Feedback are notes that programmers advert to their code to clarify what that code is meant to do. The compilers or interpreters that flip code into motion ignore feedback, however they are often important to managing software program initiatives.
Feedback assist to clarify your Python code to different programmers and might remind you of why you made the alternatives you probably did. Feedback make debugging and revising code simpler by serving to future programmers perceive the design selections behind software program.
Though feedback are primarily for builders, writing efficient ones may help in producing glorious documentation in your code’s customers. With the assistance of doc turbines like Sphinx for Python initiatives, feedback in your code can present content material in your documentation.
Let’s look underneath the hood of commenting in Python.
Feedback in Python
In line with the Python PEP 8 Type Information, there are a number of issues to bear in mind whereas writing feedback:
- Feedback ought to at all times be full and concise sentences.
- It’s higher to haven’t any remark in any respect than one which’s obscure or inaccurate.
- Feedback must be up to date commonly to replicate modifications in your code.
- Too many feedback might be distracting and cut back code high quality. Feedback aren’t wanted the place the code’s goal is apparent.
In Python, a line is said as a remark when it begins with #
image. When the Python interpreter encounters #
in your code, it ignores something after that image and doesn’t produce any error. There are two methods to declare single-line feedback: inline feedback and block feedback.
Inline Feedback
Inline feedback present brief descriptions of variables and easy operations and are written on the identical line because the code assertion:
border = x + 10 # Make offset of 10px
The remark explains the perform of the code in the identical assertion because the code.
Block Feedback
Block feedback are used to explain complicated logic within the code. Block feedback in Python are constructed equally to inline feedback — the one distinction is that block feedback are written on a separate line:
import csv
from itertools import groupby
# Get an inventory of names in a sequence from the csv file
with open('new-top-firstNames.csv') as f:
file_csv = csv.reader(f)
# Skip the header half: (sr, identify, perc)
header = subsequent(file_csv)
# Solely identify from (quantity, identify, perc)
individuals = [ x[1] for x in file_csv]
# Kind the record by first letter as a result of
# The groupby perform seems for sequential knowledge.
individuals.kind(key=lambda x:x[0])
knowledge = groupby(individuals, key=lambda x:x[0])
# Get each identify as an inventory
data_grouped = {}
for okay, v in knowledge:
# Get knowledge within the kind
# {'A' : ["Anthony", "Alex"], "B" : ["Benjamin"]}
data_grouped[k] = record(v)
Notice that when utilizing block feedback, the feedback are written above the code that they’re explaining. The Python PEP8 Type Information dictates {that a} line of code mustn’t include greater than seventy-nine characters, and inline feedback usually push strains over this size. This is the reason block feedback are written to explain the code on separate strains.
Multi-Line Feedback
Python doesn’t natively assist multi-line feedback, which suggests there’s no particular provision for outlining them. Regardless of this, feedback spanning a number of strains are sometimes used.
You may create a multi-line remark out of a number of single-line feedback by prefacing every line with #
:
# interpreter
# ignores
# these strains
You too can use multi-line strings syntax. In Python, you possibly can outline multi-line strings by enclosing them in """
, triple double quotes, or '''
, triple single quotes:
print("Multi-Line Remark")
"""
This
String is
Multi line
"""
Within the code above, the multi-line string is just not assigned to a variable, which makes the string work like a remark. At runtime, Python ignores the string, and it doesn’t get included within the bytecode. Executing the above code produces the next output:
Multi-Line Remark
Particular Feedback
Along with making your code readable, feedback additionally serve some particular functions in Python, comparable to planning future code additions and producing documentation.
Python Docstring Feedback
In Python, docstrings are multi-line feedback that designate use a given perform or class. The documentation of your code is improved by the creation of high-quality docstrings. Whereas working with a perform or class and utilizing the built-in assist(obj)
perform, docstrings is likely to be useful in giving an outline of the item.
Python PEP 257 gives an ordinary technique of declaring docstrings in Python, proven under:
from collections import namedtuple
Particular person = namedtuple('Particular person', ['name', 'age'])
def get_person(identify, age, d=False):
"""
Returns a namedtuple("identify", "age") object.
Additionally returns dict('identify', 'age') if arg `d` is True
Arguments:
identify – first identify, have to be string
age – age of particular person, have to be int
d – to return Particular person as `dict` (default=False)
"""
p = Particular person(identify, age)
if d:
return p._asdict()
return p
Within the code above, the docstring supplied particulars on how the related perform works. With documentation turbines like Sphinx, this docstring can be utilized to offer customers of your venture an outline of use this technique.
A docstring outlined slightly below the perform or class signature may also be returned by utilizing the built-in assist()
perform. The assist()
perform takes an object or perform identify as an argument, and returns the perform’s docstrings as output. Within the instance above, assist(get_person)
might be referred to as to disclose docstrings related to the get_person
perform. In the event you run the code above in an interactive shell utilizing the -i
flag, you possibly can see how this docstring might be parsed by Python. Run the above code by typing python -i file.py
.
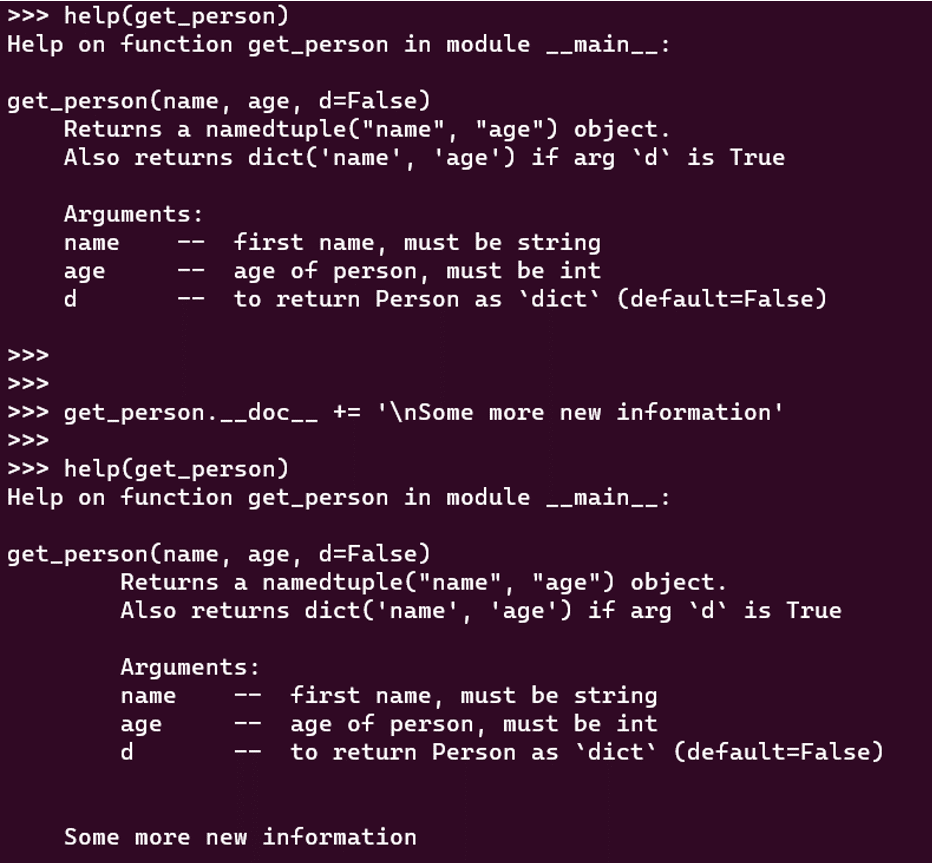
The assist(get_person)
perform name returns a docstring in your perform. The output comprises get_person(identify, age, d=False)
, which is a perform signature that Python provides mechanically.
The get_person.__ doc__
attribute may also be used to retrieve and modify docstrings programmatically. After including “Some extra new data” within the instance above, it seems within the second name to assist(get_person)
. Nonetheless, it’s unlikely that you will want to dynamically alter docstrings at runtime like this.
TODO Feedback
When writing code, there are events while you’ll need to spotlight sure strains or whole blocks for enchancment. These duties are flagged by TODO feedback. TODO feedback come in useful while you’re planning updates or modifications to your code, or should you want to inform the venture’s customers or collaborators that particular sections of the file’s code stay to be written.
TODO feedback shouldn’t be written as pseudocode — they only should briefly clarify the perform of the yet-unwritten code.
TODO feedback and single-line block feedback are very comparable, and the only distinction between them is that TODO feedback should start with a TODO prefix:
# TODO Get serialized knowledge from the CSV file
# TODO Carry out calculations on the information
# TODO Return to the person
It’s vital to notice that though many IDEs can spotlight these feedback for the programmer, the Python interpreter doesn’t view TODO feedback any otherwise from block feedback.
Greatest Practices When Writing Python Feedback
There are a variety of greatest practices that must be adopted when writing feedback to make sure reliability and high quality. Under are some suggestions for writing high-quality feedback in Python.
Keep away from the Apparent
Feedback that state the apparent don’t add any worth to your code, and must be prevented. For instance:
x = x + 4 # improve x by 4
That remark isn’t helpful, because it merely states what the code does with out explaining why it must be executed. Feedback ought to clarify the “why” fairly than the “what” of the code they’re describing.
Rewritten extra usefully, the instance above may appear like this:
x = x + 4 # improve the border width
Hold Python Feedback Brief and Candy
Hold your feedback brief and simply understood. They need to be written in commonplace prose, not pseudocode, and will exchange the necessity to learn the precise code to get a common overview of what it does. An excessive amount of element or complicated feedback don’t make a programmer’s job any simpler. For instance:
# return space by performing, Space of cylinder = (2*PI*r*h) + (2*PI*r*r)
def get_area(r,h):
return (2*3.14*r*h) + (2*3.14*r*r)
The remark above gives extra data than is important for the reader. As a substitute of specifying the core logic, feedback ought to present a common abstract of the code. This remark might be rewritten as:
# return space of cylinder
def get_area(r,h):
return (2*3.14*r*h) + (2*3.14*r*r)
Use Identifiers Rigorously
Identifiers must be used rigorously in feedback. Altering identifier names or circumstances can confuse the reader. Instance:
# return class() after modifying argument
def func(cls, arg):
return cls(arg+5)
The above remark mentions class
and argument
, neither of that are discovered within the code. This remark might be rewritten as:
# return cls() after modifying arg
def func(cls, arg):
return cls(arg+5)
DRY and WET
While you’re writing code, you need to adhere to the DRY (don’t repeat your self) precept and keep away from WET (write every part twice).
That is additionally true for feedback. Keep away from utilizing a number of statements to explain your code, and attempt to merge feedback that designate the identical code right into a single remark. Nevertheless, it’s vital to watch out while you’re merging feedback: careless merging of a number of feedback can lead to an enormous remark that violates model guides and is troublesome for the reader to comply with.
Keep in mind that feedback ought to cut back the studying time of the code.
# perform to do x work
def do_something(y):
# x work can't be executed if y is bigger than max_limit
if y < 400:
print('doing x work')
Within the code above, the feedback are unnecessarily fragmented, and might be merged right into a single remark:
# perform to do x if arg:y is lower than max_limit
def do_something(y):
if y in vary(400):
print('doing x work')
Constant Indentation
Be certain that feedback are indented on the similar stage because the code they’re describing. Once they’re not, they are often troublesome to comply with.
For instance, this remark is just not indented or positioned correctly:
for i in vary(2,20, 2):
# solely even numbers
if confirm(i):
# i must be verified by confirm()
carry out(x)
It may be rewritten as follows:
# solely even numbers
for i in vary(2,20, 2):
# i must be verified by confirm()
if confirm(i):
carry out(x)
Abstract
Feedback are an vital element of writing comprehensible code. The funding you make in writing a remark is one which your future self — or different builders who have to work in your code base — will recognize. Commenting additionally permits you to achieve deeper insights into your code.
On this tutorial, you’ve realized extra about feedback in Python, together with the assorted varieties of Python feedback, when to make use of every of them, and one of the best practices to comply with when creating them.
Writing good feedback is a ability that must be studied and developed. To observe writing feedback, think about going again and including feedback to a few of your earlier initiatives. For inspiration and to see greatest practices in motion, take a look at well-documented Python initiatives on GitHub.
While you’re able to make your individual Python initiatives stay, Kinsta’s Utility Internet hosting platform can get your code from GitHub to the cloud in seconds.
Get all of your functions, databases and WordPress websites on-line and underneath one roof. Our feature-packed, high-performance cloud platform contains:
- Simple setup and administration within the MyKinsta dashboard
- 24/7 professional assist
- The very best Google Cloud Platform {hardware} and community, powered by Kubernetes for optimum scalability
- An enterprise-level Cloudflare integration for pace and safety
- World viewers attain with as much as 35 knowledge facilities and 275+ PoPs worldwide
Take a look at it your self with $20 off your first month of Utility Internet hosting or Database Internet hosting. Discover our plans or speak to gross sales to search out your greatest match.