On this article, We’re going to discover ways to set up and use wealthy packages in Python.
RIch is a python bundle for creating some superior terminal formatting and logging. It has a number of options and features that may make your utility look nicer and even add a brand new look to your CLI utility. We can be understanding the method of putting in and fundamental utilization of the RICH bundle in Python on this article.
Putting in Wealthy
Wealthy is a python bundle, so we will set up it with the pip command, you may instantly use the pip set up command to put in wealthy however in the event you don’t need to mess up your world python surroundings, you should use a virtualenv to check out and play with a bundle in isolation. So, we’ll first arrange a digital surroundings with the virtuelenv bundle
Establishing a digital surroundings
To arrange a digital surroundings comply with the process defined within the article Create digital surroundings utilizing venv.
To arrange a recent digital surroundings, you want the virtualenv bundle, so set up the bundle globally with the command :
pip set up virtualenv
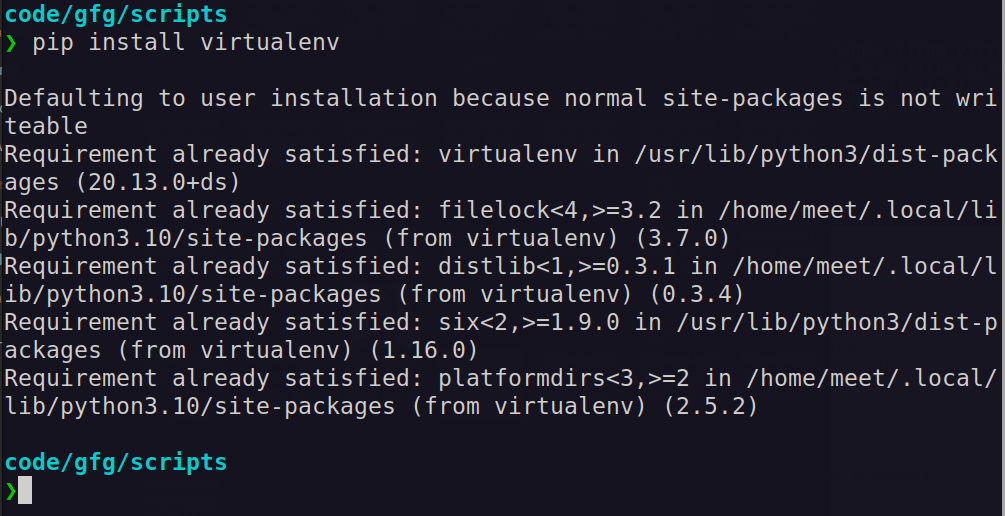
It will set up virtualenv in your world python surroundings, so we will now create a digital surroundings wherever on our system. To create a digital surroundings, we’ll use the virtualenv command adopted by the title of the digital surroundings. Be sure that to be within the desired location in your system, the beneath command will create a listing within the present location.
virtualenv venv
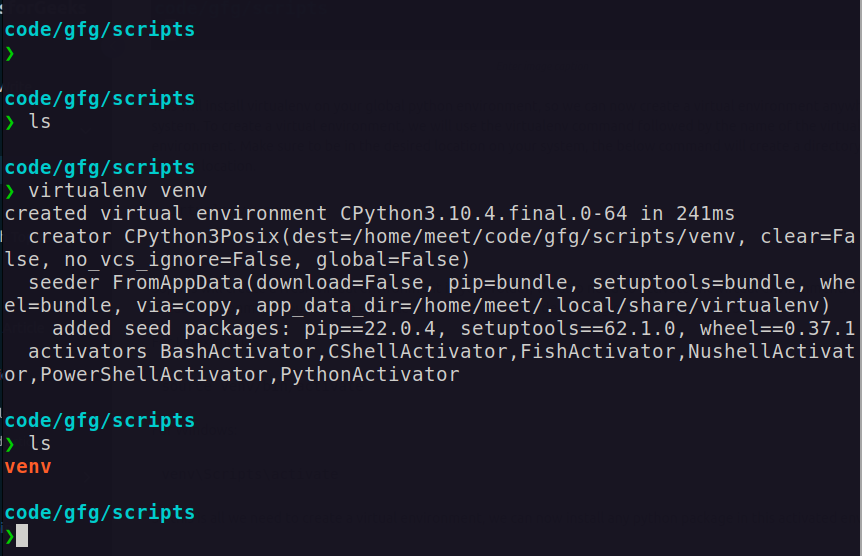
So, this can create a recent digital surroundings in your folder, we additionally have to activate the digital surroundings. To activate a digital surroundings you’ll want to execute a file referred to as activate relying in your Operation System.
For Linux/macOS:
supply venv/bin/activate
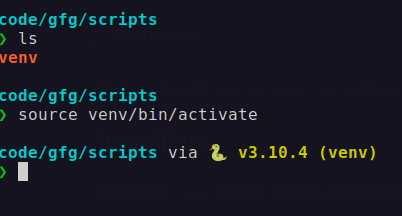
So that is all we have to create a digital surroundings, we will now set up any python bundle on this activated surroundings.
Utilizing pip to put in wealthy
We are able to now safely set up any packages in our activated digital surroundings, to put in wealthy we have to run the next command:
pip set up wealthy
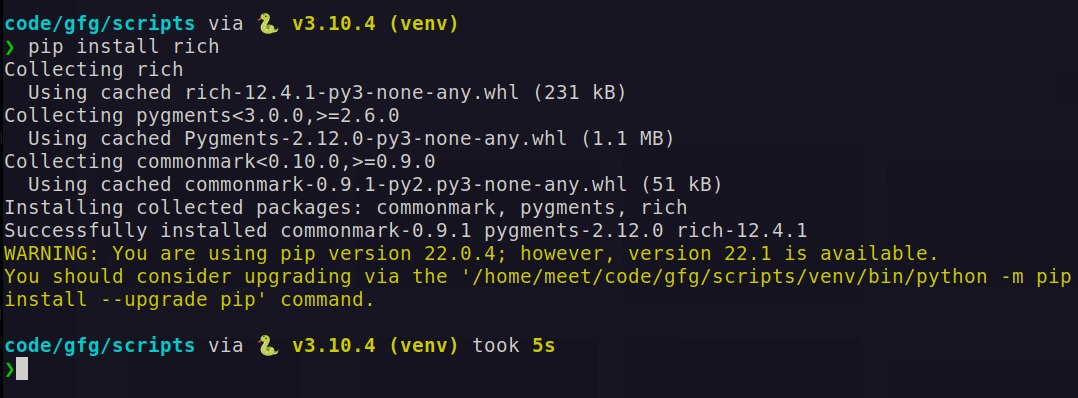
It will set up wealthy which is a python bundle. To confirm the set up has been profitable, you may run the next command with the assistance of the python -m command adopted by the bundle title on this case it’s Wealthy.
python -m wealthy
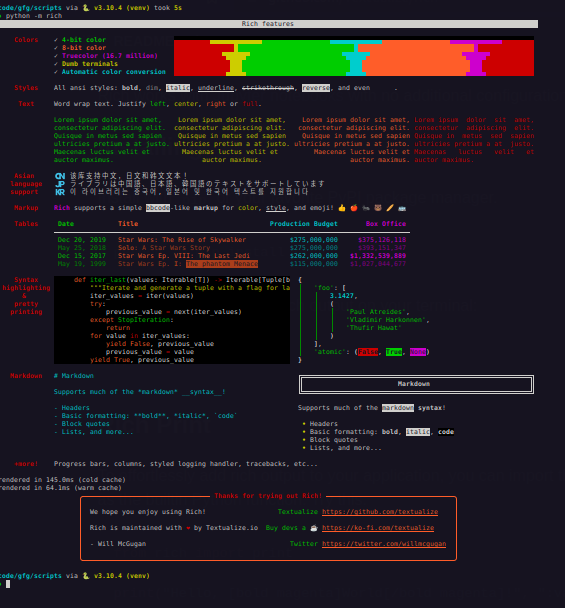
Making a Python Script to exhibit Wealthy
Now, since we’ve got the wealthy bundle put in within the digital surroundings, we will use the wealthy bundle in a python script and leverage its formatting and logging types within the terminal I/O. We’ll first create a howdy phrase script in python and exhibit a couple of different features and operators supplied by the wealthy bundle.
Python3
|
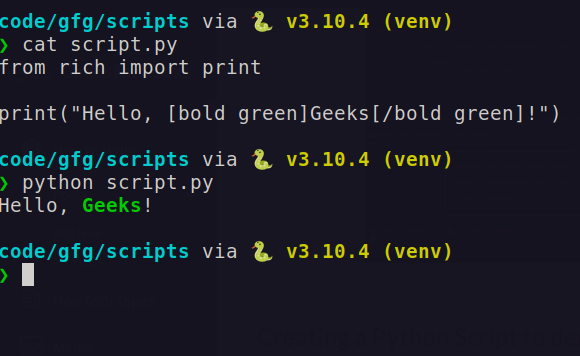
Right here, we’re importing a operate referred to as print from the wealthy bundle which we simply put in. The print operate is totally different from the common print operate supplied by python. Although it’s technically serving the identical objective it could do much more than a traditional print operate. Within the above instance, the print operate has a couple of issues written that are interpreted by python as a formatting fashion just like the [bold green] and [/bold green].
These two tags merely characterize the beginning and finish of the styling supplied within the sq. brackets. The types supplied on this instance are daring and inexperienced. You may select a couple of choices for the ornament of the textual content as supplied beneath:
The colours will be chosen from the checklist supplied within the docs with this hyperlink, the colours are 256 Commonplace colours together with a CSS-styled shade format. The ornament like daring, italics, underlined, strike, reverse, and so forth. will be supplied within the checklist from the documentation.
Creating an informative duties show
Python3
|
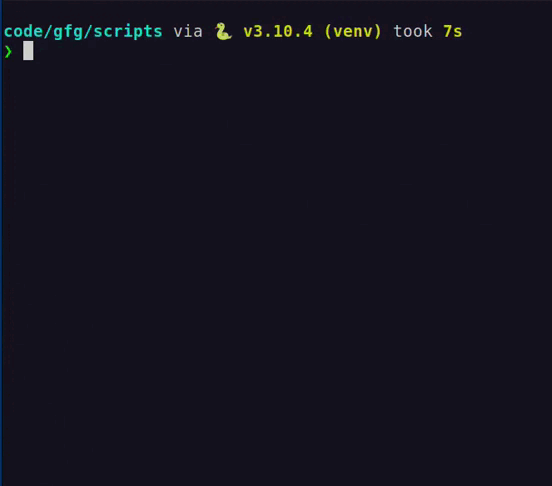
The above instance exhibits the method of displaying numerous duties in a loop. That is completed with the Console Wrapper API. The Console class offers some prolonged functionalities particularly for terminal content material. So, we instantiate a Console object and use the log technique to show the output. The standing technique is used for displaying the continued course of whereas being within the loop.
For extra choices and features within the Console class, you may discuss with the documentation of the Wealthy library.
Tables in Terminal with Wealthy
We are able to even show tables in a wealthy format with the wealthy bundle. The wealthy bundle has a solution to characterize tabular knowledge within the terminal with the Tables class. We are able to assemble a couple of pattern tables to know the basics of rendering the tabular knowledge to the console.
Python3
|
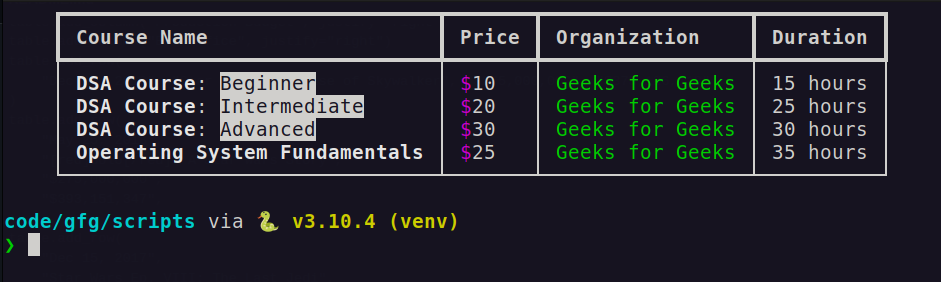
So, right here we create a desk object with preliminary changes like footer and alignment. The info is first saved as a python checklist which suggests you may add or edit the info inside itself. Through the use of the operate Dwell, we will create a desk on runtime by dynamically including columns and row values with the add_column and add_row features within the desk class.
The rows or the precise knowledge are added after inserting the columns into the info, by iterating over the beforehand outlined values from the checklist TABLE_DATA, we will parse all the row knowledge column-wise. The Dwell show operate is used for displaying the real-time development of the terminal logs and helps in visualizing the script.
We are able to even use the choice of the console to easily print the desk as an alternative of rendering the desk reside by the console.log operate.
console.print(desk)
Rendering Markdown file
We are able to even render markdown content material within the terminal with a easy markdown extension within the wealthy library in python. Right here we can be utilizing a easy markdown file to exhibit the working of the wealthy markdown rendering. The supply code pattern is supplied within the documentation and right here we have to make a couple of adjustments to make it work.
Python3
|
A pattern markdown file has been supplied beneath:
pattern.md
# Pattern markdown file Textual content with some paragraph 2nd paragraph. *Italic*, **daring**, and `monospace`. Itemized lists seem like: * this one * that one * the opposite one > Block quotes ------------ This is a numbered checklist: 1. first merchandise 2. second merchandise 3. third merchandise ```python n = 5 a = "GFG" for i in vary(0, 3): print("One thing") ``` ### An h3 header ### Tables can seem like this: measurement materials shade ---- ------------ ------------ 9 leather-based brown 10 hemp canvas pure 11 glass clear
Output:
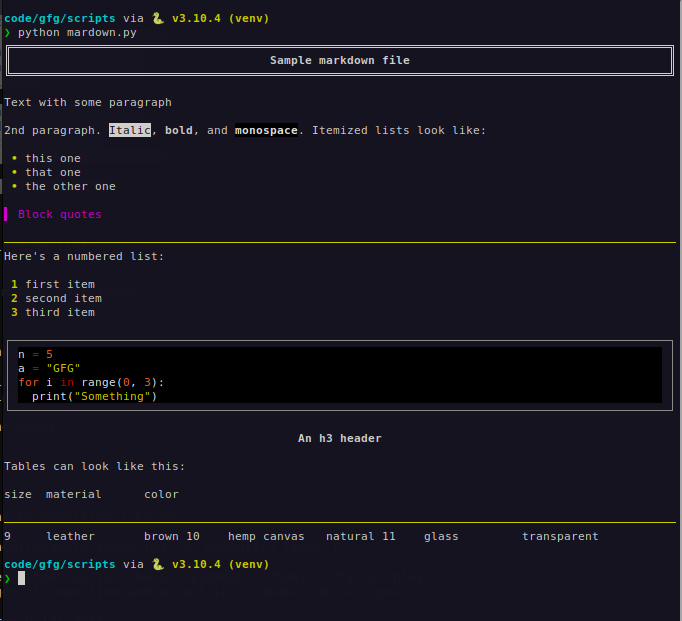
Right here, we’ll instantiate the console class for the reason that output can be on the terminal/console. Thereafter we will open the markdown file within the native repository and parse it within the Markdown operate that may render the contents and after studying the content material of the file we will print the rendered content material within the console.
Bushes in Wealthy
We are able to additionally create a tree-like construction within the terminal utilizing Wealthy. There’s a tree class in Wealthy for displaying hierarchical buildings within the terminal, we will create a easy tree occasion utilizing the wealthy tree class.
Python3
|
Output:
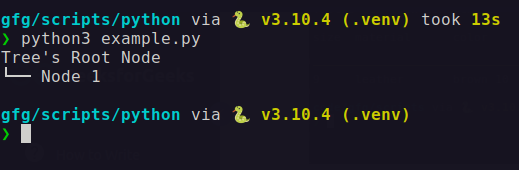
The tree class is instantiated with the Tree technique imported from the wealthy.tree module. This creates a easy root node within the tree, additional, we will add the node into the tree through the use of the add operate related to the tree. Utilizing this straightforward code, we will create extra styled bushes through the use of the console class in wealthy.
Python3
|
Output:
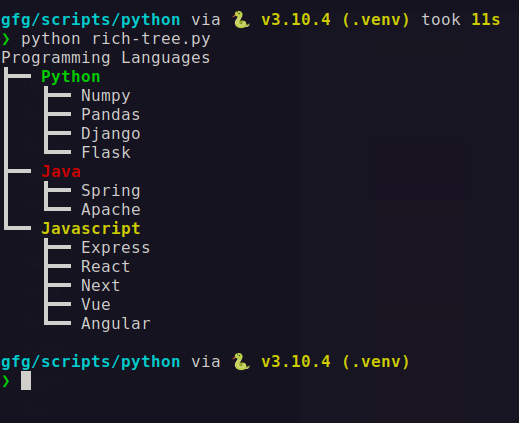
So on this instance, we’ve got used the console class, by including parameters like width which can set the width of the console and the output display screen’s width. Subsequent, we’ll instantiate a tree class that may create a tree object and shops it within the tree variable, we will title it something we wish as we’ve got named it example_tree within the earlier instance. As demonstrated earlier we will add nodes to the tree, we’ll add the node as a brand new tree, so we’ll create nested bushes. By assigning the returned worth of the add operate for the tree variable, we’ve got added a brand new node, we will add nodes additional.
Right here, the tree variable is the basis tree which has nodes like Python, Java, and Javascript with tree class situations as python_tree, java_tree, and js_tree. We have now created the python_tree with nodes like Numpy, Pandas, and Django. The java_tree has nodes like Spring and Apache, and at last, we’ve got js_tree nodes as an inventory of strings which we append dynamically utilizing a for a loop. For every ingredient within the checklist “frameworks”, we add the nodes within the js_tree.
That’s how we will use the tree class in wealthy.
Progress Bar in Wealthy
We are able to even add progress bars within the terminal utilizing wealthy. The wealthy additionally give a category for displaying progress bars utilizing the observe class. The observe class offers a view of progress bars till the loop is full and the given activity has reached 100%.
Python3
|
Output:
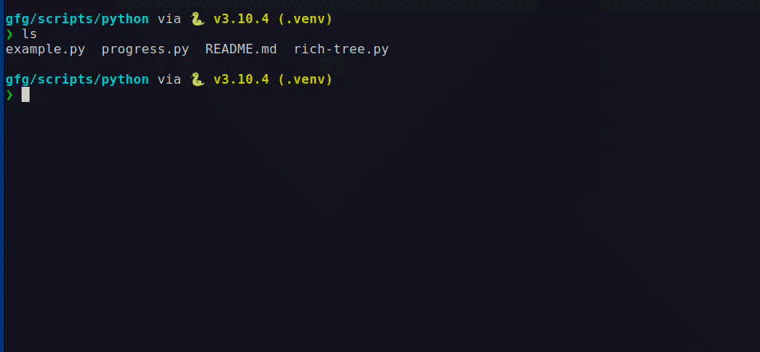
Right here, we’ve got demonstrated a fundamental instance of a easy progress bar for a loop that iterates over a variety of 10 numbers and it sleeps for a second therefore we see the transition of the progress bar.
We are able to even carry out the duty asynchronously and show the progress bar by displaying the love progress.
Python3
|
Output:
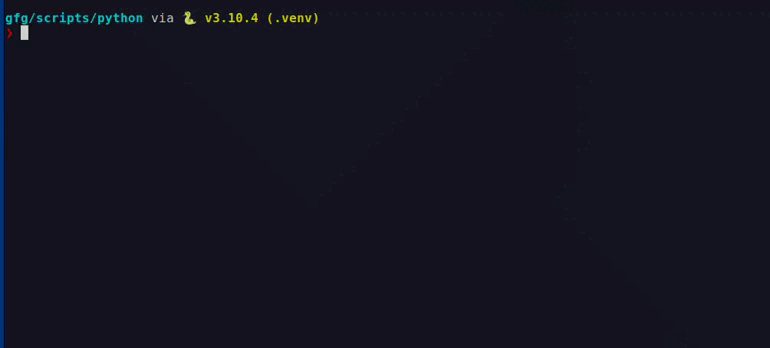
To show a couple of progress bars on the terminal, we will add use the Progress operate within the progress class. We add duties that are particular person progress bars and we increment the present progress, we’ve got included the whereas loop in order that we increment and carry out any operation until the progress bar has been accomplished.
The progress bar is incremented after the duty has been carried out right here the duty is solely sleeping for a fraction of a second however that may be any operation like fetching assets from the web or another logical operation in this system.
Right here, we’ve got added the entire parameter within the progress class. The progress class can be displayed, and the duty is added by the operate add_task which provides a progress bar within the console. The add duties operate takes within the parameter of the duty title and the entire signifies the variety of steps that have to be accomplished for the progress to succeed in 100%.