When you’re constructing a medium to large-scale SPA, likelihood is you’ll run into conditions the place you wish to higher deal with the state of your Vue elements.
In any software, a number of elements rely on the identical piece of state. Let’s think about that a number of actions from totally different elements want to mutate the identical state. To beat these challenges, Vuex helps us to keep up state throughout the applying.
On this article, I’ll information you thru implementing a Vuex module in TypeScript, then unit testing it utilizing Jest. The entire code for this tutorial is accessible on the vuex-test GitHub repository; be at liberty to fork it. Let’s get began!
What’s Vuex?
Vuex is a state administration sample and library for Vue functions that means that you can use centralized state administration in your functions, serving to you to reap the benefits of Flux-like structure. The Vuex retailer incorporates 4 core ideas:
- State
- Getters
- Mutations
- Actions
The state object incorporates the info you wish to have within the retailer, together with all of your application-level state, serving as the one supply of reality. The properties outlined within the state may be any information kind, together with a string, quantity, object, or array.
When you’d wish to have a derived state primarily based on the shop state, for instance, counting the checklist of things, filtering the gathering, or utilizing the identical set of derived state in different modules or elements, you’ll be able to outline getters.
Then again, mutations are the one means we are able to change the state. Mutations are all the time synchronous, and the payload is non-obligatory. You possibly can name a mutation through the commit, i.e., MUTATION_NAME
or payload
. It’s all the time really useful to name mutations from actions.
Actions can carry out asynchronous operations and commit the mutations. Motion handlers obtain a context object that exposes the identical set of strategies or properties on the shop occasion.
You should use context.getters
and context.state
to get the state and context.commit
to name mutations. You possibly can name motion handlers utilizing action-name
and payload
, and they’re known as from different actions throughout the retailer.
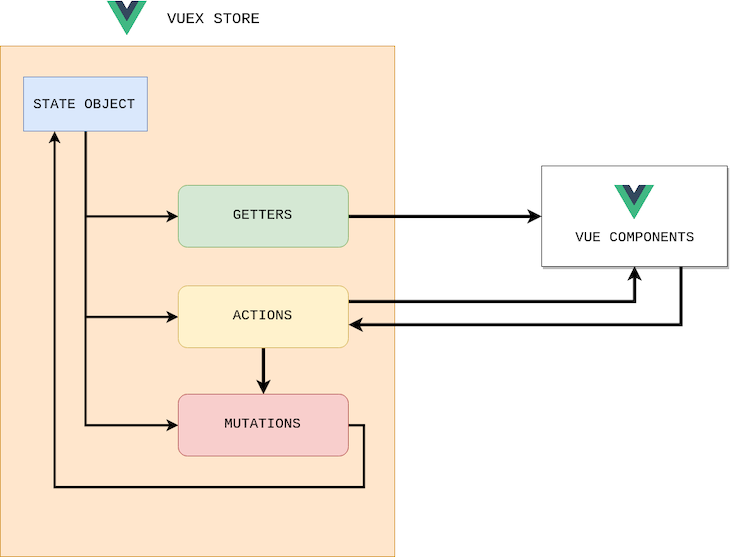
Create a Vuex module
As your software measurement will increase, your retailer can turn into bloated. To forestall this, Vuex means that you can cut up the shop into modules. Every module can comprise its personal state, getters, mutations, and actions.
Extra nice articles from LogRocket:
For instance, let’s create an software for managing a to-do checklist. First, create a brand new module for to-do operations, which is chargeable for getting all of the to-do objects and updating the state as wanted.
Our objective is to construct the module for medium to large-scale functions, due to this fact, it’s higher to separate the mutation sorts, actions known as capabilities, and the module implementation into separate recordsdata:
mutation-types.ts
: Accommodates all of the perform namesactions.ts
: Liable for all asynchronous operationsindex.ts
: The module implementation
import { IToDo } from '@/sorts/todo'; import {Module, VuexModule, Mutation, Motion} from 'vuex-module-decorators'; import TodoActions from './actions'; import * as mutationTypes from './mutation-types'; @Module({namespaced: true, identify: "Todos"}) export class ToDoModule extends VuexModule { todos:Array<IToDo> = []; loading = false; get completedTodos(){ return this.todos.filter((todo:IToDo)=> todo.accomplished); } @Mutation [mutationTypes.ON_FETCH_TODOS_STARTED]() { this.loading = true; } @Mutation [mutationTypes.ON_FETCH_TODOS_SUCCESS](information: Array<IToDo>) { this.loading = false; this.todos = information; } @Mutation [mutationTypes.ON_FETCH_TODOS_FAILED]() { this.loading = false; this.todos = []; } @Motion({rawError: true}) public async fetchTodos():Promise<void> { strive { this.context.commit(mutationTypes.ON_FETCH_TODOS_STARTED); const response: Array<IToDo> = await TodoActions.fetchTodos(); this.context.commit(mutationTypes.ON_FETCH_TODOS_SUCCESS, response); } catch (error) { this.context.commit(mutationTypes.ON_FETCH_TODOS_FAILED); } } }
The code snippet above incorporates the next implementation:
fetchTodos Motion
: Fetches the to-do objects from the REST API and commits the mutationsON_FETCH_TODOS_STARTED
mutation: Updates theloading
state attributeON_FETCH_TODOS_SUCCESS
mutation: Updates thetodos
state arrayON_FETCH_TODOS_FAILED
mutation: Resets thetodos
and updatesloading
as falsecompletedTodos
getter: Will get solely the to-do objects which might be accomplished
Initialize checks
We’ll use the Jest framework for unit testing; Jest is just a JavaScript testing framework that may be simply put in with any node-based package deal supervisor, like npm or Yarn. There are few benefits of utilizing Jest, for instance, Jest checks run in parallel, embrace built-in code protection, and assist remoted checks, mocking, and snapshot testing.
You possibly can initialize the check by making a retailer, attaching Vuex to Vue, and registering the shop. localVue
is the scoped Vue constructor that we are able to change with out affecting the worldwide Vue constructor. The code snippet under will initialize the shop:
describe('Todos Module', perform() { let retailer: any; let todosInstance: ToDoModule; beforeEach(perform() { localVue.use(Vuex); retailer = new Vuex.Retailer({}); registerStoreModules(retailer); todosInstance = getModule(ToDoModule, retailer); }); it('ought to exists', perform() { anticipate(todosInstance).toBeDefined(); }); });
Testing actions
Within the todos
module, we created the fetchTodos
motion, which fetches information from a REST API and fills the state utilizing mutations. For the reason that REST API is an exterior name, we are able to mock it utilizing a Jest perform, then validate whether or not it’s being known as and the state is being up to date:
it('fetchTodos motion ought to fill todos state', async perform() { // prepare const todosMocked = todos as Array<IToDo>; // act jest.spyOn(TodoActions, 'fetchTodos').mockImplementation( (): Promise<Array<IToDo>> => { return Promise.resolve(todosMocked); } ); await todosInstance.fetchTodos(); // assert anticipate(todosInstance.todos.size >0).toEqual(true); anticipate(TodoActions.fetchTodos).toHaveBeenCalled(); });
Testing getters
Getter capabilities merely return the state object. In our instance, now we have one getter perform, completedTodos
, which ought to return the to-do objects which might be accomplished:
it('completedTodos getter ought to return solely accomplished todos', async perform() { // prepare const completedTodos = todosInstance.completedTodos; // assert anticipate(completedTodos.each((todo:IToDo)=> todo.accomplished)).toEqual(true); });
Testing mutations
As we already know, mutations are the one method to change the state. We are able to check the ON_FETCH_TODOS_SUCCESS
mutation by sending mock to-do duties and validating whether or not the state is modified.
The code snippet under is for the success
mutation. The identical applies for the began
and error
mutations too:
it('ON_FETCH_TODOS_SUCCESS mutation ought to replace given todos', perform() { // prepare const todosTest = [ { userId: 13, id: 12, title: "Move to new city", completed: false }, { userId: 15, id: 21, title: "Finish a novel", completed: true }, ]; // act todosInstance.ON_FETCH_TODOS_SUCCESS(todosTest); // assert anticipate(todosInstance.todos.size).toEqual(2); anticipate(todosInstance.todos).toEqual(todosTest); });
Conclusion
On this tutorial, we realized about Vuex by creating and unit testing a Vuex module with TypeScript and Jest. We coated the 4 core ideas of a Vuex retailer, together with state, getters, mutations, and actions. With Vuex’s centralized state administration, you’ll be able to simplify your software and reap the benefits of Flux-like structure.
I hope you realized one thing new, and make sure you depart a remark when you’ve got any questions. Blissful coding!
Expertise your Vue apps precisely how a person does
Debugging Vue.js functions may be tough, particularly when there are dozens, if not a whole lot of mutations throughout a person session. When you’re fascinated by monitoring and monitoring Vue mutations for all your customers in manufacturing, strive LogRocket. https://logrocket.com/signup/
LogRocket is sort of a DVR for internet and cellular apps, recording actually every little thing that occurs in your Vue apps together with community requests, JavaScript errors, efficiency issues, and rather more. As a substitute of guessing why issues occur, you’ll be able to mixture and report on what state your software was in when a difficulty occurred.
The LogRocket Vuex plugin logs Vuex mutations to the LogRocket console, supplying you with context round what led to an error, and what state the applying was in when a difficulty occurred.
Modernize the way you debug your Vue apps – Begin monitoring without spending a dime.