Interface tabs are a typical web site element, however builders usually face challenges in making this sample accessible. Display screen reader customers could encounter some points studying the content material, and customers who depend on a keyboard to navigate by a web site could require some help to make navigation simpler.
This text will cowl all the things it’s good to know to create an accessible tab interface, together with constructing the HTML construction, including the CSS styling, and including JavaScript performance enhancements.
Right here’s the tabbed interface that we’ll construct on this tutorial:
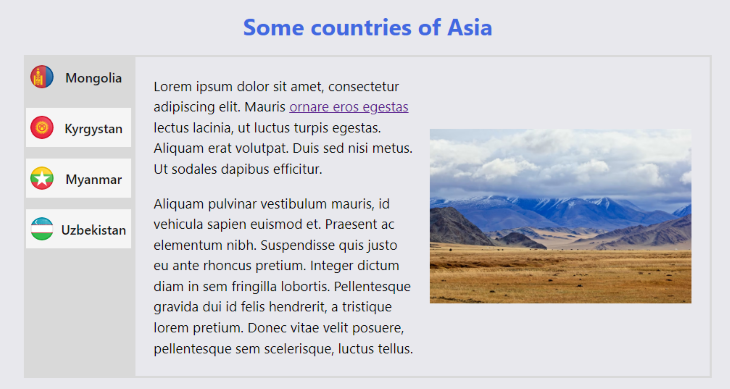
Let’s get began!
Soar forward:
Establishing the HTML construction
The HTML construction is essential as a result of it gives display screen reader customers with the context wanted to navigate the interface.
There are two ways in which we will use the HTML construction to assist outline which components might be targeted on with a keyboard. We will use a link-based strategy with the <a>
aspect as our tab set off or we will use the <button>
aspect.
Each approaches take keyboard navigation into consideration by being natively keyboard focusable, however every has benefits and downsides. We’ll cowl each approaches on this article.
Utilizing the <a>
aspect because the tab
The primary benefit of this strategy is that we will use the <a>
aspect’s performance to create the tab’s navigation impact utilizing solely HTML and CSS. The <a>
aspect brings keyboard help by caring for loads of the main target administration and navigation for display screen reader customers for us!
This strategy can be useful for customers who’ve JavaScript disabled. We’ll solely use JavaScript on the finish of this tutorial — to boost a number of the <a>
aspect’s functionalities for accessibility functions.
Extra nice articles from LogRocket:
Nonetheless, a minor drawback to utilizing the <a>
aspect because the tab is that it’s counted within the consumer’s navigation historical past. In different phrases, if the consumer visits all of the tabs after which desires to return to their earlier web site, they’ll must press the again button many occasions.
Nonetheless, this strategy does have progressive enhancement in thoughts because it gives the best tab interface performance with the bottom expertise attainable. This strategy will work even when JavaScript doesn’t load and even when CSS fails to load as effectively, so it’s price contemplating.
Utilizing the <button>
aspect because the tab
Utilizing the <button>
aspect because the tab has the benefit of not affecting navigation historical past, making it simpler for the consumer to return to their earlier web site.
Nonetheless, this strategy requires some additional concerns with HTML to cover all non-active tab content material. It additionally requires some extra choices with regard to JavaScript, akin to figuring out the way to show content material, focus administration methods, and a few accessibility enhancements.
In the event you use this strategy, you’ll want to think about what’s going to occur if JavaScript doesn’t load in your web site. With no fallback, customers will be unable to navigate your tabbed interface. There are a number of the explanation why JavaScript could fail to load. For instance, customers could have JavaScript disabled attributable to monitoring and privateness issues, or JavaScript could fail to load attributable to exterior elements.
Creating the interface tab construction
After deciding which strategy you’ll use, it’s time to begin creating the HTML construction!
Tab interface construction with the <a>
aspect strategy
Let’s begin with the person tabs. Right here, we’ll use the <a>
aspect strategy for the HTML construction:
<!-- Tab semantics --> <ul function="tablist"> <li function="presentation"> <a job="tab" href="#panel1" id="tab1">Tab one</a> </li> <li function="presentation"> <a job="tab" href="#panel2" id="tab2">Tab two</a> </li> <li function="presentation"> <a job="tab" href="#panel3" id="tab3">Tab three</a> </li> <li function="presentation"> <a job="tab" href="#panel4" id="tab4">Tab 4</a> </li> </ul> <!-- Tab content material semantics --> <div class="tabpanel-container"> <part function="tabpanel" id="panel1" aria-labelledby="tab1" tabindex="0"></part> <part function="tabpanel" id="panel2" aria-labelledby="tab2" tabindex="0"></part> <part function="tabpanel" id="panel3" aria-labelledby="tab3" tabindex="0"></part> <part function="tabpanel" id="panel4" aria-labelledby="tab4" tabindex="0"></part> </div>
Now, let’s look at the construction proven within the above code:
- On the finish of the day, a tab listing is only a listing of hyperlinks (or buttons) that can lead us to our content material, so the right semantic for the tab listing is to wrap it with our chosen aspect (
<a>
or<button>
) throughout the listing objects inside a<ul>
aspect - We add the
tablist
function to our<ul>
to offer extra context for display screen reader customers; this function is used to mark a container that wraps a set of tabs. Because thetablist
function doesn’t give the tab semantic to the kid aspect, we add thetab
function to our<a>
aspect - If a display screen reader consumer fails to learn the
tablist
andtab
roles, the HTML will nonetheless be learn as a listing of hyperlinks or buttons, which is a suitable fallback - We add the
presentation
function to the<li>
components simply to take away the semantics; this may assist forestall any bizarre interactions, whereas nonetheless sustaining our fallback in case a specific display screen reader doesn’t help ARIA roles - Our tab content material might be represented by a
<part>
aspect with thetabpanel
function. This aspect is anticipated to make use of the identical title of the tab as an accessible title. For this reason we added theid
attribute to our tabs and why we’re utilizing it as a label in our tab panels with thearia-labelledby
attribute - We add the attribute
tabindex="0"
to our tab panels to permit objects contained in the tab (like kind fields, hyperlinks, or buttons) to obtain the keyboard focus. This may make it simpler for keyboard customers to entry the content material
Tab interface construction with the <button>
aspect strategy
If we use the <button>
-based strategy, we’ll want to incorporate an additional step. We’ll want so as to add the hidden
attribute to all tab panels besides the primary tab. This attribute will conceal the weather for each sighted customers and display screen reader customers. To make sure the primary tab stays seen, it ought to have the aria-selected="true"
attribute.
Our markup for the <button>
strategy ought to look one thing like this:
<!-- Tab semantics --> <ul function="tablist"> <li function="presentation"> <button function="tab" href="#panel1" id="tab1" aria-selected="true">Tab one</button> </li> <li function="presentation"> <button function="tab" href="#panel2" id="tab2">Tab two</button> </li> <li function="presentation"> <button function="tab" href="#panel3" id="tab3">Tab three</button> </li> <li function="presentation"> <button function="tab" href="#panel4" id="tab4">Tab 4</button> </li> </ul> <!-- Tab content material semantics --> <div class="tabpanel-container"> <part function="tabpanel" id="panel1" aria-labelledby="tab1" tabindex="0"></part> <part function="tabpanel" id="panel2" aria-labelledby="tab2" tabindex="0" hidden></part> <part function="tabpanel" id="panel3" aria-labelledby="tab3" tabindex="0" hidden></part> <part function="tabpanel" id="panel4" aria-labelledby="tab4" tabindex="0" hidden></part> </div>
Extra HTML construction concerns
To this point, we’ve coated the preliminary concerns for our markup, however there are extra elements we’ll want to remember for sure use circumstances. Some elements might be managed extra dynamically, however we’ll contact on these just a little later on this article’s JavaScript part.
Relating tabs with the tab panel
We will relate tabs with the tab panel for display screen reader customers utilizing the attribute aria-controls
. Nonetheless, this strategy will solely work on the JAWS display screen reader and its use could really feel fairly verbose, as defined within the article by Heydon Pickering. As an alternative, we’ll use some focus administration methods which is able to assist keyboard customers as effectively.
Dealing with vertical tabs
To show a tab listing with a vertical orientation, you’ll want so as to add the attribute [aria-orientation="vertical"]
to the container. This may point out to display screen reader customers that the tabs are stacked vertically.
This might be essential once we begin discussing navigation methods for our pattern tabbed interface.
Right here’s an instance displaying how the [aria-orientation="vertical"]
attribute can be utilized in a code snippet:
<ul function="tablist" aria-orientation="vertical"> <li function="presentation"> <button function="tab" href="#panel1" id="tab1" aria-selected="true">Tab one</button> </li> <li function="presentation"> <button function="tab" href="#panel2" id="tab2">Tab two</button> </li> <li function="presentation"> <button function="tab" href="#panel3" id="tab3">Tab three</button> </li> <li function="presentation"> <button function="tab" href="#panel4" id="tab4">Tab 4</button> </li> </ul>
Indicating tab choice
When a consumer clicks a tab, we’ll want a technique to point out that it was chosen.
We’ll use the attribute [aria-selected="true"]
to point tab choice to display screen reader customers. Then, we’ll type it with CSS to assist point out choice for sighted customers. The [aria-selected="true"]
attribute will change dynamically with JavaScript, so it gained’t be added to our preliminary markup.
<li function="presentation"> <button function="tab" href="#panel1" id="tab1" aria-selected="true">Tab one</button> </li>
Now that we’ve got a stable HTML construction, it’s time to add some styling with CSS!
Including styling and structure with CSS
For our instance tabbed interface, we’re utilizing a link-based strategy with the <a>
aspect as our tab set off. To type the HTML tab construction, we’ll want so as to add the tab navigation, create the main target indicator, and determine the chosen state’s type.
Including the tab navigation
So as to add the tab navigation, let’s add a CSS rule utilizing the :goal
selector like so:
.tabpanel:not(:goal):not(.seen) { show: none; }
:goal
is a pseudo-class that checks if a component with an id
matches the URL’s fragment.
Since we’re utilizing the <a>
aspect right here so as to add performance, we will use this selector to cover any non-active tab panels. We’re utilizing the seen
class as an exception in our instance in order that we will add some enhancements with JavaScript later.
Creating the main target indicator
One other factor we want to remember is keyboard navigation, and one of the crucial essential issues to do in that regard is to create an enough focus indicator.
You’ve most likely seen a spotlight indicator whereas navigating a web site utilizing the tab key. Relying in your browser, will seem as a blue or black define highlighting a particular aspect.
Browsers supply default focus types, however they aren’t ample to satisfy WCAG 2.2 standards. Due to this fact, it’s vital to make use of extra styling. For extra in-depth info on the way to create an excellent focus indicator, learn Sara Souiedan’s article on this matter.
For our instance, we’ll use the next focus types:
.tab:focus-visible { background-color: royalblue; coloration: whitesmoke; define: 0.2em stable clear; }
I made a decision to make use of :focus-visible
as a substitute of the :focus
pseudo-class as a way to have the main target type activate solely with keyboard navigation. The :focus
pseudo-class may even activate when the aspect is clicked, which might be complicated for customers.
:focus-visible
could be very effectively supported in trendy browsers, so it mustn’t create any type of battle. For extra details about this property, discuss with the MDN documentation.
The “Mongolia” choice chosen beneath exhibits how our tab’s focus state would look:
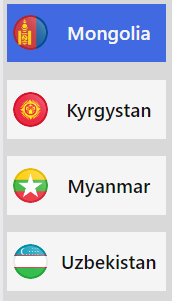
For this venture, I made a decision to make use of a clear define. That is essential for Home windows excessive distinction mode. Beneath this mode, all textual content and background colours are changed with these chosen by the working system, so we will’t rely upon the background coloration to point a spotlight state. The define is the one dependable technique to point out the main target state.
Figuring out the chosen state’s type
Now, let’s come again to what I discussed earlier relating to utilizing the aria-selected="true"
attribute to assist display screen reader customers extra simply determine the chosen tab. We will use this selector to offer a visible cue for the chosen tab as effectively!
.tab[aria-selected="true"] { background-color: var(--component-bg); }
This strategy creates a minor drawback due to how CSS specificity works. Each selectors, .tab:focus-visible
and .tab[aria-selected="true"]
, have the identical specificity degree, and each change the background-color
property, so the selector rule order is essential.
We would like the main target state’s background-color
to overwrite every other background-color
, together with that of the chosen state, so we’ll add the .tab:focus-visible
selector rule after the .tab[aria-selected="true"]
selector rule.
Right here’s our consequence; discover how each types work together with one another with none specificity drawback:
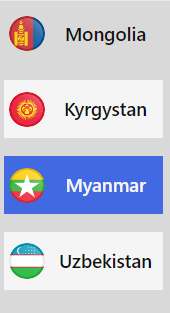
In the event you’re utilizing the <a>
aspect as your tab, you’ve already created a useful tab interface with the bottom expertise attainable!
In the event you used the <button>
-based strategy, don’t fear! Subsequent, we’re going so as to add some performance with JavaScript, which might be essential for making the tabbed interface extra accessible.
Including enhancements and performance with JavaScript
There’s a lot to unpack on this part; let’s begin by trying out what the ARIA Authoring Practices Information (APG) has to say about this element sample. Right here’s what the ARIA APG’s sample information for tab interface considers essential for the tab key, the tabindex
in tabpanel
components, and the arrow keys.
Managing the tab key
Concerning the usage of the tab key, the ARIA APG suggests the next:
When focus strikes into the tab listing, place deal with the lively
tab
aspect.
Our first activity is to take away the keyboard navigation with the tab key to the non-selected tab in order that when the secret’s pressed, the main target goes straight into the lively tab panel. Then, when shift + tab is pressed from the lively panel, the main target goes again to its respective tab. Additionally, we’ll have to point tab choice for each display screen reader customers and sighted customers.
Right here’s the code I used to handle these duties:
const TABLIST = doc.querySelector("#tablist"); const TABS = [...TABLIST.querySelectorAll(".tab")]; const setSelectedTab = (aspect) => { const selectedId = aspect.id; TABS.forEach((e) => { const id = e.getAttribute("id"); if (id === selectedId) { e.removeAttribute("tabindex"); e.setAttribute("aria-selected", "true"); } else { e.setAttribute("tabindex", "-1"); e.setAttribute("aria-selected", "false"); } }); };
Right here, we choose all tabs and retailer the one that’s being clicked. Then, we parse the array with all our tabs and examine every id
attribute to test if it’s the tab that was chosen.
If the tab isn’t the one we want to choose, we add the tabindex="-1"
and aria-selected="false"
attributes. Nonetheless, if the tab is the one we want to choose, we take away the tabindex
attribute and add the aria-selected="true"
attribute. The <a>
and <button>
components are keyboard focusable by default, so there’s no want so as to add a tabindex="0"
.
The tabindex="-1"
attribute will make a component not focusable with the tab key. When the secret’s pressed, it can transfer the main target on to our lively tab panel, enabling us to leap all our tabs. That is additionally essential as a result of it’ll additionally enable us to handle the main target of the tabs in one other that I’ll show just a little later on this article.
Now all of us have to do is add occasion listeners to our tabs to execute this operate! For a link-based strategy, we’ll want so as to add a further occasion listener to these components.
With a <button>
-based strategy, any click on occasion might be activated by the enter and house keys. Nonetheless, an <a>
aspect will solely add these occasions with the enter key, so we’ll want so as to add a keydown occasion listener to test when the house secret’s pressed.
const handleClick = () => { TABS.forEach((aspect) => { aspect.addEventListener("click on", operate () { setSelectedTab(aspect); }); }); // Prompts tab with Area. Solely vital in the event you use the <a> aspect as a tab TABS.forEach((aspect) => { aspect.addEventListener("keydown", operate (e) { if ((e.keyCode || e.which) === 32) { setSelectedTab(aspect); aspect.click on(); } }); }); };
Right here’s our consequence!
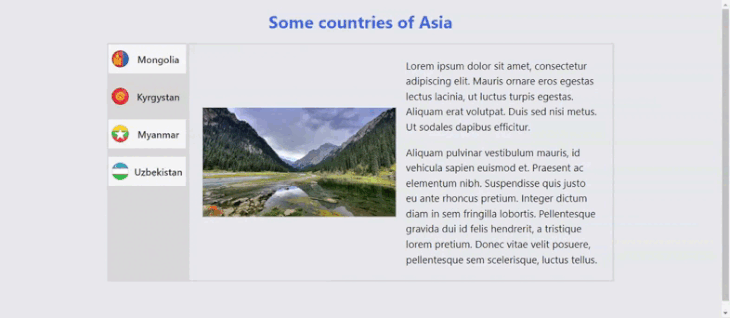
Managing the tabindex
in tabpanel
components
The ARIA APG recommends the next for the tab listing keyboard interplay:
When the tab listing comprises the main target, [tab] strikes focus to the subsequent aspect within the web page tab sequence exterior the tablist, which is the tabpanel until the primary aspect containing significant content material contained in the tabpanel is focusable.
Based mostly on this advice, our subsequent activity is to test if every tab panel comprises a focusable aspect.
For tab panels that don’t include a focusable aspect, we’ll retain the tabindex="0"
attribute we added beforehand. In any other case, we’ll replace the attribute to tabindex="-1"
, so when the tab secret’s pressed, the main target might be moved to the primary focusable aspect contained in the tab panel.
We’ll use this code snippet to test if each tab panel comprises focusable components and alter the tabindex
attribute as wanted:
const TABPANELS = [...document.querySelectorAll(".tabpanel")]; const determineTabindex = () => { TABPANELS.forEach((aspect) => { const focusableElements = aspect.querySelectorAll( 'button:not([disabled]), [href], enter:not([disabled]), choose:not([disabled]), textarea:not([disabled]), [tabindex]:not([tabindex="-1"]):not([disabled]), particulars:not([disabled]), abstract:not(:disabled)' ).size; focusableElements ? aspect.setAttribute("tabindex", "-1") : aspect.setAttribute("tabindex", "0"); }); };
Right here’s our consequence!
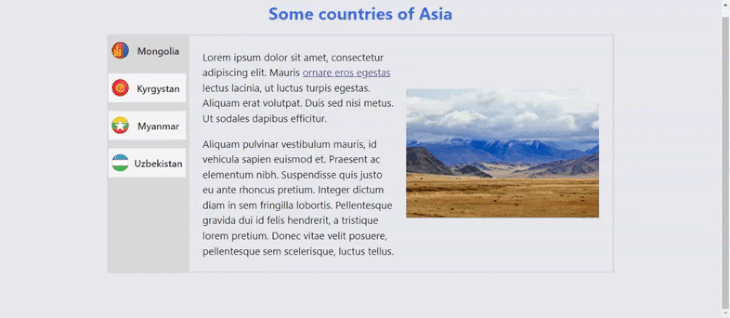
On this instance, once we attempt to use our keyboard to navigate the interface, the targeted aspect will fluctuate relying on whether or not the tab panel comprises a keyboard-focusable aspect.
Managing navigation with the arrow keys
The ARIA APG gives particular keyboard navigation suggestions relying on the orientation of the interface’s tabs.
Listed here are the suggestions when focus is on a tab aspect in a horizontal tab listing:
left arrow: Strikes focus to the earlier tab. If focus is on the primary tab, transfer focus to the final tab. Optionally, prompts the newly targeted tab.
proper arrow: Strikes focus to the subsequent tab. If focus is on the final tab aspect, transfer focus to the primary tab. Optionally, prompts the newly targeted tab.
In the event you’re constructing an interface with tabs displayed vertically, apart from utilizing the attribute aria-orientation="vertical"
you’ll have to make use of the up arrow and down arrow keys as a substitute of the left arrow and proper arrow keys.
Listed here are the suggestions when a tab listing has aria-orientation set to vertical
:
When a tab listing has its aria-orientation set to
vertical
:
down arrow performs as proper arrow is described above.
up arrow performs as left arrow is described above.
My intention for this venture was to construct a very versatile interface, however with this strategy in some unspecified time in the future the display screen will find yourself being both a horizontal tab listing or a vertical tab listing. How will we tackle this?
Let’s begin by figuring out what arrow keys we must always use. On this explicit case, we’ve got two options:
- Test the tab listing width and peak. If the peak is larger than the width, add the attribute
aria-orientation="vertical"
and help solely up arrow and down arrow navigation. In any other case, add the attributearia-orientation="horizontal"
and help solely left arrow and proper arrow navigation. - Help all 4 navigation keys on the identical time. If the consumer presses the down arrow or proper arrow, it can transfer the main target to the subsequent tab. In the event that they press the left arrow or up arrow, it can transfer the main target to the earlier tab.
I often favor the best resolution, so I’ll select the second choice. Nonetheless, every venture is totally different. In case your tab listing will all the time be in a vertical place, you‘d be higher off utilizing aria-orientation="vertical"
and supporting solely up arrow and down arrow navigation.
Right here’s the code I used, based mostly on the second choice:
const createArrowNavigation = () => { const firstTab = TABS[0]; const lastTab = TABS[TABS.length - 1]; TABS.forEach((aspect) => { aspect.addEventListener("keydown", operate (e) { if ((e.keyCode || e.which) === 38 || (e.keyCode || e.which) === 37) { if (aspect == firstTab) { e.preventDefault(); lastTab.focus(); } else { e.preventDefault(); const focusableElement = TABS.indexOf(aspect) - 1; TABS[focusableElement].focus(); } } else if ( (e.keyCode || e.which) === 40 || (e.keyCode || e.which) === 39 ) { if (aspect == lastTab) { e.preventDefault(); firstTab.focus(); } else { e.preventDefault(); const focusableElement = TABS.indexOf(aspect) + 1; TABS[focusableElement].focus(); } } }); }); };
Beforehand, we created a variable that shops all our tabs. We will use it to find out which is the primary and final navigation tab. That is essential as a result of these tabs have particular habits.
Subsequent, we will test which key codes are associated to every arrow key. For that, we will use the positioning keycode.information to test it out. To ease the method, right here’s the listing of the important thing codes:
Key | Keycode |
---|---|
Left Arrow | 37 |
Up Arrow | 38 |
Proper Arrow | 39 |
Down Arrow | 40 |
Now, we hear to every key to test the place of the targeted tab within the array and we deal with the subsequent aspect (the proper arrow and down arrow) or earlier aspect (the left arrow and up arrow) within the array with the focus()
technique. Since these components have the tabindex="-1"
attribute, we’ll have the ability to focus them with this technique.
Remember the fact that if the aspect is the primary one on the array, it can transfer the main target to the final aspect and vice versa. One other level to think about is that the arrow keys even have the operate of shifting the scrollbar; we will forestall this habits with the e.preventDefault()
technique.
Right here’s our interface, displaying the usage of arrow keys to maneuver between tabs:
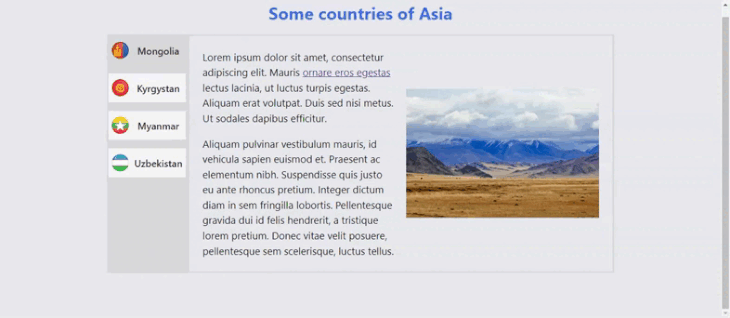
Figuring out the way to present the content material
Along with managing the targeted state of our element, we have to decide the timing for activating the newly targeted tab. In different phrases, when ought to the tab panel be proven? Ought to it’s proven when the tab is clicked or when focus is delivered to the chosen tab?
The reply to this matter is surprisingly nuanced, and W3C has a whole part relating to this matter. To summarize W3C, altering the displayed content material in the meanwhile of focusing a component (known as comply with focus) might be helpful for sure elements however creates accessibility errors for others.
Displaying content material at time of click on
Displaying content material on the time of focus could make keyboard navigation simpler for sighted customers, however display screen reader customers will not be conscious that new content material has been added to the web page. Moreover, we have to contemplate the quantity of content material that might be proven as this might impression efficiency.
I recommend displaying the content material when the tab is clicked. This selection might require an additional key press for keyboard customers, however it can present a extra inclusive expertise for display screen reader customers.
When the <a>
aspect is clicked, the main target is delivered to the chosen tab panel, which is able to cowl navigation for display screen reader customers.
Displaying content material at time of focus
In the event you determine to show the content material based mostly on focus, remember to use the <button>
strategy, somewhat than the <a>
aspect strategy.
With a <button>
aspect, you’ll have to deal with the main target administration with JavaScript. For that, we’ll come again to the handleClick
operate we created earlier within the CSS part of this text, and we’ll add some changes.
First, we’ll create a operate that provides or removes the attribute hidden
relying on whether or not the tab panel is being focused. If the tab panel is the focused merchandise, we’ll additionally transfer the keyboard focus to it. Right here’s my strategy to this situation:
const showActivePanel = (aspect) => { const selectedId = aspect.id; TABPANELS.forEach((e) => { e.hidden = "true"; }); const activePanel = doc.querySelector( `[aria-labelledby="${selectedId}"]` ); activePanel.removeAttribute("hidden"); activePanel.focus() };
What we‘re doing right here is hiding all tab panels by including to them the hidden
attribute. Subsequent, we’ll choose our focused tab panel through the use of the aria-labelledby
attribute’s worth. Keep in mind, every button
has an id
that we’re utilizing to label the tab panel through the use of this attribute; this may assist us choose the right tab panel.
Now, we simply take away the hidden
attribute after which convey the keyboard focus to it with the focus()
technique. Lastly, we add the showActivePanel
operate in our handleClick
operate to be executed on the press.
Now it’s time to see how the element works as a complete!
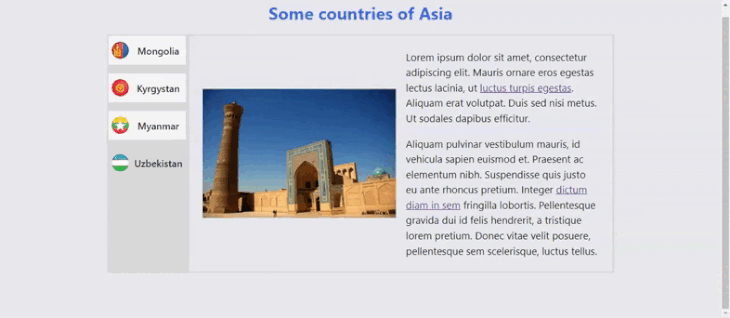
Dealing with the <a>
aspect’s preliminary state
If we choose to make use of an <a>
aspect for our tabs, we’ll have to deal with the aspect’s preliminary state if JavaScript is lively.
Listed here are the actions we need to take when the doc hundreds:
- If the positioning’s URL isn’t pointing to the tab panels, the primary panel ought to be seen, the primary tab ought to have the attribute
aria-selected="true"
and be keyboard focusable when the tab secret’s pressed; the opposite tabs ought to havearia-selected="false"
andtabindex="-1"
attributes - If the positioning’s URL is pointing to a panel, the panel on this URL ought to be seen; the respective tab ought to have the beforehand talked about attributes:
aria-selected="true"
andno tabindex
We’ll create the features for each eventualities.
const activateFirstPanel = () => { TABS[0].setAttribute("tabindex", "0"); TABS[0].setAttribute("aria-selected", "true"); TABPANELS[0].classList.add("seen"); };
This operate will cowl our first case. It’s truly fairly easy. We now have the TABS
and TABPANELS
node lists already, we will choose the primary aspect of every listing and add the required properties.
Within the earlier CSS part, I discussed that we had been utilizing the seen
class as an exception and would add some enhancements with JavaScript later. Nicely, now it’s the second for the JavaScript enhancements!
We add this class to the primary tab panel to make it seen. As soon as the consumer begins interacting with the tab, we’ll have to take away this class.
const checkInitialSelectedTab = () => { const targetedTabPanel = doc .querySelector(".tabpanel:goal") .getAttribute("aria-labelledby"); const selectedTab = doc.querySelector(`#${targetedTabPanel}`); selectedTab.setAttribute("aria-selected", "true"); selectedTab.removeAttribute("tabindex"); };
Our second situation is a little more sophisticated. Since this panel is being focused, it is going to be displayed on the display screen by default — that is as a result of selector.tabpanel:not(:goal):not(.seen)
we used beforehand. However, we have to search for the tab that prompts the panel.
For this, we’ll choose the focused tab panel with JavaScript through the use of the .tabpanel:goal
selector within the querySelector()
technique. As soon as we’ve got that node, we’ll get the aria-labelledby
attribute. The tab’s id
is identical because the one which we’re utilizing on this panel’s aria-labelledby
attribute, so we’ll use that id
to search for the tab, after which we’ll add the required attributes.
Now, we simply have to execute these features relying on what the URL comprises, so we’ll use one other operate to deal with this:
const handleInitialState = () => { TABS.forEach((e) => { e.setAttribute("tabindex", "-1"); e.setAttribute("aria-selected", "false"); }); window.location.href.indexOf("#panel") === -1 ? activateFirstPanel() : checkInitialSelectedTab(); determineTabindex(); };
Nicely, I lied. There’s just a bit bit extra work that we have to do on this operate.
For starters, let’s set the aria-selected
attribute of all tabs to false
and the tabindex
attribute to -1
; then we’ll repair the right one with the created features.
Subsequent, we have to test if a tab panel is being focused within the web site’s URL. We will use the window.location.href
technique to get the URL after which use the array indexOf
technique to test if there’s a panel focused on this URL.
While you use an array operate in a string, akin to our URL, it can create an array with every character within the string as a component of this array, if the entire world is on this URL, it’ll return the beginning place of the string, if not, it’ll return -1. That is what we’ll use to find out what operate to execute.
Now, we’ll execute the determineTabindex()
operate we beforehand created. We’ll take away the no-js
class that we added in our HTML and used to create a fallback in case JavaScript doesn’t load.
We’re virtually executed!
We beforehand created a operate to test if the tab panels have focusable components: determineTabIndex()
; that operate must be added there.
Do you bear in mind our setSelectedTab()
operate? We have to add a small line to take away the seen
class in case it was used. To take action, we’ll add the code TABPANELS[0].classList.take away("seen");
earlier than we begin checking every tab.
Lastly, let’s use the next command to ensure our operate handleInitialState()
runs when the positioning hundreds:
window.onload = handleInitialState;
Keep in mind, this part solely applies in the event you used the <a>
aspect to deal with the tabs’ navigation.
Wrapping up
Beneath is our accomplished accessible tabbed interface! After contemplating a number of choices, I used the link-based strategy with the <a>
aspect for this demo. The tabbed UI was designed with a very versatile structure without having for media queries.
See the Pen
Accessible tabbed interface by Cristian Diaz (@ItsCrisDiaz)
on CodePen.
This element addresses the principle accessibility points that builders encounter when constructing a tabbed interface: guaranteeing that it really works correctly for keyboard customers and that the knowledge is proven accurately to display screen reader customers.
Constructing an accessible element might be difficult as a result of a number of nuances of accessibility necessities, but it surely’s essential to make sure your web site is usable for everybody.
LogRocket: Debug JavaScript errors extra simply by understanding the context
Debugging code is all the time a tedious activity. However the extra you perceive your errors the better it’s to repair them.
LogRocket lets you perceive these errors in new and distinctive methods. Our frontend monitoring resolution tracks consumer engagement together with your JavaScript frontends to provide the skill to seek out out precisely what the consumer did that led to an error.
LogRocket data console logs, web page load occasions, stacktraces, sluggish community requests/responses with headers + our bodies, browser metadata, and customized logs. Understanding the impression of your JavaScript code won’t ever be simpler!