The Rust programming language has gained mainstream adoption up to now a number of years. It’s persistently ranked as essentially the most beloved programming language by builders and has been accepted into the Linux kernel. Rust allows builders to jot down right and memory-safe applications which can be as quick and as small as C applications. It’s ideally suited to infrastructure software program, together with server-side purposes, that require excessive reliability and efficiency.
Nevertheless, for server-side purposes, Rust additionally presents some challenges. Rust applications are compiled into native machine code, which isn’t transportable and is unsafe in multi-tenancy cloud environments. We additionally lack instruments to handle and orchestrate native purposes within the cloud.
Therefore, server-side Rust purposes generally run inside VMs or Linux containers, which carry vital reminiscence and CPU overhead. This diminishes Rust’s benefits in effectivity and makes it laborious to deploy companies in resource-constrained environments, similar to edge knowledge facilities and edge clouds. The answer to this downside is WebAssembly (Wasm).
Began as a safe runtime inside internet browsers, Wasm applications will be securely remoted in their very own sandbox. With a brand new era of Wasm runtimes, such because the Cloud Native Computing Basis’s WasmEdge Runtime, now you can run Wasm purposes on the server. You may compile Rust applications to Wasm bytecode, after which deploy the Wasm software within the cloud.
In line with a research revealed in IEEE Software program, Wasm apps will be 100x sooner (particularly at startup) and 1/100 smaller in comparison with natively compiled Rust apps in Linux containers. This makes them particularly nicely suited to resource-constrained environments similar to edge clouds.
Wasm runtime sandboxes have a lot smaller assault surfaces and supply higher isolation than Linux containers. Moreover, Wasm runtime is transportable throughout working programs and {hardware} platforms. As soon as a Rust program is compiled into Wasm, it may possibly run all over the place from growth to manufacturing and from the cloud to the sting.
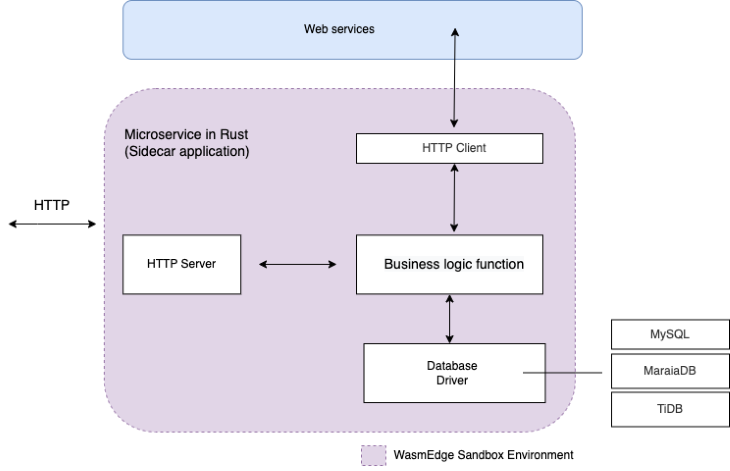
On this article, we’ll cowl the instruments, libraries, APIs, frameworks, and strategies required to construct microservices in Rust. We’ll additionally show the best way to deploy, run, and scale these microservices in WasmEdge WebAssembly Runtime.
Bounce forward:
Stipulations
To observe together with this text, it is best to have the next:
- Fundamental information of the microservice design sample
- Fundamental information of the Linux working system
- Familiarity with the Rust programming language
- Fundamental information of SQL databases
Creating an online service
The microservice is foremost an online server. WasmEdge Runtime helps asynchronous and non-blocking community sockets. You may write networking purposes in Rust, compile them into Wasm, and run them within the WasmEdge Runtime. Within the Rust ecosystem, WasmEdge helps the next:
- The tokio and mio crates for asynchronous networking purposes
- The hyper crate for HTTP server and shopper purposes
The instance under, from the microservice-rust-mysql demo app, reveals the best way to create an online server in hyper for WasmEdge. The principle listening loop of the online server is as follows:
let addr = SocketAddr::from(([0, 0, 0, 0], 8080)); let make_svc = make_service_fn(|_| { let pool = pool.clone(); async transfer { Okay::<_, Infallible>(service_fn(transfer |req| { let pool = pool.clone(); handle_request(req, pool) })) } }); let server = Server::bind(&addr).serve(make_svc); if let Err(e) = server.await { eprintln!("server error: {}", e); } Okay(())
As soon as a request is available in, the occasion handler, handle_request(),
is named asynchronously in order that it may possibly deal with a number of concurrent requests. It generates a response primarily based on the request technique and path:
async fn handle_request(req: Request<Physique>, pool: Pool) -> Consequence<Response<Physique>, anyhow::Error> { match (req.technique(), req.uri().path()) { (&Technique::GET, "https://weblog.logrocket.com/") => Okay(Response::new(Physique::from( "... ...", ))), // Merely echo the physique again to the shopper. (&Technique::POST, "/echo") => Okay(Response::new(req.into_body())), (&Technique::GET, "/init") => { let mut conn = pool.get_conn().await.unwrap(); "DROP TABLE IF EXISTS orders;".ignore(&mut conn).await?; "CREATE TABLE orders (order_id INT, product_id INT, amount INT, quantity FLOAT, transport FLOAT, tax FLOAT, shipping_address VARCHAR(20));".ignore(&mut conn).await?; drop(conn); Okay(Response::new(Physique::from("{"standing":true}"))) } (&Technique::POST, "/create_order") => { // ... ... } // Return the 404 Not Discovered for different routes. _ => { let mut not_found = Response::default(); *not_found.status_mut() = StatusCode::NOT_FOUND; Okay(not_found) } } }
Now we’ve an HTTP server for the online service.
Creating an online service shopper
A typical internet service additionally must eat different internet companies. Via tokio and/or mio crates, WasmEdge purposes can simply incorporate HTTP purchasers for internet companies. The next Rust crates are supported in WasmEdge:
The next instance reveals the best way to make an HTTP POST towards an online service API from a microservice:
let shopper = reqwest::Consumer::new(); let res = shopper .put up("http://eu.httpbin.org/put up") .physique("msg=WasmEdge") .ship() .await?; let physique = res.textual content().await?; println!("POST: {}", physique);
Making a database shopper
Most microservices are backed by databases. The next Rust crates for MySQL drivers are supported in WasmEdge:
- The myql crate is a synchronous MySQL shopper
- The mysql_async is an asynchronous MySQL shopper
The instance under reveals the best way to insert a set of data right into a database desk:
let orders = vec![ Order::new(1, 12, 2, 56.0, 15.0, 2.0, String::from("Mataderos 2312")), Order::new(2, 15, 3, 256.0, 30.0, 16.0, String::from("1234 NW Bobcat")), Order::new(3, 11, 5, 536.0, 50.0, 24.0, String::from("20 Havelock")), Order::new(4, 8, 8, 126.0, 20.0, 12.0, String::from("224 Pandan Loop")), Order::new(5, 24, 1, 46.0, 10.0, 2.0, String::from("No.10 Jalan Besar")), ]; r"INSERT INTO orders (order_id, production_id, amount, quantity, transport, tax, shipping_address) VALUES (:order_id, :production_id, :amount, :quantity, :transport, :tax, :shipping_address)" .with(orders.iter().map(|order| { params! { "order_id" => order.order_id, "production_id" => order.production_id, "amount" => order.amount, "quantity" => order.quantity, "transport" => order.transport, "tax" => order.tax, "shipping_address" => &order.shipping_address, } })) .batch(&mut conn) .await?;
The under instance reveals the best way to question a database desk and return a group of data:
let loaded_orders = "SELECT * FROM orders" .with(()) .map( &mut conn, |(order_id, production_id, amount, quantity, transport, tax, shipping_address)| { Order::new( order_id, production_id, amount, quantity, transport, tax, shipping_address, ) }, ) .await?; dbg!(loaded_orders.len()); dbg!(loaded_orders);
Constructing, deploying, and operating the microservice
The microservice-rust-mysql mission offers a whole instance of a database-driven microservice. Let’s use it for example to construct, deploy, and run this service.
The Docker CLI and Docker Desktop present seamless assist for WasmEdge software growth. From the basis listing of the mission repo, you simply want a single command to construct and produce up all of the parts of the microservice (i.e., the WasmEdge software and a mariaDB database server):
docker compose up
You may then take a look at the CRUD operations on the database by means of the microservice utilizing curl.
Alternatively, you may:
The instructions under set up the above conditions on a Linux system:
// Set up Rust curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh // Set up WasmEdge curl -sSf https://uncooked.githubusercontent.com/WasmEdge/WasmEdge/grasp/utils/set up.sh | bash -s -- -e all // Set up MySQL. It's accessible as a bundle in most Linux distros sudo apt-get replace sudo apt-get -y set up mysql-server libmysqlclient-dev sudo service mysql begin
Subsequent, construct the microservice software into Wasm bytecode:
cargo construct --target wasm32-wasi --release
Then, begin the microservice in WasmEdge Runtime:
wasmedge --env "DATABASE_URL=mysql://person:[email protected]:3306/mysql" order_demo_service.wasm
You may then use the microservice’s internet API to entry the database:
// Init the database desk curl http://localhost:8080/init // Insert a set of data curl http://localhost:8080/create_orders -X POST -d @orders.json // Question the data from the database curl http://localhost:8080/orders // Replace the data curl http://localhost:8080/update_order -X POST -d @update_order.json // Delete a document by its id curl http://localhost:8080/delete_order?id=2
Going to manufacturing
To this point, we’ve seen a whole database-driven microservice in motion. In the true world, nevertheless, an organization might have tons of of microservices. They have to be managed and orchestrated by cloud-native frameworks similar to Kubernetes.
WasmEdge purposes are totally OCI-compliant. They are often managed and saved within the Docker Hub or different Open Container Initiative repositories. Via crun integration, WasmEdge can run side-by-side with Linux container purposes in the identical Kubernetes cluster. This repository showcases the best way to run WasmEdge purposes in well-liked container toolchains together with CRI-O, containerd, Kubernetes, Form, OpenYurt, KubeEdge, and extra.
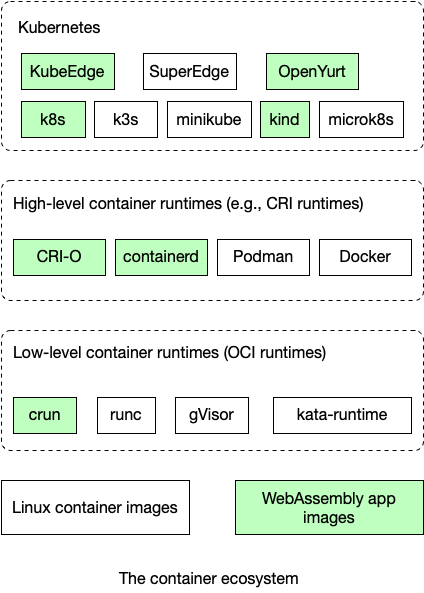
Moreover, microservices are sometimes deployed along with service frameworks. For instance, Dapr is a well-liked runtime framework for microservices. It offers a “sidecar” service for every microservice. The microservice accesses the sidecar by way of the Dapr API to find and invoke different companies on the community, handle state knowledge, and entry message queues.
The Dapr SDK for WASI (WebAssembly System Interface) allows WasmEdge-based microservices to entry their hooked up Dapr sidecars. An entire demo software with a Jamstack static internet frontend, three microservices, and a database service can also be accessible.
Conclusion
On this article, we mentioned why WebAssembly is a superb runtime sandbox format for Rust-based server-side purposes. We walked by means of concrete code examples demonstrating the best way to create an HTTP internet service, eat different internet companies, entry relational databases from Rust, after which run the compiled purposes in WasmEdge. We additionally investigated deployment considerations, similar to Kubernetes and Dapr integrations.
With these crates and template apps, it is possible for you to to construct your individual light-weight microservices in Rust!
Apart from microservices, the WasmEdge Runtime will be broadly used as an software sandbox in many alternative situations:
- The flows.community is a serverless platform for SaaS automation. You may create bots, customizations, and connectors for SaaS merchandise utilizing Rust and WebAssembly
- You may create Person Outlined Capabilities (UDFs) and Extract-Remodel-Load (ETL) capabilities for databases in WebAssembly, and have these capabilities embedded or colocated with the database in a “serverless” trend
- You may create transportable and high-performance purposes for edge gadgets, operating Android, Open Concord, and even the seL4 RTOS, utilizing WebAssembly
- WebAssembly is broadly used as a runtime for blockchains to execute sensible contracts. WasmEdge, as an illustration, runs nodes and sensible contracts for Polkadot, FileCoin, and XRP (Ripple) networks. The following-generation Ethereum blockchain VM, often known as the ewasm, can also be primarily based on WebAssembly
To study extra about WasmEdge, see the official docs.
LogRocket: Full visibility into manufacturing Rust apps
Debugging Rust purposes will be troublesome, particularly when customers expertise points which can be troublesome to breed. For those who’re concerned with monitoring and monitoring efficiency of your Rust apps, routinely surfacing errors, and monitoring sluggish community requests and cargo time, attempt LogRocket.
LogRocket is sort of a DVR for internet and cell apps, recording actually every part that occurs in your Rust app. As an alternative of guessing why issues occur, you may combination and report on what state your software was in when a problem occurred. LogRocket additionally displays your app’s efficiency, reporting metrics like shopper CPU load, shopper reminiscence utilization, and extra.
Modernize the way you debug your Rust apps — begin monitoring totally free.