Information Binding, because the identify itself, is a self-explanatory phrase. In information binding what we’ve got to do is we’ve got to seize or retailer the information in order that we are able to bind that information with one other useful resource (for instance displaying the information within the frontend half) as per our wants or we are able to additionally learn the information from a variable and show it as per our necessities. For instance, there’s a google kind, and the person enters all the small print in that kind and we’ve got to seize/retailer the information and bind it with the fronted half as per our necessities. In Spring utilizing the information binding idea, we are able to do the next two duties
- We are able to learn from a variable
- We are able to write to a variable
So the two-way information binding means we are able to carry out each learn and write operations. In our earlier article Information Binding in Spring MVC with Instance, we’ve got mentioned how one can write-to-variable activity and on this article, we primarily concentrate on the read-from-a-variable activity.
Instance Challenge
Setup the Challenge
We’re going to use Spring Device Suite 4 IDE for this mission. Please seek advice from this text to put in STS in your native machine Find out how to Obtain and Set up Spring Device Suite (Spring Instruments 4 for Eclipse) IDE? Go to your STS IDE then create a brand new maven mission, File > New > Maven Challenge, and select the next archetype as proven within the beneath picture as follows:
Add the next maven dependencies and plugin to your pom.xml file.
<!-- https://mvnrepository.com/artifact/org.springframework/spring-webmvc --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-webmvc</artifactId> <model>5.3.18</model> </dependency> <!-- https://mvnrepository.com/artifact/javax.servlet/javax.servlet-api --> <dependency> <groupId>javax.servlet</groupId> <artifactId>javax.servlet-api</artifactId> <model>4.0.1</model> <scope>supplied</scope> </dependency> <!-- plugin --> <construct> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-war-plugin</artifactId> <model>2.6</model> <configuration> <failOnMissingWebXml>false</failOnMissingWebXml> </configuration> </plugin> </plugins> </construct>
Under is the entire code for the pom.xml file after including these dependencies.
File: pom.xml
XML
|
Configuring Dispatcher Servlet
Earlier than shifting into the coding half let’s take a look on the file construction within the beneath picture.
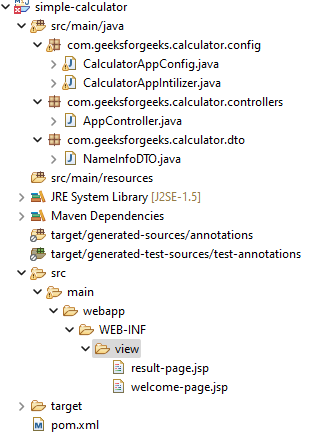
So at first create an src/primary/java folder and inside this folder create a category named CalculatorAppIntilizer and put it contained in the com.geeksforgeeks.calculator.config bundle and extends the AbstractAnnotationConfigDispatcherServletInitializer class. Discuss with the beneath picture.
And each time you might be extending this class, it has some pre summary strategies that we have to present the implementation. Now inside this class, we’ve got to only write two strains of code to Configure the Dispatcher Servlet. Earlier than that, we’ve got to create one other class for the Spring configuration file. So, go to the src/primary/java folder and inside this folder create a category named CalculatorAppConfig and put it contained in the com.geeksforgeeks.calculator.config bundle. Under is the code for the CalculatorAppConfig.java file.
File: CalculatorAppConfig.java
Java
|
And beneath is the entire code for the CalculatorAppIntilizer.java file. Feedback are added contained in the code to know the code in additional element.
File: CalculatorAppIntilizer.java
Java
|
Setup ViewResolver
Spring MVC is a Internet MVC Framework for constructing net functions. In generic all MVC frameworks present a method of working with views. Spring does that by way of the ViewResolvers, which lets you render fashions within the browser with out tying the implementation to particular view know-how. Learn extra right here: ViewResolver in Spring MVC. So for establishing ViewResolver go to the CalculatorAppConfig.java file and write down the code as follows
@Bean public InternalResourceViewResolver viewResolver() { InternalResourceViewResolver viewResolver = new InternalResourceViewResolver(); viewResolver.setPrefix("/WEB-INF/view/"); viewResolver.setSuffix(".jsp"); return viewResolver; }
And beneath is the up to date code for the CalculatorAppConfig.java file after writing the code for establishing the ViewResolver.
File: Up to date CalculatorAppConfig.java
Java
|
Two-Method Information Binding (read-from-a-variable)
At first, we’ve got to create a DTO class. So go to the src/primary/java folder and inside this folder create a category named NameInfoDTO and put it contained in the com.geeksforgeeks.calculator.dto bundle. Under is the code for the NameInfoDTO.java file. Feedback are added contained in the code to know the code in additional element.
File: NameInfoDTO.java
Java
|
Create Controller
Go to the src/primary/java folder and inside this folder create a category named AppController and put it contained in the com.geeksforgeeks.calculator.controllers bundle. Under is the code for the AppController.java file.
File: AppController.java file
Java
|
So right here on this file we’ve got to put in writing the code for studying the present property by fetching it from the DTO. And we are able to write the code one thing like this
@RequestMapping("/dwelling") public String showHomePage(Mannequin mannequin) { // Learn the present property by // fetching it from the DTO NameInfoDTO nameInfoDTO = new NameInfoDTO(); mannequin.addAttribute("nameInfo", nameInfoDTO); return "welcome-page"; }
Associated Article Hyperlink: Find out how to Create Your First Mannequin in Spring MVC
So beneath is the up to date code for the AppController.java file.
File: Up to date AppController.java file
Java
|
Create View
Now we’ve got to create a view named “welcome-page” contained in the WEB-INF/view folder with the .jsp extension. So go to the src > primary > webapp > WEB-INF and create a folder view and inside that folder create a jsp file named welcome-page. So beneath is the code for the welcome-page.jsp file. On this file, we’ve got used the idea of Spring MVC Kind Tag Library, so you could seek advice from the article to know the code.
File: welcome-page.jsp
HTML
|
So now we’ve got completed with the coding half. Let’s run and take a look at our utility.
Run Your Utility
To run our Spring MVC Utility right-click in your mission > Run As > Run on Server. And run your utility as proven within the beneath picture as depicted beneath as follows:
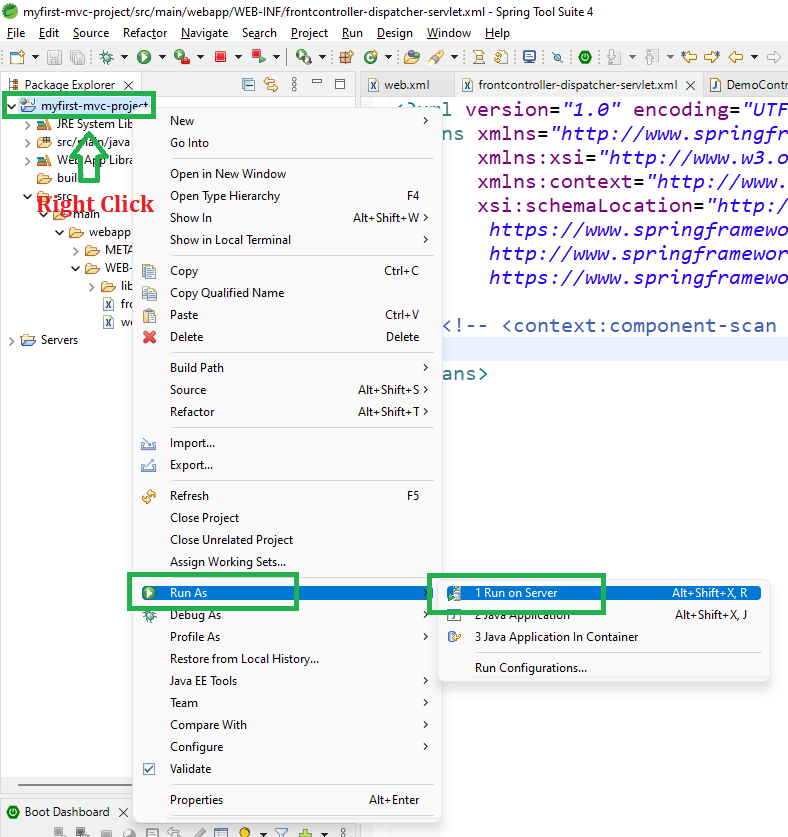
After that use the next URL to run your controller
http://localhost:8080/simple-calculator/geeksforgeeks.org/dwelling
Output:

So within the output, you possibly can see everytime you hit the URL the values are already current which suggests spring efficiently learn the values from the variable.