Laptop Imaginative and prescient is likely one of the purposes of deep neural networks that allows us to automate duties that earlier required years of experience and one such use in predicting the presence of cancerous cells.
On this article, we are going to discover ways to construct a classifier utilizing the Switch Studying method which might classify regular lung tissues from cancerous. This undertaking has been developed utilizing collab and the dataset has been taken from Kaggle whose hyperlink has been offered as properly.
Switch Studying
In a convolutional neural community, the primary activity of the convolutional layers is to reinforce the necessary options of a picture. If a specific filter is used to determine the straight strains in a picture then it is going to work for different pictures as properly that is notably what we do in switch studying. There are fashions that are developed by researchers by regress hyperparameter tuning and coaching for weeks on thousands and thousands of pictures belonging to 1000 completely different courses like imagenet dataset. A mannequin that works properly for one laptop imaginative and prescient activity proves to be good for others as properly. Due to this motive, we leverage these skilled convolutional layers parameters and tuned hyperparameter for our activity to acquire larger accuracy.
Importing Libraries
Python libraries make it very simple for us to deal with the info and carry out typical and sophisticated duties with a single line of code.
- Pandas – This library helps to load the info body in a 2D array format and has a number of capabilities to carry out evaluation duties in a single go.
- Numpy – Numpy arrays are very quick and may carry out giant computations in a really quick time.
- Matplotlib – This library is used to attract visualizations.
- Sklearn – This module accommodates a number of libraries having pre-implemented capabilities to carry out duties from knowledge preprocessing to mannequin growth and analysis.
- OpenCV – That is an open-source library primarily targeted on picture processing and dealing with.
- Tensorflow – That is an open-source library that’s used for Machine Studying and Synthetic intelligence and offers a variety of capabilities to attain advanced functionalities with single strains of code.
Python3
|
Importing Dataset
The dataset which we are going to use right here has been taken from-https://www.kaggle.com/datasets/andrewmvd/lung-and-colon-cancer-histopathological-images. . This dataset consists of 5000 pictures for 3 courses of lung situations:
- Regular Class
- Lung Adenocarcinomas
- Lung Squamous Cell Carcinomas
These pictures for every class have been developed from 250 pictures by performing Information Augmentation on them. That’s the reason we received’t be utilizing Information Augmentation additional on these pictures.
Python3
|
Output:
The information set has been extracted.
Information Visualization
On this part, we are going to attempt to perceive visualize some pictures which have been offered to us to construct the classifier for every class.
Python3
|
Output:
['lung_n', 'lung_aca', 'lung_scc']
These are the three courses that we now have right here.
Python3
|
Output:
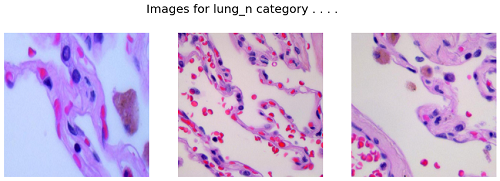
Pictures for lung_n class
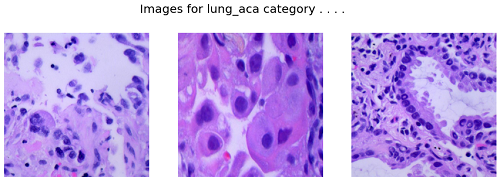
Pictures for lung_aca class
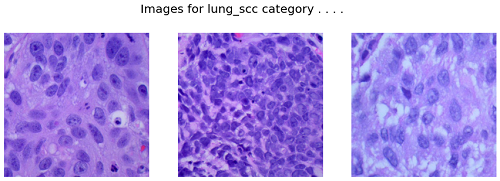
Pictures for lung_scc class
The above output might range if you’ll run this in your pocket book as a result of the code has been carried out in such a method that it’ll present completely different pictures each time you rerun the code.
Information Preparation for Coaching
On this part, we are going to convert the given pictures into NumPy arrays of their pixels after resizing them as a result of coaching a Deep Neural Community on large-size pictures is very inefficient by way of computational value and time.
For this objective, we are going to use the OpenCV library and Numpy library of python to serve the aim. Additionally, in any case the pictures are transformed into the specified format we are going to break up them into coaching and validation knowledge so, that we will consider the efficiency of our mannequin.
Python3
|
A number of the hyperparameters which we will tweak from right here for the entire pocket book.
Python3
|
One sizzling encoding will assist us to coach a mannequin which might predict smooth possibilities of a picture being from every class with the best chance for the category to which it actually belongs.
Python3
|
Output:
(12000, 256, 256, 3) (3000, 256, 256, 3)
On this step, we are going to obtain the shuffling of the info mechanically as a result of the train_test_split perform break up the info randomly within the given ratio.
Mannequin Growth
We are going to use pre-trained weight for an Inception community which is skilled on imagenet dataset. This dataset accommodates thousands and thousands of pictures for round 1000 courses of pictures.
Mannequin Structure
We are going to implement a mannequin utilizing the Practical API of Keras which can include the next components:
- The bottom mannequin is the Inception mannequin on this case.
- The Flatten layer flattens the output of the bottom fashions output.
- Then we may have two totally related layers adopted by the output of the flattened layer.
- We’ve included some BatchNormalization layers to allow secure and quick coaching and a Dropout layer earlier than the ultimate layer to keep away from any chance of overfitting.
- The ultimate layer is the output layer which outputs smooth possibilities for the three courses.
Python3
|
Output:
87916544/87910968 [==============================] – 2s 0us/step
87924736/87910968 [==============================] – 2s 0us/step
Python3
|
Output:
311
That is how deep this mannequin is that this additionally justifies why this mannequin is very efficient in extracting helpful options from pictures which helps us to construct classifiers.
The parameters of a mannequin we import are already skilled on thousands and thousands of pictures and for weeks so, we don’t want to coach them once more.
Python3
|
‘Mixed7’ is likely one of the layers within the inception community whose outputs we are going to use to construct the classifier.
Python3
|
Output:
final layer output form: (None, 14, 14, 768)
Python3
|
Callback
Callbacks are used to examine whether or not the mannequin is enhancing with every epoch or not. If not then what are the required steps to be taken like ReduceLROnPlateau decreases studying fee additional? Even then if mannequin efficiency shouldn’t be enhancing then coaching might be stopped by EarlyStopping. We are able to additionally outline some customized callbacks to cease coaching in between if the specified outcomes have been obtained early.
Python3
|
Now we are going to prepare our mannequin:
Python3
|
Output:
Mannequin coaching progress
Let’s visualize the coaching and validation accuracy with every epoch.
Python3
|
Output:
Graph of loss and accuracy epoch by epoch for coaching and validation knowledge.loss
From the above graphs, we will definitely say that the mannequin has not overfitted the coaching knowledge because the distinction between the coaching and validation accuracy could be very low.
Mannequin Analysis
Now as we now have our mannequin prepared let’s consider its efficiency on the validation knowledge utilizing completely different metrics. For this objective, we are going to first predict the category for the validation knowledge utilizing this mannequin after which examine the output with the true labels.
Python3
|
Let’s draw the confusion metrics and classification report utilizing the anticipated labels and the true labels.
Python3
|
Output:
Confusion matrix for the validation knowledge
Python3
|
Output:
Classification report for the validation knowledge
Conclusion:
Certainly the efficiency of our mannequin utilizing the Switch Studying Method has achieved larger accuracy with out overfitting which is superb because the f1-score for every class can be above 0.90 which implies our mannequin’s prediction is right 90% of the time.