From closure features and iterators to the State Machine Python library
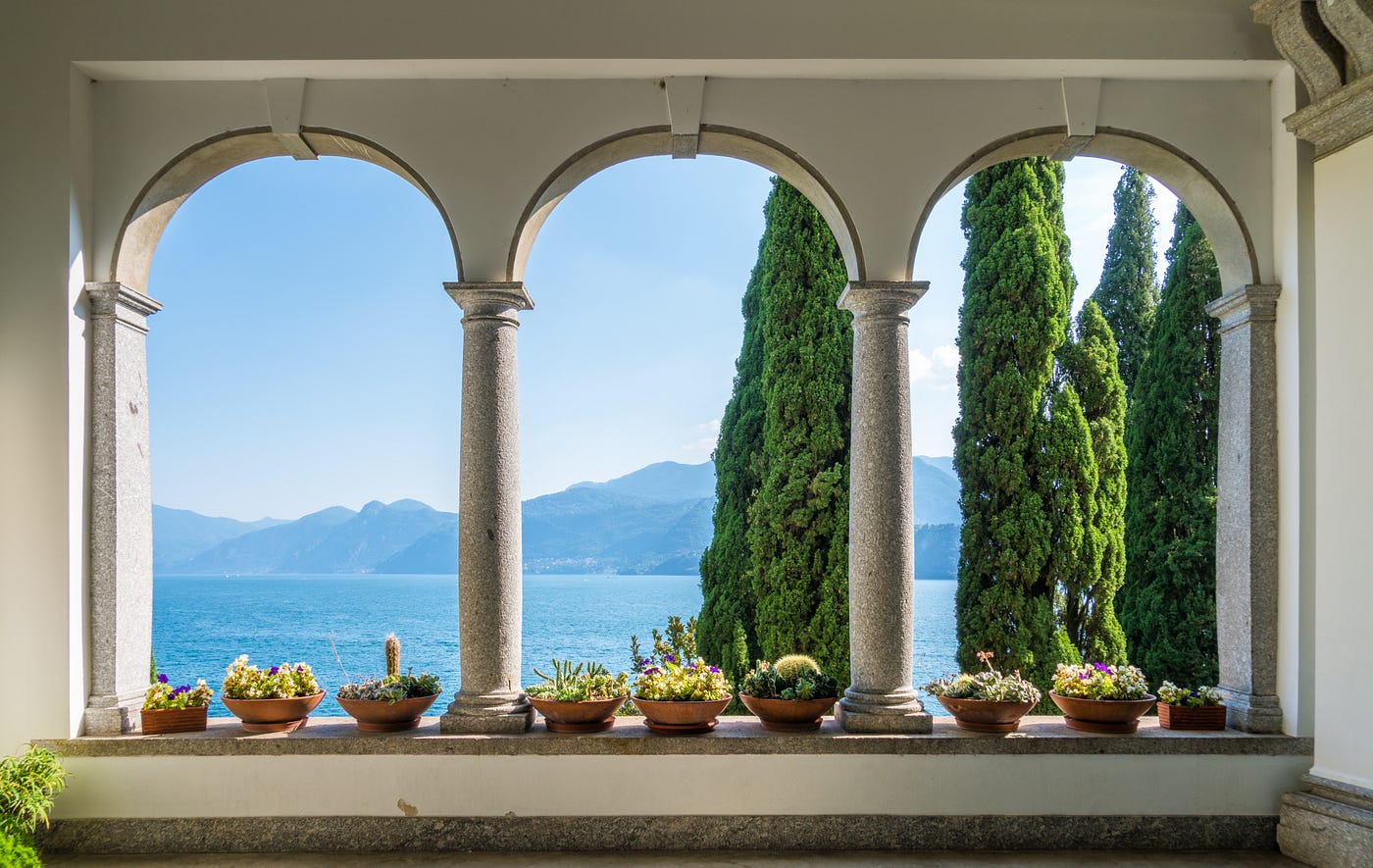
It has been stated that…
“Those that can not bear in mind the previous are condemned to repeat it”. G. Santayana, 1905
Just like in actual life, in software program, you will need to understand how the “state” is preserved, which is perhaps fascinating habits or not. State is the habits of a dynamic system at a sure time. In software program, the mathematical state of a system is modeled by retaining the values of a set of variables of curiosity. In a extra elaborate type, state in software program is modeled via state machines, whose traits are States, Transitions, and infrequently Possibilities of transitioning from one state to a different. Examples of finite state machines (state machines in brief) in machine studying are Markov chains and Markov fashions [1]. State Machines are essential in a wide range of functions:
- Security-critical techniques. Examples embrace medical units[2], energy distribution techniques [3], and transportation techniques, akin to railways [4], airplanes [5], and autonomous driving automobiles [6].
- Blockchain knowledge switch, storage, and retrieval techniques. Blockchain has additionally been known as the Infinite State Machine [7].
- Video gaming [8], conversational AI [9], graphical person interface implementation [10].
- Discovering similarities in protein sequences with BLAST(Primary Native Alignment Search Software) [11].
- Deep State House fashions for time-series forecasting [12].
As each software program developer is aware of, the best (and ugliest) method to have the state of a variable preserved between totally different perform calls is to declare this variable as a international variable. However, allow us to overlook ugly and describe 5 alternative ways to mannequin state in Python, in a use case of managing the friends of a fictional, picturesque, Mediterranean lodge. Our lodge is known as Artemis Cypress (the place Artemis was an historic Greek goddess and cypress was her sacred tree).
In creating performance to handle the lodge friends we are going to look at the next methods to handle state in Python:
- Empty listing as a default perform argument.
- Python Closures
- Iterators
- Class variables
- The State Machine Python library.
1. Empty Lists as Default Operate Arguments
Earlier than we go to our first use case, allow us to be aware the next: Usually, it’s a dangerous concept to have an empty listing as a default perform argument. We are going to clarify why in our use case under.
In our first use case, the lodge’s reservation specialist needs to maintain observe of the rooms which might be reserved by friends with pets, so as to assign further cleansing crews. So each time, a room will get reserved the place the friends have a pet, her software program calls the perform under, roomsWithPet(). The perform takes a positional argument (roomNumber) and a named argument, lr, with a default worth of an empty listing. The perform is known as 3 times, with solely the roomNumber argument. What occurs to lr? The primary time the perform will get referred to as, lr is created and crammed with the worth of roomNumber, and every subsequent time, the brand new roomNumber values are appended to the lr listing created within the first name.
And due to this fact, the output is:
In our case, that is fascinating habits, however in lots of circumstances, the place you desire a new listing to be created at every perform name, it’s not. So, you will need to know that passing an empty listing as a default argument to a perform, retains the state of the listing between perform calls.
2. Python Closures
In our second use case, 4 friends who attended a convention on the lodge arrived on the reception for check-out. The friends are staff of the identical firm and shared a big suite on the lodge. The receptionist must compute the stability due for each. She might name the perform under, with enter arguments the suite quantity (501) variety of nights (3), the suite charge/night time ($400), and the additional bills (meals on the lodge eating places) for each. However it’s a bit cumbersome to name a perform 4 occasions the place solely the final argument is totally different every time.
Python closures supply us a way more elegant method to do that. They’re interior features that may entry values within the outer perform, even after the outer perform has completed its execution [13]. In different phrases, closure features have the flexibility to bear in mind values of an enclosing perform even when these values are now not in reminiscence[14]. Usually, Python closures supply a neat method to do knowledge hiding, when we don’t need to write a category, which is the commonest method to implement knowledge hiding.
Within the code snippet under, the interior perform increaseByMeals() is a closure perform, as a result of it remembers the values of the outer perform balanceOwned(), even after the execution of the latter. So, this enables us to jot down the next code, the place balanceOwned() is known as with its three arguments solely as soon as after which after its execution, we name it 4 occasions with the meal bills of every visitor.
Rather more elegant. The output now could be:
3. Iterators
In our third use case, the friends of the lodge can reserve yoga instructors or bodily trainers for private periods. Within the code under, D is a dictionary the place keys are room numbers and values are “Y” or “PT” (“Y” for requested Yoga teacher and “PT” for requested bodily coach).
Each morning, a brand new dictionary is created, the place the room numbers are entered in response to the time the request was made. Then, so as to get the rooms which have requested Yoga, the following filter() command is executed. And now the central server is prepared for the Yoga instructors. Each time a Yoga teacher requests the following room to go to, the server simply calls subsequent(ff), which returns the following room in line to get a Yoga teacher. On this method, the Yoga instructors don’t want to speak with one another as to which room to go to subsequent. A easy iterator retains the general state of how rooms are served, and the subsequent() command takes us to the following room that must be served.
The output is:
4. Class Variables and State Machine Python Library
On this part, we are going to focus on the final two of the aforementioned methods to retain state in Python:
- Class variables. These are variables which might be outlined inside the category building, declared when the category will get constructed, and shared by all situations of the category. by class situations. If an occasion of a category modifications a category variable, then all situations of the category shall be affected, and on this method, class variables retain their state between class situations.
- The Python State Machine library. That is an open-source library [15] that enables defining states and actions on transitions from state to state.
We are going to reveal the usage of class variables and the Python State Machine library in our closing use case, which fashions the utilization of the sauna rooms in our lodge. The lodge has two sauna rooms at two totally different places, every with a capability for 2 individuals.
Our class Sauna under inherits from StateMachine and has the class variable inst_counter that counts the variety of situations of the category, by being incremented each time the constructor of the category is known as. We outline three states for every sauna room: empty (0 friends in), space_avail (one visitor in), and full (two friends in). Then we give names to the potential transitions. For instance, nospace means now we have transitioned from state space_avail to state full.
Following the definition of transitions, we outline the actions on transitions. For instance, the perform on_enter_space_avail() implements the actions when one visitor is contained in the beforehand empty sauna room. In addition to printing that there’s one sauna seat out there, the perform additionally:
- Increments the occasion variable guestcount. The employees needs to know what number of friends have used every room, to evaluate the recognition of the actual sauna location.
- Will get the present time. That is then appended to the listing entryTimes, which is an occasion variable. Time of entry is beneficial to know in order that at busy occasions, employees can organize for further towels, and so forth.
- Data the room variety of the visitor that’s utilizing the room, by appending it to the listing roomnumbers. That is for billing functions (past two periods/week, which is free, the friends are billed for added periods). In our case, the room quantity is generated randomly. Additionally, it’s fascinating to notice that the roomnumbers listing is a personal member of the category and to entry it we outline the property getroomnumbers.
Lastly, we outline cycle, which is a pipe (sequence) of the states: occupied, nospace, spacefortwo.
Beneath we create an occasion of the Sauna room, and undergo the state transitions, by calling cycle().
The output is proven under. After the primary cycle() name, it’s at state occupied, so it prints “One sauna seat out there”. After the second name, it’s at state nospace, and it prints “Sorry, the Sauna Room is full”. After the third name, it’s at state spacefortwo, and it prints “Welcome to the Sauna Room!”.
Now, allow us to get some extra data: entry occasions of the friends, present state, variety of friends to date, and room numbers.
Beneath is the output of the above code. As anticipated we’re at present in state empty, two friends have used ArtemisSauna1 to date, and we at present have one occasion of the Sauna class.
Python affords a lot of methods to retain state, or in different phrases, maintain observe of the method. That is helpful, significantly for safety-critical techniques, the place the software program concurrently retains observe of the state of many bodily processes and maps state modifications to acceptable actions. Alternatively, as many GUI (graphical person interface) builders will attest, one of the annoying defects is when the GUI doesn’t reply to person motion and as an alternative is locked in a selected state. In conclusion, whether or not our objective is to retain the state or not, we’d like to pay attention to the state-retaining idiosyncrasies of Python.
The code for this text could be discovered within the Github listing: hyperlink to the code
Thanks for studying!
REFERENCES
- Bento, C., Markov Fashions and Markov Chains Defined in Actual Life: Probabilistic Exercise Routine, Medium: In direction of Information Science, 2020, hyperlink to the article
- Bombarda, A., S. Bonfanti, and A. Gargantini, Creating Medical Gadgets from Summary State Machines to Embedded Programs: A Good Capsule Field Case Examine, in Mazzara, M., Bruel, JM., Meyer, B., Petrenko, A. (eds) Software program Expertise: Strategies and Instruments. TOOLS 2019. Lecture Notes in Laptop Science(), vol 11771. Springer, hyperlink to the article
- Zhao, J. et al., Modeling and Management of Discrete Occasion Programs Utilizing Finite State Machines With Variables and Their Functions in Energy Grids, Programs & Management Letters, vol. 61, no.1, pp. 212–222, 2012, hyperlink to the article.
- Thapaliya, A., D. Jeong, D., and G. Kwon, Failure Evaluation in Security-Vital Programs Utilizing Failure State Machine. In: Park, J., Loia, V., Yi, G., Sung, Y. (eds) Advances in Laptop Science and Ubiquitous Computing. CUTE CSA 2017 2017. Lecture Notes in Electrical Engineering, vol 474. Springer, Singapore, 2018.
- Spagnolo, C., S. Sumsurooah and S. Bozkho, Superior Good Grid Energy Distribution System for Extra Electrical Plane utility, Worldwide Convention on Electrotechnical Complexes and Programs , pp. 1–6, 2019, hyperlink to the article.
- Bae, S. H. et al., Finite State Machine primarily based Automobile System for Autonomous Driving in City Environments, twentieth Worldwide Convention on Management, Automation and Programs (ICCAS), pp. 1181–1186, 2020.
- Brooks, S., Blockchain: the Infinite State Machine, Medium: In direction of Information Science, April 2017, hyperlink to the article
- Seemann, G. and D. M. Bourg, AI for Sport Builders, O’Reilly Media, July 2004.
- Zamanirad, S. et al., State Machine Primarily based Human-Bot Dialog Mannequin and Companies, Worldwide Convention on Superior Data Programs Engineering, June 2020, hyperlink to the article.
- Couriol, B., Sturdy Engineering: Person Interfaces You Can Belief with State Machines, April 2019, https://www.infoq.com/articles/robust-user-interfaces-with-state-machines/
- Arpith, J. et al., Mercury BLASTP: Accelerating Protein Sequence Alignment, ACM Trans Reconfigurable Technol. Syst. 2008 Jun; 1(2): 9, hyperlink to the article
- Rangapuram, S. S. et al., Deep State House Fashions for Time Collection Forecasting, NeurIPS Convention, 2018.
- Ramos, L. P., Python Internal Capabilities: What Are They Good for? , hyperlink to the article
- Pathak, O., Python Closures, GeeksforGeeks, hyperlink to the article
- StateMachine Python library, hyperlink to the library.