Should you’re trying to construct a real-time chat app that’s each quick and dependable, think about using Rust and React. Rust is understood for its pace and reliability, whereas React is likely one of the hottest frontend frameworks for constructing person interfaces.
On this article, we’ll show the way to construct a real-time chat app with Rust and React that gives performance for chat, checking person standing, and indicating when a person is typing. We’ll use WebSockets to allow the two-way client-server communication.
Soar forward:
Introduction to real-time chat purposes
Actual-time chat purposes enable customers to speak with one another in actual time by way of textual content, voice, or video. This kind of app permits for extra quick messaging than different forms of communication corresponding to e mail or IM.
There are a number of the explanation why chat purposes should work in actual time:
- Improved efficiency: Extra quick communication permits for extra pure dialog
- Better responsiveness: Actual-time performance leads to improved person expertise
- Superior reliability: With real-time performance there‘s much less alternative for messages to be misplaced or delayed
Introduction to WebSockets
WebSockets permits two-way communication between the shopper and server in real-time chat purposes. Utilizing Rust to construct the WebSocket server will allow the server to deal with a lot of connections with out slowing down. This is because of Rust’s pace and reliability.
Now that now we have a greater understanding of WebSockets, let’s get began constructing our real-time chat utility!
Getting began
First, let’s assessment some conditions:
- Rust: Guarantee you may have Rust put in in your pc. Should you don’t have it, use the next command to put in it:
curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh // if you're in home windows see extra set up methodology right here https://forge.rust-lang.org/infra/other-installation-methods.html
- React: Be sure that your atmosphere is prepared for React growth; use one of many beneath instructions to put in React should you don’t have already got it:
// on mac brew set up node // on linux nvm set up v14.10.0 // on home windows you'll be able to obtain nodejs installer right here https://nodejs.org/en/obtain/
Subsequent, run the next instructions to confirm that all the pieces is put in and dealing correctly:
rustc --version cargo --version node --version npm --version
Designing the real-time chat app structure
Let’s create some design structure for our real-time chat utility. We’ll construct a easy server; our utility’s structure will cowl the next options:
- Chat: between two customers through direct messaging
- Typing indicator: notifies the recipient when a person begins typing a chat to them
- Person standing: signifies whether or not the person is on-line or offline
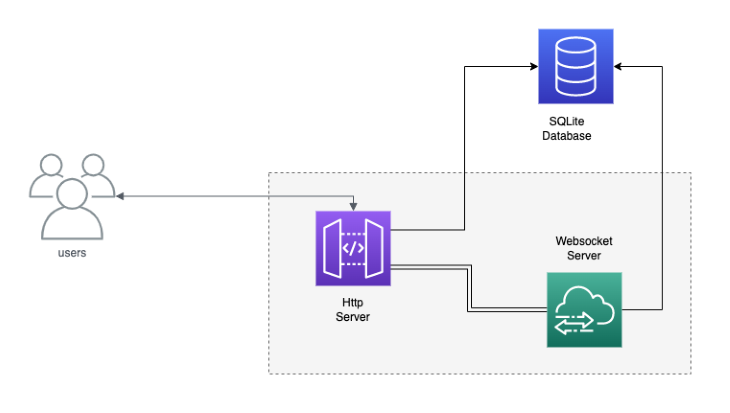
This structure may be very easy and straightforward to comply with. It consists of just some parts:
- WebSocket server: That is crucial part of our utility; it handles all of the communication between shoppers and rooms
- Room supervisor: This part is answerable for managing all of the rooms in our utility. It should create, replace, and delete rooms. This part will likely be on the HTTP server
- Person supervisor: This part is answerable for managing all of the customers in our utility. It should create, replace, and delete customers. This part will likely be on the HTTP server as nicely
- Message supervisor: This part is answerable for managing all of the messages in our utility. It should create, replace, and delete messages. This part one will likely be on the WebSocket server and the HTTP server. Will probably be used to retailer incoming messages from WebSockets and retrieve all messages already within the database when the person opens the chat room through the Relaxation API
Constructing the WebSocket server in Rust
There are various packages we will use to put in writing a WebSocket server in Rust. For this tutorial, we’ll use Actix Net; it’s a mature package deal and is simple to make use of.
To begin, create a Rust venture utilizing the next command:
cargo new rust-react-chat
Subsequent, add this package deal to the Cargo.toml
file:
[package] title = "rust-react-chat" model = "0.1.0" version = "2021" [dependencies] actix = "0.13.0" actix-files = "0.6.2" actix-web = "4.2.1" actix-web-actors = "4.1.0" rand = "0.8.5" serde = "1.0.147" serde_json = "1.0.88"
Now, set up diesel_cli
; we’ll use this as our ORM:
cargo set up diesel_cli --no-default-features --features sqlite
Right here’s how the construction of the venture ought to look:
. ├── Cargo.lock ├── Cargo.toml ├── README.md ├── chat.db ├── .env └── src ├── db.rs ├── major.rs ├── fashions.rs ├── routes.rs ├── schema.rs ├── server.rs └── session.rs └── static └── ui
Now, right here’s a little bit of details about the folders:
src
: This folder incorporates all of our Rust codestatic
: This folder incorporates all of our static property, HTML recordsdata, JavaScript recordsdata, and picturesui
: This folder incorporates our React code; we’ll compile it later to thestatic
file and export it to thestatic
folder
Subsequent, let’s write the entry level for our WebSocket server:
// src/major.rs #[macro_use] extern crate diesel; use actix::*; use actix_cors::Cors; use actix_files::Recordsdata; use actix_web::{internet, http, App, HttpServer}; use diesel::{ prelude::*, r2d2::{self, ConnectionManager}, }; mod db; mod fashions; mod routes; mod schema; mod server; mod session; #[actix_web::main] async fn major() -> std::io::End result<()> { let server = server::ChatServer::new().begin(); let conn_spec = "chat.db"; let supervisor = ConnectionManager::<SqliteConnection>::new(conn_spec); let pool = r2d2::Pool::builder().construct(supervisor).anticipate("Didn't create pool."); let server_addr = "127.0.0.1"; let server_port = 8080; let app = HttpServer::new(transfer || { let cors = Cors::default() .allowed_origin("http://localhost:3000") .allowed_origin("http://localhost:8080") .allowed_methods(vec!["GET", "POST"]) .allowed_headers(vec![http::header::AUTHORIZATION, http::header::ACCEPT]) .allowed_header(http::header::CONTENT_TYPE) .max_age(3600); App::new() .app_data(internet::Knowledge::new(server.clone())) .app_data(internet::Knowledge::new(pool.clone())) .wrap(cors) .service(internet::useful resource("https://weblog.logrocket.com/").to(routes::index)) .route("/ws", internet::get().to(routes::chat_server)) .service(routes::create_user) .service(routes::get_user_by_id) .service(routes::get_user_by_phone) .service(routes::get_conversation_by_id) .service(routes::get_rooms) .service(Recordsdata::new("https://weblog.logrocket.com/", "./static")) }) .staff(2) .bind((server_addr, server_port))? .run(); println!("Server working at http://{server_addr}:{server_port}/"); app.await }
Right here’s some details about the packages we’re utilizing:
actix_cors
: Might be used to debug the UI; we’ll settle for POST and GET requests fromlocalhost:3000
orlocalhost:8080
actix_web
: For all HTTP-related options within the Actix Net package dealactix_files
: For embedding static recordsdata to one in all our routesdiesel
: Might be used to question the info from our SQLite database. Should you desire, you’ll be able to change this to Postgres or MySQLserde_json
: Might be used to parse the JSON knowledge that we’ll ship to the React app
Creating the routes
Now let’s make routes for our server. Since we’ll use a REST HTTP and WebSocket server, we will simply put all the pieces in a single file.
First, add all of the packages we’ll want:
// src/routes.rs use std::time::Instantaneous; use actix::*; use actix_files::NamedFile; use actix_web::{get, put up, internet, Error, HttpRequest, HttpResponse, Responder}; use actix_web_actors::ws; use diesel::{ prelude::*, r2d2::{self, ConnectionManager}, }; use serde_json::json; use uuid::Uuid; use crate::db; use crate::fashions; use crate::server; use crate::session; sort DbPool = r2d2::Pool<ConnectionManager<SqliteConnection>>;
Then, add a route for embedding the house web page to the basis URL:
// src/routes.rs pub async fn index() -> impl Responder { NamedFile::open_async("./static/index.html").await.unwrap() }
That is the entry level for our WebSocket server. Proper now it’s on /ws
routes, however you’ll be able to change it to no matter route title you want. Since we already registered all of the dependencies we’d like within the major.rs
file, we will simply go the dependency to the perform parameter, like so:
// src/routes.rs pub async fn chat_server( req: HttpRequest, stream: internet::Payload, pool: internet::Knowledge<DbPool>, srv: internet::Knowledge<Addr<server::ChatServer>>, ) -> End result<HttpResponse, Error> { ws::begin( session::WsChatSession { id: 0, hb: Instantaneous::now(), room: "major".to_string(), title: None, addr: srv.get_ref().clone(), db_pool: pool, }, &req, stream ) }
Subsequent, we have to add a REST API to our route so as to get the mandatory knowledge to make our chat work:
// src/routes.rs #[post("/users/create")] pub async fn create_user( pool: internet::Knowledge<DbPool>, type: internet::Json<fashions::NewUser>, ) -> End result<HttpResponse, Error> { let person = internet::block(transfer || { let mut conn = pool.get()?; db::insert_new_user(&mut conn, &type.username, &type.cellphone) }) .await? .map_err(actix_web::error::ErrorUnprocessableEntity)?; Okay(HttpResponse::Okay().json(person)) } #[get("/users/{user_id}")] pub async fn get_user_by_id( pool: internet::Knowledge<DbPool>, id: internet::Path<Uuid>, ) -> End result<HttpResponse, Error> { let user_id = id.to_owned(); let person = internet::block(transfer || { let mut conn = pool.get()?; db::find_user_by_uid(&mut conn, user_id) }) .await? .map_err(actix_web::error::ErrorInternalServerError)?; if let Some(person) = person { Okay(HttpResponse::Okay().json(person)) } else { let res = HttpResponse::NotFound().physique( json!({ "error": 404, "message": format!("No person discovered with cellphone: {id}") }) .to_string(), ); Okay(res) } } #[get("/conversations/{uid}")] pub async fn get_conversation_by_id( pool: internet::Knowledge<DbPool>, uid: internet::Path<Uuid>, ) -> End result<HttpResponse, Error> { let room_id = uid.to_owned(); let conversations = internet::block(transfer || { let mut conn = pool.get()?; db::get_conversation_by_room_uid(&mut conn, room_id) }) .await? .map_err(actix_web::error::ErrorInternalServerError)?; if let Some(knowledge) = conversations { Okay(HttpResponse::Okay().json(knowledge)) } else { let res = HttpResponse::NotFound().physique( json!({ "error": 404, "message": format!("No dialog with room_id: {room_id}") }) .to_string(), ); Okay(res) } } #[get("/users/phone/{user_phone}")] pub async fn get_user_by_phone( pool: internet::Knowledge<DbPool>, cellphone: internet::Path<String>, ) -> End result<HttpResponse, Error> { let user_phone = cellphone.to_string(); let person = internet::block(transfer || { let mut conn = pool.get()?; db::find_user_by_phone(&mut conn, user_phone) }) .await? .map_err(actix_web::error::ErrorInternalServerError)?; if let Some(person) = person { Okay(HttpResponse::Okay().json(person)) } else { let res = HttpResponse::NotFound().physique( json!({ "error": 404, "message": format!("No person discovered with cellphone: {}", cellphone.to_string()) }) .to_string(), ); Okay(res) } } #[get("/rooms")] pub async fn get_rooms( pool: internet::Knowledge<DbPool>, ) -> End result<HttpResponse, Error> { let rooms = internet::block(transfer || { let mut conn = pool.get()?; db::get_all_rooms(&mut conn) }) .await? .map_err(actix_web::error::ErrorInternalServerError)?; if !rooms.is_empty() { Okay(HttpResponse::Okay().json(rooms)) } else { let res = HttpResponse::NotFound().physique( json!({ "error": 404, "message": "No rooms obtainable in the meanwhile.", }) .to_string(), ); Okay(res) } }
Now, let’s deal with the WebSocket connection. First, let’s import all of the packages we’d like once more:
// src/server.rs use std::collections::{HashMap, HashSet}; use serde_json::json; use actix::prelude::*; use rand::{self, rngs::ThreadRng, Rng}; use crate::session; #[derive(Message)] #[rtype(result = "()")] pub struct Message(pub String); #[derive(Message)] #[rtype(usize)] pub struct Join { pub addr: Recipient<Message>, } #[derive(Message)] #[rtype(result = "()")] pub struct Disconnect { pub id: usize, } #[derive(Message)] #[rtype(result = "()")] pub struct ClientMessage { pub id: usize, pub msg: String, pub room: String, } pub struct ListRooms; impl actix::Message for ListRooms { sort End result = Vec<String>; } #[derive(Message)] #[rtype(result = "()")] pub struct Be a part of { pub id: usize, pub title: String, }
Subsequent, let’s implement the trait to handle the WebSocket connections. This code will deal with all of the messages coming from customers and ship them again to contributors within the chat room:
// src/server.rs #[derive(Debug)] pub struct ChatServer { classes: HashMap<usize, Recipient<Message>>, rooms: HashMap<String, HashSet<usize>>, rng: ThreadRng, } impl ChatServer { pub fn new() -> ChatServer { let mut rooms = HashMap::new(); rooms.insert("major".to_string(), HashSet::new()); Self { classes: HashMap::new(), rooms, rng: rand::thread_rng() } } fn send_message(&self, room: &str, message: &str, skip_id: usize) { if let Some(classes) = self.rooms.get(room) { for id in classes { if *id != skip_id { if let Some(addr) = self.classes.get(id) { addr.do_send(Message(message.to_owned())); } } } } } } impl Actor for ChatServer { sort Context = Context<Self>; } impl Handler<Join> for ChatServer { sort End result = usize; fn deal with(&mut self, msg: Join, _: &mut Context<Self>) -> Self::End result { let id = self.rng.gen::<usize>(); self.classes.insert(id, msg.addr); self.rooms .entry("major".to_string()) .or_insert_with(HashSet::new) .insert(id); self.send_message("major", &json!({ "worth": vec![format!("{}", id)], "chat_type": session::ChatType::CONNECT }).to_string(), 0); id } } impl Handler<Disconnect> for ChatServer { sort End result = (); fn deal with(&mut self, msg: Disconnect, _: &mut Self::Context) -> Self::End result { let mut rooms: Vec<String> = vec![]; if self.classes.take away(&msg.id).is_some() { for (title, classes) in &mut self.rooms { if classes.take away(&msg.id) { rooms.push(title.to_owned()); } } } for room in rooms { self.send_message("major", &json!({ "room": room, "worth": vec![format!("Someone disconnect!")], "chat_type": session::ChatType::DISCONNECT }).to_string(), 0); } } } impl Handler<ClientMessage> for ChatServer { sort End result = (); fn deal with(&mut self, msg: ClientMessage, _: &mut Self::Context) -> Self::End result { self.send_message(&msg.room, &msg.msg, msg.id); } } impl Handler<ListRooms> for ChatServer { sort End result = MessageResult<ListRooms>; fn deal with(&mut self, _: ListRooms, _: &mut Self::Context) -> Self::End result { let mut rooms = vec![]; for key in self.rooms.keys() { rooms.push(key.to_owned()); } MessageResult(rooms) } } impl Handler<Be a part of> for ChatServer { sort End result = (); fn deal with(&mut self, msg: Be a part of, _: &mut Self::Context) -> Self::End result { let Be a part of {id, title} = msg; let mut rooms = vec![]; for (n, classes) in &mut self.rooms { if classes.take away(&id) { rooms.push(n.to_owned()); } } for room in rooms { self.send_message(&room, &json!({ "room": room, "worth": vec![format!("Someone disconnect!")], "chat_type": session::ChatType::DISCONNECT }).to_string(), 0); } self.rooms .entry(title.clone()) .or_insert_with(HashSet::new) .insert(id); } }
Dealing with the person session
Now, let’s deal with the person session. Right here we’ll obtain a message, reserve it to the database, after which ship it again to the participant within the chat room.
To begin, import all of the packages:
// src/session.rs use std::time::{Length, Instantaneous}; use actix::prelude::*; use actix_web::internet; use actix_web_actors::ws; use serde::{Deserialize, Serialize}; use diesel::{ prelude::*, r2d2::{self, ConnectionManager}, }; use crate::db; use crate::fashions::NewConversation; use crate::server;
You’ll be able to change the period of the connection to the WebSocket right here. So the HEARTBEAT
is the period to maintain the connection alive with the shopper. And CLIENT_TIMEOUT
is the period to verify if the shopper continues to be linked:
// src/session.rs const HEARBEET: Length = Length::from_secs(5); const CLIENT_TIMEOUT: Length = Length::from_secs(10); sort DbPool = r2d2::Pool<ConnectionManager<SqliteConnection>>;
Now let’s create some structs to retailer all the info we’d like:
// src/session.rs #[derive(Debug)] pub struct WsChatSession { pub id: usize, pub hb: Instantaneous, pub room: String, pub title: Possibility<String>, pub addr: Addr<server::ChatServer>, pub db_pool: internet::Knowledge<DbPool>, } #[derive(PartialEq, Serialize, Deserialize)] pub enum ChatType { TYPING, TEXT, CONNECT, DISCONNECT, } #[derive(Serialize, Deserialize)] struct ChatMessage { pub chat_type: ChatType, pub worth: Vec<String>, pub room_id: String, pub user_id: String, pub id: usize, }
This struct will likely be used for the next:
WsChatSession
: To make a customized implementation of the Actix Net actorChatMessage
: To outline the thing that will likely be despatched to and acquired from the person
Now, let’s implement our session’s Actor
and stream Handler
:
// src/session.rs impl Actor for WsChatSession { sort Context = ws::WebsocketContext<Self>; fn began(&mut self, ctx: &mut Self::Context) { self.hb(ctx); let addr = ctx.deal with(); self.addr .ship(server::Join { addr: addr.recipient(), }) .into_actor(self) .then(|res, act, ctx| { match res { Okay(res) => act.id = res, _ => ctx.cease(), } fut::prepared(()) }) .wait(ctx); } fn stopping(&mut self, _: &mut Self::Context) -> Operating { self.addr.do_send(server::Disconnect { id: self.id }); Operating::Cease } } impl Handler<server::Message> for WsChatSession { sort End result = (); fn deal with(&mut self, msg: server::Message, ctx: &mut Self::Context) -> Self::End result { ctx.textual content(msg.0); } } impl StreamHandler<End result<ws::Message, ws::ProtocolError>> for WsChatSession { fn deal with(&mut self, merchandise: End result<ws::Message, ws::ProtocolError>, ctx: &mut Self::Context) { let msg = match merchandise { Err(_) => { ctx.cease(); return; } Okay(msg) => msg, }; match msg { ws::Message::Ping(msg) => { self.hb = Instantaneous::now(); ctx.pong(&msg); } ws::Message::Pong(_) => { self.hb = Instantaneous::now(); } ws::Message::Textual content(textual content) => { let data_json = serde_json::from_str::<ChatMessage>(&textual content.to_string()); if let Err(err) = data_json { println!("{err}"); println!("Didn't parse message: {textual content}"); return; } let enter = data_json.as_ref().unwrap(); match &enter.chat_type { ChatType::TYPING => { let chat_msg = ChatMessage { chat_type: ChatType::TYPING, worth: enter.worth.to_vec(), id: self.id, room_id: enter.room_id.to_string(), user_id: enter.user_id.to_string(), }; let msg = serde_json::to_string(&chat_msg).unwrap(); self.addr.do_send(server::ClientMessage { id: self.id, msg, room: self.room.clone(), }) } ChatType::TEXT => { let enter = data_json.as_ref().unwrap(); let chat_msg = ChatMessage { chat_type: ChatType::TEXT, worth: enter.worth.to_vec(), id: self.id, room_id: enter.room_id.to_string(), user_id: enter.user_id.to_string(), }; let mut conn = self.db_pool.get().unwrap(); let new_conversation = NewConversation { user_id: enter.user_id.to_string(), room_id: enter.room_id.to_string(), message: enter.worth.be a part of(""), }; let _ = db::insert_new_conversation(&mut conn, new_conversation); let msg = serde_json::to_string(&chat_msg).unwrap(); self.addr.do_send(server::ClientMessage { id: self.id, msg, room: self.room.clone(), }) } _ => {} } } ws::Message::Binary(_) => println!("Unsupported binary"), ws::Message::Shut(cause) => { ctx.shut(cause); ctx.cease(); } ws::Message::Continuation(_) => { ctx.cease(); } ws::Message::Nop => (), } } } impl WsChatSession { fn hb(&self, ctx: &mut ws::WebsocketContext<Self>) { ctx.run_interval(HEARBEET, |act, ctx| { if Instantaneous::now().duration_since(act.hb) > CLIENT_TIMEOUT { act.addr.do_send(server::Disconnect { id: act.id }); ctx.cease(); return; } ctx.ping(b""); }); } }
Making ready the database
Subsequent, let’s put together the database. We’ll use SQLite to maintain issues easy. Right here’s how the schema will look:
The desk will likely be used for the next:
customers
: Retailer person knowledge. Since we’re not implementing a full authentication system presently, we’ll solely save the username and cellphone quantity for nowrooms
: Retailer a listing of all chat roomsconversations
: Lists the place all messages are saved in our database
Subsequent, let’s generate the database migration for our schema:
// shell diesel migration generate create_users diesel migration generate create_rooms diesel migration generate create_conversations
Right here’s how the migration SQL will look:
-- migrations/2022-11-21-101206_create_users/up.sql CREATE TABLE customers ( id TEXT PRIMARY KEY NOT NULL, username VARCHAR NOT NULL, cellphone VARCHAR NOT NULL, created_at TEXT NOT NULL, distinctive(cellphone) ) -- migrations/2022-11-21-101215_create_rooms/up.sql CREATE TABLE rooms ( id TEXT PRIMARY KEY NOT NULL, title VARCHAR NOT NULL, last_message TEXT NOT NULL, participant_ids TEXT NOT NULL, created_at TEXT NOT NULL ) -- migrations/2022-11-21-101223_create_conversations/up.sql CREATE TABLE conversations ( id TEXT PRIMARY KEY NOT NULL, room_id TEXT NOT NULL, user_id TEXT NOT NULL, content material VARCHAR NOT NULL, created_at TEXT NOT NULL )
We additionally want so as to add some dummy knowledge simply to have some examples for preliminary rendering to the shopper later:
diesel migration generate dummy_data
Right here’s how the info will look:
-- migrations/2022-11-24-034153_generate_dummy_data/up.sql INSERT INTO customers(id, username, cellphone, created_at) VALUES ("4fbd288c-d3b2-4f78-adcf-def976902d50","Ahmad Rosid","123","2022-11-23T07:56:30.214162+00:00"), ("1e9a12c1-e98c-4a83-a55a-32cc548a169d","Ashley Younger","345","2022-11-23T07:56:30.214162+00:00"), ("1bc833808-05ed-455a-9d26-64fe1d96d62d","Charles Edward","678","2022-12-23T07:56:30.214162+00:00"); INSERT INTO rooms(id, title, last_message, participant_ids, created_at) VALUES ("f061383b-0393-4ce8-9a85-f31d03762263", "Charles Edward", "Hello, how are you?", "1e9a12c1-e98c-4a83-a55a-32cc548a169d,1bc833808-05ed-455a-9d26-64fe1d96d62d", "2022-12-23T07:56:30.214162+00:00"), ("008e9dc4-f01d-4429-ba31-986d7e63cce8", "Ahmad Rosid", "Hello... are free at this time?", "1e9a12c1-e98c-4a83-a55a-32cc548a169d,1bc833808-05ed-455a-9d26-64fe1d96d62d", "2022-12-23T07:56:30.214162+00:00"); INSERT INTO conversations(id, user_id, room_id, content material, created_at) VALUES ("9aeab1a7-e063-40d1-a120-1f7585fa47d6", "1bc833808-05ed-455a-9d26-64fe1d96d62d", "f061383b-0393-4ce8-9a85-f31d03762263", "Hiya", "2022-12-23T07:56:30.214162+00:00"), ("f4e54e70-736b-4a79-a622-3659b0b555e8", "1e9a12c1-e98c-4a83-a55a-32cc548a169d", "f061383b-0393-4ce8-9a85-f31d03762263", "Hello, how are you?", "2022-12-23T07:56:30.214162+00:00"), ("d3ea6e39-ed58-4613-8922-b78f14a2676a", "1bc833808-05ed-455a-9d26-64fe1d96d62d", "008e9dc4-f01d-4429-ba31-986d7e63cce8", "Hello... are free at this time?", "2022-12-23T07:56:30.214162+00:00");
Producing the schema
Now let’s generate the schema and run the migration:
diesel database setup diesel migration run
The schema that’s generated routinely by the CLI will seem like this:
// src/schema.rs // @generated routinely by Diesel CLI. diesel::desk! { conversations (id) { id -> Textual content, room_id -> Textual content, user_id -> Textual content, content material -> Textual content, created_at -> Textual content, } } diesel::desk! { rooms (id) { id -> Textual content, title -> Textual content, last_message -> Textual content, participant_ids -> Textual content, created_at -> Textual content, } } diesel::desk! { customers (id) { id -> Textual content, username -> Textual content, cellphone -> Textual content, created_at -> Textual content, } } diesel::allow_tables_to_appear_in_same_query!( conversations, rooms, customers, );
The above code is auto generated, so don’t make any modifications to this file.
Creating the structs
Let’s create some structs to retailer all of the tables. One factor to bear in mind is that the order of the property within the struct must be the identical as that within the schema file. You’ll get the incorrect knowledge if the order doesn’t match.
// src/mannequin.rs use serde::{Deserialize, Serialize}; use crate::schema::*; #[derive(Debug, Clone, Serialize, Deserialize, Queryable, Insertable)] pub struct Person { pub id: String, pub username: String, pub cellphone: String, pub created_at: String } #[derive(Debug, Clone, PartialEq, Serialize, Deserialize, Queryable, Insertable)] pub struct Dialog { pub id: String, pub room_id: String, pub user_id: String, pub content material: String, pub created_at: String } #[derive(Debug, Clone, Serialize, Deserialize, Queryable, Insertable)] pub struct Room { pub id: String, pub title: String, pub last_message: String, pub participant_ids: String, pub created_at: String, } #[derive(Debug, Clone, Serialize, Deserialize)] pub struct NewUser { pub username: String, pub cellphone: String, } #[derive(Debug, Clone, Serialize, Deserialize)] pub struct NewConversation { pub user_id: String, pub room_id: String, pub message: String, } #[derive(Debug, Clone, Serialize, Deserialize)] pub struct RoomResponse { pub room: Room, pub customers: Vec<Person>, }
Establishing the queries
Now, let’s fetch knowledge from the database.
First, import the dependency:
// src/db.rs use chrono::{DateTime, Utc}; use diesel::prelude::*; use std::{ collections::{HashMap, HashSet}, time::SystemTime, }; use uuid::Uuid; use crate::fashions::{Dialog, NewConversation, Room, RoomResponse, Person}; sort DbError = Field<dyn std::error::Error + Ship + Sync>;
Since SQLite doesn’t have a date performance construct, we’ll create one:
// src/db.rs fn iso_date() -> String { let now = SystemTime::now(); let now: DateTime<Utc> = now.into(); return now.to_rfc3339(); }
Discovering customers by cellphone quantity
Right here, we’ll arrange a question that can implement a easy login function and allow us to discover a person by cellphone quantity. We’re utilizing this login methodology for instance solely. In manufacturing, you’ll wish to use a way that may be simply verified and debugged:
// src/db.rs pub fn find_user_by_phone( conn: &mut SqliteConnection, user_phone: String, ) -> End result<Possibility<Person>, DbError> { use crate::schema::customers::dsl::*; let person = customers .filter(cellphone.eq(user_phone)) .first::<Person>(conn) .elective()?; Okay(person) }
Including a brand new person
Right here’s a question for storing a brand new person who registers for our app. That is additionally a part of our authentication system. Once more, please don’t use this method to your manufacturing app:
// src/db.rs pub fn insert_new_user(conn: &mut SqliteConnection, nm: &str, pn: &str) -> End result<Person, DbError> { use crate::schema::customers::dsl::*; let new_user = Person { id: Uuid::new_v4().to_string(), username: nm.to_owned(), cellphone: pn.to_owned(), created_at: iso_date(), }; diesel::insert_into(customers).values(&new_user).execute(conn)?; Okay(new_user) }
With the brand new person added, we now insert new conversations:
// src/db.rs pub fn insert_new_conversation( conn: &mut SqliteConnection, new: NewConversation, ) -> End result<Dialog, DbError> { use crate::schema::conversations::dsl::*; let new_conversation = Dialog { id: Uuid::new_v4().to_string(), user_id: new.user_id, room_id: new.room_id, content material: new.message, created_at: iso_date(), }; diesel::insert_into(conversations) .values(&new_conversation) .execute(conn)?; Okay(new_conversation) }
Discovering chat rooms and contributors
Subsequent, let’s arrange a question to fetch all of the chat rooms and contributors from the database:
// src/db.rs pub fn get_all_rooms(conn: &mut SqliteConnection) -> End result<Vec<RoomResponse>, DbError> { use crate::schema::rooms; use crate::schema::customers; let rooms_data: Vec<Room> = rooms::desk.get_results(conn)?; let mut ids = HashSet::new(); let mut rooms_map = HashMap::new(); let knowledge = rooms_data.to_vec(); for room in &knowledge { let user_ids = room .participant_ids .cut up(",") .into_iter() .gather::<Vec<_>>(); for id in user_ids.to_vec() { ids.insert(id.to_string()); } rooms_map.insert(room.id.to_string(), user_ids.to_vec()); } let ids = ids.into_iter().gather::<Vec<_>>(); let users_data: Vec<Person> = customers::desk .filter(customers::id.eq_any(ids)) .get_results(conn)?; let users_map: HashMap<String, Person> = HashMap::from_iter( users_data .into_iter() .map(|merchandise| (merchandise.id.to_string(), merchandise)), ); let response_rooms = rooms_data.into_iter().map(|room| { let customers = rooms_map .get(&room.id.to_string()) .unwrap() .into_iter() .map(|id| users_map.get(id.to_owned()).unwrap().clone()) .gather::<Vec<_>>(); return RoomResponse{ room, customers }; }).gather::<Vec<_>>(); Okay(response_rooms) }
Constructing the shopper UI with React
Let’s design a person interface for our shopper app; the tip consequence will seem like this:
To begin, create a UI venture with Subsequent.js:
yarn create next-app --js ui
Add Tailwind CSS to the venture:
npm set up -D tailwindcss postcss autoprefixer npx tailwindcss init -p
Now, change the Tailwind config
file:
// ui/tailwind.config.js content material: [ "./pages/**/*.{js,ts,jsx,tsx}", "./components/**/*.{js,ts,jsx,tsx}", ]
We’ll add this package deal.json
config to export our Subsequent.js app as static HTML pages in order that we will entry them by way of the file server utilizing Actix Net:
// ui/package deal.json { "title": "ui", "model": "0.1.0", "personal": true, "scripts": { "dev": "subsequent dev", "construct": "subsequent construct && subsequent export -o ../static", ...
Subsequent, import the Tailwind CSS utility to the globals.css
file:
// ui/types/international.css @tailwind base; @tailwind parts; @tailwind utilities;
Now, let’s create some parts for our shopper app.
avatar
part
Right here we’ll create the avatar for every person:
// ui/parts/avatar.js perform getShortName(full_name="") { if (full_name.contains(" ")) { const names = full_name.cut up(" "); return `${names[0].charAt(0)}${names[1].charAt(0)}`.toUpperCase() } return `${full_name.slice(0,2)}`.toUpperCase() } export default perform Avatar({ youngsters, coloration="" }) { return ( <div className="bg-blue-500 w-[45px] h-[45px] flex items-center justify-center rounded-full" fashion={{backgroundColor: coloration}}> <span className="font-bold text-sm text-white">{getShortName(youngsters)}</span> </div> ) }
login
part
Right here we’ll create the person login part:
// ui/parts/login.js import { useState } from "react"; async perform createAccount({ username, cellphone }) { attempt { const url = "http://localhost:8080/customers/create"; let consequence = await fetch(url, { methodology: "POST", headers: { "Content material-Sort": "utility/json" }, physique: JSON.stringify({ username, cellphone }) }); return consequence.json(); } catch (e) { return Promise.reject(e); } } async perform signIn({ cellphone }) { attempt { const url = "http://localhost:8080/customers/cellphone/" + cellphone; let consequence = await fetch(url); return consequence.json(); } catch (e) { return Promise.reject(e); } } export default perform Login({ present, setAuth }) { const [isShowSigIn, setShowSignIn] = useState(false); const showSignIn = () => { setShowSignIn(prev => !prev) } const FormCreateUsername = ({ setAuth }) => { const onCreateUsername = async (e) => { e.preventDefault(); let username = e.goal.username.worth; let cellphone = e.goal.cellphone.worth; if (username === "" || cellphone === "") { return; } let res = await createAccount({ username, cellphone }); if (res === null) { alert("Didn't create account"); return; } setAuth(res) } return ( <type motion="" className="mt-4 space-y-2" onSubmit={onCreateUsername}> <div> <label className="text-sm font-light">Username</label> <enter required sort="textual content" title="username" placeholder="John Doe" className="w-full px-4 py-2 mt-2 border rounded-md focus:outline-none focus:ring-1 focus:ring-blue-600" /> </div> <div> <label className="text-sm font-light">Telephone</label> <enter required sort="textual content" title="cellphone" placeholder="+1111..." className="w-full px-4 py-2 mt-2 border rounded-md focus:outline-none focus:ring-1 focus:ring-blue-600" /> </div> <div className="flex items-baseline justify-between"> <button sort="submit" className="px-6 py-2 mt-4 text-white bg-violet-600 rounded-lg hover:bg-violet-700 w-full">Submit</button> </div> <div className="pt-2 space-y-2 text-center"> <p className="text-base text-gray-700">Have already got a username? <button onClick={showSignIn} className="text-violet-700 font-light">Signal In</button></p> </div> </type> ) } const FormSignIn = ({ setAuth }) => { const onSignIn = async (e) => { e.preventDefault(); let cellphone = e.goal.cellphone.worth; if (cellphone === "") { return; } let res = await signIn({ cellphone }); if (res === null) { alert("Didn't create account"); return; } if (!res.id) { alert(`Telephone quantity not discovered ${cellphone}`); return; } setAuth(res) } return ( <type motion="" className="mt-4 space-y-2" onSubmit={onSignIn}> <div> <label className="text-sm font-light">Telephone</label> <enter required sort="textual content" title="cellphone" placeholder="+1111..." className="w-full px-4 py-2 mt-2 border rounded-md focus:outline-none focus:ring-1 focus:ring-blue-600" /> </div> <div className="flex items-baseline justify-between"> <button sort="submit" className="px-6 py-2 mt-4 text-white bg-violet-600 rounded-lg hover:bg-violet-700 w-full">Submit</button> </div> <div className="pt-2 space-y-2 text-center"> <p className="text-base text-gray-700">Do not have username? <button onClick={showSignIn} className="text-violet-700 font-light">Create</button></p> </div> </type> ) } return ( <div className={`${present ? '' : 'hidden'} bg-gradient-to-b from-orange-400 to-rose-400`}> <div className="flex items-center justify-center min-h-screen"> <div className="px-8 py-6 mt-4 text-left bg-white max-w-[400px] w-full rounded-xl shadow-lg"> <h3 className="text-xl text-slate-800 font-semibold">{isShowSigIn ? 'Log in along with your cellphone.' : 'Create your account.'}</h3> {isShowSigIn ? <FormSignIn setAuth={setAuth} /> : <FormCreateUsername setAuth={setAuth} />} </div> </div> </div> ) }
room
part
Right here we’ll create the chat room parts:
// ui/parts/room.js import React, { useState, useEffect } from "react"; import Avatar from "./avatar"; async perform getRooms() { attempt { const url = "http://localhost:8080/rooms"; let consequence = await fetch(url); return consequence.json(); } catch (e) { console.log(e); return Promise.resolve(null); } } perform ChatListItem({ onSelect, room, userId, index, selectedItem }) { const { customers, created_at, last_message } = room; const energetic = index == selectedItem; const date = new Date(created_at); const ampm = date.getHours() >= 12 ? 'PM' : 'AM'; const time = `${date.getHours()}:${date.getMinutes()} ${ampm}` const title = customers?.filter(person => person.id != userId).map(person => person.username)[0]; return ( <div onClick={() => onSelect(index, {})} className={`${energetic ? 'bg-[#FDF9F0] border border-[#DEAB6C]' : 'bg-[#FAF9FE] border border-[#FAF9FE]'} p-2 rounded-[10px] shadow-sm cursor-pointer`} > <div className="flex justify-between items-center gap-3"> <div className="flex gap-3 items-center w-full"> <Avatar>{title}</Avatar> <div className="w-full max-w-[150px]"> <h3 className="font-semibold text-sm text-gray-700">{title}</h3> <p className="font-light text-xs text-gray-600 truncate">{last_message}</p> </div> </div> <div className="text-gray-400 min-w-[55px]"> <span className="text-xs">{time}</span> </div> </div> </div> ) } export default perform ChatList({ onChatChange, userId }) { const [data, setData] = useState([]) const [isLoading, setLoading] = useState(false) const [selectedItem, setSelectedItem] = useState(-1); useEffect(() => { setLoading(true) getRooms() .then((knowledge) => { setData(knowledge) setLoading(false) }) }, []) const onSelectedChat = (idx, merchandise) => { setSelectedItem(idx) let mapUsers = new Map(); merchandise.customers.forEach(el => { mapUsers.set(el.id, el); }); const customers = { get: (id) => { return mapUsers.get(id).username; }, get_target_user: (id) => { return merchandise.customers.filter(el => el.id != id).map(el => el.username).be a part of("") } } onChatChange({ ...merchandise.room, customers }) } return ( <div className="overflow-hidden space-y-3"> {isLoading && <p>Loading chat lists.</p>} { knowledge.map((merchandise, index) => { return <ChatListItem onSelect={(idx) => onSelectedChat(idx, merchandise)} room={{ ...merchandise.room, customers: merchandise.customers }} index={index} key={merchandise.room.id} userId={userId} selectedItem={selectedItem} /> }) } </div> ) }
dialog
part
Right here we’ll create the person dialog part:
// ui/parts/dialog.js import React, { useEffect, useRef } from "react"; import Avatar from "./avatar" perform ConversationItem({ proper, content material, username }) { if (proper) { return ( <div className="w-full flex justify-end"> <div className="flex gap-3 justify-end"> <div className="max-w-[65%] bg-violet-500 p-3 text-sm rounded-xl rounded-br-none"> <p className="text-white">{content material}</p> </div> <div className="mt-auto"> <Avatar>{username}</Avatar> </div> </div> </div> ) } return ( <div className="flex gap-3 w-full"> <div className="mt-auto"> <Avatar coloration="rgb(245 158 11)">{username}</Avatar> </div> <div className="max-w-[65%] bg-gray-200 p-3 text-sm rounded-xl rounded-bl-none"> <p>{content material}</p> </div> </div> ) } export default perform Dialog({ knowledge, auth, customers }) { const ref = useRef(null); useEffect(() => { ref.present?.scrollTo(0, ref.present.scrollHeight) }, [data]); return ( <div className="p-4 space-y-4 overflow-auto" ref={ref}> { knowledge.map(merchandise => { return <ConversationItem proper={merchandise.user_id === auth.id} content material={merchandise.content material} username={customers.get(merchandise.user_id)} key={merchandise.id} /> }) } </div> ) }
Now let’s put together the Hooks wanted to work together with our WebSocket server and REST API server.
useWebsocket
Hook
This Hook is for connecting to the WebSocket server, enabling us to ship and obtain messages:
// ui/libs/websocket.js import { useEffect, useRef } from "react"; export default perform useWebsocket(onMessage) { const ws = useRef(null); useEffect(() => { if (ws.present !== null) return; const wsUri = 'ws://localhost:8080/ws'; ws.present = new WebSocket(wsUri); ws.present.onopen = () => console.log("ws opened"); ws.present.onclose = () => console.log("ws closed"); const wsCurrent = ws.present; return () => { wsCurrent.shut(); }; }, []); useEffect(() => { if (!ws.present) return; ws.present.onmessage = e => { onMessage(e.knowledge) }; }, []); const sendMessage = (msg) => { if (!ws.present) return; ws.present.ship(msg); } return sendMessage; }
useLocalStorage
Hook
This Hook permits us to get the person knowledge from localStorage:
// ui/libs/useLocalStorage import { useEffect, useState } from "react"; export default perform useLocalStorage(key, defaultValue) { const [storedValue, setStoredValue] = useState(defaultValue); const setValue = (worth) => { attempt { const valueToStore = worth instanceof Perform ? worth(storedValue) : worth; setStoredValue(valueToStore); if (typeof window !== "undefined") { window.localStorage.setItem(key, JSON.stringify(valueToStore)); } } catch (error) { } }; useEffect(() => { attempt { const merchandise = window.localStorage.getItem(key); let knowledge = merchandise ? JSON.parse(merchandise) : defaultValue; setStoredValue(knowledge) } catch (error) {} }, []) return [storedValue, setValue]; }
useConversation
Hook
We’ll use this Hook to fetch conversations primarily based on the given room id
:
import { useEffect, useState } from "react"; const fetchRoomData = async (room_id) => { if (!room_id) return; const url = `http://localhost:8080/conversations/${room_id}`; attempt { let resp = await fetch(url).then(res => res.json()); return resp; } catch (e) { console.log(e); } } export default perform useConversations(room_id) { const [isLoading, setIsLoading] = useState(true); const [messages, setMessages] = useState([]); const updateMessages = (resp = []) => { setIsLoading(false); setMessages(resp) } const fetchConversations = (id) => { setIsLoading(true) fetchRoomData(id).then(updateMessages) } useEffect(() => fetchConversations(room_id), []); return [isLoading, messages, setMessages, fetchConversations]; }
Constructing the chat utility
Now let’s join all of our parts and Hooks to construct our chat utility in React with Subsequent.js.
First, let’s import all of the dependencies we’d like:
// ui/pages/index.js import Head from 'subsequent/head' import React, { useEffect, useState } from 'react' import Avatar from '../parts/avatar' import ChatList from '../parts/rooms' import Dialog from '../parts/dialog' import Login from '../parts/login' import useConversations from '../libs/useConversation' import useLocalStorage from '../libs/useLocalStorage' import useWebsocket from '../libs/useWebsocket'
Now, let’s arrange the state for our chat pages:
// ui/pages/index.js ... export default perform Dwelling() { const [room, setSelectedRoom] = useState(null); const [isTyping, setIsTyping] = useState(false); const [showLogIn, setShowLogIn] = useState(false); const [auth, setAuthUser] = useLocalStorage("person", false); const [isLoading, messages, setMessages, fetchConversations] = useConversations(""); ... }
The next capabilities will deal with all messages coming in or out of the WebSocket server:
handleTyping
: Updates the state to show the typing indicatorhandleMessage
: Handles incoming and outgoing messages to the stateonMessage
: Handles messages retrieved from the WebSocket serverupdateFocus
: Tells the WebSocket server if the present person continues to be typing a messageonFocusChange
: Lets the WebSocket server know when the present person is completed typingsubmitMessage
: Updates the message state after which sends the message to the server when a person hits the ship button
Right here’s how we’ll use these capabilities in our code:
// ui/pages/index.js const handleTyping = (mode) => { if (mode === "IN") { setIsTyping(true) } else { setIsTyping(false) } } const handleMessage = (msg, userId) => { setMessages(prev => { const merchandise = { content material: msg, user_id: userId }; return [...prev, item]; }) } const onMessage = (knowledge) => { attempt { let messageData = JSON.parse(knowledge); change (messageData.chat_type) { case "TYPING": { handleTyping(messageData.worth[0]); return; } case "TEXT": { handleMessage(messageData.worth[0], messageData.user_id); return; } } } catch (e) { console.log(e); } } const sendMessage = useWebsocket(onMessage) const updateFocus = () => { const knowledge = { id: 0, chat_type: "TYPING", worth: ["IN"], room_id: room.id, user_id: auth.id } sendMessage(JSON.stringify(knowledge)) } const onFocusChange = () => { const knowledge = { id: 0, chat_type: "TYPING", worth: ["OUT"], room_id: room.id, user_id: auth.id } sendMessage(JSON.stringify(knowledge)) } const submitMessage = (e) => { e.preventDefault(); let message = e.goal.message.worth; if (message === "") { return; } if (!room.id) { alert("Please choose chat room!") return } const knowledge = { id: 0, chat_type: "TEXT", worth: [message], room_id: room.id, user_id: auth.id } sendMessage(JSON.stringify(knowledge)) e.goal.message.worth = ""; handleMessage(message, auth.id); onFocusChange(); }
We’ll use the next capabilities to deal with state for updating the message and for the person login and logout:
updateMessages
: Fetches the dialog of the given roomid
when a person switches chat roomssignOut
: Updates the state to signout and removes the person knowledge from native storage
We’ll use these capabilities in our code, like so:
// ui/pages/index.js const updateMessages = (knowledge) => { if (!knowledge.id) return; fetchConversations(knowledge.id) setSelectedRoom(knowledge) } const signOut = () => { window.localStorage.removeItem("person"); setAuthUser(false); } useEffect(() => setShowLogIn(!auth), [auth])
Now, let’s show all the info to the shopper:
return ( <div> <Head> <title>Rust with react chat app</title> <meta title="description" content material="Rust with react chat app" /> <hyperlink rel="icon" href="https://weblog.logrocket.com/favicon.ico" /> </Head> <Login present={showLogIn} setAuth={setAuthUser} /> <div className={`${!auth && 'hidden'} bg-gradient-to-b from-orange-400 to-rose-400 h-screen p-12`}> <major className="flex w-full max-w-[1020px] h-[700px] mx-auto bg-[#FAF9FE] rounded-[25px] backdrop-opacity-30 opacity-95"> <apart className="bg-[#F0EEF5] w-[325px] h-[700px] rounded-l-[25px] p-4 overflow-auto relative"> <ChatList onChatChange={updateMessages} userId={auth.id} /> <button onClick={signOut} className="text-xs w-full max-w-[295px] p-3 rounded-[10px] bg-violet-200 font-semibold text-violet-600 text-center absolute bottom-4">LOG OUT</button> </apart> {room?.id && (<part className="rounded-r-[25px] w-full max-w-[690px] grid grid-rows-[80px_minmax(450px,_1fr)_65px]"> <div className="rounded-tr-[25px] w-ful"> <div className="flex gap-3 p-3 items-center"> <Avatar coloration="rgb(245 158 11)">{room.customers.get_target_user(auth.id)}</Avatar> <div> <p className="font-semibold text-gray-600 text-base">{room.customers.get_target_user(auth.id)}</p> <div className="text-xs text-gray-400">{isTyping ? "Typing..." : "10:15 AM"}</div> </div> </div> <hr className="bg-[#F0EEF5]" /> </div> {(isLoading && room.id) && <p className="px-4 text-slate-500">Loading dialog...</p>} <Dialog knowledge={messages} auth={auth} customers={room.customers} /> <div className="w-full"> <type onSubmit={submitMessage} className="flex gap-2 items-center rounded-full border border-violet-500 bg-violet-200 p-1 m-2"> <enter onBlur={onFocusChange} onFocus={updateFocus} title="message" className="p-2 placeholder-gray-600 text-sm w-full rounded-full bg-violet-200 focus:outline-none" placeholder="Sort your message right here..." /> <button sort="submit" className="bg-violet-500 rounded-full py-2 px-6 font-semibold text-white text-sm">Despatched</button> </type> </div> </part>)} </major> </div> </div> )
Conclusion
On this article, we mentioned the options of WebSockets, its purposes in Rust, and the way to use it with the actix-web
package deal. We demonstrated the way to create an environment friendly, real-time chat utility, utilizing React and Subsequent.js to determine WebSocket connections to the Actix Net server. The code from this text is out there on GitHub.
To additional enhance our pattern real-time chat utility, you can allow it to show person standing (i.e., on-line or offline) and create a web-based group chat for customers.
Please be happy to go away a remark in case you have any questions on this text. Comfortable coding!
LogRocket: Full visibility into your manufacturing React apps
Debugging React purposes may be tough, particularly when customers expertise points which can be onerous to breed. Should you’re interested by monitoring and monitoring Redux state, routinely surfacing JavaScript errors, and monitoring gradual community requests and part load time,
attempt LogRocket.
LogRocket
combines session replay, product analytics, and error monitoring – empowering software program groups to create the perfect internet and cellular product expertise. What does that imply for you?
As an alternative of guessing why errors occur, or asking customers for screenshots and log dumps, LogRocket permits you to replay issues as in the event that they occurred in your personal browser to shortly perceive what went incorrect.
No extra noisy alerting. Good error monitoring permits you to triage and categorize points, then learns from this. Get notified of impactful person points, not false positives. Much less alerts, far more helpful sign.
The LogRocket Redux middleware package deal provides an additional layer of visibility into your person classes. LogRocket logs all actions and state out of your Redux shops.
Extra nice articles from LogRocket:
Modernize the way you debug your React apps —
begin monitoring totally free.