Capabilities are units of statements that take inputs, carry out some operations, and produce outcomes. The operation of a operate happens solely when it’s known as. Moderately than writing the identical code for various inputs repeatedly, we will name the operate as a substitute of writing the identical code time and again. Capabilities settle for parameters, that are information. A operate performs a sure motion, and it’s important for reusing code. Inside a operate, there are a selection of programming statements enclosed by {}.
Instance:
int sum(int a, int b);
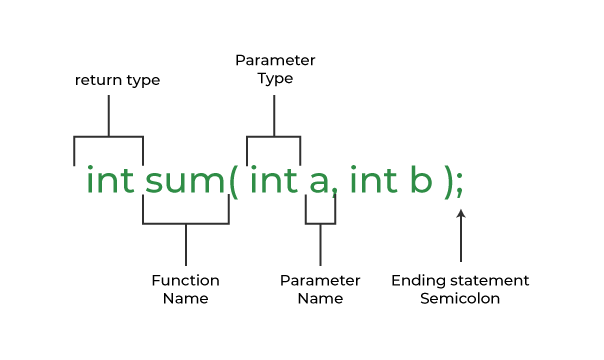
Perform Declarations
Perform declarations inform the compiler what number of parameters a operate takes, what sorts of parameters it returns, and what forms of information it takes. Perform declarations don’t want to incorporate parameter names, however definitions should.
Syntax:
return_type name_of_the_function (parameters);
Perform declaration for the sum of two numbers is proven beneath
int sum(int var1, int var2);
The parameter title shouldn’t be necessary whereas declaring features. We are able to additionally declare the above operate with out utilizing the title of the information variables.
int sum(int, int);
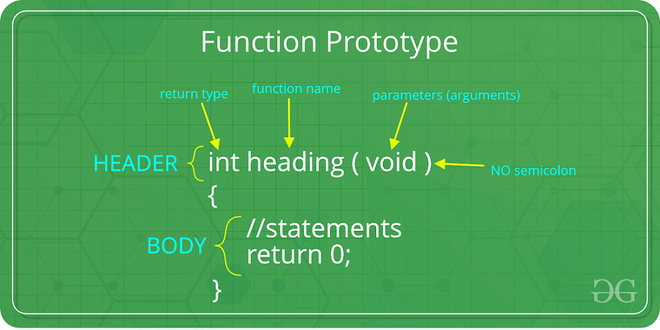
Perform Definition
A operate definition consists operate header and a operate physique.
return_type function_name (parameters) { //physique of the operate }
- Return_type: The operate at all times begins with a return kind of the operate. But when there is no such thing as a return worth then the void key phrase is used because the return kind of the operate.
- Function_Name: Title of the operate which must be distinctive.
- Parameters: Values which are handed throughout the operate name.
Perform Name
To Name a operate parameters are handed alongside the operate title. Within the beneath instance, the primary sum operate is known as and 10,30 are handed to the sum operate. After the operate name sum of a and b is returned and management can be returned again to the principle operate of this system.
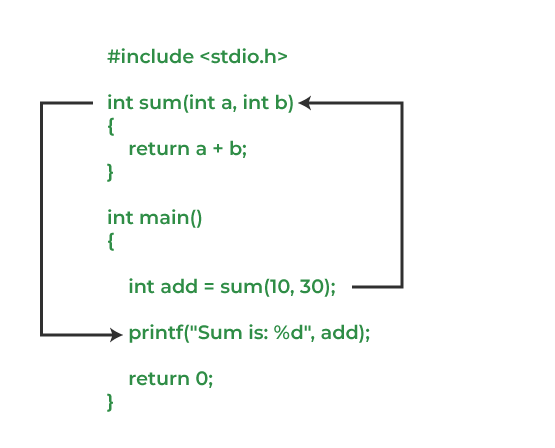
Working of Perform
C
|
Sorts of Capabilities
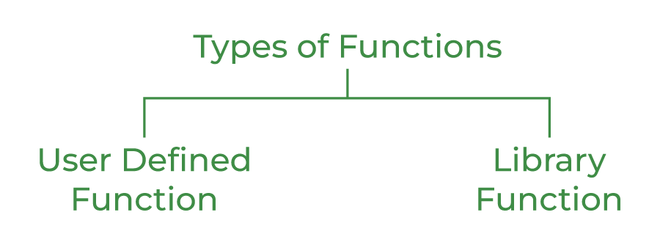
Sorts of Capabilities in C
1. Consumer Outlined Perform
Capabilities which are created by the programmer are referred to as Consumer-Outlined features or “tailored features”. Consumer-defined features will be improved and modified in accordance with the necessity of the programmer. At any time when we write a operate that’s case-specific and isn’t outlined in any header file, we have to declare and outline our personal features in accordance with the syntax.
Benefits of Consumer-Outlined features
- Changeable features will be modified as per want.
- The Code of those features is reusable in different packages.
- These features are straightforward to know, debug and preserve.
Instance:
C
|
2. Library Perform
A library operate can be known as a “built-in operate”. There’s a compiler bundle that already exists that accommodates these features, every of which has a particular that means and is included within the bundle. Constructed-in features have the benefit of being instantly usable with out being outlined, whereas user-defined features should be declared and outlined earlier than getting used.
For Instance:
pow(), sqrt(), strcmp(), strcpy() and so on.
Benefits of C library features
- C Library features are straightforward to make use of and optimized for higher efficiency.
- C library features save plenty of time i.e, operate growth time.
- C library features are handy as they at all times work.
Instance:
C
|
The Sq. root of 49.00 = 7.00
Passing Parameters to Capabilities
The worth of the operate which is handed when the operate is being invoked is called the Precise parameters. Within the beneath program 10 and 30 are referred to as precise parameters.
Formal Parameters are the variable and the information kind as talked about within the operate declaration. Within the beneath program, a and b are referred to as formal parameters.
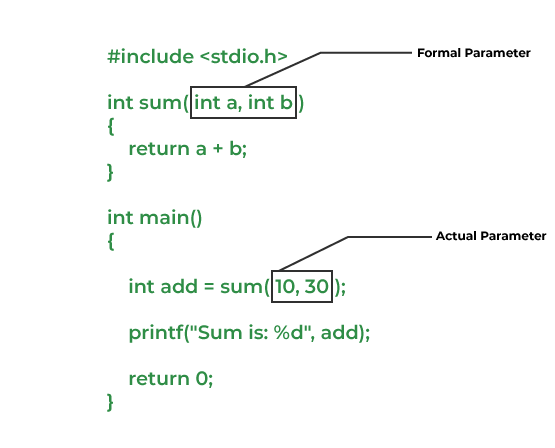
1. Cross by Worth: Parameter passing on this methodology copies values from precise parameters into operate formal parameters. Consequently, any modifications made contained in the features don’t mirror within the caller’s parameters.
Under is the C program to point out pass-by-value:
C
|
Earlier than swap Worth of var1 and var2 is: 3, 2 After swap Worth of var1 and var2 is: 3, 2
2. Cross by Reference: The caller’s precise parameters and the operate’s precise parameters seek advice from the identical places, so any modifications made contained in the operate are mirrored within the caller’s precise parameters.
Under is the C program to point out pass-by-reference:
C
|
Earlier than swap Worth of var1 and var2 is: 3, 2 After swap Worth of var1 and var2 is: 2, 3
Perform Arguments and Return values
In C programming language, features will be known as both with or with out arguments and would possibly return values. They might or won’t return values to the calling features. To know extra about operate Arguments and Return values seek advice from the article – Perform Arguments & Return Values in C.
- Perform with arguments and no return worth
- Perform with no arguments and with return worth
- Perform with argument and with no return worth
- Perform with arguments and with return worth