What’s a Linked Listing?
A Linked Listing is a linear information construction which seems to be like a sequence of nodes, the place every node is a distinct factor. Not like Arrays, Linked Listing components will not be saved at a contiguous location.
It’s principally chains of nodes, every node incorporates data similar to information and a pointer to the following node within the chain. Within the linked listing there’s a head pointer, which factors to the primary factor of the linked listing, and if the listing is empty then it merely factors to null or nothing.
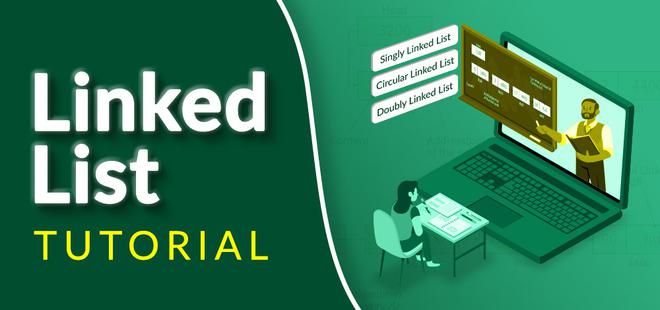
Linked Listing Tutorial
Why linked listing information construction wanted?
Listed here are a couple of benefits of a linked listing that’s listed under, it should allow you to perceive why it’s essential to know.
- Dynamic Information construction: The dimensions of reminiscence will be allotted or de-allocated at run time based mostly on the operation insertion or deletion.
- Ease of Insertion/Deletion: The insertion and deletion of components are easier than arrays since no components must be shifted after insertion and deletion, Simply the handle wanted to be up to date.
- Environment friendly Reminiscence Utilization: As we all know Linked Listing is a dynamic information construction the scale will increase or decreases as per the requirement so this avoids the wastage of reminiscence.
- Implementation: Numerous superior information constructions will be carried out utilizing a linked listing like a stack, queue, graph, hash maps, and so forth.
There are primarily three forms of linked lists:
- Single-linked listing
- Double linked listing
- Round linked listing
Traversal of things will be performed within the ahead path solely because of the linking of each node to its subsequent node.
Illustration of Single linked listing:
C++
|
C
|
Java
|
Python3
|
C#
|
Javascript
|
Generally used operations on Singly Linked Listing:
The next operations are carried out on a Single Linked Listing
- Insertion: The insertion operation will be carried out in 3 ways. They’re as follows…
- Deletion: The deletion operation will be carried out in 3 ways. They’re as follows…
- Search: It’s a strategy of figuring out and retrieving a selected node both from the entrance, the top or anyplace within the listing.
- Show: This course of shows the weather of a Single-linked listing.
Observe issues on Singly linked listing:
S.no | Query | Article |
---|---|---|
1 | Introduction to Linked Listing | View |
2 | Detect loop in a linked listing | View |
3 | Discover size of loop in linked listing | View |
4 | Perform to test if a singly linked listing is palindrome | View |
5 | Take away duplicates from a sorted linked listing | View |
6 | Take away duplicates from an unsorted linked listing | View |
7 | Take away loop in Linked Listing | View |
8 | Swap nodes in a linked listing with out swapping information | View |
9 | Transfer final factor to entrance of a given Linked Listing | View |
10 | Intersection of two Sorted Linked Lists | View |
Traversal of things will be performed in each ahead and backward instructions as each node incorporates a further prev pointer that factors to the earlier node.
Illustration of Doubly linked listing:
A Node Creation:
C++
|
C
|
Java
|
Python3
|
C#
|
Javascript
|
Generally used operations on Double-Linked Listing:
In a double-linked listing, we carry out the next operations…
- Insertion: The insertion operation will be carried out in 3 ways as follows:
- Deletion: The deletion operation will be carried out in 3 ways as follows…
- Show: This course of shows the weather of a double-linked listing.
Observe issues on Doubly linked listing:
S.no | Query | Article |
---|---|---|
1 | Reverse a Doubly Linked Listing | View |
2 | Copy a linked listing with subsequent and arbit pointer | View |
3 | Swap Kth node from starting with Kth node from finish in a Linked Listing | View |
4 | Merge Type for Doubly Linked Listing | View |
5 | Type a okay sorted doubly linked listing | View |
6 | Take away duplicates from an unsorted linked listing | View |
7 | Rotate Doubly linked listing by N nodes | View |
8 | Merge Two Balanced Binary Search Timber | View |
9 | Convert a Binary Tree into Doubly Linked Listing in spiral vogue | View |
10 | Convert a given Binary Tree to Doubly Linked Listing | View |
A round linked listing is a sort of linked listing through which the primary and the final nodes are additionally linked to one another to kind a circle, there is no such thing as a NULL on the finish.
Generally used operations on Round Linked Listing:
The next operations are carried out on a Round Linked Listing
- Insertion: The insertion operation will be carried out in 3 ways:
- Deletion: The deletion operation will be carried out in 3 ways:
- Show: This course of shows the weather of a Round linked listing.
Observe issues on Round linked listing:
S.no | Query | Article |
---|---|---|
1 | Round Linked Listing Traversal | View |
2 | Cut up a Round Linked Listing into two halves | View |
3 | Sorted insert for round linked listing | View |
4 | Examine if a linked listing is Round Linked Listing | View |
5 | Deletion from a Round Linked Listing | View |
6 | Josephus Circle utilizing round linked listing | View |
7 | Convert singly linked listing into round linked listing | View |
8 | Implementation of Deque utilizing round array | View |
9 | Alternate first and final nodes in Round Linked Listing | View |
10 | Depend nodes in Round linked listing | View |
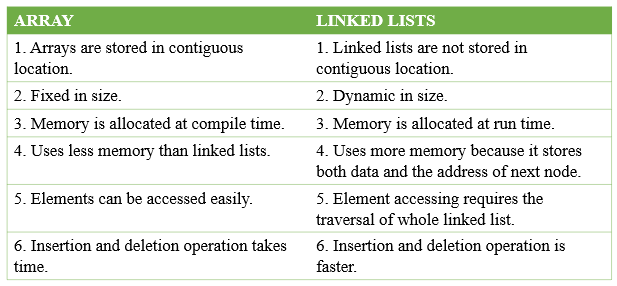
Linked Listing vs. Array
Linked Listing vs. Array in Time Complexity
Operation | Linked listing | Array |
---|---|---|
Random Entry | O(N) | O(1) |
Insertion and deletion at starting | O(1) | (N) |
Insertion and deletion at finish | O(N) | O(1) |
Insertion and deletion at a random place | O(N) | O(N) |
- Dynamic nature: Linked lists are used for dynamic reminiscence allocation.
- Reminiscence environment friendly: Reminiscence consumption of a linked listing is environment friendly as its measurement can develop or shrink dynamically based on our necessities, which suggests efficient reminiscence utilization therefore, no reminiscence wastage.
- Ease of Insertion and Deletion: Insertion and deletion of nodes are simply carried out in a linked listing at any place.
- Implementation: For the implementation of stacks and queues and for the illustration of bushes and graphs.
- The linked listing will be expanded in fixed time.
- Reminiscence utilization: Using pointers is extra in linked lists therefore, complicated and requires extra reminiscence.
- Accessing a node: Random entry isn’t doable on account of dynamic reminiscence allocation.
- Search operation expensive: Looking for a component is dear and requires O(n) time complexity.
- Traversing in reverse order: Traversing is extra time-consuming and reverse traversing isn’t doable in singly linked lists.
Listed here are a number of the purposes of a linked listing:
- Linear information constructions similar to stack, queue, and non-linear information constructions similar to hash maps, and graphs will be carried out utilizing linked lists.
- Dynamic reminiscence allocation: We use a linked listing of free blocks.
- Implementation of graphs: Adjacency listing illustration of graphs is the most well-liked in that it makes use of linked lists to retailer adjoining vertices.
- In net browsers and editors, doubly linked lists can be utilized to construct a forwards and backward navigation button.
- A round doubly linked listing will also be used for implementing information constructions like Fibonacci heaps.
- The listing of songs within the music participant is linked to the earlier and subsequent songs.
- In an online browser, earlier and subsequent net web page URLs are linked by way of the earlier and subsequent buttons.
- Within the picture viewer, the earlier and subsequent photographs are linked with the assistance of the earlier and subsequent buttons.
- Switching between two purposes is carried out through the use of “alt+tab” in home windows and “cmd+tab” in mac guide. It requires the performance of a round linked listing.
- In cellphones, we save the contacts of individuals. The newly entered contact particulars will probably be positioned on the appropriate alphabetical order.
- This may be achieved by a linked listing to set contact on the appropriate alphabetical place.
- The modifications that we made within the paperwork are literally created as nodes in doubly linked listing. We will merely use the undo choice by urgent Ctrl+Z to switch the contents. It’s performed by the performance of a linked listing.
Often requested questions (FAQs) about Linked listing:
1. What’s linked listing information construction?
Linked listing are mostly used to deal with dynamic information components. Linked listing consists of nodes and a node consists of two fields one for storing information and different for retaining the reference of subsequent node.
2. What’s linked listing instance?
A linked listing will be assumed as a garland that’s made up of flowers. Equally, a linked listing is made up of nodes. Each flower on this explicit garland is known as a node. As well as, every node factors to the following node on this listing, and it incorporates information (on this case, the kind of flower).
3. Why do we want linked listing information construction??
There are some vital benefits to utilizing linked lists over different linear information constructions. That is not like arrays, as they’re resizable at runtime. Moreover, they are often simply inserted and deleted.
4. What are linked lists used for?
The linked listing is a linear information construction that shops information in nodes. these nodes maintain each the information and a reference to the following node within the listing. Linked are very environment friendly at including and eradicating nodes due to their easy construction.
5. What’s the distinction between array and linked listing?
There are some following variations between them:
- Arrays are information constructions containing comparable information components, whereas linked lists are non-primitive information constructions containing unordered linked components.
- In an array, components are listed, however in a linked listing nodes will not be listed.
- Accessing a component array is quick if we all know the place of a component within the array, whereas within the Linked listing it takes linear time so, the Linked listing is kind of bit slower.
- Operations like insertion and deletion in arrays take quite a lot of time. Whereas, the efficiency of those operations is quicker in Linked lists.
- Arrays are of mounted measurement and their measurement is static however Linked lists are dynamic and versatile and may broaden and shrink their measurement.
6. Why is a linked listing most well-liked over an array?
Following are the explanation that linked lists are most well-liked over array
- Nodes in a linked array, insertions, and deletions will be performed at any level within the listing at a continuing time.
- Arrays are of mounted measurement and their measurement is static however Linked lists are dynamic and versatile and may broaden and shrink their measurement.
- Linked lists present an environment friendly means of storing associated information and performing fundamental operations similar to insertion, deletion, and updating of knowledge at the price of further house required for storing the handle.
- Insertion and deletion operations within the linked listing are sooner as in comparison with the array.
7. What’s the distinction between a singly and doubly linked listing?
Following are some distinction between single and double linked listing.
Singly-linked listing (SLL) | Doubly linked listing (DLL) |
---|---|
SLL nodes incorporates 2 subject information subject and subsequent hyperlink subject. | DLL nodes incorporates 3 fields information subject, a earlier hyperlink subject and a subsequent hyperlink subject. |
In SLL, the traversal will be performed utilizing the following node hyperlink solely. Thus traversal is feasible in a single path solely. | In DLL, the traversal will be performed utilizing the earlier node hyperlink or the following node hyperlink. Thus traversal is feasible in each instructions (ahead and backward). |
The SLL occupies much less reminiscence than DLL because it has solely 2 fields. | The DLL occupies extra reminiscence than SLL because it has 3 fields. |
The Complexity of insertion and deletion at a given place is O(n). | The Complexity of insertion and deletion at a given place is O(n / 2) = O(n) as a result of traversal will be constructed from begin or from the top. |
Complexity of deletion with a given node is O(n), as a result of the earlier node must be identified, and traversal takes O(n) | Complexity of deletion with a given node is O(1) as a result of the earlier node will be accessed simply |
A singly linked listing consumes much less reminiscence as in comparison with the doubly linked listing. | The doubly linked listing consumes extra reminiscence as in comparison with the singly linked listing. |
8. Which is one of the best array or linked listing?
There are some benefits and drawbacks to each arrays and linked lists in relation to storing linear information of comparable sorts.
Benefits of linked listing over arrays:
- Dynamic measurement: Linked lists are dynamic and versatile and may broaden and shrink their measurement
- Ease of Insertion/Deletion: Insertion and deletion operations in linked listing are sooner as in comparison with the array
Disadvantages of linked listing over arrays:
- If the array is sorted we are able to apply binary search to look any factor which takes O(log(n)) time. However even when the linked listing is sorted we can’t apply binary search and the complexity of looking components within the linked listing is O(n).
- A linked listing takes extra reminiscence as in comparison with the array as a result of further reminiscence house is required for the pointer with every factor within the linked listing.
9. What are the restrictions of linked listing?
Following are some limitations of the linked listing:
- Using pointers is extra in linked lists therefore, complicated and requires extra reminiscence.
- Random entry isn’t doable on account of dynamic reminiscence allocation.
- Traversing is extra time-consuming and reverse traversing isn’t doable in singly linked lists.
- Looking for a component is dear and requires O(n) time complexity.
10. Why insertion/deletion are sooner in a linked listing?
If any factor is inserted/ deleted from the array, all the opposite components after it will likely be shifted in reminiscence this takes quite a lot of time whereas manipulation in Linked Listing is quicker as a result of we simply want to govern the addresses of nodes, so no bit shifting is required in reminiscence, and it’ll not take that a lot of time.
Conclusion
There are numerous benefits of the linked listing in comparison with array, even though they clear up the same drawback to arrays, now we have additionally mentioned the benefit, disadvantages, and its software, and we concluded the truth that we are able to use a linked listing if we want the dynamic measurement of storage and listing are good for including and eradicating gadgets shortly or for duties that require sequence however will not be appropriate for querying or search components in a big assortment of information.
So, it turns into vital that we must always at all times have in mind the constructive and destructive points of a information construction and the way they relate to the issue you are attempting to unravel.
Associated articles: