Assertion capabilities in TypeScript are a really expressive kind of operate whose signature states {that a} given situation is verified if the operate itself returns.
In its fundamental type, a typical assert
operate simply checks a given predicate and throws an error if such a predicate is fake. For instance, Node.js’s assert throws an AssertionError
if the predicate is fake.
TypeScript, since its model 3.7, has gone slightly past that by implementing the help of assertions on the kind system degree.
On this article, we’re going to discover assertion capabilities in TypeScript and see how they can be utilized to precise invariants on our variables.
Desk of Contents
JavaScript-like assertions
Node.js comes with a predefined assert operate. As we talked about within the introduction, it throws an AssertionError
if a given predicate is fake:
const aValue = 10 assert(aValue === 20)
In JavaScript, this was helpful to protect in opposition to improper varieties in a operate:
operate sumNumbers(x, y) { assert(typeof x === "quantity"); assert(typeof y === "quantity"); return x + y; }
Sadly, the code circulation evaluation doesn’t take note of these assertions. Actually, they’re merely evaluated at runtime after which forgotten.
With its assertion capabilities, TypeScript’s code circulation evaluation will have the ability to use the kind of a operate (in short, its signature) to deduce some properties of our code. We will use this new characteristic to make ensures of our varieties all through our code.
TypeScript-like assertion
An assertion operate specifies, in its signature, the kind predicate to guage. For example, the next operate ensures a given worth be a string
:
operate isString(worth: unknown): asserts worth is string { if (typeof worth !== "string") throw new Error("Not a string") }
If we invoke the operate above with a given parameter, and it returns appropriately, TypeScript is aware of that worth has kind string
. Therefore, it’s going to slim down its kind to string
:
const aValue: string|quantity = "Hey" isString(aValue) // The kind of aValue is narrowed to string right here
After all, nothing prevents us from messing up the assertion. For instance, we may have written a (improper) operate as follows:
operate isString(worth: unknown): asserts worth is string { if (typeof worth !== "quantity") throw new Error("Not a string") }
Be aware that we’re now checking whether or not worth's
kind will not be quantity
, as an alternative of string
. On this case, TypeScript’s code circulation evaluation will see a Worth
of kind by no means
, as an alternative of string
as above.
Assertion capabilities might be very helpful with enums:
kind AccessLevel = "r" | "w" | "rw" const writeOnly = "w" operate allowsReadAccess(degree: AccessLevel): asserts degree is "r" | "rw" { if (!degree.contains("r")) throw new Error("Learn not allowed") } allowsReadAccess(writeOnly)
Within the instance above, we first outlined a kind whose worth can solely be both "r"
, "w"
, or "rw"
. Let’s assume such a kind merely defines the three kinds of entry to a given useful resource. We then declare an assertion operate throwing if its precise parameter doesn’t permit a learn operation.
As you’ll be able to see, we’re narrowing down the kind explicitly, stating that, if the operate returns, the worth have to be both "r"
or "rw"
. If we name allowsReadAccess
with writeOnly
because the precise parameter, we’ll get an error as anticipated, stating that "Learn entry will not be allowed"
.
One other widespread use of assertion capabilities is expressing non-nullability. The next snippet of code exhibits a means to verify a worth is outlined, that’s it’s not both null
or undefined
:
operate assertIsDefined<T>(worth: T): asserts worth is NonNullable<T> { if (worth === undefined || worth === null) { throw new Error(`${worth} will not be outlined`) } }
The place NonNullable<T>
is a TypeScript kind that excludes null
and undefined
from the legit values of the kind T.
Operate declarations and expressions
On the time of writing, assertion capabilities is probably not outlined as plain operate expressions. Usually talking, operate expressions might be seen as nameless capabilities; that’s, capabilities with out a identify:
// Operate declaration operate enjoyable() { ... } // Operate expression const enjoyable = operate() { ... }
The primary benefit of operate declarations is hoisting, which is the opportunity of utilizing the operate anyplace within the file the place it’s outlined. However, operate expressions can solely be used after they’re created.
There’s really a workaround to jot down assertion capabilities as operate expressions. As a substitute of defining the operate together with its implementation, we’ll should outline its signature as an remoted kind:
Extra nice articles from LogRocket:
// Incorrect // Error: A sort predicate is just allowed in return kind place for capabilities and strategies. // Error: Sort '(worth: any) => void' will not be assignable to kind 'void'. const assertIsNumber: asserts worth is quantity = (worth) => { if (typeof worth !== 'quantity') throw Error('Not a quantity') } // Right kind AssertIsNumber = (worth: unknown) => asserts worth is quantity const assertIsNumber: AssertIsNumber = (worth) => { if (typeof worth !== 'quantity') throw Error('Not a quantity') }
Assertion capabilities and kind guards
Assertion capabilities in TypeScript are considerably just like kind guards. Sort guards had been initially launched to carry out runtime checks to ensure the kind of a worth in a given scope.
Specifically, a kind guard is a operate that merely evaluates a kind predicate, returning both true
or false
. That is barely totally different from assertion capabilities, which, as we noticed above, are purported to throw an error as an alternative of returning false
if the predicate will not be verified.
operate isString(worth: unknown): worth is string { return typeof worth === "string" } // Sort guards may also be declared as operate expression const isStringExp = (worth: unknown): worth is string => typeof worth === "string"
There’s one other massive distinction although. Assertion capabilities may also be used with out a kind predicate, as we’ll see within the following part.
Assertion capabilities with out a kind predicate
The assertion capabilities we’ve seen thus far had been all checking whether or not a given worth had a given kind. Therefore, they had been all pretty tailor-made for the goal kind. Nonetheless, assertion capabilities give us far more energy. Specifically, we will write a very normal operate asserting a situation that will get enter as a parameter:
operate assert(situation: unknown, msg?: string): asserts situation { if (situation === false) throw new Error(msg) }
The assert
operate now inputs a situation
, whose kind is unknown
, and, presumably, a message
. Its physique merely evaluates such a situation. Whether it is false
, then assert
throws an error, as anticipated.
Be aware, nonetheless, that the signature makes use of the situation
parameter after asserts
. This manner, we’re telling TypeScript code circulation evaluation that, if the operate returns appropriately, it may possibly assume that no matter predicate we handed in was, in reality, verified.
TypeScript’s Playground offers us a reasonably good visible illustration of what the code circulation evaluation does. Let’s contemplate the next snippet of code, the place we generate a random quantity after which name assert
to verify the generated quantity is 10
:
const randomNumber = Math.random() assert(randomNumber == 10, "The quantity have to be equal to 10") randomNumber
If we examine the inferred properties of randomValue
earlier than the decision to assert
, TypeScript simply tells us the kind (Determine 1).
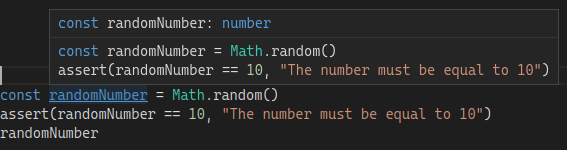
Then, as quickly as we name assert
, with the situation randomNumber == 10
, TypeScript is aware of that the worth might be 10
for the remainder of the execution (Determine 2).

randomNumber
is ready to 10.Lastly, if we try and verify the equality of randomNumber
and one other quantity, TypeScript will have the ability to consider the property with out even operating this system. For instance, the code circulation evaluation will complain in regards to the following project, saying, “This situation will all the time return ‘false’ for the reason that varieties ’10’ and ’20’ don’t have any overlap.”:
const pred = (randomNumber === 20)
Conclusion
On this article, we dove into what TypeScript assertion capabilities are and the way we will use them to have the code circulation evaluation infer a set of properties about our values. They’re a really good characteristic that is smart contemplating that TypeScript is transpiled to JavaScript, which supplies programmers much more flexibility.
Specifically, we took a have a look at a handful of usages, together with narrowing varieties down and expressing situations on the precise worth of our variables. Lastly, we briefly talked about the variations and similarities with kind guards and grasped the syntactic limitations of assertions capabilities.
Writing lots of TypeScript? Watch the recording of our latest TypeScript meetup to find out about writing extra readable code.
TypeScript brings kind security to JavaScript. There is usually a stress between kind security and readable code. Watch the recording for a deep dive on some new options of TypeScript 4.4.