Enhancing ThymeBoost’s Effectivity
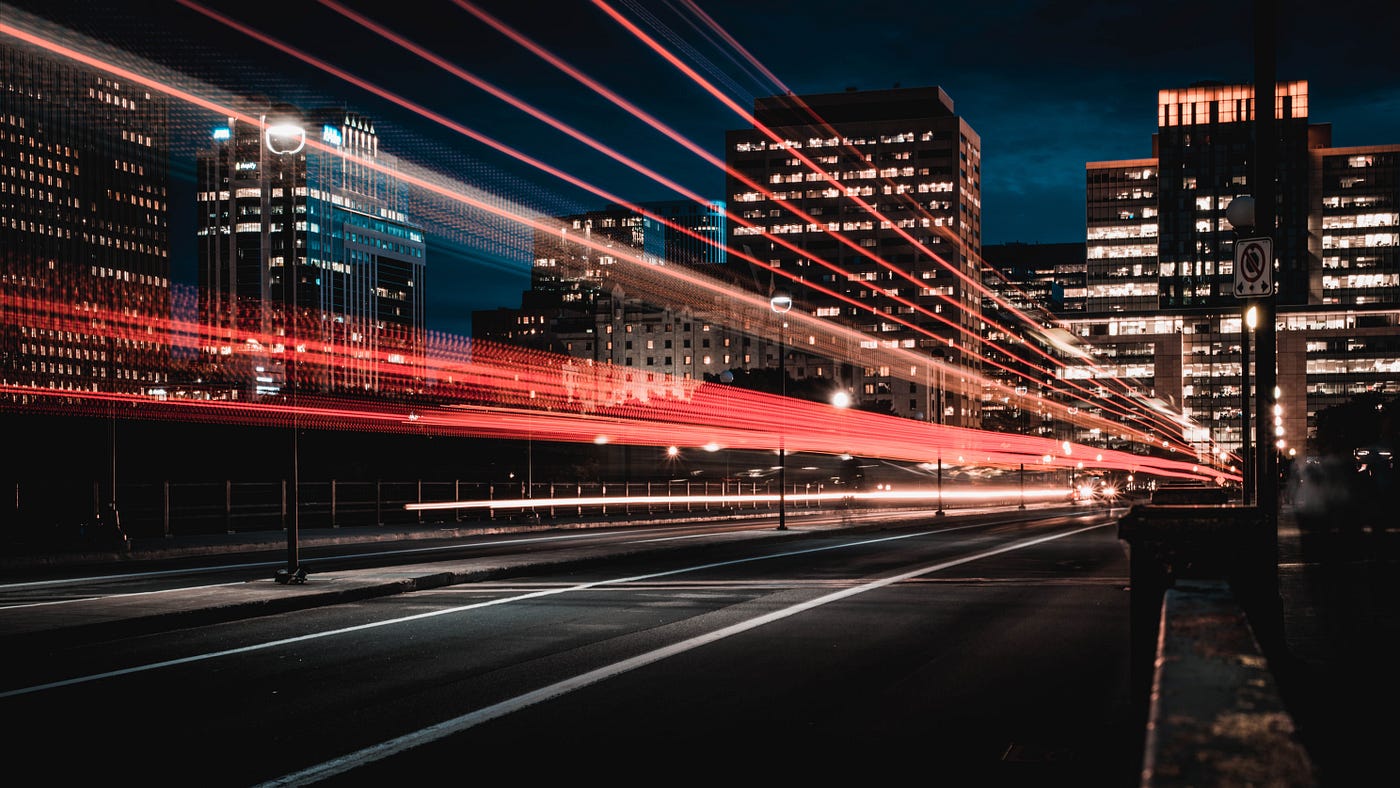
The ThymeBoost framework, at its core, is just a gradient boosting algorithm wrapped round commonplace time sequence strategies. Because of this the framework depends closely on the underlying technique’s effectivity and velocity. The boosting and extra logic on-top provides, as we’ll see on this article, accuracy however computation as nicely. Most of this heavy lifting has been beforehand achieved by way of StatsModels for issues reminiscent of ETS and ARIMA, however using Nixtla’s statistical forecasting bundle: StatsForecast, can enhance each velocity and accuracy. Making ThymeBoost and StatsForecast an ideal marriage for Time Sequence Forecasting.
An excellent TLDR for this text is:
StatsForecast is quicker than StatsModels, ThymeBoost brings accuracy features.
Introduction
Very first thing’s first, you probably have not heard of ThymeBoost then I encourage you to take a look at my earlier article giving an honest overview. With the latest launch I’ve added the StatsForecast as an optionally available dependency. In an effort to run these examples you will have to put in it:
pip set up StatsForecast
And go forward and replace ThymeBoost simply to be protected:
pip set up ThymeBoost --upgrade
Now that we’ve got that out of the way in which — the primary ‘meat and potatoes’ of this text goes to be benchmarking on the Weekly M4 dataset to see how all of those fashions are performing in each accuracy and velocity. The datasets are all open supply and stay on the M-competitions github. It’s cut up up by the usual practice and take a look at splits, so we’ll use the practice csv for becoming and the take a look at csv just for analysis utilizing the SMAPE.
Be at liberty to check this out with different datasets and let me know the way they carry out!
The primary purpose of that is to assessment how the brand new strategies stack up within the boosting framework and, in the end, to see how including them to the ThymeBoost framework can present accuracy features.
Benchmarking The Strategies
To start out off, we’ll check out essentially the most computationally heavy technique in ThymeBoost: AutoArima. Beforehand finished with PmdArima, now we will take a look at with StatsForecast by merely passing trend_estimator=‘fast_arima’
when becoming with ThymeBoost. Let’s check out some code the place we first construct our dataset then we will run ThymeBoost:
from tqdm import tqdm
from statsforecast.fashions import ETS, AutoARIMA
from ThymeBoost import ThymeBoost as tb
tqdm.pandas()
train_df = pd.read_csv(r'm4-weekly-train.csv')
test_df = pd.read_csv(r'm4-weekly-test.csv')
forecast_horizon = len(test_df.columns) - 1
train_df = train_df.rename({'V1': 'ID'}, axis=1)
train_long = pd.wide_to_long(train_df, ['V'], 'ID', 'Date')
test_df = test_df.rename({'V1': 'ID'}, axis=1)
test_df = pd.wide_to_long(test_df, ['V'], 'ID', 'Date')
train_long = train_long.dropna()
train_df = train_long.reset_index()
train_df.index = train_df['ID']
train_df = train_df.drop('ID', axis = 1)
X = train_long
X = X.reset_index()
- Observe: this code might be very inefficient at information manipulation and I’m certain there are higher methods to do it, this was simply one thing I threw collectively that works for the benchmark. The timing doesn’t embody the time it takes to run this code.
Both approach, now we’ve got our Coaching information to suit on, let’s check out the match perform:
def grouped_forecast(df):
y = df['V'].values
boosted_model = tb.ThymeBoost(verbose=0)
output = boosted_model.match(y,
seasonal_period=None,
trend_estimator=['fast_arima'])
predicted_output = boosted_model.predict(output,
forecast_horizon,
trend_penalty=True)
predictions = predicted_output['predictions']
return predictions
Right here we’re simply making a perform that can be handed after we do a groupby and apply:
def counter(df):
df['counter'] = np.arange(2, len(df) + 2)
return df
predictions = X.groupby('ID').progress_apply(grouped_forecast)
predictions = predictions.reset_index()
predictions = predictions.groupby('ID').apply(counter)
test_df = test_df.reset_index()
benchmark_df = predictions.merge(test_df, left_on=['ID', 'counter'],
right_on=['ID', 'Date'])def smape(A, F):
return 100/len(A) * np.sum(2 * np.abs(F - A) / (np.abs(A) + np.abs(F)))
tqdm.pandas()
def grouped_smape(df):
return smape(df['V'], df['predictions'])
take a look at = benchmark_df.groupby('ID').progress_apply(grouped_smape)
print(np.imply(take a look at))
Then we simply get the typical SMAPE for the given outputs, all the things right here needs to be good however let me know if there are any errors which might muddy the benchmark.
Working this offers you a mean SMAPE worth of 8.61 and it ought to take roughly 10 minutes.
Subsequent, let’s simply run Nixtla’s Auto Arima by itself and see the way it performs.
We’ll simply change that groupby forecast perform to:
def grouped_forecast(df):
y = df['V'].values
ar_model = AutoARIMA().match(y)
predictions = pd.DataFrame(ar_model.predict(forecast_horizon)['mean'],
columns=['predictions'])
return predictions
Re-running the SMAPE calculation chunk above offers you a SMAPE of 8.93 and a time of roughly 4 minutes.
Alright, nice, so we’ve got proven some accuracy features by simply boosting the Auto-Arima process. This could come as no shock as I confirmed very comparable ends in an article deep diving Gradient Boosted Arima. However I do wish to caveat that boosting will not be a Panacea and doesn’t all the time enhance upon Arima, however it’s nonetheless an attention-grabbing remark.
The subsequent step needs to be apparent. We’ve taken a take a look at the ‘quick’ Auto-Arimain ThymeBoost in addition to StatsForecast’s Auto-Arimawithout boosting. Subsequent we should always see how these stack as much as utilizing PmdArima’s Auto-Arimain ThymeBoost.
When you have been working this code up till now, buckle up.
This subsequent bit will take a while…
def grouped_forecast(df):
y = df['V'].values
boosted_model = tb.ThymeBoost(verbose=0, n_rounds=None)
output = boosted_model.match(y,
seasonal_period=None,
trend_estimator=['arima'],
arima_order='auto')
predicted_output = boosted_model.predict(output,
forecast_horizon,
trend_penalty=True)
predictions = predicted_output['predictions']
return predictions
And the outcomes?
A SMAPE of 8.78, but it surely took 90 minutes. Appears like boosting Pmd Arima outperforms Nixtla’s StatsForecast out of the field but it surely takes fairly awhile.
Arima will not be the entire choices in StatsForecast, one other implementation is an ETS technique. With these new strategies we will truly make the most of these quicker implementations in ThymeBoost’s autofit
technique. To do that we simply must go quick=True
when calling autofit. A brand new forecast perform would then seem like this:
def grouped_forecast(df):
y = df['V'].values
boosted_model = tb.ThymeBoost(verbose=0, n_rounds=None)
output = boosted_model.autofit(y,
seasonal_period=[52],
optimization_type='grid_search',
optimization_strategy='holdout',
lag=26,
optimization_metric='smape',
verbose=False,
quick=False
) predicted_output = boosted_model.predict(output,
forecast_horizon,
trend_penalty=True)
predictions = predicted_output['predictions']
return predictions
This ends in a SMAPE of seven.88 and it takes about 80 minutes. Positively the very best plug-and-play accuracy out of all the things examined however we’re sort of dishonest by doing mannequin choice.
One factor to notice is that passing a seasonal size of 52 to StatsForecast’s strategies will not be a terrific thought. For ETS it errors and for Auto-Arima it takes approach too lengthy. That is one space the place making the most of how ThymeBoost works truly will increase velocity as lengthy seasonal durations take considerably longer in an ARIMA setup.
A number of different strategies have been examined and you’ll view the benchmark outcomes beneath:

When it comes to the acronyms:
- TB: ThymeBoost
- SF: StatsForecast
- NS: Non-Seasonal
- Mult: Multiplicative Seasonality
- Quick: ThymeBoost using StatsForecast beneath the hood
At a excessive stage, the very best performing is the Quick AutoFit technique from ThymeBoost. For some odd motive, becoming ThymeBoost with seasonality and quick Arima doesn’t carry out too nicely, the truth is it’s considerably worse than utilizing PmdArima’s Auto-Arima. One other remark is that boosting plain ETS strategies from StatsForecast could damage the accuracy over simply regular becoming with non-boosting strategies. This may increasingly change if we alter the global_cost
parameter within the match perform because the default will not be optimum the entire time.
Conclusion
The most recent model of ThymeBoost has some added functionality to herald StatsForecast’s strategies. With this we will see elevated velocity and doubtlessly accuracy over the earlier implementation.
Like cake, ThymeBoost must have batter as a base. StatsForecast could also be that superior batter over StatsModels. The gradient boosting is simply the sprinkles on prime.
For those who loved this text, you may take a look at another time-series associated posts I’ve written: