Introduction
On this article, we’ll find out about NestJS, Vite, and esbuild; how they work collectively; and configure a NestJS app to utilize each different instruments as dependencies. Within the course of, we’ll get to learn to work with them in actual life eventualities, their main options, and use instances.
Leap forward:
What’s NestJS?
NestJS is a Node.js framework for constructing environment friendly and scalable enterprise server-side/backend purposes. In response to the documentation, it helps all newest ECMAScript variations of each JavaScript and TypeScript.
NestJS combines the well-known programming ideas and philosophies of OOP, useful programming, and useful reactive programming to resolve the architectural challenges within the design of backend purposes which might be scalable, maintainable, simply testable, and should not tightly coupled collectively.
Though NestJS is platform unbiased and might work with any Node.js library if a binding is written for it, NestJS makes use of Specific as a dependency by default, and may also be configured to make use of Fastify. This ease in configuring the framework through uncovered APIs with different third-party modules makes it very straightforward for builders to customise the framework on a case-by-case foundation.
What’s Vite?
Vite is a construct software with a number of options, chief of which is a near-instant dev server startup time. It leverages the introduction of native ES modules within the browser and tooling written with languages that compile to native code to resolve the problems with earlier construct instruments (webpack, Parcel, and so on.) regarding efficiency.
Vite works by first dividing the modules in an software into two classes, dependencies and supply code, as a result of dependencies not often change throughout improvement. Vite pre-bundles these dependencies utilizing esbuild below the hood. For supply code which may want remodeling (CSS, JSX, and so on.), Vite serves them over native ESM to the browser.
Because the browser makes the requests for the supply code, Vite transforms and masses them on demand and the browser can use route-based code-splitting and conditional dynamic imports to bundle the wanted code, making it a reasonably quick course of.
What’s esbuild?
esbuild is a blazing quick JavaScript bundler written in Go and makes use of Go’s parallelism and skill to remodel supply code to machine code. Its options embody, amongst others:
- Enormous plugin help
- A minifier
- TypeScript and JSX help
- Each ES2015 and CommonJS module help
- Tree-shaking capabilities
Putting in and configuring a NestJS app
Now that we now have checked out NestJS, Vite, and esbuild at a excessive stage, let’s proceed to find out about how they work collectively by configuring a NestJS app to utilize each Vite and esbuild as dependencies. Within the course of, we‘ll learn to work with them in actual life eventualities, their main options, and prime use instances.
To get began with NestJS, go forward and set up the CLI, which bootstraps the starter code. That is an particularly higher choice for individuals who are new to NestJS.
An alternative choice is to clone the starter repo from GitHub. Be aware that to put in the JavaScript taste of the starter mission, we will clone this repo, however you’ll want Babel to compile vanilla JavaScript).
For our functions, we’re going to go forward and set up the CLI. Run the next command:
npm i -g @nestjs/cli
After we’re executed putting in the CLI, we will go forward to create a brand new Nest mission:
nest new nest_vite_esbuild_demo
The output of operating this command is proven under:
nest new nest_vite_esbuild_demo took 24s ⚡ We are going to scaffold your app in a couple of seconds.. CREATE nest_vite_esbuild_demo/.eslintrc.js (665 bytes) CREATE nest_vite_esbuild_demo/.prettierrc (51 bytes) CREATE nest_vite_esbuild_demo/README.md (3340 bytes) CREATE nest_vite_esbuild_demo/nest-cli.json (118 bytes) CREATE nest_vite_esbuild_demo/bundle.json (2007 bytes) CREATE nest_vite_esbuild_demo/tsconfig.construct.json (97 bytes) CREATE nest_vite_esbuild_demo/tsconfig.json (546 bytes) CREATE nest_vite_esbuild_demo/src/app.controller.spec.ts (617 bytes) CREATE nest_vite_esbuild_demo/src/app.controller.ts (274 bytes) CREATE nest_vite_esbuild_demo/src/app.module.ts (249 bytes) CREATE nest_vite_esbuild_demo/src/app.service.ts (142 bytes) CREATE nest_vite_esbuild_demo/src/predominant.ts (208 bytes) CREATE nest_vite_esbuild_demo/check/app.e2e-spec.ts (630 bytes) CREATE nest_vite_esbuild_demo/check/jest-e2e.json (183 bytes) ? Which bundle supervisor would you ❤️ to make use of? (Use arrow keys) ❯ npm yarn pnpm
Ensure you have the newest Node.js model put in in your machine (besides v13, which is not supported).
As we will see from the above, the mission listing has been populated with Nest’s core information, dependencies, and base modules. Comply with the steps and choose the bundle supervisor of your alternative. On this publish, we’re utilizing npm. The folder construction on the finish of the set up is proven under.
Extra nice articles from LogRocket:
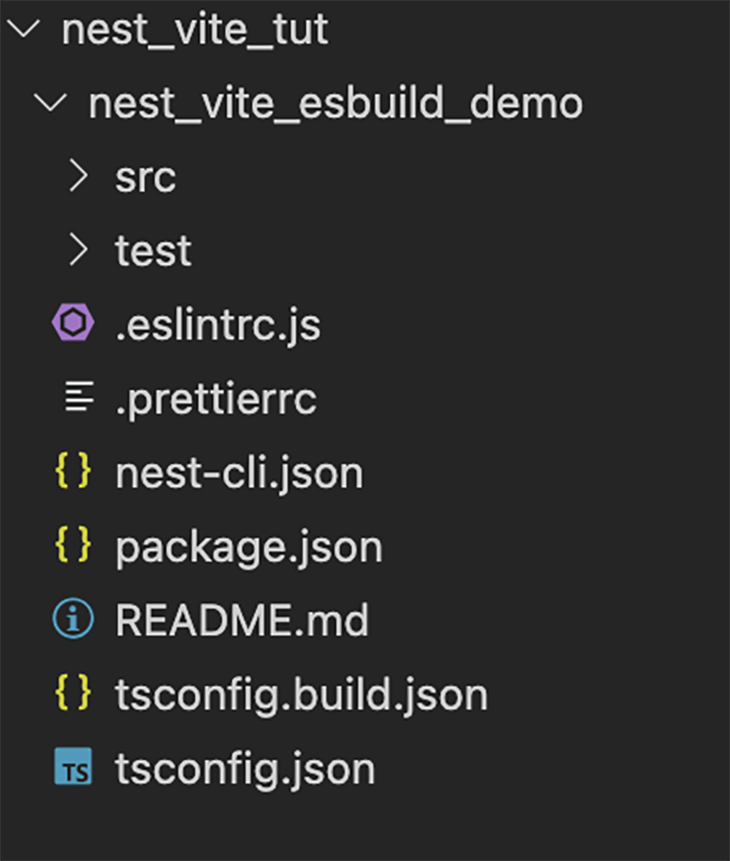
Subsequent, we will navigate into the folder by operating the next command.
cd nest_vite_esbuild_demo
Then, go forward and begin the mission:
npm run begin
The output of operating that command is proven under.
npm run begin > [email protected] begin > nest begin [Nest] 33031 - 08/08/2022, 3:22:16 AM LOG [NestFactory] Beginning Nest software... [Nest] 33031 - 08/08/2022, 3:22:16 AM LOG [InstanceLoader] AppModule dependencies initialized +48ms [Nest] 33031 - 08/08/2022, 3:22:16 AM LOG [RoutesResolver] AppController {/}: +8ms [Nest] 33031 - 08/08/2022, 3:22:16 AM LOG [RouterExplorer] Mapped {/, GET} route +3ms [Nest] 33031 - 08/08/2022, 3:22:16 AM LOG [NestApplication] Nest software efficiently began +8ms
As a substitute for the above, we will additionally begin our software in improvement mode to observe for file adjustments, recompile the construct, and reload the dev server by operating the command under:
npm run begin:dev
Then, navigate to http://localhost:3000/
within the browser. Additional, we will additionally go forward and carry out a handbook set up of NestJS’s core dependencies. On this setup, we will arrange our mission construction as we want, simply by operating the command under.
npm i --save @nestjs/core @nestjs/frequent reflect-metadata
Exploring the NestJS boilerplate
Once we navigate to the src
listing inside our mission folder, we will see the default information created for us. We’ve:
- The
app.controller.ts
file, which represents our handler with only one route - The
app.service.ts
file, which handles something associated to technique or utility features, in order to maintain the controller slim - The
app.module
file, which handles the bottom module for our software (a approach of structuring our software parts) - The
app.controller.spec.ts
file, which handles testing our controller logic - The
predominant.ts
file, which is entry level of our software and creates a Nest software occasion utilizing the coreNestFactory
operate
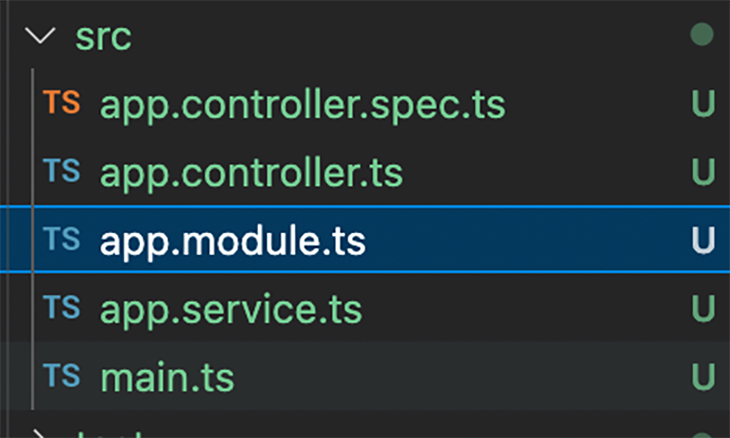
src
listingNestJS encourages builders to maintain their software structure as modular as potential, with every listing contained in the supply listing representing a single module. Every Nest software should have a minimum of one module, a root module, which is the start line for constructing the applying graph.
For instance, we will run the next command to create a brand new module:
nest g module product_demo
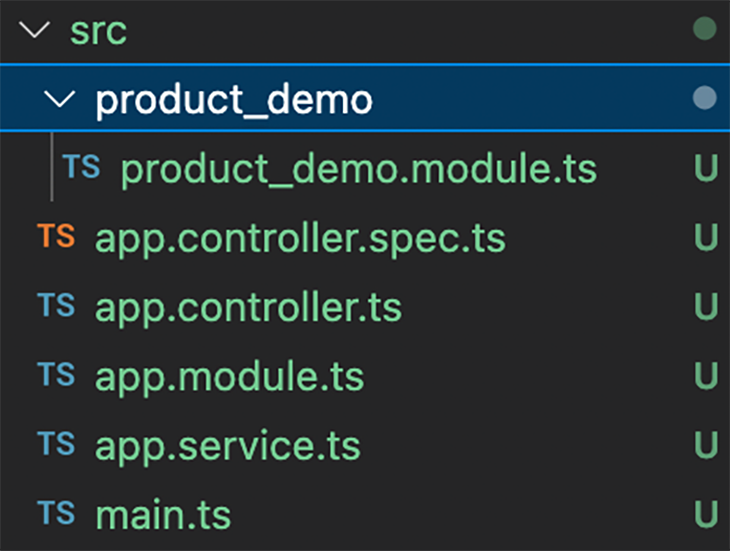
src
listingAs you’ll be able to see from the above, we now have created a product_demo
module. We will then go forward to create the opposite wanted information — controller
and providers
— and likewise import the product_demo
module into the app base module.
For extra data concerning NestJS fundamentals and options, we will make reference to the documentation, together with testing, suppliers, lifecycle occasions, decorators, middleware, modules and so on., to achieve mastery of the framework. Subsequent, allow us to go forward and arrange Vite and esbuild on our NestJS software.
Putting in Vite and esbuild with NestJS
Vite is plugin-based and likewise comes with a well-optimized and quick construct course of, which may tremendously enhance your total developer productiveness and expertise. It additionally helps TypeScript out of the field.
Now, let’s combine Vite into our NestJS backend app. We’re going to set up Vite through the plugin, which runs a Node dev server with scorching module alternative.
Run the command under as a improvement dependency.
npm set up vite vite-plugin-node -D
Subsequent, within the root of our mission listing, we will create the vite.config.ts
file, which configures our mission to utilize the plugin. Let’s see the contents of that file under.
import { defineConfig } from 'vite'; import { VitePluginNode } from 'vite-plugin-node'; export default defineConfig({ // ...vite configures server: { // vite server configs, for particulars see [vite doc](https://vitejs.dev/config/#server-host) port: 3000 }, plugins: [ ...VitePluginNode({ // Nodejs native Request adapter // currently this plugin support 'express', 'nest', 'koa' and 'fastify' out of box, // you can also pass a function if you are using other frameworks, see Custom Adapter section adapter: 'nest', // tell the plugin where is your project entry appPath: './src/main.ts', // Optional, default: 'viteNodeApp' // the name of named export of you app from the appPath file exportName: 'viteNodeApp', // Optional, default: 'esbuild' // The TypeScript compiler you want to use // by default this plugin is using vite default ts compiler which is esbuild // 'swc' compiler is supported to use as well for frameworks // like Nestjs (esbuild dont support 'emitDecoratorMetadata' yet) // you need to INSTALL `@swc/core` as dev dependency if you want to use swc tsCompiler: 'esbuild', }) ], optimizeDeps: { // Vite doesn't work effectively with optionnal dependencies, // mark them as ignored for now exclude: [ '@nestjs/microservices', '@nestjs/websockets', 'cache-manager', 'class-transformer', 'class-validator', 'fastify-swagger', ], }, });
Subsequent, we have to replace the entry level of our app server file (predominant.ts
) to export the app named viteNodeApp
(within the Vite config exportName
area above) or every other identify we now have configured within the Vite config file above. See under.
import { NestFactory } from '@nestjs/core'; import { AppModule } from './app.module'; if (import.meta.env.PROD) { async operate bootstrap() { const app = await NestFactory.create(AppModule); await app.pay attention(3000); } bootstrap(); } export const viteNodeApp = NestFactory.create(AppModule);
Subsequent, in our bundle.json
file, we will go forward and add an npm script to run the dev server with the common npm run dev
command:
"scripts": { "dev": "vite" }
As we now have earlier talked about, Vite comes with a dev server that serves our supply information over native ESM. Once we run our app with the Vite dev server utilizing the npm run dev
command after your entire setup, we’ll see the output under.
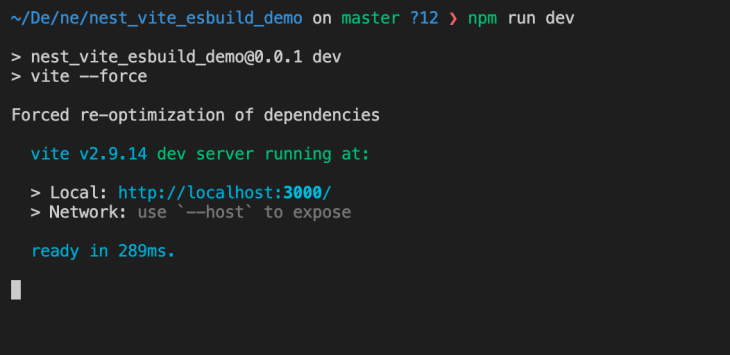
npm run dev
commandTo make certain every thing continues to be working as anticipated, run the check suite with the npm run check
command and we will see the output under.
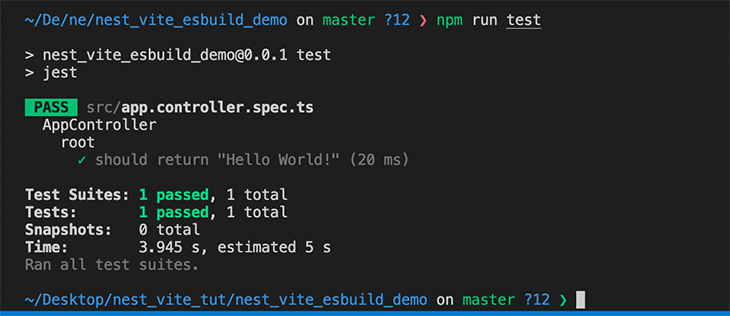
npm run check
commandThe plugin additionally makes use of Vite’s server-side rendering mode to construct our app. To utilize this characteristic, we will go forward and add a construct script to our bundle.json
file (utilizing Vite to construct our app as a substitute of Nest):
"scripts": { "construct": "vite construct" },
The vite construct
command bundles our code with Rollup and spits out extremely optimized property for our manufacturing setting. Once we construct our app with Vite by operating the npm run construct
command after your entire setup, we will see the output under.
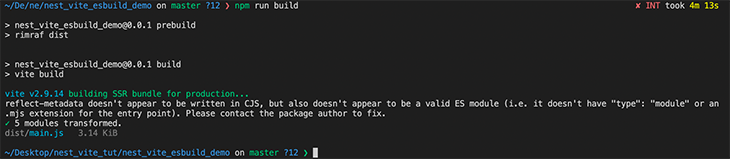
npm run construct
commandAs talked about earlier than, Vite makes use of esbuild below the hood to transpile TypeScript to JavaScript. It’s a very quick transpilation course of — it might probably even be as much as 20 occasions sooner than common TypeScript compilers, and HMR updates can mirror within the browser in below 50ms.
One of many benefits of utilizing the Vite plugin (vite-plugin-node) is that we will select to make use of both esbuild or swc to compile our TypeScript information, although we used solely esbuild on this publish.
Conclusion
On this publish, we now have realized get going with NestJS, Vite, and esbuild. As we now have seen, NestJS has an attention-grabbing and relatively new method to constructing Node.js purposes with a philosophy round OOP, FP, and FRP. These enhancements tremendously enhance developer time and productiveness in the long term, as we don’t need to trouble about design our modules and parts. NestJS solves that for us and proposes a one-module-per-folder sample. We will additionally use the facility of TypeScript to jot down our backend code.
Vite, however, is on a complete new stage on the planet of construct instruments. It’s coming at a time the place widespread JS-based bundlers and construct tooling within the NodeJS ecosystem wanted a lift when it comes to efficiency. Vite compiles our code to native code utilizing a very totally different method from webpack and Parcel. As we now have realized, it makes use of the browser and native ESM for bundling.
In all, we now have mixed these superior applied sciences to construct a easy boilerplate backend that may be a place to begin to your subsequent NestJS mission.