Most React functions talk with distant knowledge sources to persist and retrieve knowledge data. Net software improvement groups these days have a tendency to make use of REST and GraphQL-like communication patterns to implement their distant knowledge supply interfaces. Then, frontend improvement groups must make community requests with varied libraries by means of their React apps to sync knowledge between the consumer facet and the server facet.
For speaking with RESTful providers, the best means is to make use of the inbuilt Fetch API or a library like Axios within the part to mount state-like occasions. Then, it’s a must to write extra logic to implement loading state UI enhancements. Lastly, to make your app much more user-friendly and optimized by way of knowledge caching, deduplicated API queries, and pre-fetching, you could have to write down extra code than your client-side enterprise logic!
That is the place libraries like SWR and TanStack Question — previously React Question — might help you sync your knowledge supply’s state along with your React app’s state by way of caching, pre-fetching, question deduplication, and varied different usability options.
On this article, I’ll examine the options of SWR and the TanStack Question library with a sensible instance challenge. Right here’s what we’ll cowl:
What’s React SWR?
SWR is an open supply, light-weight, and TypeScript-ready library that provides a number of Hooks for fetching knowledge in React with caching. The abbreviation “SWR” stands for State Whereas Re-validate, a generic caching precept from HTTP RFC 5861.
React SWR was first launched in 2019 by way of its v0.1.2 public launch.
Highlighted options
This library gives the next highlighted options:
Characteristic | Description |
---|---|
Light-weight measurement and excessive efficiency | In keeping with BundlePhobia, the SWR library weighs ~4.2 kilobytes when gzipped. The SWR improvement workforce focuses on efficiency and being light-weight with the tree-shaking bundling technique |
Minimal, configurable, and re-usable API | SWR additionally focuses on providing a minimal, developer-friendly API for React builders that gives performance-friendly options. You possibly can implement many of the belongings you want with a single Hook, useSWR .
Despite the fact that the API is minimal, it enables you to tweak the caching system and conduct with a world configuration and lots of Hook choices. |
Inbuilt options for builders and customers | SWR helps paginated requests and offers the useSWRInfinite Hook to implement infinite loading. It additionally works with the React Suspense API, SSG, and SSR, and gives pre-fetching, re-validation on focus, and community standing re-fetching, like usability enhancements for app customers. |
Utilizing React SWR
Now that we have now an summary of SWR’s options for optimized knowledge fetching in React, let’s create a pattern app with SWR and consider it to seek out comparability factors with TanStack Question.
We will mock our API backend with delayed guarantees on the consumer facet to attempt SWR, however that strategy doesn’t give an actual knowledge fetching expertise. Let’s as an alternative create a easy RESTful API with Node.js. We will create a RESTful API server in seconds with the json-server
package deal.
First, set up the json-server
package deal globally:
npm set up -g json-server # --- or --- yarn world add json-server
Subsequent, add the next content material to a brand new file named db.json
:
{ "merchandise": [ { "id": 1, "name": "ProX Watch", "price": 20 }, { "id": 2, "name": "Magic Pencil", "price": 2 }, { "id": 3, "name": "RevPro Wallet", "price": 15 }, { "id": 4, "name": "Rice Cooker", "price": 25 }, { "id": 5, "name": "CookToday Oven", "price": 10 } ] }
Subsequent, run the next command to begin a RESTful CRUD server primarily based on the db.json
file:
json-server --watch --port=5000 --delay=1000 db.json
Now, we will entry our CRUD API by way of http://localhost:5000/merchandise
. You possibly can check it with Postman if you need. In our instance, we added a 1000ms delay to simulate community latency.
Let’s create a brand new React app and fetch knowledge by way of SWR. In case you are already an SWR consumer otherwise you’ve experimented with SWR earlier than, you possibly can test the whole challenge in this GitHub repository and proceed to the TanStack Question part under.
Create a brand new React app, as normal:
npx create-react-app react-swr-example cd react-swr-example
Putting in the package deal
Subsequent, set up the swr
package deal with the next command:
Extra nice articles from LogRocket:
npm set up swr # --- or --- yarn add swr
We’ll use Axios on this tutorial, so set up it, too, with the next command. You should utilize any HTTP request library or the inbuilt fetch
, since SWR expects simply guarantees.
npm set up axios # --- or --- yarn add axios
Implementing knowledge fetchers and customized Hooks
We’ll consider SWR by making a easy product administration app that lists some merchandise and allows you to add new ones. First, we have to retailer our native mock API’s base URL within the .env
file. Create a brand new file named .env
and add the next content material:
REACT_APP_API_BASE_URL = "http://localhost:5000"
Subsequent, use the bottom URL within the Axios world configuration by including the next content material to the index.js
file:
import React from 'react'; import ReactDOM from 'react-dom/consumer'; import axios from 'axios'; import './index.css'; import App from './App'; axios.defaults.baseURL = course of.env.REACT_APP_API_BASE_URL; const root = ReactDOM.createRoot(doc.getElementById('root')); root.render( <App /> );
We’ll hold all app elements in our App.js
file to take care of the tutorial’s simplicity. Clear all the things in your App.js
file and add the next imports:
import React, { useState } from 'react'; import useSWR from 'swr'; import axios from 'axios'; import './App.css';
Right here, we import the useSWR
Hook from swr
to retrieve cached knowledge data, somewhat than calling Axios capabilities immediately.
For fetching knowledge with out RESTful URL parameters, we usually want to offer two parameters to the useSWR
Hook: a novel key (often the URL) and a fetcher perform, which is a JavaScript perform that returns asynchronous knowledge.
Add the next code that features a fetcher:
perform fetcher(url) { return axios.get(url).then(res => res.knowledge); } async perform addProduct(product) { let response = await axios.put up('/merchandise', product); return response.knowledge; }
Right here, the fetcher
perform asynchronously returns knowledge by way of Axios and the addProduct
perform equally posts product knowledge and returns the newly created product.
Now, we will use the useSWR(‘/merchandise’, fetcher)
assertion in purposeful elements to fetch cached merchandise, however SWR builders suggest utilizing re-usable customized Hooks. Add the next Hook to the App.js
file:
perform useProducts() { const { knowledge, error, mutate } = useSWR('/merchandise', fetcher); return { merchandise: knowledge, isLoading: !knowledge, isError: !!error, mutate }; }
Our useProducts
customized Hook outputs the next props:
merchandise
: An array of merchandise after fetching knowledge from the API; it turns intoundefined
if no knowledge is offered from the APIisLoading
: Loading indicator primarily based on API knowledgeisError
: A boolean worth to point loading errorsmutate
: A perform to replace cached knowledge that displays on UI immediately
Utilizing SWR for knowledge fetching
Now we will use the useProducts
knowledge Hook to replace the UI from backend knowledge. Create the Merchandise
part to record out all the obtainable merchandise:
perform Merchandise() { const { merchandise, isLoading, isError } = useProducts(); if(isError) return ( <div>Unable to fetch merchandise.</div> ); if(isLoading) return ( <div>Loading merchandise...</div> ); return ( merchandise.map((product) => ( <div key={product.id} className="product-item"> <div>{product.identify}</div> <div>${product.value}</div> </div> )) ); }
The Merchandise
part renders conditionally primarily based on the useProducts
Hook props. For those who use this Hook a number of occasions in lots of elements, SWR will provoke just one HTTP request, per the request deduplication function, then, the fetched knowledge might be shared with all elements for the rendering course of by way of the useProducts
Hook.
Mutating cached knowledge and invalidating requests
Create a part referred to as AddProduct
and implement a means so as to add a brand new product with the next code:
perform AddProduct({ goToList }) { const { merchandise, mutate } = useProducts(); const [product, setProduct] = useState({ id: merchandise.size + 1, identify: '', value: null }); const [disabled, setDisabled] = useState(true); async perform handleAdd() { goToList(); mutate(async () => { return [...products, await addProduct(product)] }, { optimisticData: [...products, product], rollbackOnError: true, revalidate: false } ); } perform handleFieldUpdate(e) { const factor = e.goal; const worth = factor.kind === 'quantity' ? parseInt(factor.worth) : factor.worth; const nextProduct = {...product, [element.name]: worth}; setProduct(nextProduct); setDisabled(!nextProduct.identify || !nextProduct.value); } return( <div className="product-form"> <enter kind="textual content" identify="identify" placeholder="Title" autoFocus onChange={handleFieldUpdate}/> <enter kind="quantity" identify="value" min="1" placeholder="Worth" onChange={handleFieldUpdate}/> <button onClick={handleAdd} disabled={disabled}>Add</button> </div> ); }
Learn the mutate
perform name rigorously:
mutate(async () => { return [...products, await addProduct(product)] }, { optimisticData: [...products, product], rollbackOnError: true, revalidate: false } );
Right here, we ask SWR to replace rendered merchandise immediately with the optimisticData
possibility; then, we will use the addProduct
perform name to insert the desired factor into the database. We will additionally return the up to date merchandise record from the async perform as a result of our SWR mutation expects up to date knowledge data from the async perform’s return worth.
As the ultimate step, add the exported App
part and full the implementation:
perform App() { const [ mode, setMode ] = useState('record'); return ( <> <div className="menu-bar"> <div onClick={() => { setMode('record') }} className={mode === 'record' ? 'chosen' : ''}>All merchandise</div> <div onClick={() => { setMode('add') }} className={mode === 'add' ? 'chosen' : ''}>Add product</div> </div> <div className="wrapper"> { mode === 'record' ? <Merchandise/> : <AddProduct goToList={() => setMode('record')}/> } </div> </> ); } export default App;
Now run the applying:
npm begin # --- or --- yarn begin
First, examine how SWR caches the ProductList
part’s knowledge — you will notice the loading textual content solely as soon as. Later, you’ll obtain the cached content material.
Have a look at the next preview:
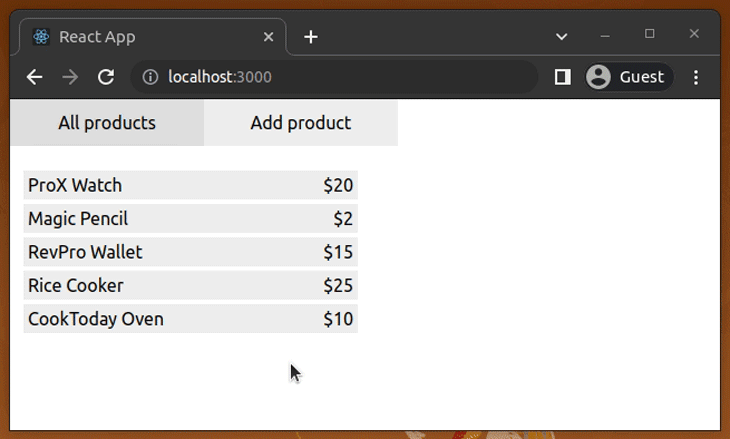
Subsequent, discover how SWR improves usability by immediately manipulating the rendered content material earlier than updating and re-fetching knowledge within the background inside the AddProduct
part. Add a brand new product, and see that the information report is instantly rendered, as proven under:
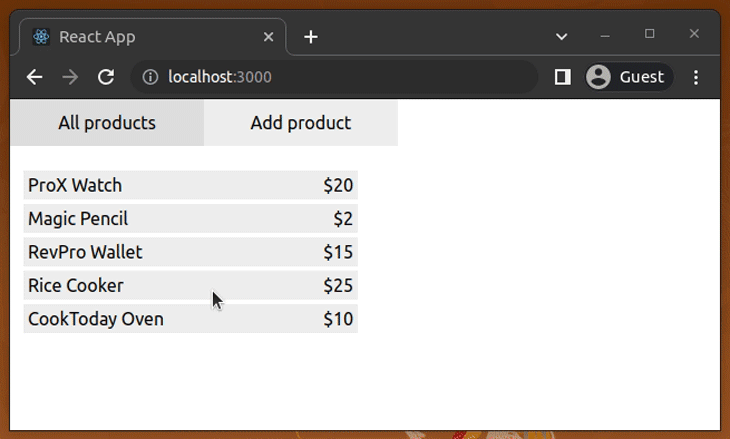
Lastly, SWR comes with some extra options, like re-validation on focus and examine the community tab to see community calls:
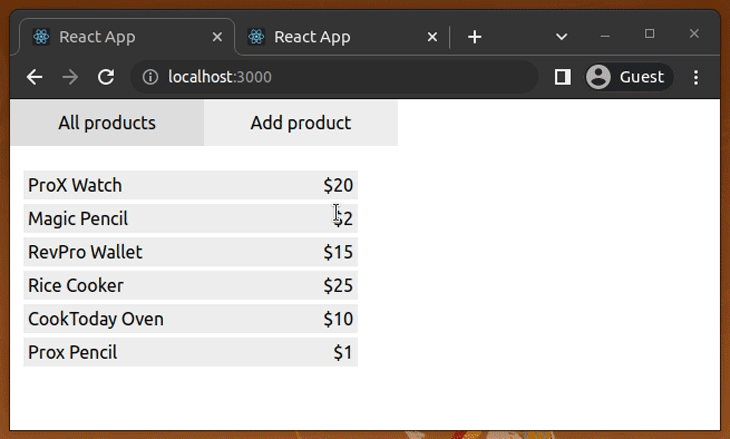
What’s TanStack Question?
TanStack Question is one other open supply, full-featured, TypeScript-ready library that provides an API for knowledge fetching and caching in React apps. It implements the library’s agnostic core logic in a separate inner package deal and gives the React Question adaptor package deal particularly for React.
TanStack Question for React offers Hooks, courses, and an official, devoted GUI-based developer instrument for syncing consumer state and server state in React apps. Equally, the event workforce plans to supply official adaptor packages for different frontend libraries, i.e., TanStack Vue Question, Svelte Question, and many others.
TanStack Question was first launched in 2014 by way of its v0.0.6 public launch, about one 12 months after React’s preliminary launch.
Highlighted options
This library gives the next highlighted options:
Characteristic | Description |
---|---|
Batteries-included, framework-like expertise | TanStack Question gives a framework-like expertise for React builders, with a devoted developer instrument, devoted Hooks for each particular process, OOP courses for higher code group, and JavaScript-props-based occasion handlers. |
Detailed, configurable, and re-usable API | TanStack Question strives to offer an in depth, configurable, and full-featured API for fetching and caching distant knowledge inside React apps. It gives a number of hooks and courses from its API core for higher code group. |
Inbuilt options for builders and customers | TanStack Question helps paginated requests and offers the useInfiniteQuery Hook to implement infinite loading.
It additionally gives a React Suspense API, SSG, and SSR help for builders — pre-fetching, re-validation on focus, and community standing re-fetching like usability enhancements for app customers. |
Utilizing TanStack Question
Now that we’ve reviewed the options that TanStack Question gives for optimized knowledge fetching in React, let’s create a pattern app and consider it to seek out out comparability factors with React SWR.
In case you are already a TanStack Question consumer otherwise you’ve experimented with TanStack Question earlier than, you possibly can test the whole challenge in this GitHub repository and skip to the comparability part.
First, configure the mock API server and begin it as we did within the React SWR part. Now, create one other React challenge to implement the earlier easy product administration app with TanStack Question:
npx create-react-app tanstack-query-example cd tanstack-query-example
Putting in the package deal
Set up the @tanstack/react-query
package deal with the next command:
npm set up @tanstack/react-query # --- or --- yarn add @tanstack/react-query
Set up the Axios package deal and outline the bottom URL by following the identical steps we did within the SWR part. Get able to rewrite the earlier app with TanStack Question!
Clear all the things within the App.js
file and add the next imports:
import React, { useState } from 'react'; import { QueryClient, QueryClientProvider, useQuery, useQueryClient, useMutation } from '@tanstack/react-query'; import axios from 'axios'; import './App.css';
Right here, the useQuery
and useMutation
Hooks assist with knowledge fetching and updating (cached knowledge). We will use the QueryClient
class to create a broker-like occasion to entry or manipulate cached knowledge. The useQueryClient
Hook returns the present QueryClient
reference in all app elements.
The QueryClientProvider
part permits entry to cached knowledge for your complete React app, just like the inbuilt Context.Supplier
part within the React Context API.
Implementing knowledge fetchers and customized Hooks
Much like SWR, now we will create a wrapper for Axios, a perform to insert a product to the database, and a customized Hook to fetch cached merchandise, as proven under:
perform fetcher(url) { return axios.get(url).then(res => res.knowledge); } async perform addProduct(product) { let response = await axios.put up('/merchandise', product); return response.knowledge; } perform useProducts() { const { knowledge, isLoading, error } = useQuery(['products'], () => fetcher('/merchandise')); return { merchandise: knowledge, isLoading, isError: !!error }; }
Not like SWR, right here, we have now the handy isLoading
prop for conditional rendering, however with model 4, we have to ship each an array-based, distinctive key and a URL section to the useQuery
Hook as a result of the Hook calls the fetcher perform with a context object — it doesn’t move the distinctive key string immediately, the best way SWR does.
Utilizing TanStack Question for knowledge fetching
We will use the identical Merchandise
part supply from the SWR challenge, for the reason that customized Hook is sort of the identical:
perform Merchandise() { const { merchandise, isLoading, isError } = useProducts(); if(isError) return ( <div>Unable to fetch merchandise.</div> ); if(isLoading) return ( <div>Loading merchandise...</div> ); return ( merchandise.map((product) => ( <div key={product.id} className="product-item"> <div>{product.identify}</div> <div>${product.value}</div> </div> )) ); }
We will use the useProducts
Hook in a number of elements with out worrying about RESTful HTTP request duplication points, since TanStack Question additionally deduplicates related requests the best way SWR does.
Mutating cached knowledge and invalidating requests
Create a part referred to as AddProduct
and implement a means so as to add a brand new product with the next code:
perform AddProduct({ goToList }) { const { merchandise } = useProducts(); const queryClient = useQueryClient(); const mutation = useMutation((product) => addProduct(product), { onMutate: async (product) => { await queryClient.cancelQueries(['products']); const previousValue = queryClient.getQueryData(['products']); queryClient.setQueryData(['products'], (previous) => [...old, product]); return previousValue; }, onError: (err, variables, previousValue) => queryClient.setQueryData(['products'], previousValue), onSettled: () => queryClient.invalidateQueries(['products']) }); const [product, setProduct] = useState({ id: merchandise ? merchandise.size + 1 : 0, identify: '', value: null }); const [disabled, setDisabled] = useState(true); async perform handleAdd() { setTimeout(goToList); mutation.mutate(product); } perform handleFieldUpdate(e) { const factor = e.goal; const worth = factor.kind === 'quantity' ? parseInt(factor.worth) : factor.worth; const nextProduct = {...product, [element.name]: worth}; setProduct(nextProduct); setDisabled(!nextProduct.identify || !nextProduct.value); } return( <div className="product-form"> <enter kind="textual content" identify="identify" placeholder="Title" autoFocus onChange={handleFieldUpdate}/> <enter kind="quantity" identify="value" min="1" placeholder="Worth" onChange={handleFieldUpdate}/> <button onClick={handleAdd} disabled={disabled}>Add</button> </div> ); }
TanStack Question gives a full-featured mutation API that gives clear entry to your complete mutation lifecycle. As you possibly can see, we have now onMutate
, onError
, and onSettled
callbacks to implement our mutation technique.
On this instance, we replace the cached knowledge immediately with the brand new product object, then let TanStack Question ship a request to the POST
endpoint to replace the server state within the background.
SWR gives the mutation technique as an inbuilt function with restricted customization help, however this isn’t a dealbreaker, as SWR’s mounted mutation technique solves virtually all builders’ wants. Nonetheless, TanStack Question enables you to implement a mutation technique as you want, not like SWR.
Let’s create a brand new question consumer for the App
part:
const queryClient = new QueryClient();
A question consumer occasion helps present entry to the cached knowledge data in each app part.
Lastly, add the exported App
part supply to your App.js
file:
perform App() { const [ mode, setMode ] = useState('record'); return ( <QueryClientProvider consumer={queryClient}> <div className="menu-bar"> <div onClick={() => { setMode('record') }} className={mode === 'record' ? 'chosen' : ''}>All merchandise</div> <div onClick={() => { setMode('add') }} className={mode === 'add' ? 'chosen' : ''}>Add product</div> </div> <div className="wrapper"> { mode === 'record' ? <Merchandise/> : <AddProduct goToList={() => setMode('record')}/> } </div> </QueryClientProvider> ); } export default App;
We now must wrap our app elements with the QueryClientProvider
library part by offering the question consumer reference to get useQueryClient
functioning correctly in all baby elements.
Begin the RESTful mock server and run the app — you will notice the identical app we carried out with SWR. Attempt to open two tabs and add new merchandise; you will notice the re-validation-on-focus function in motion, as we anticipate.
Now, let’s examine each the SWR and TanStack Question libraries primarily based on the above findings.
SWR vs. TanStack Question
Primary CRUD options
Earlier, we tried knowledge retrieval and manipulation (fetching and mutation) to check CRUD help in each caching libraries. Each SWR and TanStack Question provide the options required to implement the pattern app.
SWR strives to offer each function in a minimal means, which can encourage builders to write down much less code for knowledge caching-related actions. However a minimal API design can typically include limitations for in-depth customization. TanStack Question offers primary fetching and mutation options in a extra customizable means than SWR, whereas SWR gives related options in a extra minimal means than TanStack Question.
Each libraries are backend-agnostic with a promise-based fetcher perform, so you should use each SWR and TanStack Question with REST, GraphQL, or some other communication mechanisms with most well-liked libraries you want: Axios, Unfetch, graphql-request, and many others.
General, each libraries ought to fulfill builders’ necessities for primary fetching and mutation help.
Recognition and developer help
An open supply library usually turns into widespread and positive aspects GitHub stargazers in just a few conditions:
- When extra builders use the precise library
- When that library offers better-than-average developer help
- When their repositories are well-maintained
Each of those libraries have many GitHub stargazers. Each libraries even have nice developer communities — builders assist one another by answering help queries on the GitHub repositories of those libraries. React SWR doesn’t provide an official developer instrument for debugging, however a group member created a GUI developer instrument for debugging functions.
TanStack Question maintains extra detailed, well-organized, and supportive official documentation than SWR. Nonetheless, each libraries provide wonderful instance initiatives/code snippets for builders to shortly perceive their primary ideas.
Developer instruments
A devoted GUI debugging instrument will not be obligatory for a third-party library. Nonetheless, a subject like caching is certainly complicated, so a developer instrument for a caching library can actually save improvement time.
TanStack Question comes with an official developer instrument, however SWR’s developer group created a non-official however well-used swr-devtools for SWR.
swr-devtools is minimal and solely exhibits you read-only knowledge, but it surely does embrace the essential info you want for debugging:
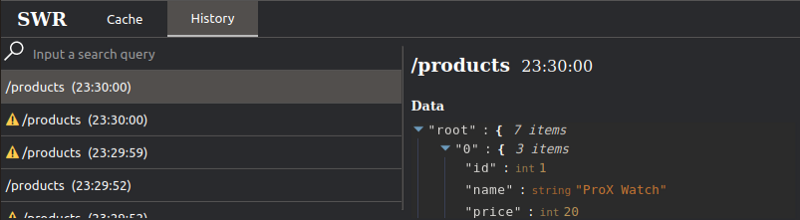
The TanStack Question developer instrument exhibits you the cached knowledge and allows you to manipulate the cached content material, not like the read-only swr-devtools:
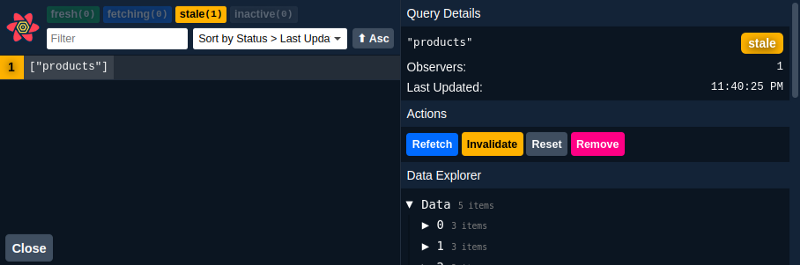
In keeping with the GitHub problem tracker, the swr-devtools challenge is planning so as to add help for cache manipulation into the developer instruments panel.
Inbuilt usability options
There are three key causes to make use of a library like SWR or TanStack Question:
- Cut back the quantity of code you might want to write to sync server-state and React app-state
- Use distant sources optimally by way of knowledge caching and deduplicated queries, like ideas
- Enhance software usability with a real-time expertise
Usability enchancment is a vital purpose for caching and question optimization, so each libraries competitively present the next usability options:
- Re-validation on focus
- Community standing re-fetching
- Knowledge pre-fetching
- Revalidation primarily based on a time interval
TanStack Question offers the next extra usability options:
- Scroll restoration for saving the infinite scroll place when the consumer returns to the part once more
- Question cancellation to cease long-running queries
- Offline mutation help
Bundle measurement and efficiency optimizations
Not all customers have super-fast web connections or use high-end computer systems. Subsequently, sustaining a wholesome bundle measurement and implementing efficiency optimizations assist all customers run your app easily, no matter their web pace and laptop specs. It’s follow to eat the optimum {hardware} sources from the consumer’s laptop for internet functions.
React SWR is a really light-weight library: BundlePhobia measures its gzipped measurement as solely 4.2kB. TanStack Question is a bit heavy resulting from its in depth options, so it’s 11.4 kB gzipped. It’s certainly greater than 4 occasions the dimensions of React’s core library!
Each libraries do render optimizations, request deduplication, and cache optimizations internally. Observe {that a} caching library wouldn’ increase HTTP request dealing with pace — HTTP request efficiency is determined by varied components, such because the HTTP consumer library efficiency, the browser’s JavaScript engine implementation, community pace, present CPU load, and many others.
SWR vs. TanStack Question: Abstract
Let’s summarize the above comparability components in a single desk. Have a look at the next desk and examine SWR and TanStack Question side-by-side:
Comparability issue | React SWR | TanStack Question |
---|---|---|
General API design | Offers a minimal API for builders with some mounted options | Offers an in depth and considerably complicated API for builders with absolutely customizable options |
Bundle measurement (gzipped) | 4.2 KB | 11.4 KB |
Recognition, group help, and documentation | Good group, a well-maintained repository, and general good documentation with demos | Good group, well-maintained repository, and informative documentation with many sensible examples and full API reference |
Primary knowledge fetching and mutation options | Satisfies developer necessities, however the developer has to write down extra code for some options and should face in-depth customization points | Satisfies developer necessities with in-depth customization help. Builders who attempt to combine it with smaller initiatives could discover the API a bit extra complicated than it ought to be |
Efficiency optimizations | Helps request deduplication, render optimizations, and optimized caching | Helps request deduplication, render optimizations, and optimized caching |
Inbuilt usability options | Revalidation on focus, community standing re-fetching, knowledge pre-fetching, and re-validation primarily based on an interval | Revalidation on focus, community standing re-fetching, knowledge pre-fetching, revalidation primarily based on an interval, request cancellation, offline mutation, and scroll restoration |
Inbuilt options for builders | Gives pagination and infinite loading options. The developer group carried out a developer instrument GUI with Chrome and Firefox extensions. Helps persisting cache into exterior storage places (i.e., localStorage ). |
Gives pagination and infinite loading options. It comes with an official developer instrument GUI with cache manipulation help. Helps persisting cache into exterior storage places (i.e., localStorage ). |
React Suspense | Supported | Supported |
Official help for different frontend libraries | No, related group libraries obtainable: sswr | In progress, related group libraries obtainable: vue-query |
Conclusion
On this article, we created a pattern React software with each SWR and TanStack Question libraries, then we in contrast them in accordance with the developer expertise and obtainable options.
Each libraries competitively carry out higher and have varied professionals and cons, as we’ve outlined right here. React SWR’s objective is to offer a minimal API to unravel the caching downside in React request dealing with by sustaining a light-weight library. In the meantime, TanStack Question strives to supply a completely featured resolution for a similar downside.
Typically, TanStack Question appears to be like like a framework that gives all the things you want from one improvement package , like Angular — SWR, alternatively, appears to be like extra like React in that it focuses on fixing just one downside. React launched purposeful elements to scale back complexity in class-based elements, so builders who like that sort of simplicity could desire SWR over TanStack Question.
Builders who like to work with detailed/strong APIs and search framework-like, all-in-one options for knowledge caching could select TanStack Question over SWR. The TanStack Question workforce is planning to supply official help for Svelte, SolidJS, Vue.js, and vanilla JavaScript apps with adaptor libraries for the core TanStack Question library. Nonetheless, the frontend developer group has already carried out a number of open-source caching libraries in accordance with TanStack Question and React SWR APIs for different frontend frameworks.
Our conclusion? Attempt each libraries. Choose one in accordance with your API preferences. TanStack Question has a number of distinctive options and in-depth customizations that SWR doesn’t help on the time of writing this text. It’s seemingly each libraries will grow to be equally absolutely featured and strong, particularly if SWR commits to implementing some lacking functionalities within the close to future.
Nonetheless, from the minimal API design perspective, SWR is already full and gives the obligatory options you search with out rising the bundle measurement additional, which TanStack Question definitely will do.
LogRocket proactively surfaces and diagnoses crucial points in your React apps
1000’s of engineering and product groups use LogRocket to scale back the time it takes to grasp the basis explanation for technical and usefulness points of their React apps. With LogRocket, you may spend much less time on back-and-forth conversations with prospects and take away the limitless troubleshooting course of. LogRocket lets you spend extra time constructing new issues and fewer time fixing bugs.
Proactively repair your React apps — attempt LogRocket right now.