Can we use PyTorch in Rust? What are Rust bindings? What’s tch-rs? A glance on neural networks in Rust
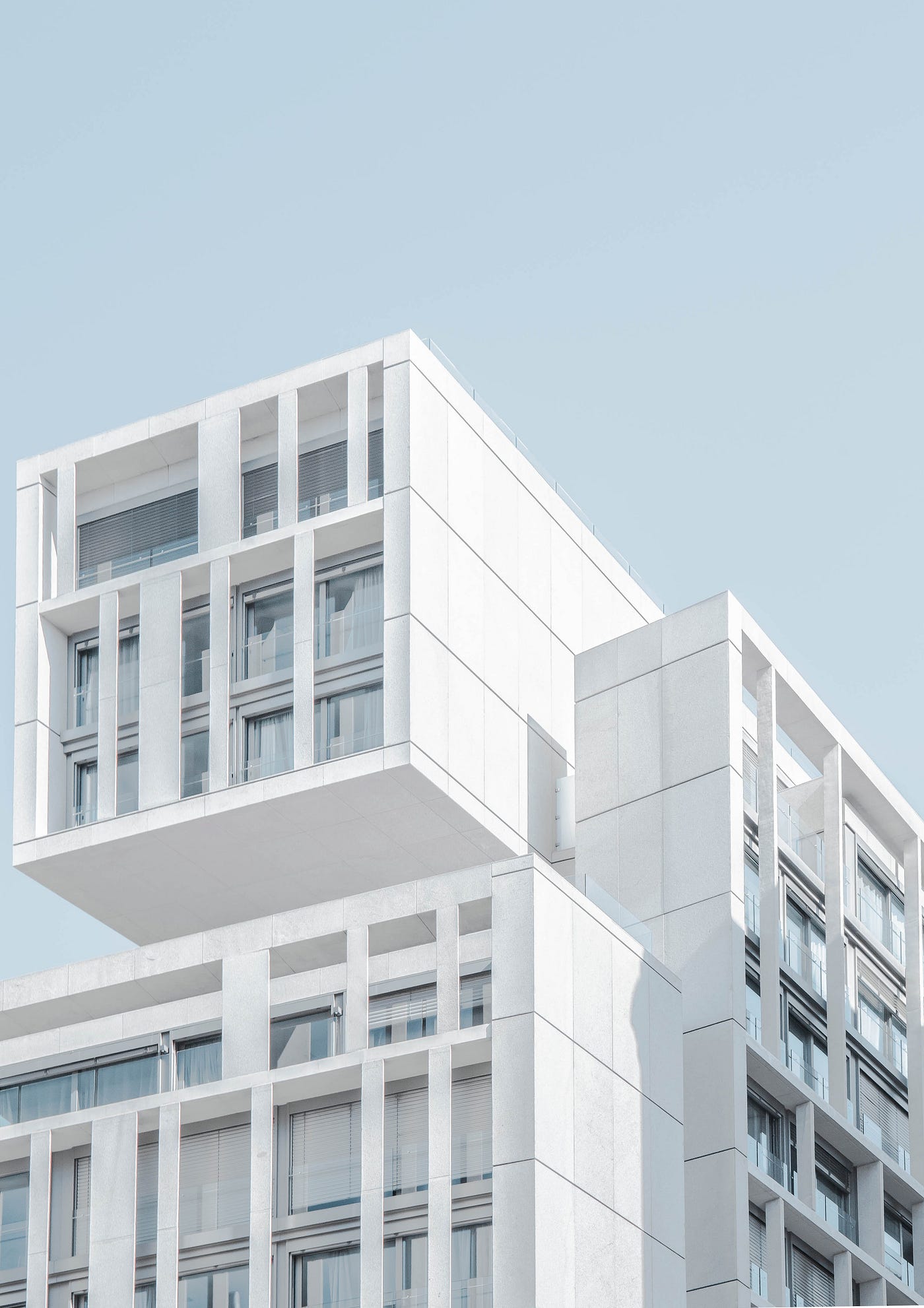
It’s been some time for the reason that final time once we had a have a look at Rust and its software to Machine Studying — please, scroll right down to the underside for the earlier tutorials on ML and Rust. At the moment I wish to current you a step ahead, introducing neural networks in Rust. There exists a Rust Torch, which permits us to create any type of neural community we would like. The Bindings are the important thing level to touchdown a Rust Torch. Bindings permit the creation of overseas operate interfaces or FFIs, which create a bridge between Rust and capabilities/codes written in a language. Good examples may be discovered within the Rust nomicon
To create bindings with C and C++ we will use bindgen, a library that mechanically generated Rust FFI. From bindings to C++ api of PyTorch, Laurent Mazare has helped the Rust group to have a Rustacean model of PyTorch. Because the GitHub web page says, tch offers skinny wrappers across the C++ libtorch . The massive benefit is that the library is strictly much like the unique ones, so there aren’t any studying limitations to beat. The core code is sort of straightforward to learn.
To start with, let’s take a look on the code. That is the very best place to begin to get an extra understanding of the Rust infrastructure.
Firstly, to have an concept about Rust FFI we will peep these recordsdata . Most of them are mechanically generated, whereas Laurent and coworkers have put collectively magnificent items of code to attach C++ Torch APIs with Rust.
Following, we will begin studying the core code in src
, specifically, let’s take a look at init.rs
. After the definition of an enum Init
there’s a public operate pub fn f_init
, which matches the enter initialisation methodology and returns a tensor for weights and one for biases. We are able to study the usage of match
which displays swap
in C and match
in Python 3.10. Weights and bias tensors are initialised by random, uniform, Kaiming, or orthogonal strategies (fig.1).
Then, for the kind enum Init
now we have the strategies implementation impl Init
. The carried out methodology is a setter pub fn set(self, tensor: &mut Tensor)
which is a good instance to additional respect the idea of possession and borrowship in Rust:
We talked about borrowship in our very first tutorial. It’s the correct time to know higher this idea. Suppose we might have the same set
operate:
pub fn set(self, tensor: Tensor){}
In the principle code, we might name this operate, passing a tensor Tensor
. The Tensor
might be set and we might be glad. Nevertheless, what if we’re calling set
on Tensor
once more? Properly, we’d run into the error worth used right here after transfer
. What does this imply? This error is telling you that you just moved Tensor
into set
. A transfer
means that you’ve got transferred possession to self
in set
While you’re calling set(self, tensor: Tensor)
once more, you wish to have possession again of Tensor
for organising once more. Fortunately in Rust this isn’t attainable, in a different way in C++. In Rust, as soon as a transfer
has been carried out the reminiscence allotted for the method will get deallocated. Thus, what we wish to do right here is to borrow the worth of Tensor
to set
so we will preserve possession. To do this we have to name Tensor
by reference, so tensor: &Tensor
. Since we expect Tensor
to mutate we’ll have so as to add mut
so: tensor: &mut Tensor
Shifting ahead, we will see one other vital factor, which is easy and makes use of the Init
class: Linear
, particularly a completely related neural community layer:
Fig. 3 reveals how straightforward is to arrange a completely related layer, which is product of a weight matrix ws_init
and bias matrix bs_init
. The default initialisation is made with tremendous::Init::KaimingUniform
for weights, a operate we noticed above.
The primary absolutely related layer can then be created with the operate linear
. As you possibly can see within the operate signature, particularly what’s between the <...>
, there are just a few fascinating issues (fig.4). Firstly, the lifetime annotation'a
. As we stated above Rust mechanically recognises when a variable has gone out of scope and may be freed. We are able to annotate some variables to have a particular lifetime, so we will determine how lengthy they will dwell. The usual annotation is 'a
the place '
denotes a lifetime parameter. One vital factor to recollect is that this signature doesn’t modify something throughout the operate, however it tells the operate borrower to recognise all these variables whose lifetime can fulfill the constraints we’re imposing.
The second argument is T: Borrow<tremendous::Path<'a>
This annotation means: take nn::Path
laid out in var_store.rs
and borrow this kind to T
. Any sort in Rust is free to borrow as a number of differing kinds. This kind might be used to outline the enter {hardware} (e.g. GPU), as you possibly can see with vs:T
. Lastly, the enter and output dimensions of the community are specified as integers in_dim: i64, out_dim: i64
together with the LinearConfig
for initialization of weight and bias c: LinearConfig.
It’s time to get our palms soiled and play with Torch Rust. Let’s arrange a easy linear neural community, then a sequential community, and at last a convolutional neural community utilizing the MNIST dataset. As at all times yow will discover all of the supplies on my ML ❤ Rust repo. Yann LeCun and Corinna Cortes maintain the copyright of MNIST dataset and it has been made accessible underneath the phrases of the Artistic Commons Attribution-Share Alike 3.0 license.
A easy neural community in Rust
As at all times, step one for a brand new Rust venture is cargo new NAME_OF_THE_PROJECT
on this case simple_neural_networks
. Then, we will begin organising the Cargo.toml
with all of the packages we want: we’ll be utilizing mnist
, ndarry
and clearly tch
— fig.5. I made a decision to make use of mnist
to extract the unique MNIST information, so we will see rework and cope with array and tensors. Be at liberty to make use of the imaginative and prescient
useful resource already current in tch.
We’ll be utilizing mnist
to obtain the MNIST dataset, and ndarray
to carry out some transforms on the picture vectors, and convert them into tch::Tensor
.
Let’s soar to the foremost.rs
code. In a nutshell, we want:
- to obtain and extract the MNIST photographs and return a vector for coaching, validation, and take a look at information.
- From these vectors, we’ll must carry out some conversion to
Tensor
so we’ll have the ability to usetch
. - Lastly, we’ll implement a sequence of epochs, in every epoch we’ll multiply the enter information with the neural community weight matrix and we’ll carry out backpropagation to replace the load values.
mnist
mechanically downloads the enter recordsdata from right here. We have to add options = ['download']
in Cargo.toml
to activate the obtain performance. After recordsdata have been downloaded, uncooked information is extracted — download_and_extract()
— and subdivided into coaching, validation and take a look at units. Be aware that the principle operate won’t return something, so you have to specify -> Outcomes<(), Field<dyn, Error>>
and Okay(())
on the finish of the code (fig.6)
Now, the very first Torch factor of the code: convert an array to Tensor.
The output information from mnist
is Vec<u8>
. The coaching vector construction has aTRAIN_SIZE
variety of photographs, whose dimensions areHEIGHT
instances WIDTH
. These three parameters may be specified as usize
sort and, along with the enter data-vector, they are often handed to image_to_tensor
operate, as proven in fig.7, returning Tensor
The enter Vec<u8>
information may be reshaped to Array3
with from_shape_vec
and values are normalised and transformed to f32
, particularly .map(|x| *x as f32/256.0)
. From an array it’s straightforward to construct up a torch Tensor as proven on line 14, Tensor::of_slice(inp_data.as_slice().unwrap());
. The output tensor dimension might be dim1 x (dim2*dim3)
For our coaching information, setting TRAIN_SIZE=50'000
, HEIGHT=28
and WIDTH=28
, the output coaching tensor dimension might be 50'000 x 784
.
Equally, we’ll convert the labels to a tensor, whose dimension might be dim1
— so for the coaching labels we’ll have a 50'000
lengthy tensor https://github.com/Steboss/ML_and_Rust/blob/aa7d495c4a2c7a416d0b03fe62e522b6225180ab/tutorial_3/simple_neural_networks/src/foremost.rs#L42
We’re now prepared to start out tackling with linear neural community. After a zero-initialization of weight and bias matrices:
let mut ws = Tensor::zeros(&[(HEIGHT*WIDTH) as i64, LABELS], type::FLOAT_CPU).set_requires_grad(true);let mut bs = Tensor::zeros(&[LABELS], type::FLOAT_CPU).set_requires_grad(true);
which resembles the PyTorch implementation, we will begin computing the neural community weights.
Fig.8 reveals the principle routine to run the coaching of a linear neural community. Firstly, we can provide a reputation to the outermost for loop with 'prepare
The apostrophe, on this case, is just not an indicator of a lifetime, however of loop identify. We’re monitoring the loss for every epoch. If two consecutive losses distinction is lower than THRES
we will cease the outermost cycle as we reached convergence — you possibly can disagree, however for the second let’s preserve it 🙂 The complete implementation is tremendous easy to learn, just a bit caveat in extracting the accuracy from the computed logits
and the roles is completed 🙂
When you’re prepared you possibly can immediately run your entire foremost.rs
code with cargo run
On my 2019 MacBook Professional, 2.6GHZ, 6-CORE Intel Core i7, 16GB RAM, the computation takes lower than a minute, attaining a take a look at accuracy of 90.45% after 65 epochs
Sequential neural community
Let’s now see the sequential neural community implementation https://github.com/Steboss/ML_and_Rust/tree/grasp/tutorial_3/custom_nnet
Fig.9 explains how the sequential community is created. Firstly, we have to import tch::nn::Module
. Then we will create a operate for the neural community fn web(vs: &nn::Path) -> impl Module
. This operate returns an implementation for Module
and receives as enter nn::Path
which is structural information concerning the {hardware} to make use of for working the community (e.g. CPU or GPU). Then, the sequential community is carried out as a mixture of linear layer of enter dimension IMAGE_DIM
and HIDDEN_NODES
nodes, a relu
and a ultimate linear layer with HIDDEN_NODES
inputs and LABELS
output.
Thus, in the principle code we’ll name the neural community creation as:
// arrange variable retailer to verify if cuda is accessible
let vs = nn::VarStore::new(Machine::cuda_if_available());// arrange the seq web
let web = web(&vs.root());// arrange optimizer
let mut choose = nn::Adam::default().construct(&vs, 1e-4)?;
together with an Adam optimizer — bear in mind the ?
on the finish of choose
in any other case you’ll return a Consequence<>
sort which doesn’t have the performance we want. At this level we will merely adopted the process as per PyTorch, so we’ll arrange quite a lot of epochs and carry out the backpropagation withthe optimizer’s backward_step
methodology with a given loss
Convolutional neural community
Our ultimate step for at present is coping with convolutional neural community: https://github.com/Steboss/ML_and_Rust/tree/grasp/tutorial_3/conv_nnet/src
At first, you possibly can discover we at the moment are utilizing nn::ModuleT
. This module trait is an extra prepare parameter. That is generally used to distinguish the behaviour of the community between coaching and analysis. Then, we will begin defining the construction of the community Web
which is product of two conv2d layers and two linear ones. The implementation of Web
states how the community is made, the 2 convolutional layers have a stride of 1 and 32, padding 32 and 64, and dilation of 5 and 5 respectively. The linear layers obtain an enter of 1024 and the ultimate layer returns an output of 10 parts. Lastly, we have to outline the ModuleT
implementation for Web
. Right here, the ahead step forward_t
receives an extra boolean argument, prepare
and it’ll return a Tensor
. The ahead step applies the convolutional layer, together with max_pool_2d
and dropout
. The dropout step is only for coaching functions, so it’s sure with the boolean prepare
.
To extend the coaching efficiency, we’ll prepare the conv-layer with batches from the enter tensor. Because of this you have to implement a operate to separate into random batches the enter tensors:
generate_random_index
takes the enter picture array and the batch dimension we wish to break up it to. It creates an output tensor of random integers ::randint
.
Fig.13 reveals the coaching step. The enter dataset is break up into n_it
batches the place let n_it = (TRAIN_SIZE as i64)/BATCH_SIZE;
. For every batch we compute the loss from the community and again propagate the error with backward_step
.
Working the convolutional community on my native laptop computer required jiffy, attaining a validation accuracy of 97.60%.
You made it! I’m pleased with you! At the moment we had a little bit peep to tch
and arrange just a few laptop imaginative and prescient experiments. We noticed the interior construction of the code for the initialization and the linear layer. We reviewed some vital ideas about borrowship in Rust and we discovered what’s a lifetime annotation. Then, we jumped into the implementation of a easy linear neural community, a sequential neural community, and a convolutional one. Right here we discovered course of enter photographs and convert them to tch::Tensor.
We noticed use the module nn:Module
for a easy neural community, to implement a ahead step and we noticed additionally its extension nn:ModuleT
. For all these experiments we noticed two strategies to carry out backpropagation, both with zero_grad
and backward
or with backward_step
immediately utilized to the optimizer.
I hope you loved my tutorial 🙂 Keep tuned for the subsequent episode.