So that you’re a JavaScript whiz who can construct just about something — however can everybody use it? On this article, we’ll have a look at some methods to make wealthy, accessible JavaScript interfaces. We’ll illustrate our methods by constructing a easy Sudoku puzzle that works for everybody, even those that are blind or partially sighted.
Desk of contents
Planning for accessibility from the beginning
Retrofitting accessibility to an interface may be troublesome and time-consuming; you’ll at all times get higher outcomes for those who plan for accessibility proper from the beginning. You’ll have heard of the acronym POUR which is an effective way to method planning for accessibility.
POUR stands for:
- Perceivable: All customers ought to have the ability to understand the interface. Visible parts ought to have equal textual content options, and colour schemes needs to be pleasant to folks with low-color imaginative and prescient
- Operable: All customers ought to have the ability to function the interface. Be sure that every part may be operated with no mouse and that the contact or click on targets are giant sufficient for all customers
- Comprehensible: The interface needs to be as easy and as intuitive as attainable
- Strong: The interface ought to work throughout a full vary of present browsers and assistive applied sciences. This will likely imply utilizing solely applied sciences which might be nicely established somewhat than those who supply the “newest and best” options
For a grid-based recreation like Sudoku, the best problem with regard to accessibility is making the interface comprehensible. Sudoku requires that gamers know which numbers are entered into every cell of the grid. To be accessible, the Sudoku recreation might want to convey this data to customers who could not have the ability to see the gameboard.
A basic nine-digit Sudoku puzzle with 81 cells is manner too large for most individuals to have the ability to deal with if the board isn’t seen. So, my first determination is to simplify issues and make a four-digit Sudoku with 16 cells as a substitute.
Subsequent, there must be a constant method to describe the gameboard — a notation system. My first intuition is to make use of a easy XY coordinate system, like in chess. Nevertheless, a Sudoku grid is extra advanced than a chess board, it’s actually a set of grids inside a bigger grid.
Right here’s the notation system that I settled on:
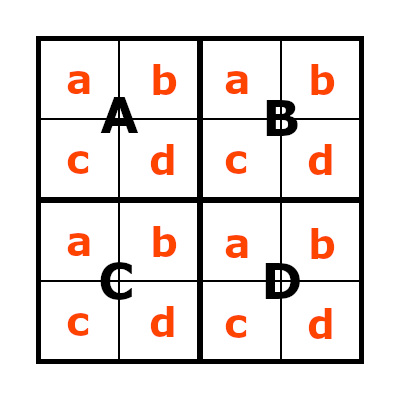
The gameboard consists of 4 squares organized in a two-by-two grid.
The squares within the high row are designated as sq. A and sq. B. The squares within the backside row are designated as sq. C and sq. D.
Every sq. accommodates 4 cells organized in a two-by-two grid. The cells within the high row of every sq. are designated as cell a and cell b. The cells within the backside row are designated as cell c and cell d.
Extra nice articles from LogRocket:
This notation can describe any cell on the board and conveys the positional data a participant wants to unravel the puzzle. So, if we incorporate mechanisms to announce a display screen reader consumer’s present place on the board and record the numbers within the corresponding row, column, and sq., the sport needs to be comprehensible.
Constructing with POUR ideas in thoughts
Now it’s time to begin constructing, maintaining the POUR ideas in thoughts. A key tactic in constructing accessible interfaces is to keep away from reinventing the wheel. If a long-established customary HTML management is on the market to do one thing, then use it in line with its specification, making use of CSS to create any desired visible results.
This miniature Sudoku consists of 16 containers organized in a grid, every able to holding a single quantity. So, I used 16 <enter kind="quantity>
parts. These parts are actually designed for this job, plus they’ve a readonly
attribute to mark the mounted worth cells that should be included to make the Sudoku solvable.
I used <div>
tags to group the cells into squares and a CSS Grid Format to arrange issues. Utilizing a CSS Grid Format as a substitute of an HTML <desk>
permits me to order the cells within the code in line with my notation system (Aa, Ab, Ac, Advert, Ba, Bb, and many others.). The tab order of a web page coordinates with its underlying construction, so it will assist preserve the interface comprehensible when a keyboard consumer strikes from one <enter>
to a different utilizing the tab key.
<div class="board"> <div class="sq."> <enter kind="quantity" min="0" max="4" size="1" worth=""> <enter kind="quantity" min="0" max="4" worth="2" readonly> <enter kind="quantity" min="0" max="4" worth=""> <enter kind="quantity" min="0" max="4" worth=""> </div> <div class="sq."> <enter kind="quantity" min="0" max="4" worth="3" readonly> <enter kind="quantity" min="0" max="4" worth=""> <enter kind="quantity" min="0" max="4" worth=""> <enter kind="quantity" min="0" max="4" worth="1" readonly> </div> <div class="sq."> <enter kind="quantity" min="0" max="4" worth="2" readonly> <enter kind="quantity" min="0" max="4" worth=""> <enter kind="quantity" min="0" max="4" worth=""> <enter kind="quantity" min="0" max="4" worth="4" readonly> </div> <div class="sq."> <enter kind="quantity" min="0" max="4" worth=""> <enter kind="quantity" min="0" max="4" worth=""> <enter kind="quantity" min="0" max="4" worth="1" readonly> <enter kind="quantity" min="0" max="4" worth=""> </div> </div>
*, *:earlier than, *:after { box-sizing: border-box; } physique { font-size: 1.25rem; line-height: 1.35; } .board { width: 40vh; max-width: 90vw; top: 40vh; max-height: 90vw; padding: 0; border: 2px stable black; show: grid; grid-template: repeat(2,1fr) / repeat(2,1fr); } .sq. { width: 100%; top: 100%; margin: 0; padding: 0; border: 2px stable black; show: grid; grid-template: repeat(2,1fr) / repeat(2,1fr); } .sq. enter { show: block; colour: black; width: 100%; top: 100%; margin: 0; padding: 0; text-align: middle; font-size: 7vh; border: 1px stable black; -moz-appearance: textfield; } /* Cover HTML5 Up and Down arrows. */ .sq. enter::-webkit-outer-spin-button, .sq. enter::-webkit-inner-spin-button { -webkit-appearance: none; margin: 0; }
Choosing colour and measurement
Some frequent issues that internet interfaces face with regard to accessibility are textual content that’s too small and UI colours that don’t present sufficient distinction to be simply seen.
By way of measurement, a superb rule of thumb is for textual content to be displayed with an efficient font measurement no smaller than 16 CSS pixels (which is completely different from machine pixels). For the Sudoku recreation, I’ve gone with a minimal of 20 pixels (specified as 1.25rem
the place 1rem
is 16 pixels), and in addition elevated the usual line-height
to 1.35 to area issues out a bit extra and make them simpler to learn.
I’ve specified the gameboard’s general measurement utilizing vh
models (1vh
is 1% of the viewport’s top) to attempt to make it as giant as attainable whereas additionally maintaining the sport controls seen throughout the identical viewport.
The sport predominantly makes use of black and white to maximise distinction. Nevertheless, I additionally want to point out which cells are read-only and I need to use colour to point out the place the keyboard focus at the moment is throughout the gameboard and (optionally) spotlight appropriate and incorrect cells.
This brings up one other frequent concern — coping with low colour imaginative and prescient. It appears instinctive to make use of inexperienced to focus on appropriate cells and purple to focus on incorrect cells, however purple and inexperienced are a really poor alternative for almost all of individuals with low colour imaginative and prescient.
As a substitute, I opted to make use of sky blue to designate appropriate cells, reddish-purple to designate incorrect cells, and yellow to focus on place. The precise colour shades have been chosen from the Wong palette. For read-only (readonly
) cells, I used white textual content on a darkish grey background. All these colour combos additionally present adequate distinction, as measured utilizing the WebAIM Distinction Checker.
.appropriate { background-color: #56B4E9; } .incorrect { background-color: #CC79A7; } .sq. enter:focus { background-color: #F0E442; } .sq. enter:read-only { background-color: #333; colour: white; }
Incorporating extra help with WAI-ARIA
It might not operate but, however we now have one thing that appears like a miniature Sudoku recreation. Utilizing a mouse, we will simply level and click on to enter numbers in every cell of the grid, and it additionally works utilizing simply the keyboard.
We nonetheless want to have the ability to see the board so as to navigate by it, which is of no use for folks with restricted or no imaginative and prescient. Thankfully, the Internet Accessibility Initiative – Accessible Wealthy Web Purposes (WAI-ARIA) may also help incorporate extra help.
The aria-label
attribute is used so as to add further textual content to issues like kind parts which might be learn aloud by display screen reader software program when they’re centered on by a consumer. I’ve used this attribute so as to add the notation to every cell.
<div class="board"> <div aria-label="Sq. A" class="sq."> <enter aria-label="Sq. A, Cell A." kind="quantity" min="0" max="4" size="1" worth=""> <enter aria-label="Sq. A, Cell B. The worth of this cell is mounted." kind="quantity" min="0" max="4" worth="2" readonly> <enter aria-label="Sq. A, Cell C." kind="quantity" min="0" max="4" worth=""> <enter aria-label="Sq. A, Cell D." kind="quantity" min="0" max="4" worth=""> </div> ... </div>
I’m not required so as to add the worth of the cell to the aria-label
as the worth is learn out by default. However, I occur to know that some display screen readers don’t inform the consumer when an <enter>
factor is read-only, so I’ve included that data.
Including interactivity
Now it’s time to make the sport really do one thing.
We would like to have the ability to:
- Test if the gameboard is accomplished appropriately
- Present the total resolution to a participant who’s having bother finishing the puzzle
- Restart the sport from scratch
- Give gamers the choice of highlighting which cells are appropriate and that are incorrect
To attain these targets, we have to retailer the right reply for every cell someplace and add controls under the gameboard to set off every of the actions.
There are a number of methods we might retailer the right solutions. I’ve opted for including a information
attribute to every cell, like this:
<enter aria-label="Sq. A, Cell A." data-correct="1" kind="quantity" min="0" max="4" size="1" worth="">
The sport controls should be accessible, so I’ll apply the identical design ideas as earlier than and use inventory HTML within the type of <button>
and <enter kind="checkbox">
parts.
Every factor wants a novel id
in order that I can connect occasion listeners to the factor. I’ll use the usual <label>
factor to make sure that the spotlight toggle checkbox is accessible. I’ll additionally add an empty <div>
that I can use to show bulletins to gamers.
<div class="controls"> <label><enter id="spotlight" kind="checkbox"> HIGHLIGHT</label> <button id="examine">CHECK YOUR ANSWER</button> <button id="present">SHOW SOLUTION</button> <button id="restart">RESTART</button> </div> <div id="announcement-all-users"></div>
4 easy JavaScript capabilities are all that’s wanted to make the sport work on a fundamental degree:
let lang = "en"; const messages = { en: { boardCorrect: "Congratulations, you accomplished the Sudoku appropriately!", boardIncorrect: "Hmmm... Not fairly appropriate! Attempt once more. Test 'HIGHLIGHT' to see the place you went flawed." }, announcementAllUsersDisplayed: false } operate highlightCells(e){ doc.querySelectorAll('.sq. enter:read-write').forEach( (cell) => { // toggle appropriate and incorrect courses for every non read-only cell if(doc.querySelector('#spotlight').checked){ if(cell.worth === cell.dataset.appropriate){ cell.classList.add("appropriate"); cell.classList.take away("incorrect"); } else { cell.classList.add("incorrect"); cell.classList.take away("appropriate"); } } else { cell.classList.take away("appropriate","incorrect"); } } ); } doc.querySelector('#spotlight').addEventListener('change', highlightCells ); operate checkAnswer(e) { let allCorrect = true; doc.querySelectorAll('.sq. enter').forEach( (cell) => { // examine every cell to see whether it is appropriate if( cell.worth !== cell.dataset.appropriate){ allCorrect = false; } } ); // make normal announcement of success/failure if(allCorrect){ doc.querySelector('#announcement-all-users').innerHTML = messages[lang].boardCorrect; } else { doc.querySelector('#announcement-all-users').innerHTML = messages[lang].boardIncorrect; } messages.announcementAllUsersDisplayed = true; } doc.querySelector('#examine').addEventListener('click on', checkAnswer ); operate showSolution(e){ if(messages.announcementAllUsersDisplayed){// take away any current normal annoucements doc.querySelector('#announcement-all-users').innerHTML = ""; messages.announcementAllUsersDisplayed = false; } doc.querySelectorAll('.sq. enter').forEach( (cell) => { // set the worth of every cell to the right worth cell.worth = cell.dataset.appropriate; } ); } doc.querySelector('#present').addEventListener('click on', showSolution ); operate restartGame(e){ if(messages.announcementAllUsersDisplayed){ // take away any current normal annoucements doc.querySelector('#announcement-all-users').innerHTML = ""; messages.announcementAllUsersDisplayed = false; } doc.querySelectorAll('.sq. enter:read-write').forEach( (cell) => { // reset every non read-only cell to be empty if(!cell.classList.accommodates("readonly")){ cell.worth = ""; } } ); } doc.querySelector('#restart').addEventListener('click on', restartGame );
We now have a working Sudoku recreation that’s perceivable, operable, and sturdy (test it out on CodePen), however we nonetheless have a bit of labor to do to make it absolutely comprehensible.
Additionally, some updates could possibly be made to make the sport simpler to function. For instance, the highlightCells
operate solely works on a visible degree. To be helpful for display screen reader customers we have to broaden it somewhat. Every <enter>
factor of the gameboard already has an aria-label
attribute, so we will manipulate that so as to add or take away the right or incorrect data when the spotlight checkbox is toggled:
let lang = "en"; const messages = { en: { cellCorrect: "This cell is appropriate", cellIncorrect: "This cell is inaccurate", boardCorrect: "Congratulations, you accomplished the Sudoku appropriately!", boardIncorrect: "Hmmm... Not fairly appropriate! Attempt once more. Test 'HIGHLIGHT' to see the place you went flawed." }, announcementAllUsersDisplayed: false } operate highlightCells(e){ if(doc.querySelector('#spotlight').checked){ doc.querySelector('#highlight-help').classList.take away('v-hidden'); } else { doc.querySelector('#highlight-help').classList.add('v-hidden'); } doc.querySelectorAll('.sq. enter:read-write').forEach( (cell) => { let ariaLabelSplit = cell.ariaLabel.cut up('.'); if(doc.querySelector('#spotlight').checked){ if(cell.worth === cell.dataset.appropriate){ cell.classList.add("appropriate"); cell.classList.take away("incorrect"); cell.ariaLabel = ariaLabelSplit[0] + ". " + messages[lang].cellCorrect; } else { cell.classList.add("incorrect"); cell.classList.take away("appropriate"); cell.ariaLabel = ariaLabelSplit[0] + ". " + messages[lang].cellIncorrect; } } else { cell.classList.take away("appropriate","incorrect"); cell.ariaLabel = ariaLabelSplit[0]; } } ); } doc.querySelector('#spotlight').addEventListener('change', highlightCells );
The spotlight operate ought to now work for everybody with solely minimal changes. One large piece of the puzzle remains to be lacking although (for those who’ll pardon the pun).
The Sudoku recreation is solved when every row, column, and sq. accommodates the numbers one by 4 exactly as soon as. So, display screen reader customers want a fast and straightforward non-visual method to discover out which numbers are in a specific row, column, or sq.. For the primary time on this venture, we have to construct further performance into the sport for simply this consumer group.
I made a decision to deal with this by including a 'learn'
operate to the sport, triggered by a keyboard shortcut. That is the place the aria-live
attribute is available in. If we add this attribute to a component (for instance, a easy <div>
), then when the content material of that factor modifications it’s learn out by the display screen reader software program.
This operate can be utilized to make bulletins to display screen reader customers by altering the contents of the factor with JavaScript. We’ll add an aria-live
attribute to the present normal announcement factor and one other to the factor for messages meant solely for display screen reader customers. This final message could possibly be positioned offscreen within the ultimate model of the sport.
<div id="announcement-sr-only" aria-live="well mannered"></div> <div id="announcement-all-users" aria-live="well mannered"></div>
The "well mannered"
worth signifies that when the contents of the reside factor change, the display screen reader will end what it’s at the moment studying out earlier than studying the announcement. This works nicely for Sudoku, but when we would have liked to alert a consumer to one thing extra urgent we might set aria-live="assertive"
which might end in our announcement being learn out right away.
Right here’s how the studying operate works for our Sudoku recreation:
- Whereas the consumer’s keyboard focus is in one of many recreation’s cells, they press R (learn) adopted by both R (row), C (column), or S (sq.)
- A abstract of the contents of the present row, column, or sq. is displayed within the
announcement-sr-only
factor
Keyboard shortcuts is usually a little bit of a minefield with display screen readers, as many keys have already got shortcuts assigned, and people assignments can change relying on the display screen reader’s mode. So use keyboard shortcuts sparingly, solely connect the listener to parts the place it’s wanted, and do a number of testing!
To permit the studying operate to work, I added an id
attribute to assist me iterate by the cells, a data-id
factor for iterating by squares, and a further class to the readonly
cells so I might simply goal these cells.
<div class="board"> <div data-id="A" class="sq."> <enter id="11" data-id="A" aria-label="Sq. A, Cell A." data-correct="1" kind="quantity" min="0" max="4" size="1" worth=""> <enter id="12" data-id="B" aria-label="Sq. A, Cell B. The worth of this cell is mounted." data-correct="2" kind="quantity" min="0" max="4" worth="2" class="readonly"> <enter id="21" data-id="C" aria-label="Sq. A, Cell C." data-correct="4" kind="quantity" min="0" max="4" worth=""> <enter id="22" data-id="D" aria-label="Sq. A, Cell D." data-correct="3" kind="quantity" min="0" max="4" worth=""> </div> <div data-id="B" class="sq."> <enter id="13" data-id="A" aria-label="Sq. B, Cell A. The worth of this cell is mounted." data-correct="3" kind="quantity" min="0" max="4" worth="3" class="readonly"> <enter id="14" data-id="B" aria-label="Sq. B, Cell B." data-correct="4" kind="quantity" min="0" max="4" worth=""> <enter id="23" data-id="C" aria-label="Sq. B, Cell C." data-correct="2" kind="quantity" min="0" max="4" worth=""> <enter id="24" data-id="D" aria-label="Sq. B, Cell D. The worth of this cell is mounted." data-correct="1" kind="quantity" min="0" max="4" worth="1" class="readonly"> </div> ... </div>
The under code demonstrates how I arrange the interactivity for studying rows. The processes for studying columns and squares are comparable.
const measurement = 4; let lang = "en"; const messages = { en: { cellValue: "The worth of this cell is ", cellEmpty: "This cell is empty.", cellEmptyShort: "Empty", cellCorrect: "This cell is appropriate", cellCorrectShort: "appropriate", cellIncorrect: "This cell is inaccurate", cellIncorrectShort: "incorrect", cellFixedShort: "mounted worth", boardCorrect: "Congratulations, you accomplished the Sudoku appropriately!", boardIncorrect: "Hmmm... Not fairly appropriate! Attempt once more. Test 'HIGHLIGHT' to see the place you went flawed.", studying: "Studying", currentRow: "present row", currentColumn: "present column", currentSquare: "sq." }, announcementScreenreaderOnlyDisplayed: false, announcementAllUsersDisplayed: false } let keyboardNavCapture = 0; let capturedKeys = ""; doc.querySelector('.board').addEventListener('keydown', (e) => { // The keyboard focus must be throughout the gameboard for the shortcut to operate if (e.defaultPrevented) { return; // Do nothing if the occasion was already processed. This helps avoids interfering with current display screen reader keyboard shortcuts } if(e.key === "Escape"){ // enable the consumer to cancel {a partially} captured motion keyboardNavCapture = 0; capturedKeys = ""; } else if(keyboardNavCapture === 0){ if(e.key === "r" || e.key === "R"){ // READ motion. Seize the following key press to find out what to learn keyboardNavCapture = 1; capturedKeys = "R"; e.preventDefault(); } } else { capturedKeys += e.key.toUpperCase(); keyboardNavCapture = keyboardNavCapture - 1; e.preventDefault(); if(keyboardNavCapture == 0){ let actionType = capturedKeys[0]; let actionDetails = capturedKeys.substring(1); capturedKeys = ""; if(actionType === "R"){ // READ motion let activeCellId = parseInt(doc.activeElement.id); if( (actionDetails === "R" || actionDetails === "C" || actionDetails === "S") && !Quantity.isNaN(activeCellId) ){ let row = parseInt(doc.activeElement.id[0]) * 10; let column = parseInt(doc.activeElement.id[1]); let cellValue = ""; let announcementScreenreaderOnly = messages[lang].studying + " "; swap (actionDetails){ case "R": // Learn out the present row announcementScreenreaderOnly += messages[lang].currentRow + ":"; for (let col = 1; col < (measurement + 1); col++) { cellValue = doc.getElementById("" + (row + col)).worth; if(cellValue === ""){ cellValue = messages[lang].cellEmptyShort; } else if(doc.getElementById("" + (row + col)).classList.accommodates('readonly')){ cellValue += " (" + messages[lang].cellFixedShort + ")"; } else if(doc.querySelector('#spotlight').checked){ if(cellValue === doc.getElementById("" + (row + col)).dataset.appropriate){ cellValue += " (" + messages[lang].cellCorrectShort + ")"; } else { cellValue += " (" + messages[lang].cellIncorrectShort + ")"; } } announcementScreenreaderOnly += " " + cellValue; if(col < measurement){ announcementScreenreaderOnly += ","; } } break; // swap assertion continues for studying columns C and squares S } doc.querySelector("#announcement-sr-only").innerText = announcementScreenreaderOnly; } } } } });
Having constructed the fundamentals for keyboard navigation, I additionally added keyboard shortcuts for every of the sport controls. These are triggered by the C key adopted by a personality representing the management. For instance, CR restarts the sport and CS reveals the answer.
I additionally added a “the place am I?” operate (triggered by the W key), and a “go” operate (triggered by the G key adopted by the notation for a particular cell) to permit fast journey across the gameboard. You possibly can see how these are carried out within the ultimate CodePen.
Take a look at, check, check!
Theoretically, we must always now have a completely accessible Sudoku puzzle! As ever although, apply and principle don’t at all times match up, so it’s vital to check the sport in a number of methods.
As a primary check, at all times examine that the interface may be absolutely operated with no mouse. Our recreation passes this with flying colours! We will use the tab key or the keyboard shortcuts to maneuver across the grid, and the sport controls can all be triggered utilizing the keyboard too.
N.B., any ultimate model of the sport will want adequate documentation to tell customers about any keyboard shortcuts
With the essential keyboard-only check handed, it’s time to maneuver on to testing with a display screen reader.
NVDA on a Home windows PC is a stable alternative for developer testing, and it’s free. You must also use JAWS in case you have the funds to entry a duplicate. WebAIM’s overview covers the fundamentals wanted to make use of NVDA for testing. In the event you’re on a Mac and have the VoiceOver display screen reader out there, WebAIM has an ideal intro for utilizing VoiceOver to guage Internet Accessibility.
N.B., utilizing a display screen reader on CodePen is somewhat difficult; you’ll get higher outcomes exporting the code and putting it by yourself server for testing
I examined the Sudoku recreation utilizing NVDA and nearly every part labored nicely. Nevertheless, there was one sudden merchandise. It seems that when NVDA enters a readonly
cell it switches out of 'focus'
mode and again into 'browse'
mode. This mode swap kills the keyboard shortcuts, making the sport extremely nonintuitive to make use of. Though discovering this error was irritating, it neatly demonstrates the necessity for testing.
I briefly tried utilizing the disabled
attribute as a substitute, however NVDA won’t even place the keyboard deal with disabled cells in order that didn’t work. As a substitute, I reworked the code to utterly take away the readonly
attributes, after which I used JavaScript to implement read-only conduct on these cells.
In an excellent world, our Sudoku recreation ought to now be examined throughout different display screen readers and suggestions needs to be gained from actual customers. It’s best to at all times embrace this ultimate step in your improvement course of at any time when attainable.
Conclusion
Every thing on the internet may be made accessible. By planning for accessibility from the beginning and following the POUR ideas all through your design and construct you may create wealthy JavaScript interfaces that everybody can use.
Take a look at the ultimate code for your self on CodePen or on this standalone web page (for display screen reader testing). I’ve additionally included a number of further controls in an effort to simulate display screen reader output with out utilizing NVDA your self, and get an concept of how the sport may work for those who had partial sight or full lack of imaginative and prescient. Let me know what you assume!
LogRocket: Debug JavaScript errors extra simply by understanding the context
Debugging code is at all times a tedious job. However the extra you perceive your errors the better it’s to repair them.
LogRocket means that you can perceive these errors in new and distinctive methods. Our frontend monitoring resolution tracks consumer engagement along with your JavaScript frontends to provide the means to seek out out precisely what the consumer did that led to an error.
LogRocket information console logs, web page load instances, stacktraces, gradual community requests/responses with headers + our bodies, browser metadata, and customized logs. Understanding the affect of your JavaScript code won’t ever be simpler!