At Bell Labs, Dennis Ritchie developed the C programming language between 1971 and 1973. C is a mid-level structured-oriented programming and general-purpose programming. It is without doubt one of the outdated and hottest programming languages. There are various purposes wherein C programming language is used, together with language compilers, working programs, assemblers, community drivers, textual content editors, print spoolers, trendy purposes, language interpreters, databases, and utilities.
On this article, you’re going to get the continuously and most requested C programming interview questions and solutions on the more energizing and skilled ranges.
C Programming Interview Questions – For Freshers
1. Why is C referred to as a mid-level programming language?
On account of its capacity to assist each low-level and high-level options, C is taken into account a middle-level language. It’s each an assembly-level language, i.e. a low-level language, and a higher-level language. Applications which are written in C are transformed into meeting code, they usually assist pointer arithmetic (low-level) whereas being machine-independent (high-level). Subsequently, C is sometimes called a middle-level language. C can be utilized to put in writing working programs and menu-driven client billing programs.
2. What are the options of the C programming language?
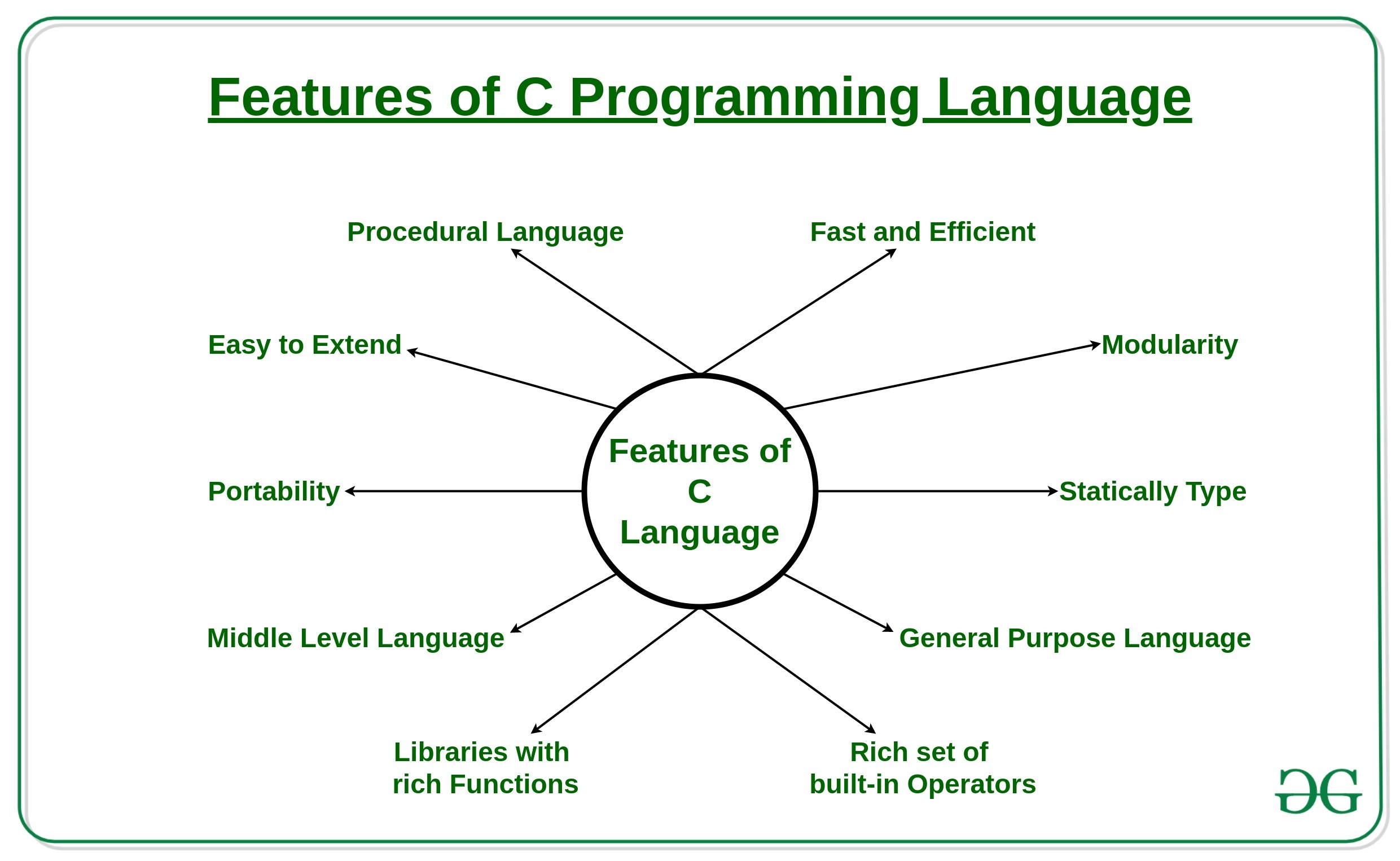
For extra data, seek advice from the article – Options of C programming language.
3. What are primary knowledge sorts supported within the C Programming Language?
Every variable in C has an related knowledge sort. Every knowledge sort requires totally different quantities of reminiscence and has some particular operations which might be carried out over it. It specifies the kind of knowledge that the variable can retailer like integer, character, floating, double, and so forth. In C knowledge sorts are broadly categorized into 4 classes:
- Primitive knowledge sorts: Primitive knowledge sorts might be additional categorized into an integer, and floating knowledge sorts.
- Void Varieties: Void knowledge sorts come underneath primitive knowledge sorts. Void knowledge sorts present no outcome to their caller and don’t have any worth related to them.
- Consumer Outlined knowledge sorts: These knowledge sorts are outlined by the consumer to make this system extra readable.
- Derived knowledge sorts: Information sorts which are derived from primitive or built-in knowledge sorts.
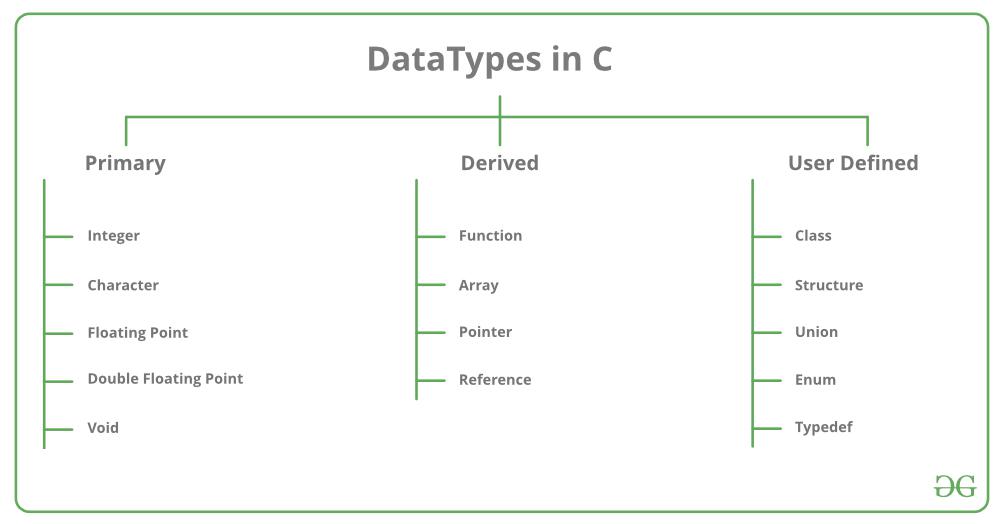
For extra data, seek advice from the article – Information Varieties in C.
4. What are tokens in C?
Tokens are identifiers or the smallest single unit in a program that’s significant to the compiler. In C we’ve the next tokens:
- Key phrases: Predefined or reserved phrases within the C programming language. Each key phrase is supposed to carry out a particular activity in a program. C Programming language helps 32 key phrases.
- Identifiers: Identifiers are user-defined names that include an arbitrarily lengthy sequence of digits or letters with both a letter or the underscore (_) as a primary Character. Identifier names can’t be equal to any reserved key phrases within the C programming language. There are a algorithm which a programmer should comply with as a way to identify an identifier in C.
- Constants: Constants are regular variables that can’t be modified in this system as soon as they’re outlined. Constants seek advice from a hard and fast worth. They’re additionally known as literals.
- Strings: Strings in C are an array of characters that finish with a null character (‘ ). Null character signifies the top of the string;
- Particular Symbols: Some particular symbols in C have some particular which means and thus, they can’t be used for every other function in this system. # = {} () , * ; [] are the particular symbols in C programming language.
- Operators: Symbols that set off an motion when they’re utilized to any variable or every other object. Unary, Binary, and ternary operators are used within the C Programming language.
For extra data, seek advice from the article – Tokens in C
5. What do you imply by the scope of the variable?
Scope in a programming language is the block or a area the place an outlined variable could have its existence and past that area, the variable is robotically destroyed. Each variable has its outlined scope. In easy phrases, the scope of a variable is the same as its life in this system. The variable might be declared in three locations These are:
- Native Variables: Inside a given perform or a block
- World Variables: Out of all features globally inside this system.
- Formal Parameters: In perform parameters solely.
For extra data, seek advice from the article – Scope in C
6. What are preprocessor directives in C?
In C preprocessor directives are thought of the built-in predefined features or macro that act as a directive to the compiler and are executed earlier than this system execution. There are a number of steps concerned in writing and executing a program in C. Most important sorts of Preprocessor Directives are Macros, File Inclusion, Conditional Compilation, and Different directives like #undef, #pragma, and so forth.
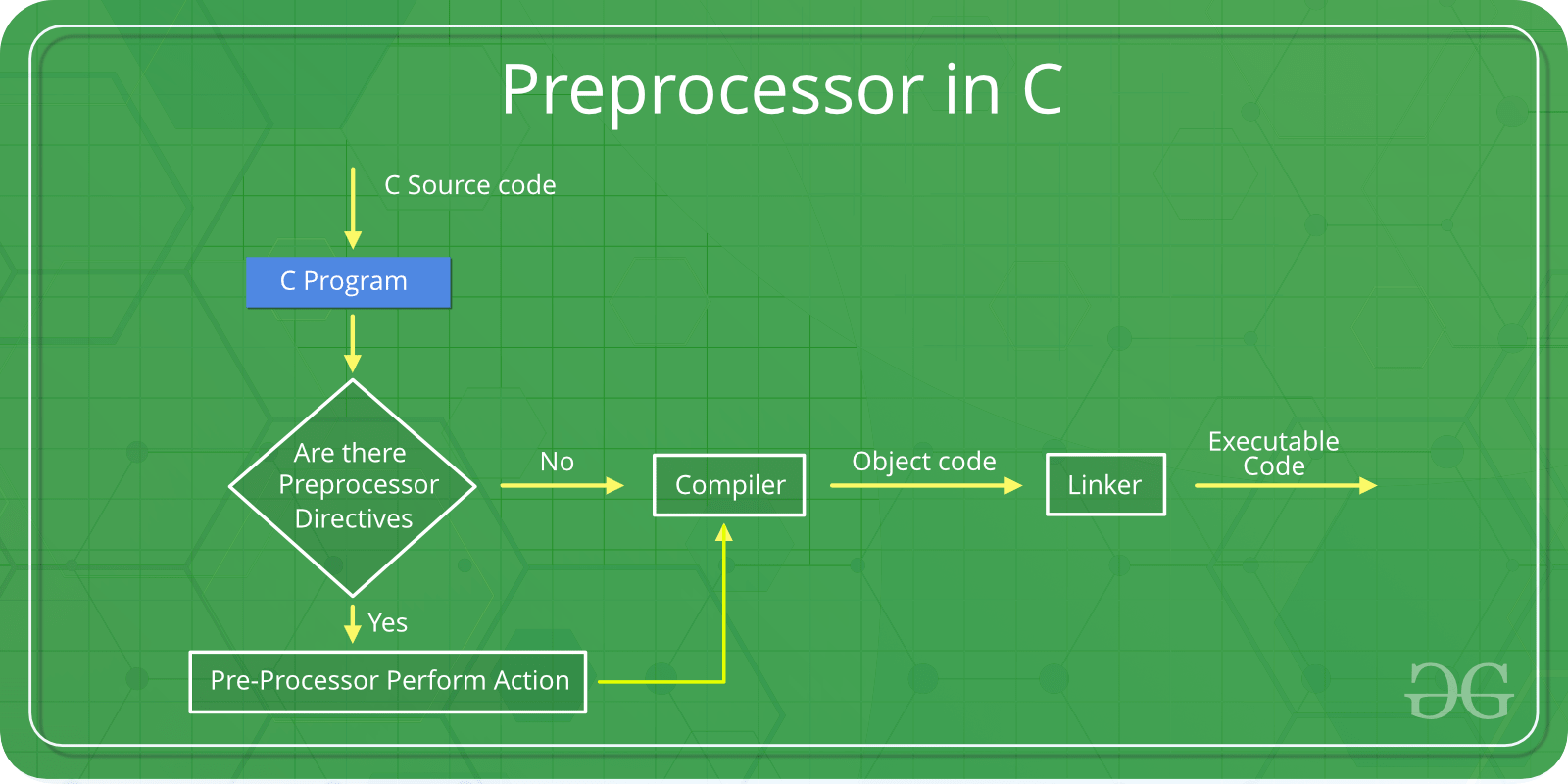
For extra data, seek advice from the article – Preprocessor Directives in C
7. What’s using static variables in C?
Static variables within the C programming language are used to protect the info values between perform calls even after they’re out of their scope. Static variables protect their values of their scope they usually can be utilized once more in this system with out initializing once more. Static variables have an preliminary worth assigned to 0 with out initialization.
C
|
Preliminary worth of static variable 0 Preliminary worth of variable with out static 0
For extra data, seek advice from the article – Static Variables in C
8. What’s the distinction between malloc() and calloc() within the C programming language?
calloc() and malloc() library features are used to allocate dynamic reminiscence. Dynamic reminiscence is the reminiscence that’s allotted in the course of the runtime of this system from the heap section. “stdlib.h” is the header file that’s used to facilitate dynamic reminiscence allocation within the C Programming language.
Parameter | Malloc() | Calloc() |
---|---|---|
Definition | It’s a perform that creates one block of reminiscence of a hard and fast measurement. | It’s a perform that assigns a couple of block of reminiscence to a single variable. |
Variety of arguments | It solely takes one argument. | It takes two arguments. |
Velocity | malloc() perform is quicker than calloc(). | calloc() is slower than malloc(). |
Effectivity | It has excessive time effectivity. | It has low time effectivity. |
Utilization | It’s used to point reminiscence allocation. | It’s used to point contiguous reminiscence allocation. |
For extra data, seek advice from the article – Dynamic Reminiscence Allocation in C utilizing malloc(), calloc(), free() and realloc()
9. What do you imply by dangling pointers and the way are dangling pointers totally different from reminiscence leaks in C programming?
Pointers pointing to deallocated reminiscence blocks in C Programming are often known as dangling pointers i.e, every time a pointer is pointing to a reminiscence location and In case the variable is deleted and the pointer nonetheless factors to that very same reminiscence location then it is named a dangling pointer variable.
In C programming reminiscence leak happens after we allocate reminiscence with the assistance of the malloc() or calloc() library perform, however we overlook to free the allotted reminiscence with the assistance of the free() library perform. Reminiscence leak causes this system to make use of an undefined quantity of reminiscence from the RAM which makes it unavailable for different operating packages this causes our program to crash.
10. Write a program to transform a quantity to a string with the assistance of sprintf() perform within the C library.
C
|
The string for the num is 32.230000
For extra data, seek advice from the article – sprintf() in C
11. What’s recursion in C?
Recursion is the method of creating the perform name itself instantly or not directly. A recursive perform solves a specific drawback by calling a duplicate of itself and fixing smaller subproblems that sum up the unique issues. Recursion helps to cut back the size of code and make it extra comprehensible. The recursive perform makes use of a LIFO ( Final In First Out ) construction like a stack. Each recursive name in this system requires additional house within the stack reminiscence.
For extra data, seek advice from the article – Recursion
12. What’s the distinction between the native and world variables in C?
Native variables are declared inside a block or perform however world variables are declared outdoors the block or perform to be accessed globally.
Native Variables |
World Variables |
---|---|
Declared inside a block or a perform. | Variables which are declared outdoors the block or a perform. |
By default, variables retailer a rubbish worth. | By default worth of the worldwide worth is zero. |
The lifetime of the native variables is destroyed after the block or a perform. | The lifetime of the worldwide variable exists till this system is executed. |
Variables are saved contained in the stack until they’re specified by the programmer. | The storage location of the worldwide variable is set by the compiler. |
To entry the native variables in different features parameter passing is required. | No parameter passing is required. They’re globally seen all through this system. |
13. What are pointers and their makes use of?
Pointers are used to retailer the tackle of the variable or a reminiscence location. Pointer will also be used to refer to a different pointer perform. The principle function of the pointer is to avoid wasting reminiscence house and enhance execution time. Makes use of of pointers are:
- To cross arguments by reference
- For accessing array components
- To return a number of values
- Dynamic reminiscence allocation
- To implement knowledge buildings
- To do system-level programming the place reminiscence addresses are helpful
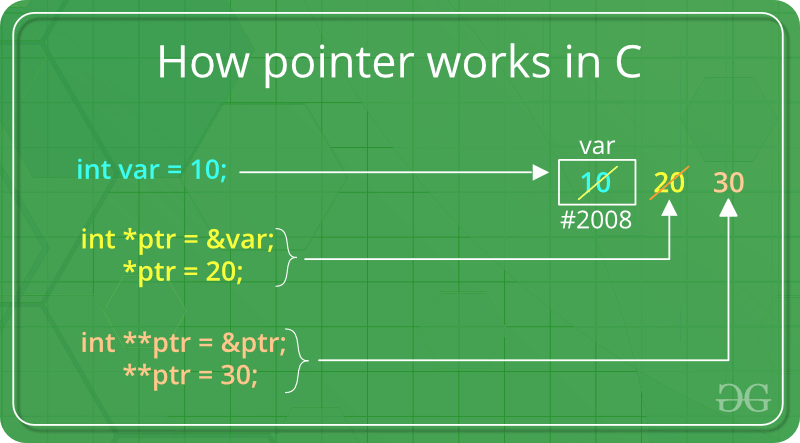
For extra data, seek advice from the article – Pointer Makes use of in C.
14. What’s typedef in C?
In C programming, typedef is a key phrase that defines an alias for an current sort. Whether or not it’s an integer variable, perform parameter, or construction declaration, typedef will shorten the identify.
Syntax:
typedef <existing-type> <alias-name>
Right here,
- current sort is already given a reputation.
- alias identify is the brand new identify for the present variable.
Instance:
typedef lengthy lengthy ll
15. What are loops and the way can we create an infinite loop in C?
Loops are used to execute a block of statements repeatedly. The assertion which is to be repeated might be executed n occasions contained in the loop till the given situation is reached. There are two sorts of loops Entry managed and Exit managed loops in C programming language. An Infinite loop is a bit of code that lacks a practical exit. So, it repeats indefinitely. There might be solely two issues when there may be an infinite loop in this system. One it was designed to loop endlessly till the situation is met throughout the loop. One other might be improper or unhappy break situations in this system.
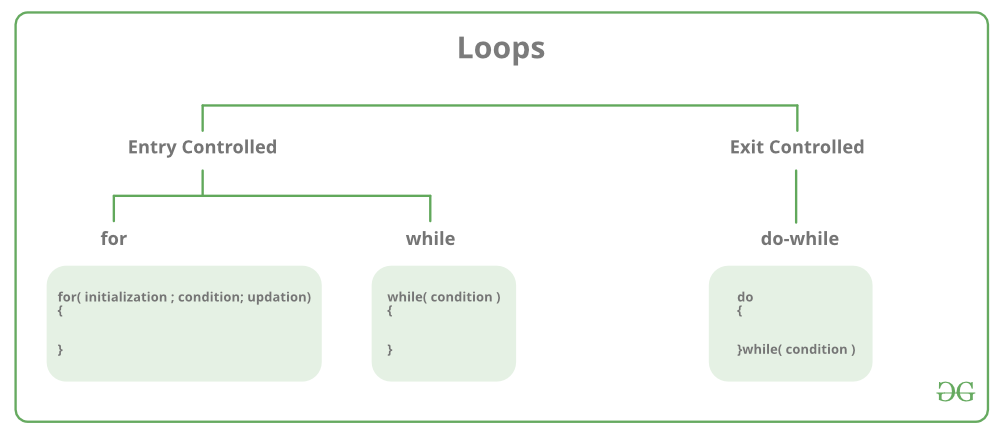
Under is this system for infinite loop in C:
C
|
For extra data, seek advice from the article – Loops in C.
16. What’s the distinction between sort casting and kind conversion?
Sort Casting |
Sort Conversion |
---|---|
The info sort is transformed to a different knowledge sort by a programmer with the assistance of a casting operator. | The info sort is transformed to a different knowledge by a compiler. |
It may be utilized to each appropriate knowledge sorts in addition to incompatible knowledge sorts. | Sort conversion can solely be utilized to solely appropriate knowledge sorts. |
In Sort casting as a way to forged the info sort into one other knowledge sort, a caste operator is required | In sort conversion, there isn’t any want for a casting operator. |
Sort casting is extra environment friendly and dependable. | Sort conversion is much less environment friendly and fewer dependable than sort casting. |
Sort casting takes place throughout this system design by the programmer. | Sort conversion is completed at compile time. |
Syntax: destination_data_type = (target_data_type) variable_to_be_converted; |
Syntax: int a = 20; float b; b = a; // a = 20.0000 |
For extra data, seek advice from the article – Sort Casting and Sort Conversion.
17. What are header recordsdata and their makes use of?
C language has quite a few libraries which include predefined features to make programming simpler. Header recordsdata include predefined customary library features. All header recordsdata should have a ‘.h’ extension. Header recordsdata include perform definitions, knowledge sort definitions, and macros which might be imported with the assistance of the preprocessor directive ‘#embrace’. Preprocessor directives instruct the compiler that these recordsdata are wanted to be processed earlier than the compilation.
There are two sorts of header recordsdata i.e, Consumer-defined header recordsdata and Pre-existing header recordsdata. For instance, if our code must take enter from the consumer and print desired output to the display then ‘stdio.h’ header file have to be included in this system as #embrace<stdio.h>. This header file accommodates features like scanf() and printf() that are used to take enter from the consumer and print the content material.
For extra data, seek advice from the article – Header Recordsdata in C
18. What are the features and their sorts?
The perform is a block of code that’s used to carry out a activity a number of occasions slightly than writing it out a number of occasions in our program. Features keep away from repetition of code and enhance the readability of this system. Modifying a program turns into simpler with the assistance of perform and therefore reduces the possibilities of error. There are two sorts of features:
- Consumer-defined Features: Features which are outlined by the consumer to cut back the complexity of huge packages. They’re constructed solely to fulfill the situation wherein the consumer is going through points and are generally often known as “tailored features”.
- Constructed-in Features: Library features are offered by the compiler bundle and include particular features with particular and totally different meanings. These features give programmers an edge as we are able to instantly use them with out defining them.
For extra data, seek advice from the article – Features in C
19. What’s the distinction between macro and features?
A macro is a reputation that’s given to a block of C statements as a pre-processor directive. Macro is outlined with the pre-processor directive. Macros are pre-processed which signifies that all of the macros can be preprocessed earlier than the compilation of our program. Nevertheless, features are usually not preprocessed however compiled.
Macro | Operate |
---|---|
Macros are preprocessed. | Features are compiled. |
Code size is elevated utilizing macro. | Code size stays unaffected utilizing perform. |
Execution pace utilizing a macro is quicker. | Execution pace utilizing perform is slower. |
The macro identify is changed by the macro worth earlier than compilation. | Switch of management takes place in the course of the perform name. |
Macro doesn’t test any Compile-Time Errors. | Operate test Compile-time errors. |
For extra data, seek advice from the article – Macro vs Features
C Programming Interview Questions – Intermediate Stage
20. The way to convert a string to numbers in C?
In C we’ve 2 primary strategies to transform strings to numbers i.e, Utilizing string stream, Utilizing stoi() library Operate, and utilizing atoi() library perform.
- sscanf(): It reads enter from a string slightly than customary enter.
- atoi(): This perform takes a string literal or a personality array as an argument and an integer worth is returned.
For extra data, seek advice from the article – String to Numbers in C
21. What are reserved key phrases?
Each key phrase is supposed to carry out a particular activity in a program. Their which means is already outlined and can’t be used for functions apart from what they’re initially meant for. C Programming language helps 32 key phrases. Some examples of reserved key phrases are auto, else, if, lengthy, int, swap, typedef, and so forth.
For extra data, seek advice from the article – Variables and Key phrases in C
22. What’s a construction?
The construction is a key phrase that’s used to create user-defined knowledge sorts. The construction permits storing a number of sorts of knowledge in a single unit. The construction members can solely be accessed by the construction variable.
Instance:
struct scholar
{
char identify[20];
int roll_no;
char tackle[20];
char department[20];
};
Under is the C program to implement construction:
C
|
Identify: Kamlesh_Joshi Roll_No: 27 Deal with: Haldwani Department: Laptop Science And Engineering
For extra data, seek advice from the article – Construction in C
23. What’s union?
A union is a user-defined knowledge sort that permits customers to retailer a number of sorts of knowledge in a single unit. Nevertheless, a union doesn’t occupy the sum of the reminiscence of all members. It holds the reminiscence of the most important member solely. For the reason that union allocates one frequent house for all of the members we are able to entry solely a single variable at a time. The union might be helpful in lots of conditions the place we need to use the identical reminiscence for 2 or extra members.
Syntax:
union name_of_union
{
data_type identify;
data_type identify;
};
For extra data, seek advice from the article – Union in C
24. What’s an r-value and worth?
An “l-value” refers to an object with an identifiable location in reminiscence (i.e. having an tackle). An “l-value” will seem both on the proper or left aspect of the project operator(=). An “r-value” is an information worth saved in reminiscence at a given tackle. An “r-value” refers to an object with out an identifiable location in reminiscence (i.e. with out an tackle). An “r-value” is an expression that can’t be assigned a worth, due to this fact it could possibly solely exist on the proper aspect of an project operator (=).
Instance:
int val = 20;
Right here, val is the ‘l-value’, and 20 is the ‘r-value’.
For extra data, seek advice from the article – r-value and l-value in C
25. What’s the distinction between name by worth and name by reference?
Name by worth |
Name by Reference |
---|---|
Values of the variable are handed whereas perform calls. | The tackle of a variable(location of variable) is handed whereas the perform name. |
Dummy variables copy the worth of every variable within the perform name. | Dummy variables copy the tackle of precise variables. |
Adjustments made to dummy variables within the referred to as perform don’t have any impact on precise variables within the calling perform. | We are able to manipulate the precise variables utilizing addresses. |
A easy approach is used to cross the values of variables. | The tackle values of variables have to be saved in pointer variables. |
For extra data, seek advice from the article – Name by Worth and Name by Reference
26. What’s the sleep() perform?
sleep() perform in C permits the customers to attend for a present thread for a given period of time. sleep() perform will sleep the current executable for the given period of time by the thread however different operations of the CPU will perform correctly. sleep() perform returns 0 if the requested time has elapsed.
For extra data, seek advice from the article – sleep() Operate in C
27. What are enumerations?
In C, enumerations (or enums) are user-defined knowledge sorts. Enumerations enable integral constants to be named, which makes a program simpler to learn and keep. For instance, the times of the week might be outlined as an enumeration and can be utilized wherever in this system.
enum enumeration_name{constant1, constant2, ... };
C
|
Within the above instance, we declared “day” because the variable, and the worth of “Wed” is allotted to day, which is 2. So because of this, 2 is printed.
For extra data, seek advice from the article – Enumeration (or enum) in C
28: What’s a unstable key phrase?
Unstable key phrase is used to forestall the compiler from optimization as a result of their values can’t be modified by code that’s outdoors the scope of present code at any time. The System at all times reads the present worth of a unstable object from the reminiscence location slightly than conserving its worth in a brief register on the level it’s requested, even when earlier instruction is requested for the worth from the identical object.
29. Write a C program to print the Fibonacci collection utilizing recursion and with out utilizing recursion.
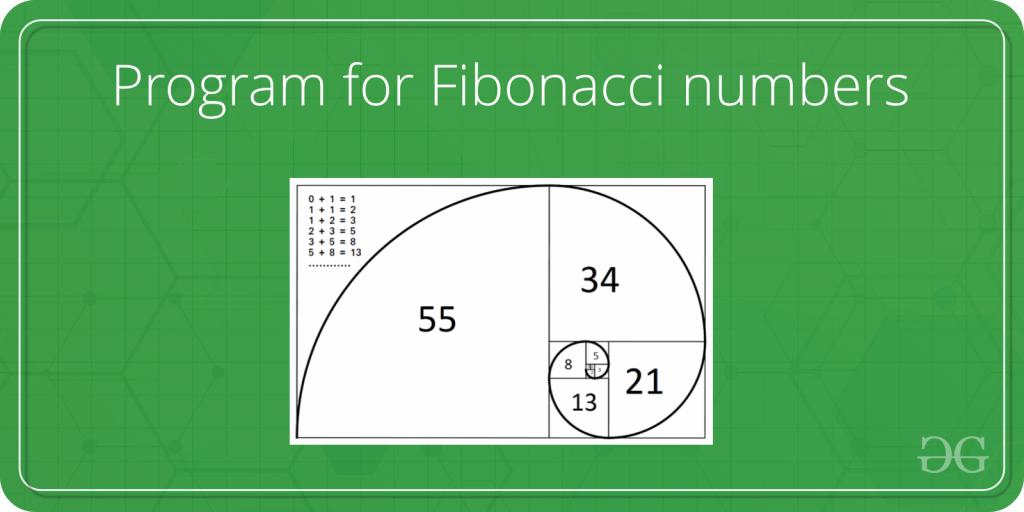
C
|
Output: Please Enter variety of Parts: 5 Fibonacci Sequence with the assistance of Recursion: 0 1 1 2 3 Fibonacci Sequence with out Utilizing Recursion: 0 1 1 2 3
For extra data, seek advice from the article – Fibonacci Numbers
30. Write a C program to test whether or not a quantity is prime or not.
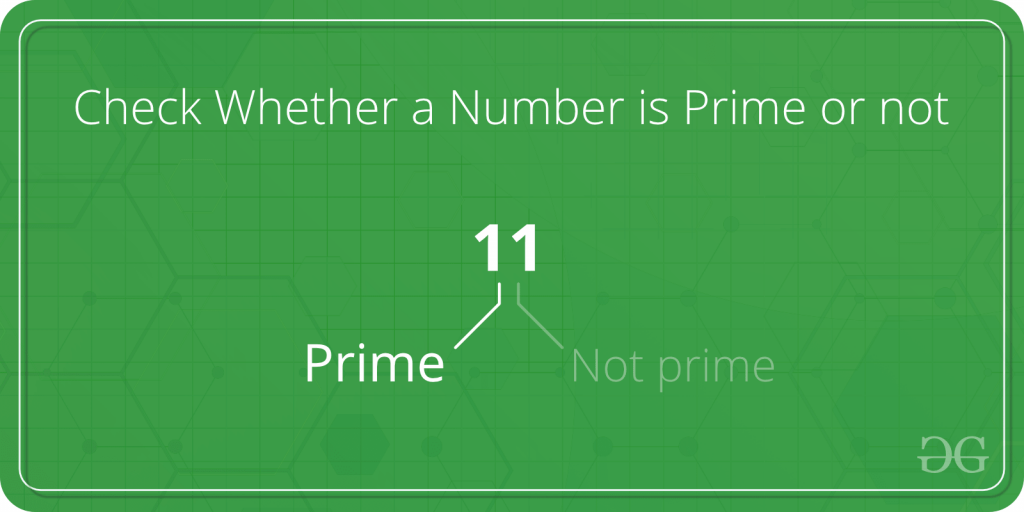
C
|
For extra data, seek advice from the article – Prime or Not
31. How is supply code totally different from object code?
Supply Code | Object Code |
---|---|
Supply code is generated by the programmer. | object code is generated by a compiler or one other translator. |
Excessive-level code which is human-understandable. | Low-level code is just not human-understandable. |
Supply code might be simply modified and accommodates much less variety of statements than object code. | Object code can’t be modified and accommodates extra statements than supply code. |
Supply code might be modified over time and isn’t system particular. | Object code might be modified and is system particular. |
Supply code is much less near the machine and is enter to the compiler or every other translator. | Supply code is extra near the machine and is the output of the compiler or every other translator. |
Language translators like compilers, assemblers, and interpreters are used to translate supply code to object code. | Object code is machine code so it doesn’t require any translation. |
For extra data, seek advice from the article – Supply vs Object Code.
32. What’s static reminiscence allocation and dynamic reminiscence allocation?
- Static reminiscence allocation: Reminiscence allocation which is completed at compile time is named static reminiscence allocation. Static reminiscence allocation saves operating time. It’s quicker than dynamic reminiscence allocation as reminiscence allocation is completed from the stack. This reminiscence allocation methodology is much less environment friendly as in comparison with dynamic reminiscence allocation. It’s principally most well-liked within the array.
- Dynamic reminiscence allocation: Reminiscence allocation achieved at execution or run time is named dynamic reminiscence allocation. Dynamic reminiscence allocation is slower than static reminiscence allocation as reminiscence allocation is completed from the heap. This reminiscence allocation methodology is extra environment friendly as in comparison with static reminiscence allocation. It’s principally most well-liked within the linked listing.
For extra data, seek advice from the article – Static and Dynamic Reminiscence Allocation in C
33. What’s pass-by-reference in features?
Cross by reference permits a perform to change a variable with out making a duplicate of the variable. The Reminiscence location of the handed variable and parameter is identical, so any adjustments achieved to the parameter might be mirrored by the variables as nicely.
C
|
For extra data, seek advice from the article – Cross By Reference
34. What’s a reminiscence leak and learn how to keep away from it?
Each time a variable is outlined some quantity of reminiscence is created within the heap. If the programmer forgets to delete the reminiscence. This undeleted reminiscence within the heap is known as a reminiscence leak. The Efficiency of this system is decreased because the quantity of accessible reminiscence was decreased. To keep away from reminiscence leaks, reminiscence allotted on the heap ought to at all times be cleared when it’s now not wanted.
For extra data, seek advice from the article – Reminiscence Leak
35. What are command line arguments?
Arguments which are handed to the primary() perform of this system within the command-line shell of the working system are often known as command-line arguments.
Syntax:
int primary(int argc, char *argv[]){/*code which is to be executed*/}
For extra data, seek advice from the article – Command Line Arguments in C
36. What’s an auto key phrase?
Each native variable of a perform is named an automated variable within the C language. Auto is the default storage class for all of the variables that are declared inside a perform or a block. Auto variables can solely be accessed throughout the block/perform they’ve been declared. We are able to use them outdoors their scope with the assistance of pointers. By default auto key phrase include a rubbish worth.
For extra data, seek advice from the article – Storage Courses in C
37. Write a program to print “Howdy-World” with out utilizing a semicolon.
C
|
For extra data, seek advice from the article – Howdy World in C
38. Write a C program to swap two numbers with out utilizing a 3rd variable.

C
|
Values earlier than swap are var1 = 50 and var2 = 60 Values after swap are var1 = 60 and var2 = 50
39. Write a program to test whether or not a string is a palindrome or not.
C
|
abba is a Palindrome
40. Clarify modifiers.
Modifiers are key phrases which are used to alter the which means of primary knowledge sorts in C language. They specify the quantity of reminiscence that’s to be allotted to the variable. There are 5 knowledge sort modifiers within the C programming language:
- lengthy
- brief
- signed
- unsigned
- lengthy lengthy
C Programming Interview Questions – Skilled Stage
41. Write a program to print factorial of a given quantity with the assistance of recursion.
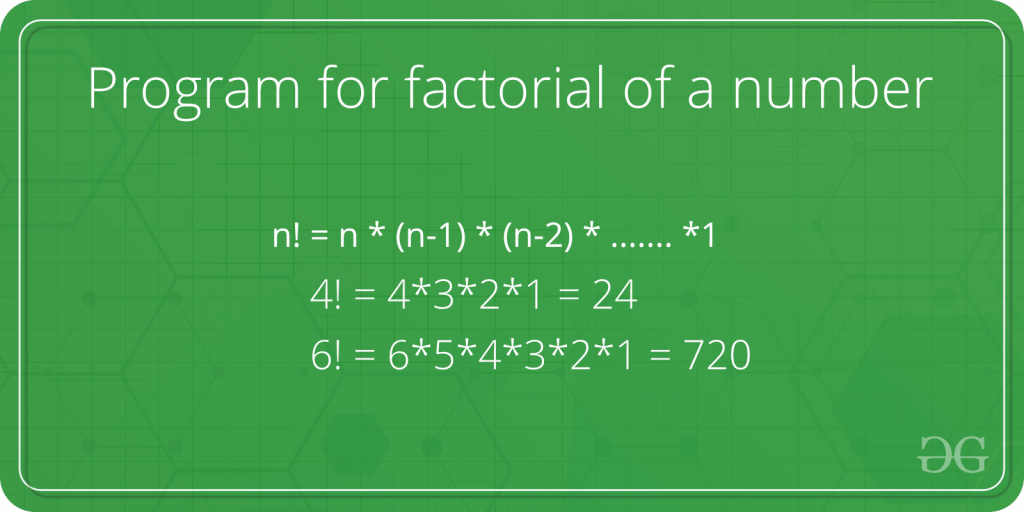
C
|
Factorial of 5 is 120
42. Write a program to test an Armstrong quantity.
C
|
Enter Quantity 0 is an Armstrong quantity
43. Write a program to reverse a given quantity.
C
|
Output:
Enter Quantity to be reversed : Quantity After reversing digits is: 321
44. What’s using an extern storage specifier?
The extern key phrase is used to increase the visibility of the C variables and features within the C language. Extern is the brief identify for exterior. It’s used when a specific file must entry a variable from every other file. Extern key phrase will increase the redundancy and variables with extern key phrase are solely declared not outlined. By default features are seen all through this system, so there isn’t any must declare or outline extern features.
45. What’s using printf() and scanf() features in C Programming language? Additionally, clarify format specifiers.
printf() perform is used to print the worth which is handed because the parameter to it on the console display.
Syntax:
print(“%X”,variable_of_X_type);
scanf() methodology, reads the values from the console as per the info sort specified.
Syntax:
scanf(“%X”,&variable_of_X_type);
In C format specifiers are used to inform the compiler what sort of information might be current within the variable throughout enter utilizing scanf() or output utilizing print().
- %c: Character format specifier used to show and scan character.
- %d, %i: Signed Integer format specifier used to print or scan an integer worth.
- %f, %e, or %E: Floating-point format specifiers are used for printing or scanning float values.
- %s: This format specifier is used for String printing.
- %p: This format specifier is used for Deal with Printing.
For extra data, seek advice from the article – Format Specifier in C
46. What’s close to, far, and big pointers in C?
- Close to Pointers: Close to pointers are used to retailer 16-bit addresses solely. Utilizing the close to pointer, we can’t retailer the tackle with a measurement higher than 16 bits.
- Far Pointers: A far pointer is a pointer of 32 bits measurement. Nevertheless, data outdoors the pc’s reminiscence from the present section will also be accessed.
- Large Pointers: Large pointer is usually thought of a pointer of 32 bits measurement. However bits positioned outdoors or saved outdoors the segments will also be accessed.
47. Point out file operations in C.
In C programming Fundamental File Dealing with Strategies offers the fundamental functionalities that programmers can carry out towards the system.
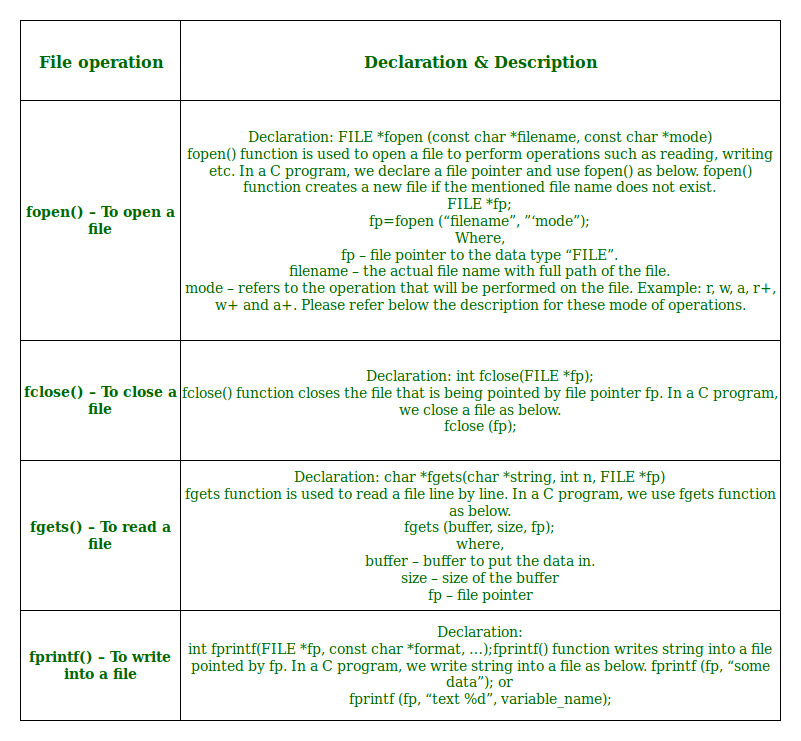
48. Write a Program to test whether or not a linked listing is round or not.
C
|
For extra data, seek advice from the article – Round Linked Record
49. Write a program to Merge two sorted linked lists.
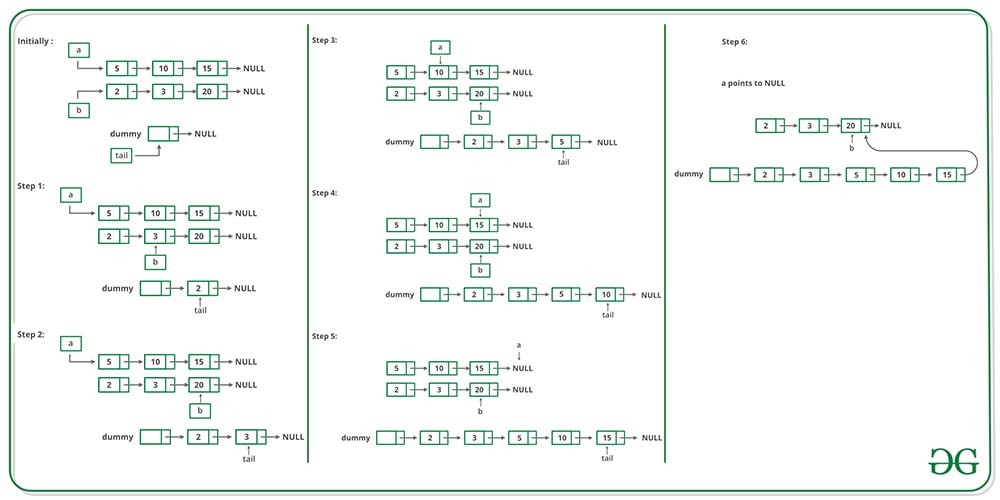
C
|
Merged Linked Record is: 2 3 5 10 15 20
For extra data, seek advice from the article – Merge Two Sorted Linked Record
50. What’s the distinction between getc(), getchar(), getch() and getche().
- getc(): The perform reads a single character from an enter stream and returns an integer worth (sometimes the ASCII worth of the character) if it succeeds. On failure, it returns the EOF.
- getchar(): Not like getc(), gechar() can learn from customary enter; it’s equal to getc(stdin).
- getch(): It’s a nonstandard perform and is current in ‘conio.h’ header file which is usually utilized by MS-DOS compilers like Turbo C.
- getche(): It reads a single character from the keyboard and shows it instantly on the output display with out ready for enter key.
For extra data, seek advice from the article – Distinction between getc(), getchar(), getch(), getche()