look at the execution time of apply perform on a big dataset.
Create the dataset
We are going to create a dataset having 5 Million rows and 4 columns. Every column can have an integer worth from 0 to 50.
import numpy as np
import pandas as pddf = pd.DataFrame(np.random.randint(0, 50, dimension=(5000000, 4)), columns=('a','b','c','d'))df.form
# (5000000, 5)df.head()

Outline the perform
Now, let’s outline our perform that returns some values primarily based on the values throughout the current columns of the dataframe/enter variables.
def infer(a,b,c,d):
if a==0:
return d
elif a <= 25 & a>0:
return b-c
else:
return b+c
Apply perform
Now, we’ll use the ‘apply’ perform to name the infer perform outlined within the above step.
import timebegin = time.time()
df['e'] = df.apply(lambda x: infer(x['a'], x['b'], x['c'], x['d']), axis = 1)
finish = time.time()
print(finish - begin)### Time taken: 200 seconds
The apply perform takes round 200 seconds to get the outcomes.
Let’s take a look at the choice approaches:
Possibility 1: Parallelization
Parallelization is achieved by dividing a big chunk of knowledge into smaller chunks and these smaller chunks are processed parallelly utilizing the potential of a number of CPUs and cores.
There are a few packages equivalent to Swifter and Dask utilizing which we will obtain parallelization. On this weblog, we’ll take a look at swifter as a result of it is extremely straightforward to make use of and achieves nearly the identical outcomes or higher ends in some instances.
We will merely name the swifter package deal after our dataframe after which proceed with the apply perform.
## set up the package deal
!pip set up swifter## import the package deal
import swifter## use the swifter package deal
begin = time.time()
df['e'] = df.swifter.apply(lambda x: infer(x['a'], x['b'], x['c'], x['d']), axis = 1)finish = time.time()
print(finish - begin)## Time taken: 60 seconds
So, with the Swifter package deal, we will cut back the execution time by greater than 3x, not unhealthy for a easy addition of the package deal title.
Swifter tries to implement the apply perform in the very best means by both vectorizing it or parallelizing it within the backend utilizing Dask or by merely utilizing pandas apply if the dataset is small.
Possibility 2: Loops
I do know it sounds stunning however you probably have a dataset that doesn’t have too many columns then this system might be useful to get even higher outcomes as in comparison with the swifter package deal.
The trick is to first convert the dataframe right into a default gentle information construction equivalent to an array or a dictionary after which iterate by the rows to govern the dataset.
begin = time.time()## empty record
list2 = []## including 'e' column with default 0
df['e'] = 0## convert dataframe into array
for row in df.values:
if row[0] == 0:
row[4] = row[3]elif row[0] <= 25 & row[0] > 0:
row[4] = row[1]-row[2]
else:
row[4] = row[1] + row[2]
list2.append(row)df2 = pd.DataFrame(list2, columns=['a', 'b', 'c', 'd','e'])
finish = time.time()
print(finish - begin)## Time taken: 20 seconds
So, iterating by an array utilizing the for loop, we’re in a position to cut back our execution time to twenty seconds which is a 10x enchancment over the apply perform.
Possibility 3: Vectorization
Vectorization is the strategy of implementing array operations on a dataset. Within the background, it applies the operations to all the weather of an array or sequence in a single go (not like a for loop which manipulates one row at a time).
It’s the finest follow to vectorize your python code for reaching the most effective efficiency.
# utilizing vectorization
begin = time.time()
df['e'] = df['b'] + df['c']
df.loc[df['a'] <= 25, 'e'] = df['b'] -df['c']
df.loc[df['a']==0, 'e'] = df['d']finish = time.time()
print(finish - begin)
## 0.28007707595825195 sec
So, we’re in a position to cut back the run time to 0.28 seconds which is ~700x higher than the run time of the apply perform.
- We checked out 3 alternate options to the Apply perform in python particularly if the dataset has numerous rows.
- We realized in regards to the parallel processing functionality of the swifter package deal.
- We discovered that loops in python are usually not that unhealthy if used neatly.
- For a big dataset, vectorization of the code gave the most effective efficiency enchancment of round 700x over the apply perform. So, it’s best follow to make use of vectorization for manipulating a big dataset.