Correctly typing the youngsters
prop in React can pose some issue at first. For those who strive typing them as particular JSX sorts, you could run into points rendering the kid parts. There’s additionally the issue with the paradox of selection, as there are a number of accessible choices to kind the kids prop. This may increasingly result in determination fatigue.
On this article, I’ll share my advisable options to this, primarily based on expertise. For completeness, I’ll additionally share another, arguably related approaches.
Bounce forward:
Let’s get began.
Kids in JSX
Once you write a JSX expression with opening and shutting tags, the content material handed between them is known as their “youngster”.
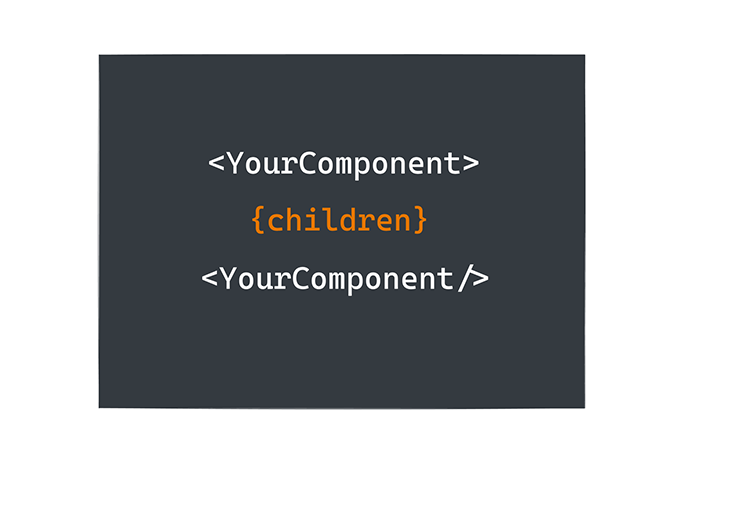
Contemplate the next contrived instance:
<Border> Hey, I symbolize the JSX youngsters! </Border>
On this instance, the literal string Hey, I symbolize the JSX youngsters!
refers back to the youngster rendered inside Border
.
Conversely, to realize entry to the content material handed between JSX closing and opening tags, React passes these in a particular prop: props.youngsters
For instance, Border
might obtain the youngsters
prop as follows:
const Border = ({youngsters}) => { return <div model={{border: "1px strong crimson"}}> {youngsters} </div> }
Border
accepts the youngsters
prop, then renders the youngsters
inside a div
with a border model of 1px strong crimson
.
That is the fundamental utilization of the youngsters
prop, i.e., to obtain and manipulate the content material handed inside the opening and shutting tags of a JSX expression.
Supported youngsters sorts
Strictly talking, there’s a handful of supported content material sorts that may go inside the opening and shutting tags of your JSX expression. Under are a few of the most used ones:
Strings
Literal strings are legitimate youngsters sorts, as proven under:
<YourComponent> This can be a legitimate youngster string </YourComponent />
Notice that in YourComponent
, props.youngsters
will merely be the string This can be a legitimate youngster string
.
JSX
You may equally go different JSX components as legitimate youngsters. That is often useful when composing completely different nested parts. Under’s an instance:
<Wrapper> <YourFirstComponent /> <YourSecondComponent /> </Wrapper>
It is usually utterly acceptable to combine youngsters sorts, as proven under:
<Wrapper> I'm a legitimate string youngster <YourFirstComponent /> <YourSecondComponent /> </Wrapper>
JavaScript expressions
Expressions are equally legitimate youngsters sorts, as proven under:
<YourFirstComponent> {myScopedVariableReference} </YourFirstComponent>
Do not forget that expressions in JSX
are written in curly braces.
Features
Features are equally legitimate youngsters sorts as proven under:
<YourFirstComponent> {() => <div>{myScopedVariableReference}</div>} </YourFirstComponent>
As you may see, the youngsters
prop might be represented by fairly a variety of information sorts! Your first inclination is likely to be to kind these out manually, like so:
kind Props = () => JSX.Factor const YourComponent = ({youngsters} : Props) => { return youngsters }
This may appear to be a good suggestion, however it doesn’t totally symbolize the youngsters
prop. What about fragments, portals, and ignored render values, akin to undefined
, null
, true
, or false
?
A full illustration could look one thing like this:
kind ReactText = string | quantity; kind ReactChild = ReactElement | ReactText; interface ReactNodeArray extends Array<ReactNode> {} kind ReactFragment = {} | ReactNodeArray; kind ReactNode = ReactChild | ReactFragment | ReactPortal | boolean | null | undefined; kind Props = { youngsters: ReactNode } // supply: https://github.com/DefinitelyTyped/DefinitelyTyped/blob/d076add9f29db350a19bd94c37b197729cc02f87/sorts/react/index.d.ts
See the prolonged sorts for ReactPortal and ReactElement. Do they appear advanced? There’s a very good likelihood they do.
The purpose I’m making an attempt to make is, in observe, you don’t need to kind the youngsters
prop manually. As an alternative, I recommend utilizing the formally supported sorts mentioned under.
Utilizing the PropsWithChildren
kind
The React.PropsWithChildren
kind takes your element prop and returns a union kind with the youngsters
prop appropriately typed. No further work from you wanted.
In observe, right here’s the definition for the PropsWithChildren
kind:
kind PropsWithChildren<P> = P & { youngsters?: ReactNode };
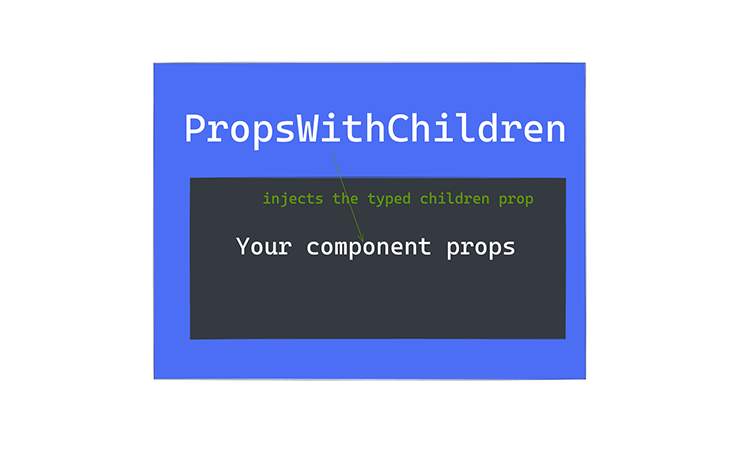
propsWithChildren
kind worksAssuming you had a element Foo
with props FooProps
:
kind FooProps = { title: 'foo' } export const Foo = (props: FooProps) => { return null }
You may go forward and introduce the youngsters
prop as follows:
import { PropsWithChildren } from 'react' kind FooProps = { title: 'foo' } export const Foo = (props: PropsWithChildren<FooProps>) => { return props.youngsters }
Once you go PropsWithChildren
to your element prop FooProps
, you get the youngsters
prop internally typed.
Usually, that is the advisable approach to go about typing the youngsters
prop as a result of it requires much less boilerplate and the youngsters
prop is implicitly typed.
Explicitly utilizing the ReactNode
kind
In circumstances the place it’s essential to explicitly kind the youngsters
prop, you may go forward and use the ReactNode
kind.
Keep in mind the definition for the PropsWithChildren
kind:
kind PropsWithChildren<P> = P & { youngsters?: ReactNode };
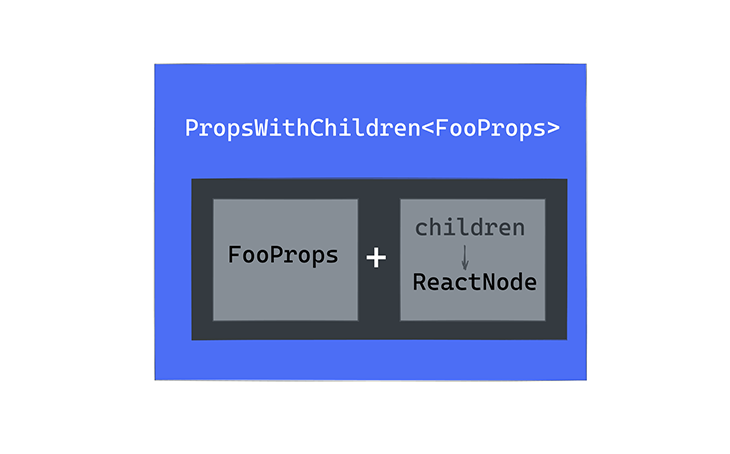
As an alternative of counting on PropsWithChildren
, it’s also possible to kind the youngsters
prop instantly:
import { ReactNode } from 'react' kind FooProps = { title: 'foo' // look right here 👇 youngsters: ReactNode } export const Foo = (props: FooProps) => { return props.youngsters }
Utilizing the FunctionComponent
(or FC
) kind
The FunctionComponent
generic interface might also be used to appropriately kind the youngsters
prop. Internally, this interface depends on PropsWithChildren
.
Right here’s the way you’d use this:
import { FunctionComponent } from 'react' kind FooProps = { title: 'foo' } export const Foo: FunctionComponent<FooProps> = (props) => { return props.youngsters }
Notice that the FC
kind is an alias for FunctionComponent
for ease. Their usages are comparable, as proven under:
import { FC } from 'react' kind FooProps = { title: 'foo' } export const Foo: FC<FooProps> = (props) => { return props.youngsters }
Utilizing the Element
kind for sophistication parts
Most trendy React codebases not use class parts, besides in particular use circumstances.
If you end up needing to kind the youngsters
prop in a category element, leverage the Element
prop, as proven under:
import { Element } from 'react' kind FooProps = { title: 'foo' } class Foo extends Element<FooProps> { render() { return this.props.youngsters } }
Much like the FunctionComponent
interface and its FC
alias, the Element
kind routinely consists of the youngsters
prop.
Extra nice articles from LogRocket:
Conclusion
The place potential, use the PropsWithChildren
kind, however don’t be afraid to kind the youngsters
prop instantly as properly, whether or not that’s in a category or useful element.
LogRocket: Full visibility into your manufacturing React apps
Debugging React purposes might be tough, particularly when customers expertise points which might be onerous to breed. For those who’re inquisitive about monitoring and monitoring Redux state, routinely surfacing JavaScript errors, and monitoring gradual community requests and element load time,
strive LogRocket.
LogRocket
combines session replay, product analytics, and error monitoring – empowering software program groups to create the perfect net and cellular product expertise. What does that imply for you?
As an alternative of guessing why errors occur, or asking customers for screenshots and log dumps, LogRocket helps you to replay issues as in the event that they occurred in your individual browser to rapidly perceive what went mistaken.
No extra noisy alerting. Sensible error monitoring helps you to triage and categorize points, then learns from this. Get notified of impactful person points, not false positives. Much less alerts, far more helpful sign.
The LogRocket Redux middleware package deal provides an additional layer of visibility into your person classes. LogRocket logs all actions and state out of your Redux shops.
Modernize the way you debug your React apps —
begin monitoring without spending a dime.
LogRocket: Full visibility into your net and cellular apps
LogRocket is a frontend software monitoring resolution that allows you to replay issues as in the event that they occurred in your individual browser. As an alternative of guessing why errors occur, or asking customers for screenshots and log dumps, LogRocket helps you to replay the session to rapidly perceive what went mistaken. It really works completely with any app, no matter framework, and has plugins to log extra context from Redux, Vuex, and @ngrx/retailer.
Along with logging Redux actions and state, LogRocket data console logs, JavaScript errors, stacktraces, community requests/responses with headers + our bodies, browser metadata, and customized logs. It additionally devices the DOM to document the HTML and CSS on the web page, recreating pixel-perfect movies of even probably the most advanced single-page and cellular apps.