GraphQL is the brand new buzzword in API growth. Whereas RESTful APIs stay the most well-liked option to expose information from purposes, they arrive with many limitations that GraphQL goals to resolve.
GraphQL is a question language created by Fb, which was was an open-source challenge in 2015. It gives an intuitive and versatile syntax for describing and accessing information in an API.
This information will discover methods to construct a GraphQL Node.js challenge. We’ll use GraphQL to construct a Todo software within the Specific.js net framework for Node.
What Is GraphQL?
From the official documentation: “GraphQL is a question language for APIs and a runtime for fulfilling these queries along with your present information. GraphQL supplies an entire and comprehensible description of the information in your API, provides purchasers the ability to ask for precisely what they want and nothing extra, makes it simpler to evolve APIs over time, and permits highly effective developer instruments.”
GraphQL is a server-side runtime for executing queries utilizing the sort system you outlined on your information. Additionally, GraphQL is just not tied to any particular database or storage engine. As a substitute, it’s backed by your present code and information retailer. You may get an in depth comparability of those applied sciences with the GraphQL vs. RESTful API information.
To create a GraphQL service, you begin by defining schema varieties and creating fields utilizing these varieties. Subsequent, you present a perform resolver to be executed on every discipline and sort every time information is requested by the shopper facet.
GraphQL Terminology
GraphQL kind system is used to explain what information will be queried and what information you possibly can manipulate. It’s the core of GraphQL. Let’s focus on other ways we will describe and manipulate information in GraphQ.
Sorts
GraphQL object varieties are information fashions containing strongly typed fields. There must be a 1-to-1 mapping between your fashions and GraphQL varieties. Under is an instance of GraphQL Sort:
kind Person {
id: ID! # The "!" means required
firstname: String
lastname: String
e mail: String
username: String
todos: [Todo] # Todo is one other GraphQL kind
}
Queries
GraphQL Question defines all of the queries {that a} shopper can run on the GraphQL API. It’s best to outline a RootQuery that can include all present queries by conference.
Under we outline and map the queries to the corresponding RESTful API:
kind RootQuery {
person(id: ID): Person # Corresponds to GET /api/customers/:id
customers: [User] # Corresponds to GET /api/customers
todo(id: ID!): Todo # Corresponds to GET /api/todos/:id
todos: [Todo] # Corresponds to GET /api/todos
}
Mutations
If GraphQL Queries are GET requests, mutations are POST, PUT, PATCH, and DELETE requests that manipulate GraphQL API.
We’ll put all of the mutations in a single RootMutation to display:
kind RootMutation {
createUser(enter: UserInput!): Person # Corresponds to POST /api/customers
updateUser(id: ID!, enter: UserInput!): Person # Corresponds to PATCH /api/customers
removeUser(id: ID!): Person # Corresponds to DELETE /api/customers
createTodo(enter: TodoInput!): Todo
updateTodo(id: ID!, enter: TodoInput!): Todo
removeTodo(id: ID!): Todo
}
You observed using -input varieties for the mutations akin to UserInput, TodoInput. It’s at all times greatest observe to at all times outline Enter varieties for creating and updating your sources.
You may outline the Input varieties just like the one beneath:
enter UserInput {
firstname: String!
lastname: String
e mail: String!
username: String!
}
Resolvers
Resolvers inform GraphQL what to do when every question or mutation is requested. It’s a fundamental perform that does the laborious work of hitting the database layer to do the CRUD (create, learn, replace, delete) operations, hitting an inside RESTful API endpoint, or calling a microservice to satisfy the shopper’s request.
You may create a brand new resolvers.js file and add the next code:
import sequelize from '../fashions';
export default perform resolvers () {
const fashions = sequelize.fashions;
return {
// Resolvers for Queries
RootQuery: {
person (root, { id }, context) {
return fashions.Person.findById(id, context);
},
customers (root, args, context) {
return fashions.Person.findAll({}, context);
}
},
Person: {
todos (person) {
return person.getTodos();
}
},
}
// Resolvers for Mutations
RootMutation: {
createUser (root, { enter }, context) {
return fashions.Person.create(enter, context);
},
updateUser (root, { id, enter }, context) {
return fashions.Person.replace(enter, { ...context, the place: { id } });
},
removeUser (root, { id }, context) {
return fashions.Person.destroy(enter, { ...context, the place: { id } });
},
// ... Resolvers for Todos go right here
}
};
}
Schema
GraphQL schema is what GraphQL exposes to the world. Due to this fact, the categories, queries, and mutations shall be included contained in the schema to be uncovered to the world.
Under is methods to expose varieties, queries, and mutations to the world:
schema {
question: RootQuery
mutation: RootMutation
}
Within the above script, we included the RootQuery and RootMutation we created earlier to be uncovered to the world.
How Does GraphQL Work With Nodejs and Expressjs
GraphQL supplies an implementation for all main programming languages, and Node.js is just not exempted. On the official GraphQL web site, there’s a part for JavaScript help, and in addition, there are different implementations of GraphQL to make writing and coding in GraphQL easy.
GraphQL Apollo supplies an implementation for Node.js and Specific.js and makes it simple to get began with GraphQL.
You’ll discover ways to create and develop your first GraphQL software in Nodes.js and Specific.js backend framework utilizing GraphQL Apollo within the subsequent part.
Organising GraphQL With Specific.js
Constructing a GraphQL API server with Specific.js is simple to get began. On this part, we are going to discover methods to construct a GraphQL server.
Initialize Venture With Specific
First, it’s good to install and arrange a brand new Specific.js challenge.
Create a folder on your challenge and set up Specific.js utilizing this command:
cd <project-name> && npm init -y
npm set up categorical
The command above creates a brand new package deal.json file and installs the Specific.js library into your challenge.
Subsequent, we are going to construction our challenge as proven within the picture beneath. It’ll include completely different modules for the options of the challenge akin to customers, todos, and so forth.
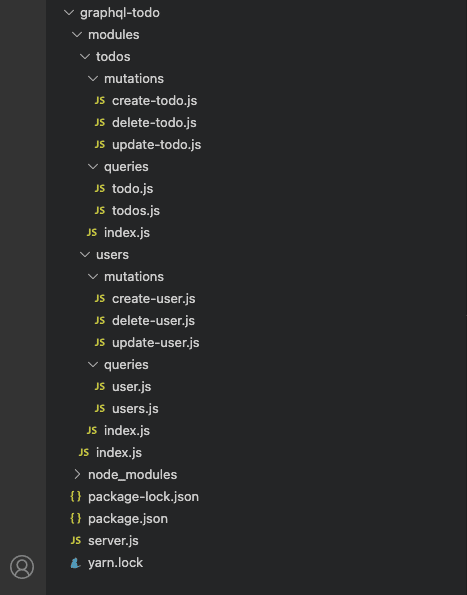
Initialize GraphQL
Let’s begin by putting in the GraphQL Specific.js dependencies. Run the next command to put in:
npm set up apollo-server-express graphql @graphql-tools/schema --save
Creating Schemas and Sorts
Subsequent, we’re going to create an index.js file contained in the modules folder and add the next code snippet:
const { gql } = require('apollo-server-express');
const customers = require('./customers');
const todos = require('./todos');
const { GraphQLScalarType } = require('graphql');
const { makeExecutableSchema } = require('@graphql-tools/schema');
const typeDefs = gql`
scalar Time
kind Question {
getVersion: String!
}
kind Mutation {
model: String!
}
`;
const timeScalar = new GraphQLScalarType({
identify: 'Time',
description: 'Time customized scalar kind',
serialize: (worth) => worth,
});
const resolvers = {
Time: timeScalar,
Question: {
getVersion: () => `v1`,
},
};
const schema = makeExecutableSchema({
typeDefs: [typeDefs, users.typeDefs, todos.typeDefs],
resolvers: [resolvers, users.resolvers, todos.resolvers],
});
module.exports = schema;
Code Walkthrough
Let’s work by means of the code snippet and break it down:
Step 1
First, we imported the required libraries and created default question and mutation varieties. The question and mutation solely set the model of the GraphQL API for now. Nevertheless, we are going to prolong the question and mutation to incorporate different schemas as we proceed.
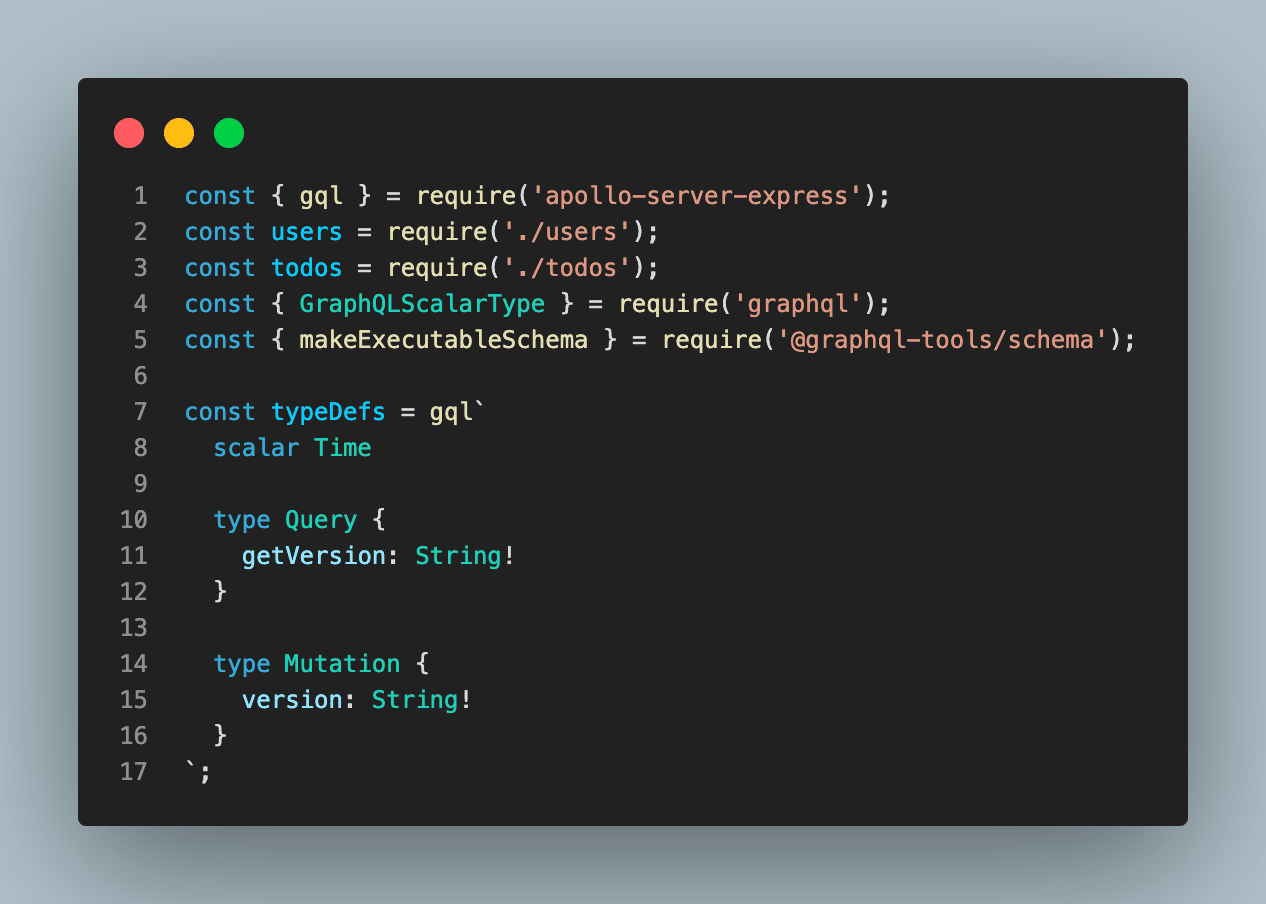
Step 2:
Then we created a brand new scalar kind for time and our first resolver for the question and mutation created above. As well as, we additionally generated a schema utilizing the makeExecutableEchema perform.
The generated schema contains all the opposite schemas we imported and also will embrace extra after we create and import them.
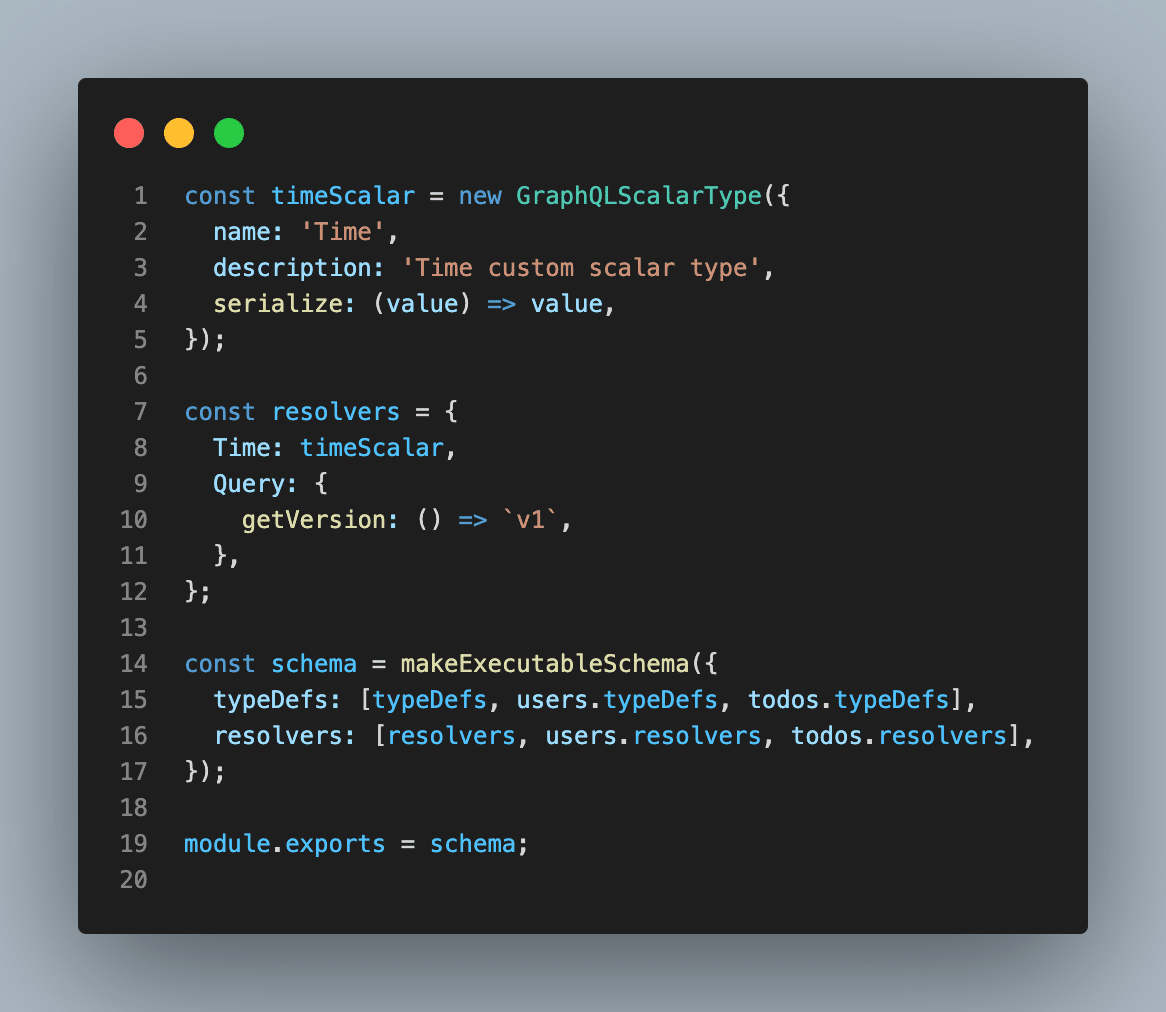
The above code snippet exhibits that we imported completely different schemas into the makeExecutableEchema perform. This method helps us in structuring the applying for complexity. Subsequent, we’re going to create the Todo and Person schemas we imported.
Creating Todo Schema
The Todo schema exhibits easy CRUD operations that customers of the applying can carry out. Under is the schema that implements the Todo CRUD operation.
const { gql } = require('apollo-server-express');
const createTodo = require('./mutations/create-todo');
const updateTodo = require('./mutations/update-todo');
const removeTodo = require('./mutations/delete-todo');
const todo = require('./queries/todo');
const todos = require('./queries/todos');
const typeDefs = gql`
kind Todo {
id: ID!
title: String
description: String
person: Person
}
enter CreateTodoInput {
title: String!
description: String
isCompleted: Boolean
}
enter UpdateTodoInput {
title: String
description: String
isCompleted: Boolean
}
prolong kind Question {
todo(id: ID): Todo!
todos: [Todo!]
}
prolong kind Mutation {
createTodo(enter: CreateTodoInput!): Todo
updateTodo(id: ID!, enter: UpdateTodoInput!): Todo
removeTodo(id: ID!): Todo
}
`;
// Present resolver features on your schema fields
const resolvers = {
// Resolvers for Queries
Question: {
todo,
todos,
},
// Resolvers for Mutations
Mutation: {
createTodo,
updateTodo,
removeTodo,
},
};
module.exports = { typeDefs, resolvers };
Code Walkthrough
Let’s work by means of the code snippet and break it down:
Step 1:
First, we created a schema for our Todo utilizing GraphQL kind, enter, and prolong. The prolong key phrase is used to inherit and add new queries and mutations to the prevailing root question and mutation we created above.
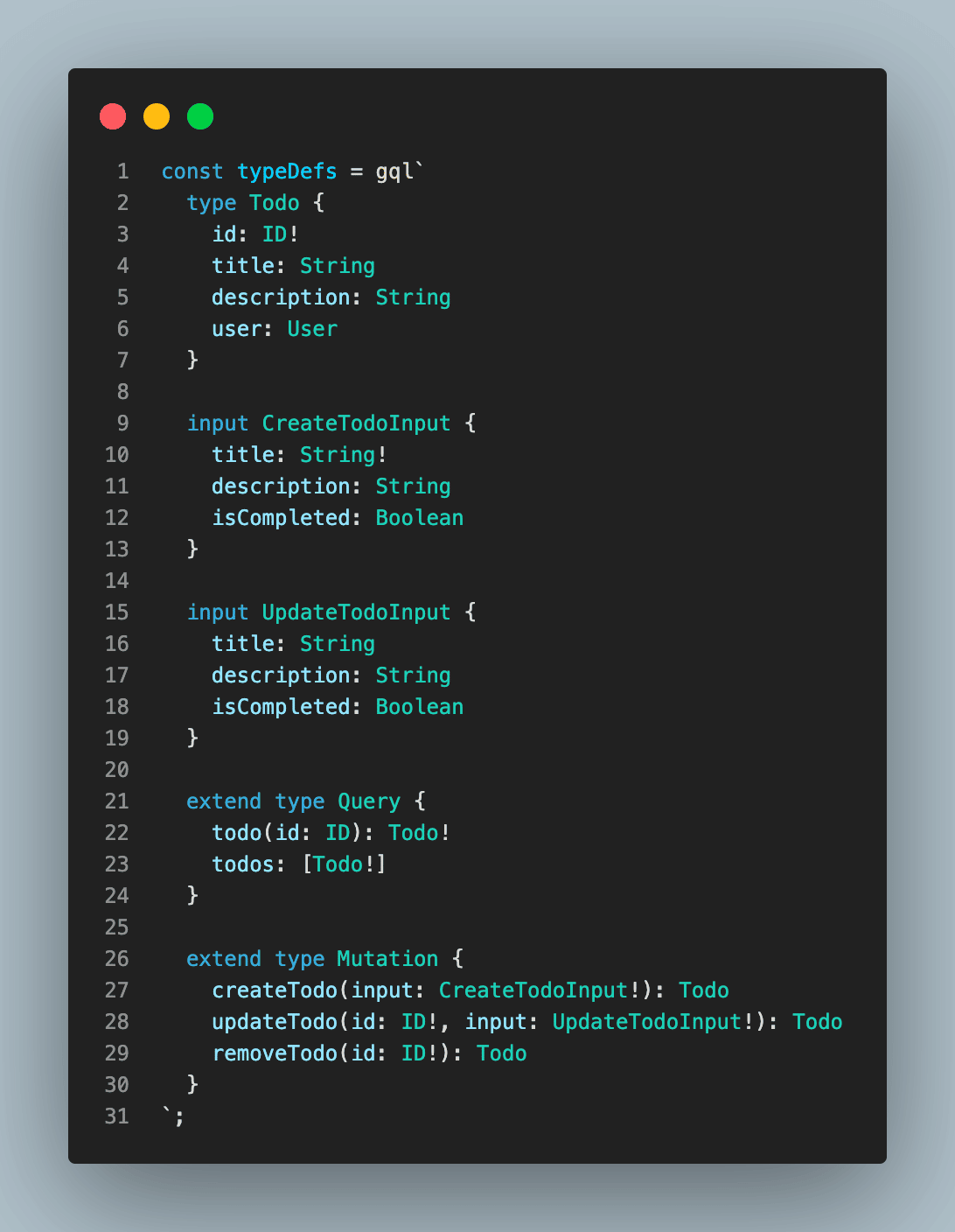
Step 2:
Subsequent, we created a resolver, which is used to retrieve the right information when a specific question or mutation is known as.
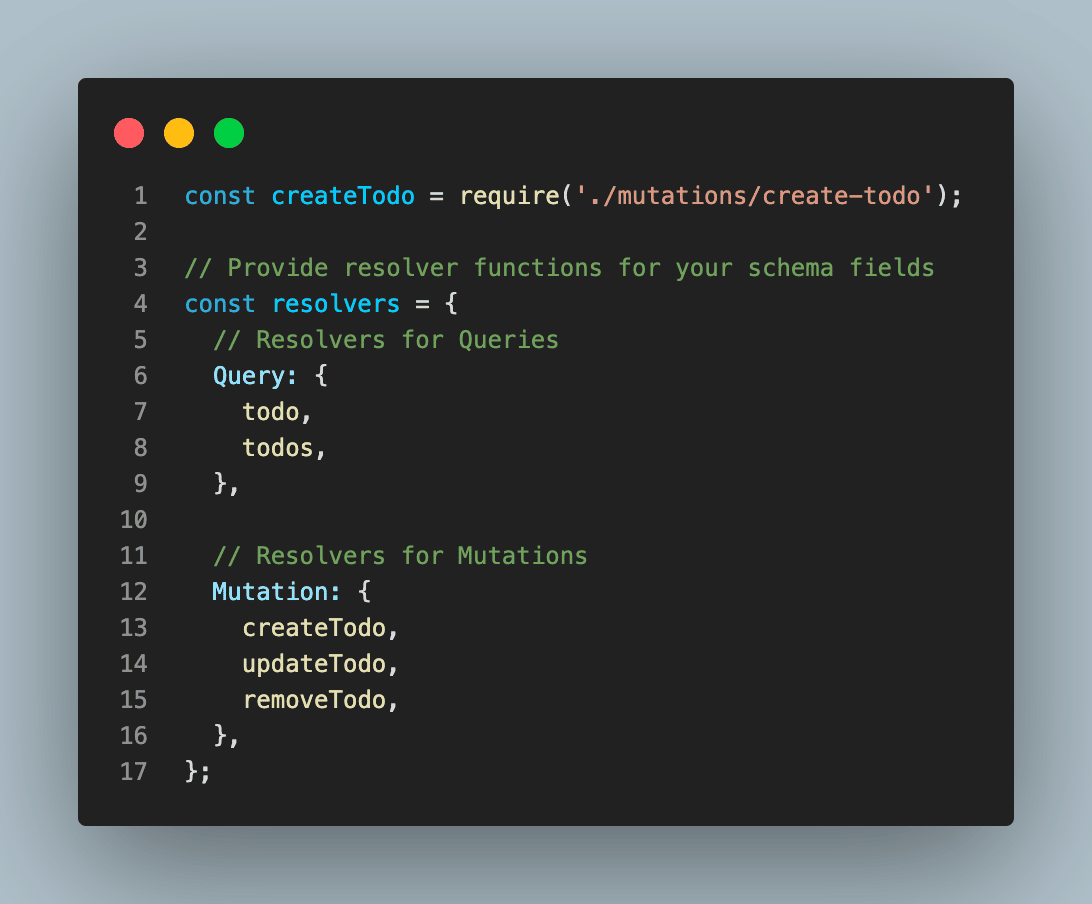
With the resolver perform in place, we will create particular person strategies for the enterprise logic and database manipulation as proven within the create-todo.js instance.
Create a create-user.js file within the <code>./mutations</code> folder and add the enterprise logic to create a brand new Todo in your database.
const fashions = require('../../../fashions');
module.exports = async (root, { enter }, context) => {
return fashions.todos.push({ ...enter });
};
The code snippet above is a simplified approach of making a brand new Todo in our database utilizing the Sequelize ORM. You may be taught extra about Sequelize and methods to set it up with Node.js.
You may comply with the identical step to create many schemas relying in your software or you possibly can clone the entire challenge from GitHub.
Subsequent, we’re going to arrange the server with Specific.js and run the newly created Todo software with GraphQL and Node.js
Organising and Working the Server
Lastly, we are going to arrange our server utilizing the apollo-server-express library we set up earlier and configure it.
The apollo-server-express is a straightforward wrapper of Apollo Server for Specific.js, It’s advisable as a result of it has been developed to slot in Specific.js growth.
Utilizing the examples we mentioned above, let’s configure the Specific.js server to work with the newly put in apollo-server-express.
Create a server.js file within the root listing and paste within the following code:
const categorical = require('categorical');
const { ApolloServer } = require('apollo-server-express');
const schema = require('./modules');
const app = categorical();
async perform startServer() {
const server = new ApolloServer({ schema });
await server.begin();
server.applyMiddleware({ app });
}
startServer();
app.hear({ port: 3000 }, () =>
console.log(`Server prepared at http://localhost:3000`)
);
Within the code above, you’ve efficiently created your first CRUD GraphQL server for Todos and Customers. You can begin your growth server and entry the playground utilizing http://localhost:3000/graphql. If every little thing is profitable, you ought to be offered with the display screen beneath:
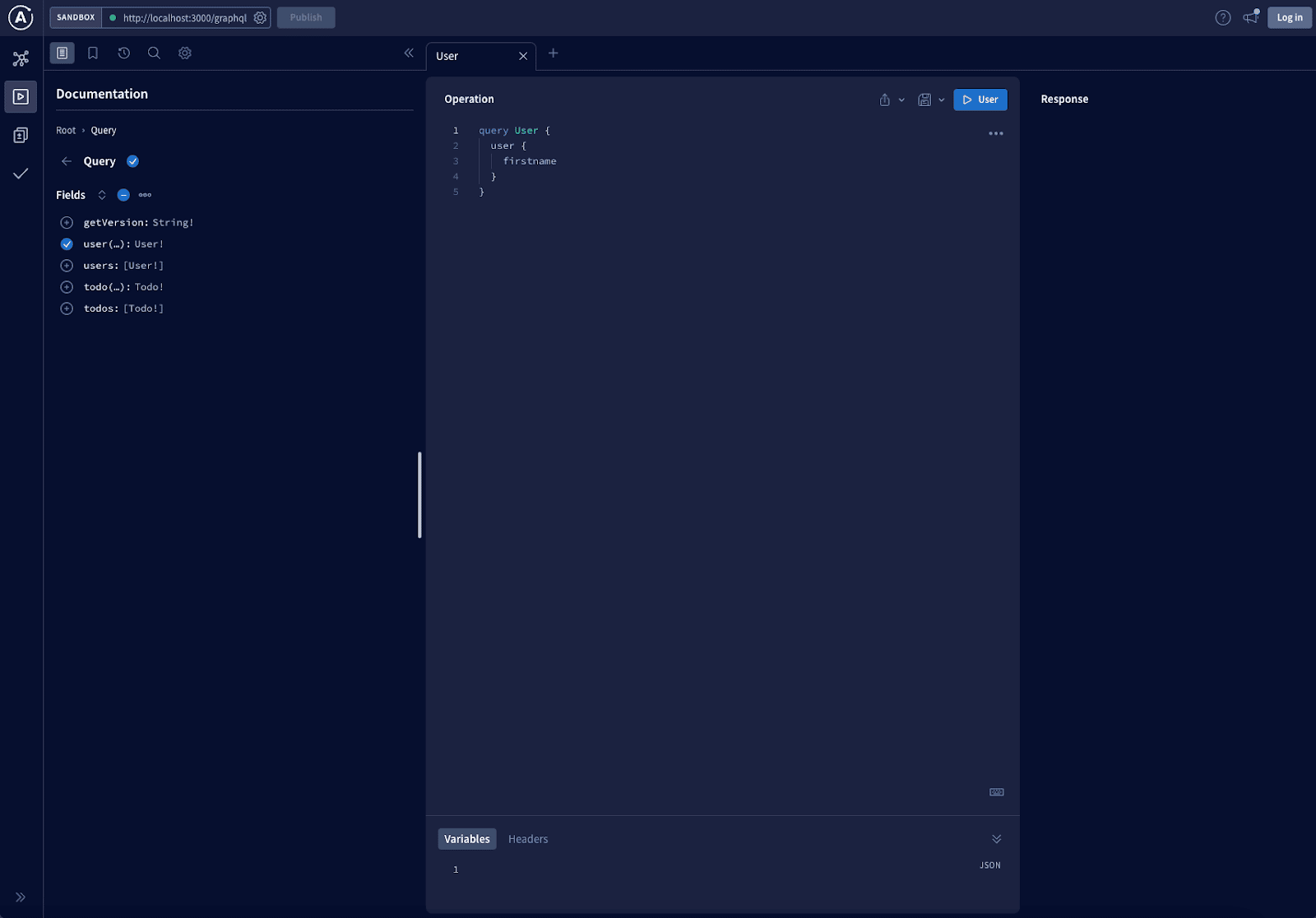
Abstract
GraphQL is trendy expertise supported by Fb that simplifies the tedious work concerned in creating large-scale APIs with RESTful architectural patterns.
This information has elucidated GraphQL and demonstrated methods to develop your first GraphQL API with Specific.js.
Tell us what you construct utilizing GraphQL.
Get all of your purposes, databases and WordPress websites on-line and below one roof. Our feature-packed, high-performance cloud platform contains:
- Straightforward setup and administration within the MyKinsta dashboard
- 24/7 knowledgeable help
- One of the best Google Cloud Platform {hardware} and community, powered by Kubernetes for max scalability
- An enterprise-level Cloudflare integration for pace and safety
- World viewers attain with as much as 35 information facilities and 275+ PoPs worldwide
Check it your self with $20 off your first month of Utility Internet hosting or Database Internet hosting. Discover our plans or speak to gross sales to search out your greatest match.