In Unity, the best way a sport is offered to the participant is every part. Each the smoothness of the controls and the gameplay they’re experiencing are key, and realizing how this data is proven to the participant provides you with a bonus in the best way you strategy your Unity tasks.
For that purpose, we’re going to be discussing replace features, studying their implementations, and deciding when to make use of every.
Right here’s the desk of contents for those who’d like to leap forward:
What’s a body in Unity?
You may think about a body as an image, and in case you have a number of comparable footage exhibiting quickly, you possibly can create the phantasm of motion.
A body is a time period inherited from animation, the place some widespread values are 24, 30, and 60 frames per second (FPS). On the subject of video games, we are saying that “it’s working at 60 FPS” when there are 60 new photographs in a second offered to the participant. That is referred to as body charge, and body interval is the time that occurs between every body.
In our instance of 60 FPS, 60 could be the body charge, and body interval could be 1/60 x 1000 = 16.67 milliseconds.
In Unity, a body is taken into account a rendered picture offered to the participant’s display screen. Usually talking, Unity doesn’t care about time, nevertheless it does about frames with its property Time.DeltaTime
that calculates time between frames.
If for some purpose, like having heavy processing throughout gameplay, time between frames slows down, this drops the body charge and provides the feeling to the participant that the sport is working slowly.
Explaining the replace features
Through the lifetime of a script, Unity executes its features in a selected order. You may learn extra in regards to the execution order right here, however to maintain this text easy, we will likely be specializing in two phases: physics and sport logic.
Throughout every body, Unity executes so as all of the occasion features of the energetic scripts.
It’ll first execute the physics simulation logic, calling FixedUpdate
first, then the remainder of the physics occasions.
Then it’ll examine for Enter occasions from the person.
Lastly, in the course of the sport logic, it’ll execute the Replace
operate, all the sport logic features, and at last LateUpdate
.
It’s necessary to notice that every occasion is executed in the identical order throughout all of the scripts on Unity, so in case you have ten scripts that use FixedUpdate
, these can have greater execution precedence than different ten scripts which have code of their Replace
methodology; identical factor with LateUpdate
with the decrease precedence within the order for this instance.
Replace features order
Right here we are able to see the order of those features: we might create an empty object in a brand new Unity scene and fix this script to it.
On this script, we are going to print out to the console the order of the features that will get executed. We have now arrange three flags referred to as continueUpdate
, continueFixedUpdate
, and continueLateUpdate
to stop our features from executing the identical code a number of instances and filling the console with pointless outcomes:
utilizing System.Collections; utilizing System.Collections.Generic; utilizing UnityEngine; public class UpdateOrder : MonoBehaviour { bool continueUpdate=true; bool continueFixedUpdate=true; bool continueLateUpdate=true; // Begin is known as earlier than the primary body replace void Begin() { Debug.Log("Begin"); } // Awake is known as when the item is enabled void Awake() { Debug.Log("Awake"); } //FixedUpdate is known as earlier than Replace, on the identical charge based mostly on Delta Time void FixedUpdate() { if(continueFixedUpdate) { Debug.Log("Fastened Replace"); continueFixedUpdate=false; } } // Replace is known as as soon as per body void Replace() { if(continueUpdate) { Debug.Log("Replace"); continueUpdate=false; } } //Late Replace is known as after replace void LateUpdate() { if(continueLateUpdate) { Debug.Log("Late Replace"); continueLateUpdate=false; } } }
The output will likely be one thing like this:
Awake
will get referred to as first for the reason that GameObject was energetic, then Begin
, then FixedUpdate
, Replace
, and at last LateUpdate
.
FixedUpdate
FixedUpdate is often used for physics calculations because it has the identical frequency because the physics system: by default it executes each 0.02 seconds (50 instances per second), however you possibly can double-check it with Time.fixedDeltaTime
.
It’s also possible to change the frequency of FixedUpdate
in Unity by going to Edit > Mission Settings > Time > Fastened Timestep.
Replace
Replace
is a operate that will get referred to as each body if the MonoBehaviour
is enabled.
If the body charge is 60 FPS, it’ll execute 60 instances a second; if it’s 30, it will likely be 30 instances a second.
If the script will get disabled (i.e., enabled=false
), it’ll cease.
Extra nice articles from LogRocket:
LateUpdate
Like Replace
, LateUpdate
executes each body if the MonoBehaviour
is enabled, and it’ll cease if it’s disabled.
It’ll execute after all of the replace features are referred to as, and it’s beneficial to make use of LateUpdate
on digicam scripts as a substitute of Replace
since it might probably hold monitor of objects which have already been moved in an Replace
operate.
Please take into account that the order of execution between Replace
and LateUpdate
has nothing to do with velocity. Replace
just isn’t quicker than LateUpdate
; they’re executed in numerous order to do totally different stuff. Whereas Replace
is perhaps used to maneuver objects in a time interval, we might use LateUpdate
to replicate the outcomes after these objects have been moved, and it’s protected to imagine that they’re in a brand new state.
The place to make use of every replace operate
FixedUpdate
It’s beneficial to make use of FixedUpdate
when coping with physics, like Rigidbodies updates.
Within the following instance, we added three cubes with a Rigidbody
every.
What the script does in every case is that it takes a reference of the Rigidbody
of every dice and applies a power upwards within the object they’re connected to. The one distinction between them is the kind of replace operate they use.
The dice on the left has this script; it will likely be utilizing FixedUpdate
:
utilizing System.Collections; utilizing System.Collections.Generic; utilizing UnityEngine; public class FixedUpdateRigidBody : MonoBehaviour { public Rigidbody rb; // Begin is known as earlier than the primary body replace void Begin() { rb = GetComponent<Rigidbody>(); } //Is executed based mostly on the Fastened Timestep (by default 50 instances per second) void FixedUpdate() { rb.AddForce(10.0f * Vector3.up); } }
The dice within the heart will likely be utilizing this one, utilizing Replace
:
utilizing System.Collections; utilizing System.Collections.Generic; utilizing UnityEngine; public class UpdateRigidBody : MonoBehaviour { public Rigidbody rb; // Begin is known as earlier than the primary body replace void Begin() { rb = GetComponent<Rigidbody>(); } //Is executed each body void Replace() { rb.AddForce(10.0f * Vector3.up); } }
And the dice on the suitable will likely be utilizing this script, calling LateUpdate
:
utilizing System.Collections; utilizing System.Collections.Generic; utilizing UnityEngine; public class LateUpdateRigidBody : MonoBehaviour { public Rigidbody rb; // Begin is known as earlier than the primary body replace void Begin() { rb = GetComponent<Rigidbody>(); } //Is executed each body after Replace void LateUpdate() { rb.AddForce(10.0f * Vector3.up); } }
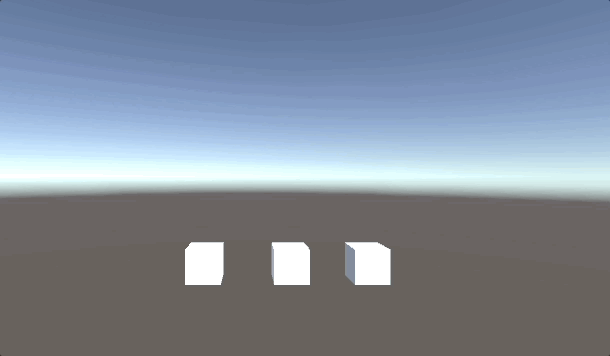
FixedUpdate
, Replace
, LateUpdate
You may see a transparent illustration on how every replace operate works. FixedUpdate
will likely be executed as soon as each time step is settled in Unity; that’s the reason the motion is fixed and regular.
Alternatively, the dice within the heart is utilizing the Replace
operate each body, so every time the operate is known as to use power upward, and since every body is extra frequent than the time step of FixedUpdate
, you possibly can see that it goes up manner quicker. It’s because it’s being referred to as many instances greater than the dice on the left.
And within the case of the third dice that’s utilizing the LateUpdate
operate, it’s being executed every body too, however after the Replace
operate due the order of execution mentioned above.
Please notice that to the human eye, the distinction between executing code within the Replace
operate and LateUpdate
operate is just about the identical. You couldn’t inform which dice is utilizing the Replace
or LateUpdate
operate.
Replace
Replace
is known as each body, so if it is advisable learn the enter of the participant, that is the proper operate to deal with it so that you don’t miss any occasion.
LateUpdate
LateUpdate
executes after all of the Updates
features have been referred to as. That is related as a result of the order of execution on Replace
is perhaps just a little chaotic, and you’ll’t be certain which script will likely be executed inside all of your scripts that execute Replace
.
Nevertheless, with LateUpdate
, you possibly can make sure that you’ll name this operate after all of the states modified with Replace
happen, so you possibly can work with the ultimate outcomes of your objects.
It’s beneficial to make use of LateUpade
when following objects with the digicam and updating the UI for the gamers.
Last ideas
There are two fundamental ideas you would take from this text. For one, there’s an order of execution that goes: Replace
is executed every body; FixedUpdate
is executed at a selected charge outlined within the editor; and LateUpdate
is executed after all of the Replace
features have been referred to as.
And the second is that this order just isn’t based mostly on every script; it’s based mostly in your entire challenge, which means that each one the Replace
occasions will execute on the identical time, and after these are completed, LateUpdate
will execute. Due to this, one FixedUpdate
operate might be referred to as on the identical time the opposite two are referred to as, so attempt your finest to order your features and concepts earlier than executing every part on the identical time.
You ought to be conscious that the states of your objects and positions might be modified throughout these occasions and plan forward for the very best strategy to have probably the most secure expertise on your gamers.
I hope this text has been helpful to you. Glad gamedev!
LogRocket: Full visibility into your net and cell apps
LogRocket is a frontend software monitoring resolution that allows you to replay issues as in the event that they occurred in your individual browser. As an alternative of guessing why errors occur, or asking customers for screenshots and log dumps, LogRocket permits you to replay the session to shortly perceive what went flawed. It really works completely with any app, no matter framework, and has plugins to log extra context from Redux, Vuex, and @ngrx/retailer.
Along with logging Redux actions and state, LogRocket information console logs, JavaScript errors, stacktraces, community requests/responses with headers + our bodies, browser metadata, and customized logs. It additionally devices the DOM to file the HTML and CSS on the web page, recreating pixel-perfect movies of even probably the most advanced single-page and cell apps.