Evaluating Brute drive and Black-box Optimization Strategies in Python
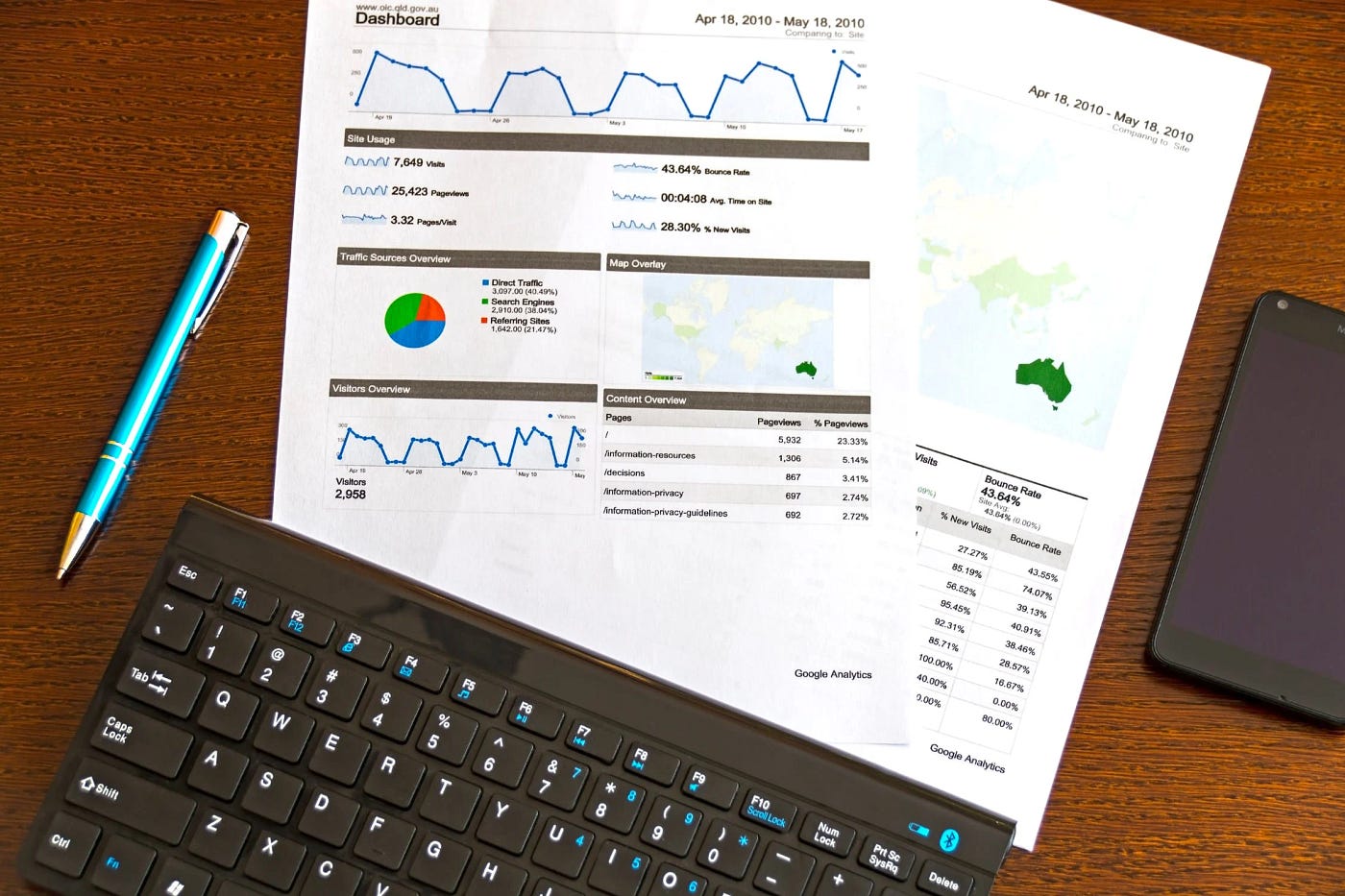
In machine studying, hyperparameters are values used to manage the educational course of for a machine studying mannequin. That is to be distinguished from inner machine studying mannequin parameters which can be realized from the info. Hyperparameters are values which can be exterior to machine studying coaching knowledge that decide the optimality of a machine studying mannequin’s efficiency. Every distinctive set of hyperparameters correspond to a novel machine studying mannequin. The set of all attainable hyperparameter mixtures can grow to be fairly giant for many state-of-the-art machine studying fashions. Happily, most machine studying mannequin packages include default hyperparameter values that obtain respectable baseline efficiency. Because of this the info scientist or machine studying engineer can use fashions out of the field with out having to fret about hyperparameter choice at the beginning. These default fashions typically outperform what a knowledge scientist or engineer would be capable of check and choose manually.
Conversely, to optimize efficiency, the info scientist or machine studying engineer should check a variety of values for hyperparameters which can be distinct from the default values. This could grow to be fairly cumbersome and inefficient to carry out manually. For that reason many algorithms and libraries have been designed to automate the method of hyperparameter choice. Hyperparameter choice is an train in optimization, the place the target operate is represented by how poorly the mannequin performs. The optimization process is to search out the very best set of parameters that minimizes how poorly a machine studying mannequin performs. For those who discover the machine studying mannequin with the least poor efficiency, that corresponds to the mannequin with the very best efficiency.
The house of optimization is kind of wealthy with literature spanning, brute drive strategies and black-box non-convex optimization. Brute drive optimization is the duty of exhaustively trying to find the very best set of parameters of all attainable hyperparameter mixtures. Whether it is attainable to exhaustively search the hyperparameter house it would give the set of hyperparameters that give the globally optimum answer. Sadly, exhaustively looking out the hyperparameter house is commonly not possible by way of computationally sources and time.It is because hyperparameter tuning machine studying fashions falls into the class of non-convex optimization. This can be a kind of optimization the place discovering a world optimum just isn’t possible since it might get caught in certainly one of a number of suboptimal ‘traps’, additionally referred to as native minima, that make it troublesome for the algorithm to go looking the total house of hyperparameters.
Alternate options to brute drive optimization are black-box non-convex optimization optimization strategies. Black-box non-convex optimization algorithms discover suboptimal options, native minima (or maxima), which can be optimum sufficient primarily based on some predefined metric.
Python has instruments for brute drive optimization and black field optimization. The GridSearchcv within the mannequin choice module permits brute drive optimization.The RBFopt python bundle is a black-box optimization library developed by IBM. It really works by utilizing a radial foundation features to construct and refine the surrogate fashions of the operate being optimized. It’s helpful as a result of it makes no assumptions in regards to the form or conduct of the operate being optimized. It has been used to optimize advanced fashions corresponding to deep neural networks.
The duty of constructing, testing and evaluating mannequin hyperparameters and machine studying algorithms is commonly collaborative in nature. With this in thoughts, I will likely be working with DeepNote, a collaborative knowledge science pocket book that makes it straightforward for knowledge scientists to work collectively on machine studying and knowledge analytic duties. Right here we’ll stroll by means of methods to apply every of those optimization instruments for tuning hyperparameters of a classification mannequin. We’ll think about the supervised machine studying process of predicting if a buyer won’t make a repeat buy, which is named churning. We’ll work with the fictional Telco Churn knowledge set which is publicly accessible on Kaggle. The info set is free to make use of, modify and share below the Apache 2.0 License.
Studying in Telco Churn Information
To begin let’s import the python pandas library and skim our knowledge right into a pandas knowledge body and show the primary 5 rows of information:
import pandas as pd
df = pd.read_csv("telco_churn.csv")
We see that the info comprises fields corresponding to buyer ID, gender, senior citizen standing, and extra. If we hoover our cursor over the cell output to the left we’ll see the next:
We see that we’ve the sector ‘churn’, which corresponds as to if or not a buyer made a repeat buy. A worth of ‘No’ signifies that the shopper has made repeat purchases and a price of ‘Sure’ signifies that the shopper stopped making purchases.
We’ll construct a easy classification mannequin that takes the fields gender, SeniorCitizen, InternetService, DeviceProtection, MonthlyCharges, and TotalCharges as inputs and predicts whether or not or not the shopper will churn. To do that we have to convert our categorical columns into machine readable values that may be handed as inputs into our machine studying fashions. Let’s do that for gender, SeniorCitizen, InternetService, and DeviceProtection:
#convert categorical columns
#convert categorical columns
df['gender'] = df['gender'].astype('class')
df['gender_cat'] = df['gender'].cat.codes
df['SeniorCitizen'] = df['SeniorCitizen'].astype('class')
df['SeniorCitizen_cat'] = df['SeniorCitizen'].cat.codes
df['InternetService'] = df['InternetService'].astype('class')
df['InternetService_cat'] = df['InternetService'].cat.codes
df['DeviceProtection'] = df['DeviceProtection'].astype('class')
df['DeviceProtection_cat'] = df['DeviceProtection'].cat.codes
And let’s show the ensuing columns:
df[['gender_cat', 'SeniorCitizen_cat', 'InternetService_cat', 'DeviceProtection_cat']].head()
We additionally must do one thing comparable with the Churn column:
df['Churn'] = df['Churn'].astype('class')
df['Churn_cat'] = df['Churn'].cat.codes
Subsequent factor we have to do is clear up our TotalCharges column by changing invalid values with NaN and imputing NaNs with the imply of TotalCharges
df['TotalCharges'] = pd.to_numeric(df['TotalCharges'], 'coerce')
df['TotalCharges'].fillna(df['TotalCharges'].imply(), inplace=True)
Now let’s put together our inputs and our output. We’ll outline a variable X which will likely be a sequence containing the columns gender, SeniorCitizen, InternetService, DeviceProtection, MonthlyCharges, and TotalCharges. Our output will likely be a variable referred to as Y which is able to comprise the Churn values:
#outline enter and output
X = df[['TotalCharges', 'MonthlyCharges', 'gender_cat', 'SeniorCitizen_cat', 'InternetService_cat', 'DeviceProtection_cat']]
y = df['Churn_cat']
Subsequent, let’s cut up our knowledge for coaching and testing. We’ll use the train_test_split technique from the model_selection module in scikit-learn:
from sklearn.model_selection import train_test_split
X_train, X_test, y_train, y_test = train_test_split(X, y, random_state=42)
Modeling with Default Parameters
To begin, we’ll construct a random forest classification mannequin. The random forest algorithm is a sort of tree-based ensemble mannequin algorithm that makes use of a mixture of determination bushes to forestall overfitting. Let’s import the random forest class from the ensemble module in scikit-learn:
from sklearn.ensemble import RandomForestClassifier
Subsequent let’s outline our random forest classifier mannequin object and match our mannequin to our coaching knowledge. By leaving the argument of the RandomForestClassifier empty we’re outline a mannequin with predefined default parameters:
mannequin = RandomForestClassifier()
mannequin.match(X_train, y_train)
Let’s print the default parameter values of our mannequin. To do that we merely name the get_params() technique on our mannequin object:
mannequin.get_params()
We’ll use precision to judge our classification mannequin. This can be a good selection for imbalance classification issues corresponding to churn prediction. Let’s consider the precision on the maintain out set of targets:
from sklearn.metrics import precision_scorey_pred_default = mannequin.predict(X_test)precision = precision_score(y_test, y_pred_default)precision
Now let’s take a look at how we apply brute drive grid search to search out the very best random forest classification mannequin.
Brute Power Optimization with GridSearchCV
Brute drive looking out strategies corresponding to GridSearchCv work by exhaustively trying to find the very best set of hyperparameters over all the search house. To begin let’s import the GridSearchCV technique from the mannequin choice module in scikit-learn:
from sklearn.model_selection import GridSearchCV
Let’s additionally outline a dictionary which we’ll use to specify our grid of parameters. Let’s outline a spread of estimators (determination tree from 10 to 100), max depth from, of the choice tree, from 5 to twenty, max options equal to sqrt, and criterion equal to the gini index (which is the metric used to separate teams within the determination tree:
params = {'n_estimators': [10, 100],
'max_features': ['sqrt'],
'max_depth' : [5, 20],
'criterion' :['gini']}
Subsequent let’s outline our grid search object with our parameter dictionary:
grid_search_rf = GridSearchCV(estimator=mannequin, param_grid=params, cv= 20, scoring='precision')
And match the item to our coaching knowledge:
grid_search_rf.match(x_train, y_train)
And from there we are able to show the very best parameters:
gscv_params = grid_search_rf.best_params_gscv_params
And redefine our random forest mannequin with the optimum parameters:
gscv_params = grid_search_rf.best_params_model_rf_gscv = RandomForestClassifier(**gscv_params)model_rf_gscv.match(X_train, y_train)
Let’s consider the precision on the maintain out set of targets:
y_pred_gscv = model_rf_gscv.predict(X_test)precision_gscv = precision_score(y_test, y_pred_gscv)precision_gscv
We see that our precision really outperforms the default values. Whereas that is good, for a wide range of parameter values and bigger knowledge units this technique can grow to be intractable. Various strategies corresponding to black field optimization and bayesian optimization are higher decisions for hyperparameter tuning.
Black-Field Optimization with RBFopt
Let’s now think about black-box hyperparameter optimization with RBFopt. RBFopt works by utilizing radial foundation operate to construct and refine the surrogate mannequin of the operate being optimized. That is usually used for a operate with no closed-form expression and plenty of hills and valleys. That is in distinction to easy well-known features with closed-form expressions corresponding to a quadratic or exponential operate.
To begin let’s set up RBFopt:
%pip set up -U rbfopt
Subsequent we have to outline a listing of higher and decrease bounds for our mannequin parameters. The decrease sure listing will comprise 10 for the variety of estimators and 5 for the max depth. The higher sure listing will comprise 100 for the variety of estimators and 20 for the max depth:
lbounds = [10, 5]ubounds = [100, 20]
Subsequent let’s import RBFopt and the cross validation technique:
import rbfoptfrom sklearn.model_selection import cross_val_score
Subsequent we have to outline our goal operate. It should take inputs for n_estimators and max_depth and construct a number of fashions for every set of parameters. For every mannequin we’ll calculate and return the precision. We search to search out the set of values for n_estimators and max_depth that maximize precision. Since RBFopt finds the minimal, so as to discover the set of parameters that maximize precision, we’ll return the unfavorable of precision:
def precision_objective(X):
n_estimators, max_depth = X
n_estimators = int(n_estimators)
max_depth = int(max_depth)
params = {'n_estimators':n_estimators, 'max_depth': max_depth}
model_rbfopt = RandomForestClassifier(criterion='gini', max_features='sqrt', **params)
model_rbfopt.match(X_train, y_train)
precision = cross_val_score(model_rbfopt, X_train, y_train, cv=20, scoring='precision')
return -np.imply(precision)
Subsequent we specify the variety of runs, operate calls, and dimensions:
num_runs = 1max_fun_calls = 8ndim = 2
Right here we solely run with 8 operate calls. For those who want to run for greater than 10 operate calls you need to set up the bonmin and ipopt packages. Directions for set up may be discovered on their respective linked GitHub pages.
Now, let’s specify our goal operate and run RBFopt:
obj_fun = precision_objectivebb = rbfopt.RbfoptUserBlackBox(dimension=ndim, var_lower=np.array(lbounds, dtype=np.float), var_upper=np.array(ubounds, dtype=np.float), var_type=['R'] * ndim, obj_funct=obj_fun)settings = rbfopt.RbfoptSettings(max_evaluations=max_fun_calls)alg = rbfopt.RbfoptAlgorithm(settings, bb)
And retailer the target worth and options of their respective variables:
fval, sol, iter_count, eval_count, fast_eval_count = alg.optimize()obj_vals = fval
We then retailer the integer values options in a dictionary:
sol_int = [int(x) for x in sol]
params_rbfopt = {'n_estimators': sol_int[0], 'max_depth': sol_int[1]}
params_rbfopt
We see that RBFopt finds optimum values of 81 and 5 for n_estimators and max_depth respectively.
After which move these optimum parameters into our new mannequin and match to our coaching knowledge:
model_rbfopt = RandomForestClassifier(criterion=’gini’, max_features=’sqrt’, **params_rbfopt)model_rbfopt.match(X_train, y_train)
And consider the precision:
y_pred_rbfopt = model_rbfopt.predict(X_test)precision_rbfopt = precision_score(y_test, y_pred_rbfopt)precision_rbfopt
We see that we’ve a slight enchancment in precision with the quicker optimization algorithm. That is particularly helpful for when you’ve gotten giant hyperparameter search areas.
The code used on this put up is accessible on GitHub.
Conclusions
Having a great understanding of the accessible instruments for hyperparameter tuning machine studying fashions is crucial for each knowledge scientist. Whereas the default hyperparameters of most machine studying algorithms give good baseline efficiency, hyperparameter tuning is commonly essential to see enchancment on the baseline efficiency. Brute drive optimization strategies are helpful as they exhaustively search the hyperparameter house which is able to assure an enchancment on baseline efficiency from default parameters. Sadly, brute drive optimization is useful resource intensive by way of time and computation. For these causes, extra environment friendly black-box optimization strategies, like RBFopt, are helpful alternate options to brute drive optimization. RBFopt is a really helpful black-box approach that ought to be part of each knowledge science toolkit for hyperparameter optimization.